Creating A Router In Vanilla Javascript

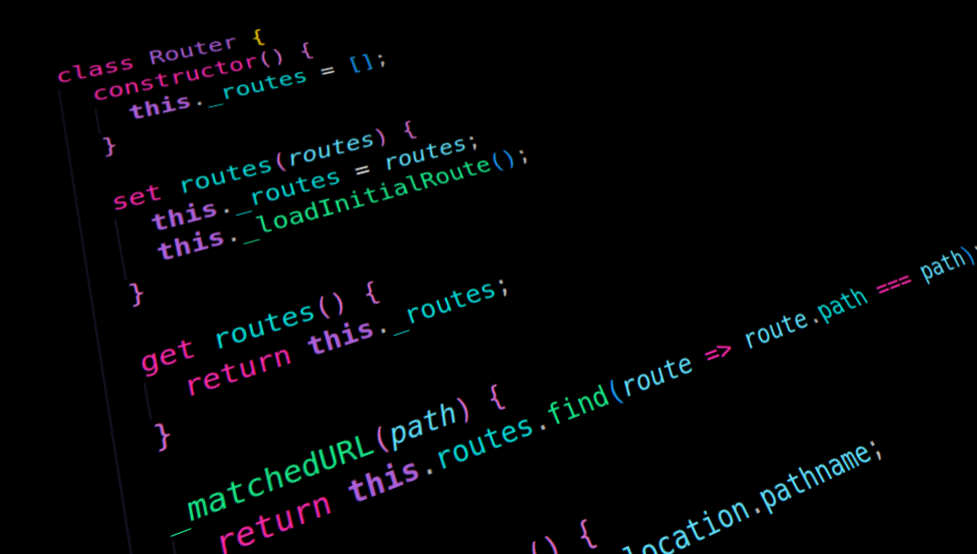
Navigation is one of the biggest challenges when dealing with a single-page application. You need to ensure that the user does not notice any difference in the website's behavior compared to that of a multi-page application.
Any links that redirect the user within the application must be handled so that they do not cause page reloads but render the desired component instead.
Nowadays, we have frameworks like React that provide libraries such as React Router that handle the challenge very efficiently without us even having to think about it.
Routing must be handled manually when a framework is not used, and even with one, understanding basic SPA routers is valuable and insightful.
📋 Prerequisites
To understand the working of this router, you need to have some basic knowledge of the below-mentioned topics:
Vanilla Javascript fundamentals (like functions, objects, arrays, and strings)
window.location property
🔍 An overview
The most basic requirement for routing is routes. We must tell the router which routes to look for and what to do upon reaching that route. This can be achieved by having a data structure to store the routes and their corresponding callback functions.
Since a route will have its path and a callback function to be called on that path, we can create key-value pairs for both of these properties and store them in an object. Each route will be an object.
The router needs to iteratively check all the routes to match the current routes with the existing ones. Therefore, we should store these route objects in an iterable data structure. We can create an array of objects for this.
Hence, we need to have a predefined array of routes. This array will contain objects. Each object will have a route and a callback function.
This callback function triggers every time a user navigates to a route, rendering the desired component. Below is an example of the predefined routes.
const routes = [
{ path: '/', callback: () => setUpHomeView() },
{ path: '/bookmarks', callback: () => setUpBookmarksView() },
{ path: '/book', callback: () => setUpBookView() },
{ path: '/error', callback: () => setUpErrorView() },
];
Now that the router has route information, it needs to perform routing. That means it should look for the current route, match it with the existing routes, load a route, or handle any errors (such as route not found).
The routing process can be divided into the following tasks: finding the current route, matching routes, loading a route by calling its callback method, handling event listeners or errors, etc.
Hence, we can create separate methods for each task, which collectively perform routing.
Since all these methods are related, we can use a Javascript class to create our router‘s instance. Javascript classes provide a blueprint for creating objects with similar methods, which perfectly suits our router’s requirements.
🔀 Creating the router class
Constructor
Let us begin with the constructor. Whenever the router’s instance is created, we want to set an empty array named routes to prevent any unexpected behavior due to an undefined routes array. We will add the routes later using a setter method.
We need a separate setter method to set the routes rather than using the constructor itself because we will define our routes outside the router class (in a different module) but we will initialize the router in the same module (where the class exists). This allows us to handle the routes more dynamically since they are not hard-coded in the class.
