Redis for Pub/Sub Messaging
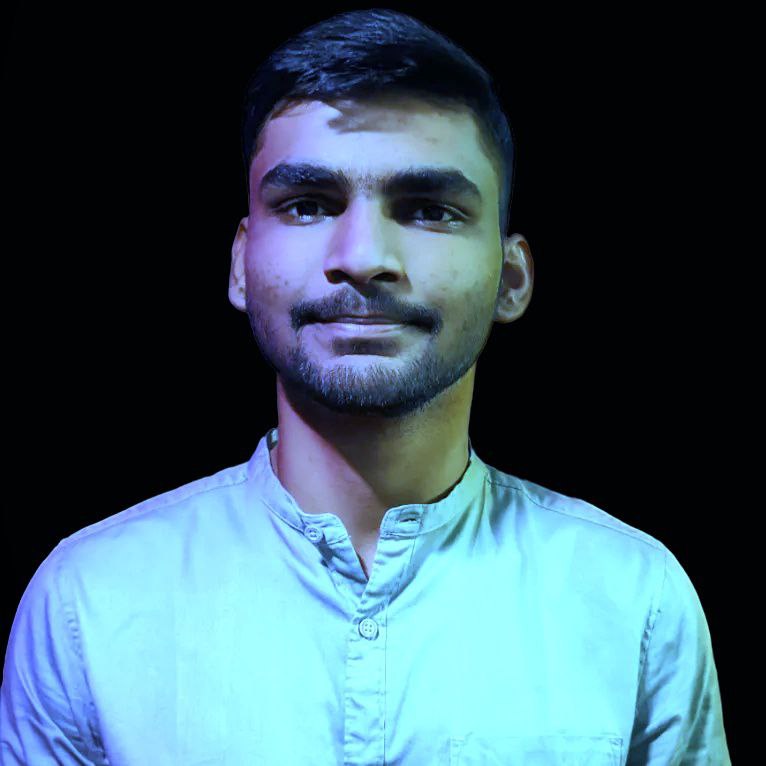
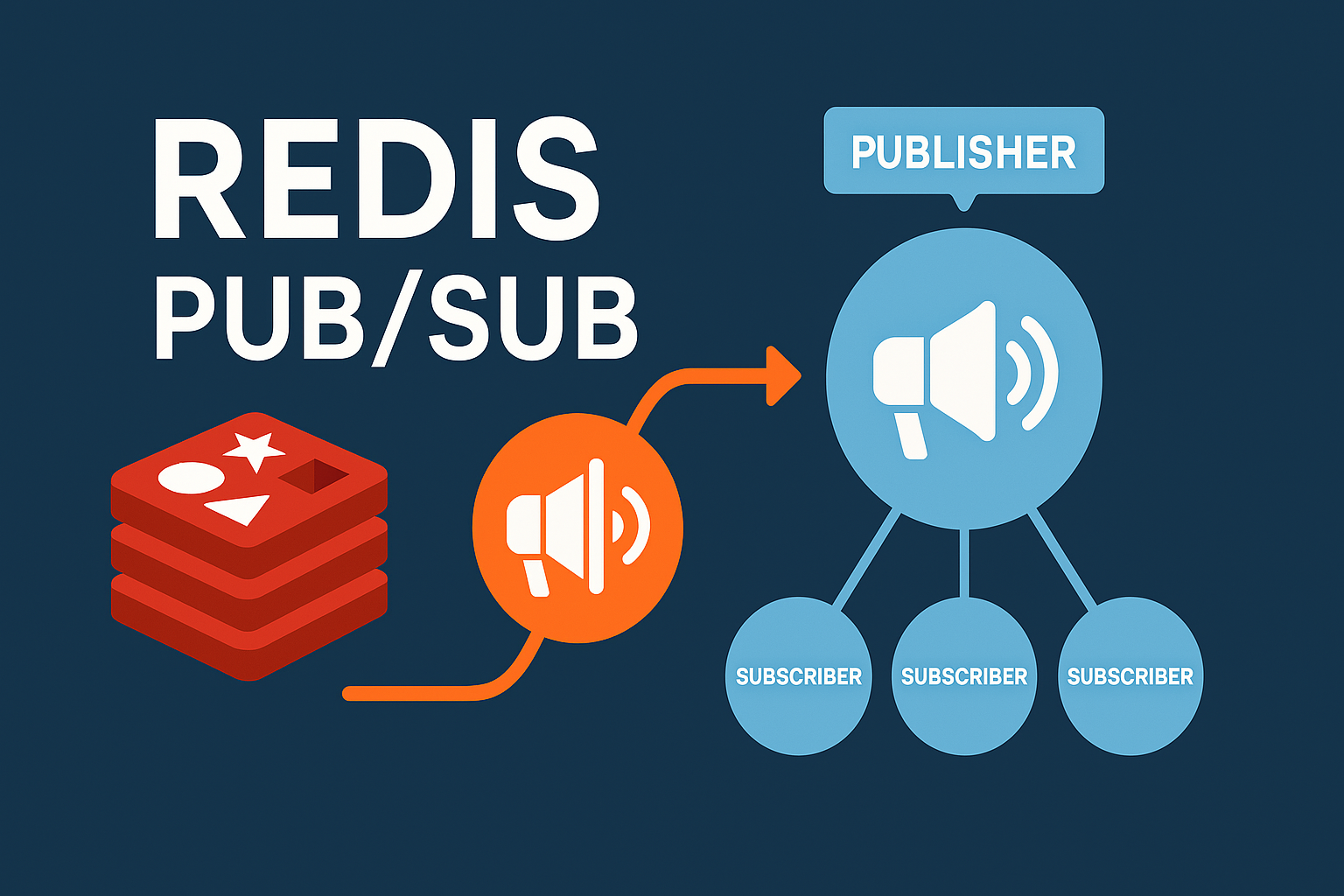
Introduction
This blog is second installment of Redis in Action blog series and if you’ve read the first blog on Ratelimiting using Redis, you are aware of what Redis is and how it works as a versatile in-memory data store. Today we’re diving into one of the Redis’s most elegant features: Pub/Sub Messaging.
In the world of microservices, communicatio is everything. Services need to talk to each other without becoming tightly coupled, maintain stability under load and often exchange information in realtime. This is where Publish/Subscribe (Pub/Sub) pattern shines.
Pub/Sub is a messaging pattern where senders or publishers don’t send messages directly to the receivers. Instead, they publish messages to channels without knowledge of which subscribers might be listening. This decoupling is particularly valuable in distributed systems. For example, Reddit works on a similar pattern, where publishers posts in a subreddit (channel) without knowing which user or receiever will get that post and read it.
Redis offers a lightweight, blazing fast implementation of this pattern that can serve as a message broker between your microservices. Let’s see how it works and when it makes sense to use it.
Core concepts of Redis Pub/Sub
Redis Pub/Sub Basics
The Pub/Sub mechanism in Redis revolves around these three simple concepts.
Channels: Named message routes (like subreddits on Reddit) eg.
user_signups
orpayment_events
.Publishers: Clients that send messages to specific channels
Subscribers: Clients that listen for messages on one or more channels.
When a publisher sends a message to a channel, Redis broadcasts that message to all subscribers of that channel in realtime. The operation is async, so publishers don’t wait for confirmations/acknowledgements that subscribers recieved the message.
The beauty of Redis Pub/Sub lies in the simplicity. The entire featues can be accessed with just a handful of commands:
PUBLISH channel message
SUBSCRIBE channel [channel ...]
PSUBSCRIBE pattern [pattern ...]
UNSUBSCRIBE [channel ...]
PUNSUBSCRIBE [pattern ...]
