Interview prep questions I use

Table of contents
- What is SDLC?
- What is a Data Structure?
- What are Binary Trees?
- What are Binary Search Trees?
- What is the difference between Array and LinkedList?
- What is Recursion?
- What is Doubly Linked Lists?
- What is a Graph?
- Differentiate between linear and non-linear data structure?
- Which sorting algorithm is the best?
- How does variable declaration affect memory?
- What are dynamic data structures?
- What is the difference between ArrayList and Vector?
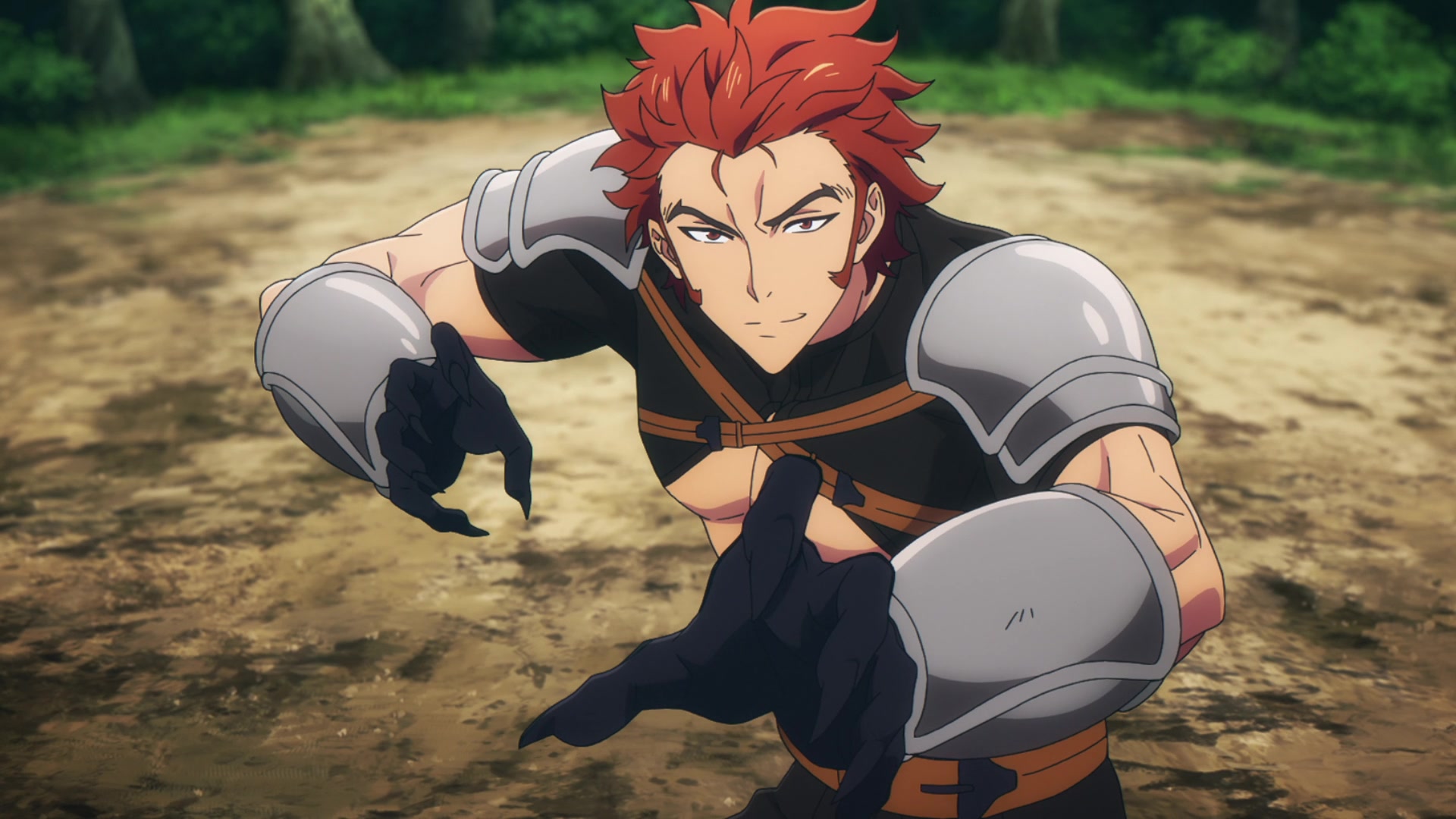
[56]
These are some of the questions I always go back to when I need to prepare for a technical interview. This is not an exhaustive list, so as and when I will encounter anything important that should be included here, I will be doing it.
What is SDLC?
A SDLC or Software Development Life Cycle is a process used to plan and manage the development of a software. The SDLC is a set of activities that are performed over a period of time to ensure that the software is developed in a way that is efficient and effective.
What is a Data Structure?
A Data Structure is a storage format that defines the way data is stored, organized and manipulated. Some popular data structures are Arrays, Trees and Graphs.
What are Binary Trees?
Binary trees are an extension of linked list data structure where each node has two children. It has two nodes at all times, a left node and a right node.
What are Binary Search Trees?
In binary search tree, the left subtree contains nodes whose keys are less than the node’s key value. It stores the data in such a way that it can be retrieved very efficiently.
What is the difference between Array and LinkedList?
An array is a collection of similar elements stored at contiguous memory locations. A linked list, on the other hand is like an array which is a linear data structure in which the elements are not necessarily stored in a contiguous manner. It is basically a sequence of nodes, where each node points towards the next node forming a chain-like structure.
What is Recursion?
Recursion refers to a function calling itself based on a terminating condition. It uses LIFO and therefore makes use of the stack data structure.
What is Doubly Linked Lists?
Doubly Linked Lists are a special type of linked lists in which traversal across the data elements can be done in both directions.
What is a Graph?
It is a type of data structure that contains a set of ordered pairs, these ordered pairs are also referred to as edges or arcs and are used to connect nodes where data can be stored and retrieved.
Differentiate between linear and non-linear data structure?
Linear Data Structure: In this, data elements are adjacent to each other. Examples are Arrays, Linked Lists, Stacks and Queues.
Non-Linear Data Structure: In this, each data element can connect two or more than two adjacent data elements. Examples are Trees and Graphs.
Which sorting algorithm is the best?
There are many types of sorting algorithms: Quick Sort, Bubble Sort, Balloon Sort, Radix Sort, Merge Sort, etc. But no algorithm can be considered as the best or fastest because each is designed for a specific type of DS where it performs the best.
How does variable declaration affect memory?
The amount of memory to be allocated or reserved depends on the data type being stored in that variable. For example, if a variable is declared to be of integer type, then 32 bits of memory storage will be reserved for that variable.
What are dynamic data structures?
Dynamic data structures are structures that expand and contract as a program runs. It provides a flexible means of manipulating data because it can adjust according to the size of the data.
What is the difference between ArrayList and Vector?
ArrayList is not synchronized, it needs to be synchronized explicitly using
Collections.synchronizedList
.While, Vector is synchronized.
Since, ArrayList is not synchronized it is not Thread Safe and on the other hand, Vector becomes Thread Safe.
Furthermore, since ArrayList is not Thread Safe multiple thread can work together making it faster in execution and on the other hand, in Vector only one thread will work at a time since it is Thread Safe making it slower.
Subscribe to my newsletter
Read articles from Pranav Bawgikar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Pranav Bawgikar
Pranav Bawgikar
Hiya 👋 I'm Pranav. I'm a recent computer science grad who loves punching keys, napping while coding and lifting weights. This space is a collection of my journey of active learning from blogs, books and papers.