We create a router object right after finishing the class and export it.
class Router {
// Initialize with an empty routes array
constructor() {
this.routes = [];
}
}
export default new Router();
Setting the routes
We will set the routes using a setter method routes
.
The setter will receive the routes array and assign it to the _routes
variable. Together with the assignment of the routes, we also call a method, _loadInitialRoute()
.
We can also create a getter method to fetch the routes for consistency.
set routes(routes) {
this._routes = routes;
this._loadInitialRoute();
}
get routes() {
return this._routes;
}
The _
in front of a variable shows that the particular variable is private and should not be directly accessible outside the router’s class.
Handling the initial route
After getting the predefined routes, the router should fetch the current URL from the browser, which will be the initial route.
There will be two ways through which the navigation links in the browser will change. First, via user events like clicks from within the web application, using one of the router’s methods, and second, through window events such as popstate
or the landing URL itself.
Here’s the _loadInitialRoute
method.
_loadInitialRoute() {
// Get the current path
const path = window.location.pathname;
this._loadRoute(path);
}
We get the current route by using the window.location
object. We can easily access its pathname property to fetch the current path. We then call another method _loadRoute(path)
and pass the path to it for further processing.
The initial route method is crucial as this works on URLs that are not set via the router. Meaning, this method should be triggered when the application is initialized or when the popstate
event is triggered (the user moves back or front via the browser’s navigation buttons).
We called the _LoadInitialRoute
method via the setter method, which handles the case where the application is initialized. We will need to add an event listener to the popstate
event to handle the case where the user manually navigates through the browser’s navigation buttons.
addHandlerRouter() {
window.addEventListener('popstate', () => this._loadInitialRoute());
}
}
We can call the method wherever we import the router object. I used a generic name rather than a specific one (like addHandlerInitialRoute
) so that if the router is modified, other event listeners can be added using the same method.
Notice that this is not a private method since it doesn't begin with an underscore (_
). This is because we need to access this outside our router’s class, where we import the router’s instance.
Loading routes
We handled the case where a user navigates through the browser or loads a specific URL. We are yet to handle navigation from within the web application via clicks using one of the router methods. First, let us continue with the flow of the previous function and understand how a route is loaded, irrespective of where it was initialized.
Here’s the _loadRoute
method.
_loadRoute(path) {
// Checking if the provided route exists in the predefined routes
const matchedRoute = this._matchedURL(path);
// If the route doesn't exist, throw error
if (!matchedRoute) throw new Error('Route Not Found');
// Calling the callback function that we set with the predefined route
matchedRoute.callback();
}
We check if the provided route exists. For this, we use another method called _matchedURL(path)
, and pass it the path.
It checks for the existence of the provided route with the predefined routes and returns the object of the matched route. If the route is not matched, we throw an error instead.
This is what a matched route would look like:
{ path: '/book', callback: () => setUpBookView() }
This is one of the objects we stored in our predefined routes. Its path property was matched with the provided route. This is stored in the matchedRoute
variable.
We then call the callback function matchedRoute.callback()
, which will handle whatever needs to be done on that particular route.
Matching the routes
Let us have a quick look at the _matchedURL
method we used before to get the matched route.
_matchedURL(path) {
return this.routes.find(route => route.path === path);
}
The method loops over the predefined routes with the help of the find
method and returns the matched route object if found.
Handling the navigation through the router
We are now familiar with how the router processes a route. We also handled the URL changes when a user navigates via browser navigation buttons or on the application’s initialization.
The final and important case to handle is navigation upon user-generated events from within the web application, such as clicking a button in the navigation bar.
We need to add event listeners to the elements that trigger navigation. If the element is an anchor tag (<a></a>
) then do not forget to prevent its default behavior using e.preventDefault()
to prevent page loads.
Here is the navigateTo
method that will trigger the navigation.
navigateTo(path) {
window.history.pushState('', '', path);
const splitPath = path.split('?');
if (splitPath.length > 1) {
path = splitPath[0];
}
this._loadRoute(path);
window.scrollTo({
top: 0,
left: 0,
});
}
The method will receive a path. It will start by utilizing the pushState
method of the history
API to add the path to the browser’s history.
We need to load this path using the loadRoute
method, but there is one additional step here compared to the loadInitialRoute
method. Before the route is passed to the loadRoutes
method, we ensure no query parameters are attached to the path string.