Redis vs Traditional Message Brokers
Unlike heavyweight message brokers like RabbitMQ and Apache Kafka. Redis Pub/Sub is remarkably lightweight. Here’s the comparison:
Strengths
Simplicity: Minimal config, and straighforward API
Speed: Sub-millisecond latency for message delivery
Integration: If you’re already using Redis, it’s one less system to maintain
Limitations
No persistance: Messages are only delivered to the currently connected subscribers
No guaranteed delivery: If a subscriber is offline it misses the message
No message queues: Messages can’t be processed later by reconnecting clients
Use cases for Redis Pub/Sub
Redis Pub/Sub excels in scenarios requiring realtime messaging with minimal latency:
Realtime notifications: Chat applications, live dashboards, activity feeds
Event driven microservices: Services reacting to events from other services
IoT Applications: Distributing sensor data to multiple processing services
Live monitoring systems: Broadcasting metrics to various visualization tools
Designing a Pub/Sub System with Redis
Architecture Overview
A typical Redis Pub/Sub architecture in a microservices environment looks like this:
Service A might be an order service publishing new orders, Service B a payment processor both publishing and consuming events, and Service C a notification service subscribing to relevant events.
Scalability Considerations
Redis Pub/Sub scales horizontally on the subscriber side. You can have multiple instances of the same service subscribed to the same channel, and each will receive a copy of each message.
For high-volume systems, you can consider these strategies:
Channel segmentation: Use multiple fine-grained channels instead of a few broad ones
Load distribution: For heavy publishing, Redis Cluster can distribute channels across nodes
Multiple Redis instances: For complete isolation of different messaging domains
Let’s take a look at code examples
Publisher (Order Service):
const redis = require('redis');
const publisher = redis.createClient();
// When a new order is created
function createOrder(orderData) {
// Save order to database
const orderId = saveToDatabase(orderData);
// Publish event to Redis
publisher.publish('orders:created', JSON.stringify({
id: orderId,
amount: orderData.amount,
customerId: orderData.customerId,
timestamp: Date.now()
}));
return orderId;
}
Subscriber (Notification service):
const redis = require('redis');
const subscriber = redis.createClient();
subscriber.on('message', (channel, message) => {
if (channel === 'orders:created') {
const order = JSON.parse(message);
sendOrderConfirmationEmail(order.customerId, order.id, order.amount);
}
});
subscriber.subscribe('orders:created');
Best Practices and Patterns
Channel Naming Conventions
A good naming convention makes your system easier to understand and maintain:
Hierarchical structure:
domain:entity:action
(e.g.,orders:payment:succeeded
)Service-oriented:
service-name:event-type
(e.g.,payment-service:refund-processed
)Versioning: Add version when message formats might change (e.g.,
users:created:v1
)
Security
Redis Pub/Sub transmits messages in plaintext by default. For secure setups:
Use Redis ACLs to restrict which clients can publish/subscribe to which channels
Enable TLS for encrypted communication between Redis and clients
Keep Redis servers in a private network, accessible only to your services
Monitoring and Debugging
Redis provides with simple commands to inspect the Pub/Sub Activity
# List all active channels with subscribers
PUBSUB CHANNELS
# Count subscribers for specific channels
PUBSUB NUMSUB channel1 channel2
# Count pattern subscribers
PUBSUB NUMPAT
For comprehensive monitoring:
Use Redis INFO command to track overall Pub/Sub memory usage
Implement client-side metrics for message volumes and processing times
Log channel activity for debugging and auditing
Performance Optimization
To get the most out of Redis Pub/Sub:
Keep messages small: Large messages consume more bandwidth and memory
Avoid blocking operations: Subscriber callbacks should be quick and non-blocking
Use pattern matching judiciously:
PSUBSCRIBE
with wildcards is powerful but more resource-intensive
Real World Example: Microservice in action
I'll walk you through an example of an ecommerce which processes order using Redis Pub/Sub.
