Making Decisions and Repeating Actions with Control Statements in JavaScript

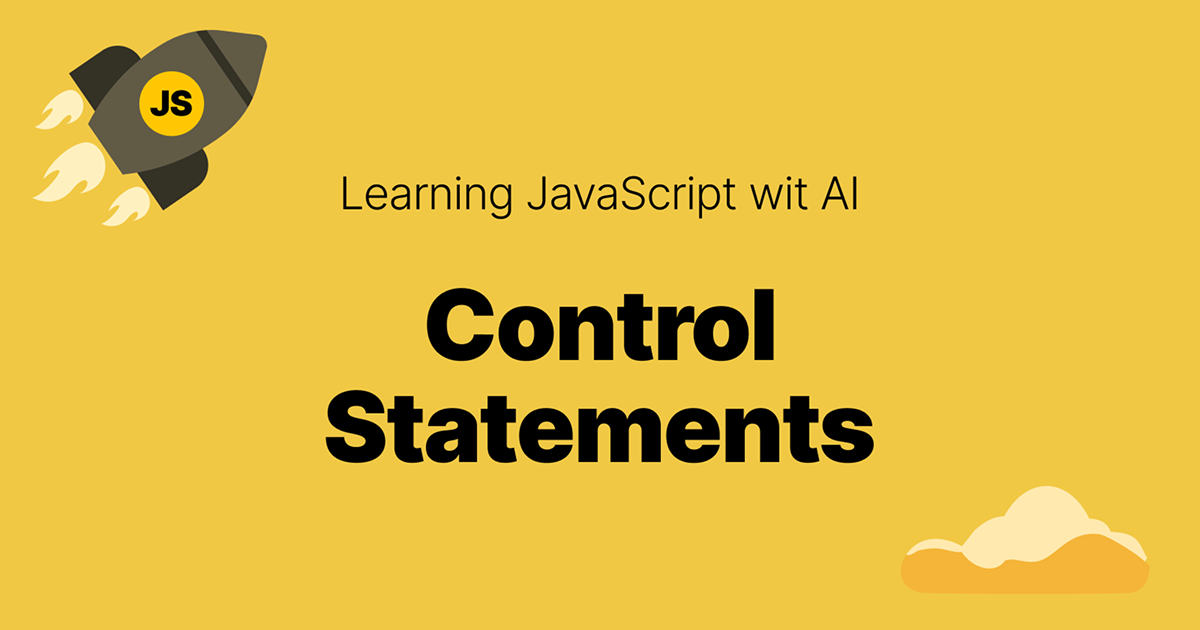
If you’ve ever tried to build something in JavaScript and thought, “How do I make my code choose what to do — or do something over and over?”… you were bumping into control statements.
And if that sounds a bit technical, don’t worry. You’ve probably used this kind of logic in your everyday life without even thinking about it.
“If it’s raining, I’ll bring an umbrella.”
“While the coffee’s brewing, I’ll check my email.”
Those are control statements in plain English — and JavaScript works in a similar way. The difference is, you have to write out the instructions a little more precisely. That’s what this post is all about.
We’re continuing our blog series inspired by the book Learn JavaScript Coding with AI. And today, we’re diving into the part of JavaScript that gives your code the power to think, decide, and repeat — without repeating yourself.
Why are ‘control statements’?
Let’s say you want to build a quiz, and only show the “Congratulations!” message if the user scores above 80. Or maybe you want to loop through a list of questions and show them one at a time.
That’s control statements at work.
They let your code respond to what’s happening — whether that’s a user input, a change in data, or a specific condition being met. And once you get the hang of them, your code will go from rigid and static… to dynamic and interactive.
In this post, we’ll break down four of the most common ones: if, switch, while, and for. Each of them serves a different purpose, but together they form the logic backbone of your JavaScript code.
If statement
Think of if as your code’s way of asking, “Should I do this?” And if the answer is yes, it does it.
Here’s the basic format:
if (condition) {
// code runs if condition is true
}
So if you’re checking someone’s age:
let age = 20;
if (age >= 18) {
console.log("You are an adult.");
}
Want to add more paths? Use else and else if:
let score = 85;
if (score >= 90) {
console.log("A grade");
} else if (score >= 75) {
console.log("B grade");
} else {
console.log("C grade");
}
It’s clear, readable, and helps you avoid writing the same check over and over again.
Generate If statements faster
Typing out multiple conditions — especially for something like a signup form — can get tedious. That’s where AI tools really shine.
Try this prompt in ChatGPT:
“Generate an if statement to validate a signup form. Username must be at least 5 characters. A password must be at least 8. If both are valid, log ‘Signup successful!’”
You’ll get code that works right out of the box, plus you’ll learn from how it’s structured. AI becomes your coding partner, not your crutch.
Switch statement
While if is great for binary choices, switch steps in when you have several options to evaluate — like checking what day it is or which button was clicked.
Here’s what it looks like:
let day = 3;
switch (day) {
case 1:
console.log("Monday");
break;
case 2:
console.log("Tuesday");
break;
case 3:
console.log("Wednesday");
break;
default:
console.log("Invalid day");
}
The break keyword is important. Without it, JavaScript will keep running the next cases even if one has matched.
Example: a simple grading system
Want to build a quick grading system? Here’s how AI can help:
“Write a switch statement for student grades: A, B, C, D, F. Each should print a custom message. Include a default case for invalid input.”
Try pasting that into your AI tool and exploring the output. You’ll get a fast-working version and save yourself the trouble of rewriting repetitive code.
While loops
Sometimes, you need to keep doing something until a condition changes. That’s where while comes in.
let i = 0;
while (i < 5) {
console.log(i);
i++;
}
This will print 0 to 4.
The key thing to remember? Make sure your condition eventually becomes false — otherwise you’ll get an infinite loop.
And if you ever need your code to run at least once before checking the condition, use do…while:
let i = 0;
do {
console.log(i);
i++;
} while (i < 5);
Breaking a loop when you need to
Sometimes you want out — early. Maybe the user input is valid, or the task is complete. Use break to exit a loop mid-way:
let i = 0;
while (true) {
if (i === 3) break;
console.log(i);
i++;
}
This prints 0, 1, and 2 — then stops when i hits 3.
For loops
If while is more “do this until,” then for is “do this X number of times.”
Here’s a classic for loop:
for (let i = 0; i < 5; i++) {
console.log(i);
}
Perfect when you know how many times you need to repeat something.
It’s also great for arrays:
et colors = ["red", "green", "blue"];
for (let i = 0; i < colors.length; i++) {
console.log(colors[i]);
}
More importantly, you’ll see how logic and repetition work together in real applications.
Ready to practice?
This is the perfect moment to pause and play. Try writing a few if statements on your own. Loop through a few arrays. And if you get stuck, use AI as your debugging buddy.
If you’re following along with the book Learn JavaScript Coding with AI, this section is where things start to click. You’re not just writing code — you’re learning to think like a developer.
And once that switch flips, everything gets easier.
This article is a summary of “Learn JavaScript with AI — A Smarter Way to Code” by D-Libro.
Subscribe to my newsletter
Read articles from David Ocean directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