The router will always throw an error if we load a route with query parameters, since there are no query parameters in the predefined routes. Therefore, we check for any existing query parameters and remove them from the path string since they are useless for this router.
The splitPath
variable stores an array in which the path is available in a split form. The first index contains the actual pathname and the second index stores the rest of the string with the query parameter. If the splitPath’s
length is 1 or less, then it simply means query parameters do not exist, and we can proceed with directly loading the path.
After handling query parameters, we call the loadRoutes
method and pass the path. We should also scroll the window to the top every time we use this navigateTo
method to mimic a new page load.
A side note:
You may have noticed that I did not handle any query parameters in the loadInitialRoute
method, which also calls the loadRoute
method, just like the navigateTo
method does.
This is because the loadInitialRoute
method uses window.location.pathname
. The pathname
property of the location object only returns a pathname without its query parameters and hence the location object already handled the query parameters for us.
Since we manually pass the routes to the navigateTo
method, handling the query parameters will ensure correct working even if a route with query parameters is passed.
The final code
Here I am providing the whole router class altogether.
class Router {
constructor() {
this._routes = [];
}
set routes(routes) {
this._routes = routes;
this._loadInitialRoute();
}
get routes() {
return this._routes;
}
_matchedURL(path) {
return this.routes.find(route => route.path === path);
}
_loadInitialRoute() {
const path = window.location.pathname;
this._loadRoute(path);
}
_loadRoute(path) {
const matchedRoute = this._matchedURL(path);
if (!matchedRoute) throw new Error('Route Not Found');
matchedRoute.callback();
}
navigateTo(path) {
window.history.pushState('', '', path);
const splitPath = path.split('?');
if (splitPath.length > 1) {
path = splitPath[0];
}
this._loadRoute(path);
window.scrollTo({
top: 0,
left: 0,
});
}
addHandlerRouter() {
window.addEventListener('popstate', () => this._loadInitialRoute());
}
}
export default new Router();
How to use the router
Now we have all the logic we need to run the router. The router object is initialized and exported directly from the router module, where the class is written.
Import the router to the module where you want to use the router’s public methods, such as the setter method, addHandlerRouter
and the navigateTo
method.
// Import the router
import Router from './router';
const controlRouter = function () {
// Define the routes of your project
const routes = [
{ path: '/', callback: () => setUpHomeView() },
{ path: '/bookmarks', callback: () => setUpBookmarksView() },
{ path: '/book', callback: () => setUpBookView() },
{ path: '/error', callback: () => setUpErrorView() },
];
// Add the routes to the router using the setter method
Router.routes = routes;
// Add event handler to the desired events
Router.addHandlerRouter();
};
// A single function to initialize your project where you can initialize the router
const init = function () {
controlRouter();
// Any other initialization functions of your projects
}
init();
The above is just an example of how to use the router. It is based on the module system but you can modify its usage according to your needs.
In the example, a controlRouter
function defines the routes, sets the routes to the router, and adds the event handlers to the desired events. This function can be called together with other initialization functions of your project.
Here’s an example of how the navigateTo
method can be used. You can use the method to suit your needs.
try {
// Fetch some data and navigate the user to a component that displays the data
Router.navigateTo('/book');
} catch (error) {
// ... error handling
// Navigate to the error page on encountering an error
Router.navigateTo('/error');
}
The method makes it easy to handle navigation throughout the application with simplicity.
🚧 Some challenges that I faced, and you can avoid
Before I conclude this blog, I would like to mention a few challenges I faced while working with the router. This can help you avoid them and give you a great head start for your project.
Using hash
to display unique components
I was working on a project where you can search and share books with some more additional features. To display those books, I was using the URL hash.
I attached a hash with the book’s ID to the current URL. I added an event handler on hash change that would load the book component whenever the hash change event is triggered. This was working fine until my project got more complex.
As soon as I added more routes, this way turned into a disaster.
Every time there was a hash change event, this function to load the book component would be triggered.
The route did not matter, I could put this hash with the book’s ID to any route and it would display the component.
The router helped solve this issue. I easily created a /book
route to provide a separate route to my unique book component. Now I placed a hash on the /book
route to display the book component.
But this did not solve the whole problem; I could still place the hash anywhere, and it would load the component, and any kind of hash change event would trigger the book component load.