The Players
Order Service: Creates new orders and publishes
orders:created
eventsPayment Service: Processes payments and publishes
payments:completed
eventsInventory Service: Updates stock levels based on order events
Notification Service: Sends emails based on various events
Analytics Service: Tracks business metrics based on all events
The Flow
Customer places an order through the web app
Order Service saves the order and publishes
orders:created
with order detailsPayment Service receives the event, processes payment, then publishes
payments:completed
Inventory Service receives both events, first marking items as reserved, then confirming deduction
Notification Service sends order confirmation after
orders:created
and payment confirmation afterpayments:completed
Analytics Service silently collects all events to update dashboards
This decoupled architecture allows each service to focus on its core responsibilities without direct dependencies on other services.
When to use (and avoid) Redis Pub/Sub
Ideal Scenarios
Redis Pub/Sub is perfect for:
Real-time updates: When speed matters more than guaranteed delivery
Broadcast notifications: When many services need the same information
System status changes: Broadcasting state changes across a distributed system
Non-critical events: Where occasional missed messages are acceptable
When to consider alternatives
Look elsewhere when you need:
Guaranteed delivery: Consider Redis Streams, RabbitMQ, or Kafka
Message persistence: Redis Streams or a dedicated message broker
Complex routing logic: RabbitMQ's exchanges and bindings
Exactly-once processing: Systems with robust message acknowledgment and deduplication
Conclusion
Redis Pub/Sub offers a refreshingly simple approach to event-driven communication in microservice architectures. Its minimal API, blazing speed, and seamless integration with existing Redis deployments make it an attractive option for many real-time messaging needs.
While it won't replace full-featured message brokers for complex or mission-critical messaging, Redis Pub/Sub finds its sweet spot in scenarios where simplicity and performance are paramount. It's the lightweight champion of real-time messaging.
Until then, I encourage you to experiment with Redis Pub/Sub in your own projects. Start small, perhaps with a notification system or real-time dashboard, and experience firsthand the elegant simplicity it brings to service communication.
Some Additional Resources
Previous posts in this series
Subscribe to my newsletter
Read articles from Pranav Tripathi directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
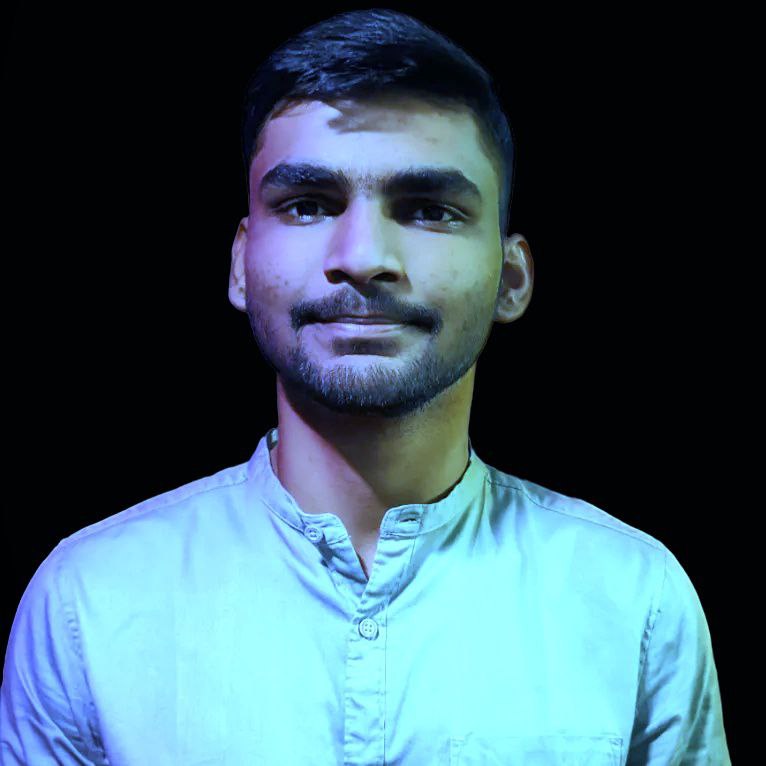
Pranav Tripathi
Pranav Tripathi
I'm a dedicated full-stack developer specializing in building dynamic and scalable applications. I have a strong focus on delivering efficient and user-friendly solutions, and I thrive on solving complex problems through technology.