How to generate and save a folder structure in YAML/JSON/text format using Node.js?

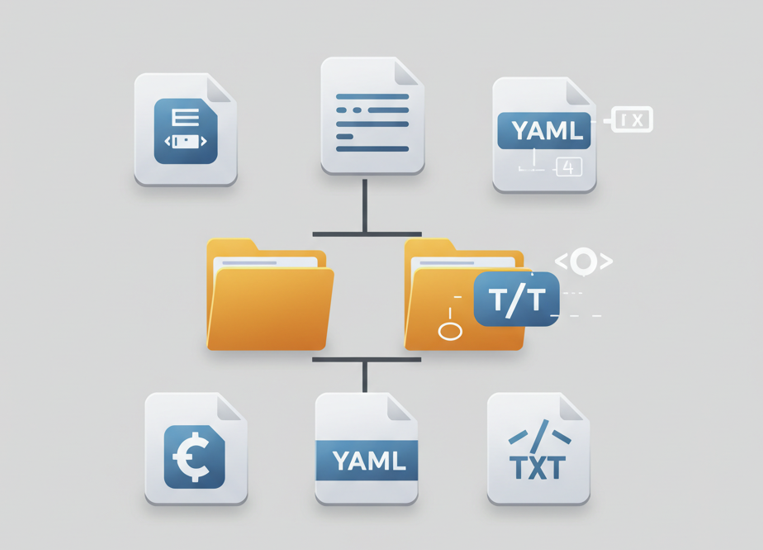
I created a Node.js script that:
Recursively scans a directory and lists all files and folders.
Excludes unnecessary directories like
node_modules
,.git
,dist
, etc.Outputs the structure in different formats (text, JSON, and YAML).
Uses separate scripts for each format instead of one script handling all.
I created three separate Node.js scripts to generate and save the folder structure in TXT, JSON, and YAML formats:
Save Folder Structure as text (
generate_txt.js
)import { readdirSync, statSync, writeFileSync } from "fs"; import { join } from "path"; const EXCLUDED_DIRS = new Set(["node_modules", ".git", "dist", "build", "out"]); /** * Recursively retrieves folder structure and formats it as a TXT hierarchy. */ function getFolderStructure(dir, prefix = "") { let structure = ""; const items = readdirSync(dir).filter( (item) => !EXCLUDED_DIRS.has(item) && !item.startsWith(".") ); items.forEach((item, index) => { const fullPath = join(dir, item); const isDir = statSync(fullPath).isDirectory(); const isLast = index === items.length - 1; structure += `${prefix}${isLast ? "└── " : "├── "}${item}${ isDir ? "\\" : "" }\n`; if (isDir) { structure += getFolderStructure( fullPath, prefix + (isLast ? " " : "│ ") ); } }); return structure; } // Get directory to scan const rootDir = process.argv[2] || process.cwd(); const outputFile = process.argv[3] || "folder_structure.txt"; // Generate structure and save const folderStructure = getFolderStructure(rootDir); writeFileSync(outputFile, folderStructure, "utf8"); console.log(`Folder structure saved to ${outputFile}`);
Save Folder Structure as JSON (
generate_json.js
)import { readdirSync, statSync, writeFileSync } from "fs"; import { join } from "path"; const EXCLUDED_DIRS = new Set(["node_modules", ".git", "dist", "build", "out"]); /** * Recursively retrieves folder structure as a JSON object. */ function getFolderStructure(dir) { let structure = {}; const items = readdirSync(dir).filter( (item) => !EXCLUDED_DIRS.has(item) && !item.startsWith(".") ); items.forEach((item) => { const fullPath = join(dir, item); const isDir = statSync(fullPath).isDirectory(); structure[item] = isDir ? getFolderStructure(fullPath) : null; }); return structure; } // Get directory to scan const rootDir = process.argv[2] || process.cwd(); const outputFile = process.argv[3] || "folder_structure.json"; // Generate structure and save const folderStructure = getFolderStructure(rootDir); writeFileSync(outputFile, JSON.stringify(folderStructure, null, 2), "utf8"); console.log(`Folder structure saved to ${outputFile}`);
Save Folder Structure as YAML (
generate_yaml.js
)import { readdirSync, statSync, writeFileSync } from "fs"; import { join } from "path"; const EXCLUDED_DIRS = new Set(["node_modules", ".git", "dist", "build", "out"]); /** * Recursively retrieves folder structure and converts it to YAML format. */ function getFolderStructure(dir, depth = 0) { let structure = ""; const items = readdirSync(dir).filter( (item) => !EXCLUDED_DIRS.has(item) && !item.startsWith(".") ); items.forEach((item) => { const fullPath = join(dir, item); const isDir = statSync(fullPath).isDirectory(); structure += `${" ".repeat(depth)}${item}: ${isDir ? "" : "null"}\n`; if (isDir) { structure += getFolderStructure(fullPath, depth + 1); } }); return structure; } // Get directory to scan const rootDir = process.argv[2] || process.cwd(); const outputFile = process.argv[3] || "folder_structure.yaml"; // Generate structure and save const folderStructure = getFolderStructure(rootDir); writeFileSync(outputFile, folderStructure, "utf8"); console.log(`Folder structure saved to ${outputFile}`);
How to Use These Scripts?
Save each script separately as:
generate_txt.js
generate_json.js
generate_yaml.js
Run them using the terminal:
node generate_txt.js /path/to/directory output.txt node generate_json.js /path/to/directory output.json node generate_yaml.js /path/to/directory output.yaml
If no directory is specified, it defaults to the current working directory.
If no output file is specified, it defaults to
folder_structure.{format}
.
Example Outputs:
Text Output (
folder_structure.txt
)app\ about\ page.tsx admin\ customers\ page.tsx
JSON Output (
folder_structure.json
){ "app": { "about": { "page.tsx": null }, "admin": { "customers": { "page.tsx": null } } } }
YAML Output (
folder_structure.yaml
)app: about: page.tsx: null admin: customers: page.tsx: null
Subscribe to my newsletter
Read articles from Vinay Patel directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Vinay Patel
Vinay Patel
Hi, My name is Vinay Patel, and I'm an aspiring software developer.