The only solution was not to depend on the hash event, which brings us to my next challenge.
Using query parameters
I wasn’t aware of how query parameters work until I faced the issue with the hash change event. These were a game-changer for me.
I modified the router to handle any query parameters before loading a route. This helped me to avoid the use of a hash change event to load my unique components.
This is why the navigateTo
method of this router specifically handles the query parameters.
Handling router’s history
After learning about query params, I was using them to handle my collections. All the collection data would be stored in a Javascript object, and upon opening a collection, this data would be added to the URL using query parameters and then displayed on the screen.
I did this through URLs because my collections had to be shareable, hence I decided to store all their data in the URL itself. The user could simply use the direct URL for sharing the collection.
But this created two major issues. First, the URLs became too long to be shared after adding a few books. Apps like WhatsApp would break the URLs in the chats.
Second, the browser would add these URLs with collection data to the history. Whenever a book was deleted, it was removed from the URL. But since the browser was adding the URL to history, upon going back, it would display the collection with the deleted book as well.
This is where I realized I need a database to make the sharing feature work seamlessly, and hence I chose to use Supabase to store my collection’s data.
🔮 Future improvements
Dynamic routes
The current version of the router can handle any query parameters like /book?id=someId
. This is a static route. A better way to implement this would be via dynamic routing.
A dynamic route for the same would look like /book/:id
.
This improves the URL readability and helps with caching and SEO optimization.
Readability: The route
/book/123
looks more readable and intuitive as compared to the route/book?id=123
.Caching: Browsers may not cache the static route
/book?id=123
and/book?id=456
as unique routes since they both denote the same route with different parameters. Dynamic routes like/book/123
and/book/456
can help the browser uniquely identify these routes.SEO Optimization: SEO crawlers may treat a static route
/book?id=123
less significantly when compared to a dynamic route/book/123
since a dynamic route can tell it is a unique route but a static route cannot define its uniqueness.
A query parameter is helpful in the case of storing search queries and filters since this data is only used to render the component with the stored data, but the component is not dependent on this data.
But for a component that depends on the data, such as the book’s ID to render the book, this should be handled via dynamic routing.
Route guards
A route guard can help secure routes that contain sensitive data. Routes such as /admin
should check for user authentication and user role. The current router cannot be used for routes with sensitive data.
When navigating to such routes, there should be a way to identify whether the user is authenticated and what is the role of the user. Implementing a route guard can allow the router to handle routes with sensitive data.
Error handling
You can easily modify this router to handle the case when a route is not found. You can choose to simply display an error page or any other way that suits your application.
The _loadRoute
and the _navigateTo
methods can help implement error handling for not-found routes. Below is an example.
_loadRoute(path) {
try {
const matchedRoute = this._matchedURL(path);
if (!matchedRoute) throw new Error('Route Not Found');
matchedRoute.callback();
} catch (err) {
console.error(err);
this.navigateTo('/page-not-found');
}
}
We can modify the _loadRoute
method to follow a try-catch
block implementation. After catching the error in the catch block, we can implement the error handling logic, such as using the navigateTo
method to redirect users to an existing path that tells them that the route did not match using a clean UI.
The reason this section is in future improvements is that the error handling can be even more robust. The above is just a small example. A router should handle more cases, such as:
User Authentication
Invalid dynamic route parameter
Undefined callbacks of defined routes
The final code did not include this part, so you can customize it with your error handling method.
✅ Conclusion
Routing is one of the key roles that frameworks assist us with. Understanding how a router works is essential to realizing what frameworks do behind the scenes. Implementing such a router in vanilla JS can help you understand the working with a practical implementation.
This is a basic version of a router. It only handles static routes, but it is good enough for a simple SPA project and gives you a great head start to develop a solid understanding of routers.
Further, you can try to implement your error-handling logic in this router or maybe something more complex from the future improvements section. This can help you understand the logic in more depth.
I would love to see any further modifications you implement in this router. Please do share with me. Happy Coding!
🚀 Check out the router’s practical implementation
Here’s the project where I implemented routing using this router. Please check it out, I would love to hear your thoughts on it.
📚Shelf Share: Search, Share, and Bookmark Books
Here’s the GitHub repository. Please do star it if you liked the project!
References
Subscribe to my newsletter
Read articles from Pranav Patani directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
