What are React Hooks ??

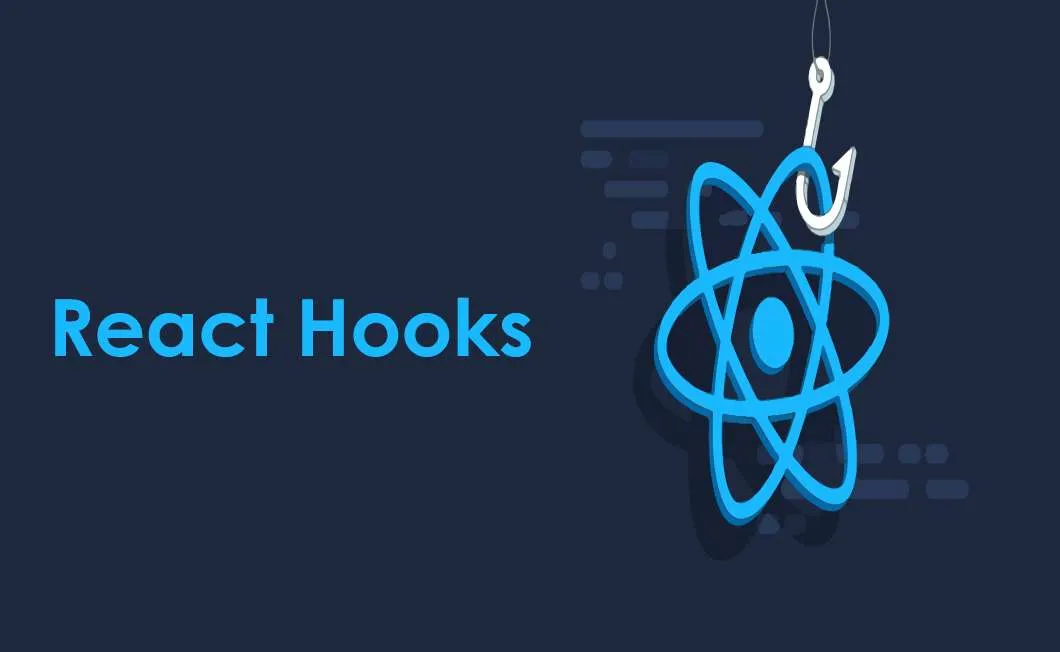
React Hooks allow you to use state and lifecycle features in functional components without writing class components. Introduced in React 16.8, Hooks make React development more efficient and readable.
Letβs break down the most important Hooks and how to use them! π
πΉ 1. useState β Managing State
π useState
lets you add state inside a functional component.
Example: Counter App
import { useState } from "react";
export default function Counter() {
const [count, setCount] = useState(0);
return (
<div>
<h1>Count: {count}</h1>
<button onClick={() => setCount(count + 1)}>Increment</button>
</div>
);
}
πΉ How it works?
count
β Holds the state valuesetCount
β Updates the stateuseState(0)
β Initializescount
to 0
πΉ 2. useEffect β Side Effects in Components
π useEffect
runs after the component renders. It's used for fetching data, updating the DOM, or setting up event listeners.
Example: Fetching Data
import { useState, useEffect } from "react";
export default function Users() {
const [users, setUsers] = useState([]);
useEffect(() => {
fetch("https://jsonplaceholder.typicode.com/users")
.then((res) => res.json())
.then((data) => setUsers(data));
}, []); // Empty array means it runs only on mount
return (
<ul>
{users.map((user) => (
<li key={user.id}>{user.name}</li>
))}
</ul>
);
}
πΉ How it works?
Runs once when the component mounts (because of
[]
)Calls API and updates
users
state
Variants of useEffect:
useEffect(() => { ... })
β Runs on every renderuseEffect(() => { ... }, [])
β Runs only once (on mount)useEffect(() => { ... }, [count])
β Runs when count changes
πΉ 3. useRef β Accessing DOM Elements & Preserving Values
π useRef
is used for getting a reference to a DOM element or storing values without causing re-renders.
Example: Focusing an Input
import { useRef, useEffect } from "react";
export default function FocusInput() {
const inputRef = useRef(null);
useEffect(() => {
inputRef.current.focus(); // Auto-focus input field on mount
}, []);
return <input ref={inputRef} type="text" placeholder="Type here..." />;
}
πΉ How it works?
useRef(null)
β Creates a mutable referenceinputRef.current
β Directly accesses the input
πΉ 4. useCallback β Optimizing Functions
π useCallback
memoizes functions so they donβt get re-created on every render, improving performance.
Example: Avoid Unnecessary Re-renders
import { useState, useCallback } from "react";
export default function Counter() {
const [count, setCount] = useState(0);
const increment = useCallback(() => {
setCount((prev) => prev + 1);
}, []);
return (
<div>
<h1>{count}</h1>
<button onClick={increment}>Increment</button>
</div>
);
}
πΉ Why use useCallback
?
Prevents
increment
from recreating on each renderImproves performance in child components
πΉ 5. useMemo β Optimizing Expensive Calculations
π useMemo
caches a computed value so it doesnβt re-calculate unnecessarily.
Example: Expensive Calculation
import { useState, useMemo } from "react";
export default function ExpensiveCalculation() {
const [number, setNumber] = useState(0);
const squared = useMemo(() => {
console.log("Calculating square...");
return number * number;
}, [number]);
return (
<div>
<h1>Square: {squared}</h1>
<button onClick={() => setNumber((prev) => prev + 1)}>Increase</button>
</div>
);
}
πΉ How it works?
Only re-computes when
number
changesAvoids unnecessary calculations
πΉ 6. useContext β Global State Management
π useContext
helps share global state without prop drilling.
Example: Theme Context
import { createContext, useContext } from "react";
const ThemeContext = createContext("light");
export function ThemeProvider({ children }) {
return <ThemeContext.Provider value="dark">{children}</ThemeContext.Provider>;
}
export function ThemedComponent() {
const theme = useContext(ThemeContext);
return <h1>Current Theme: {theme}</h1>;
}
πΉ Why use useContext
?
Avoids prop drilling
Shares state across multiple components
π Other Useful Hooks
useReducer
β LikeuseState
, but for complex state logicuseImperativeHandle
β Exposes methods from a child componentuseLayoutEffect
β LikeuseEffect
, but runs before the screen updates
π― Final Thoughts
Hooks simplify React development by making functional components more powerful. Mastering them will help you write cleaner, reusable, and optimized React apps!
π‘ Now, go build something amazing with React Hooks! π
Got questions? Drop them below! β¬οΈ Happy coding! π
Subscribe to my newsletter
Read articles from Deepak Modi directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Deepak Modi
Deepak Modi
Hey! I'm Deepak, a Full-Stack Developer passionate about web development, DSA, and building innovative projects. I share my learnings through blogs and tutorials.