React JS Compiler: Understanding How React Code Gets Compiled
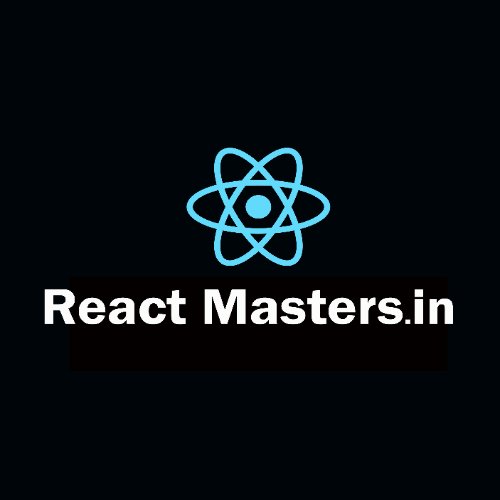
Introduction
React JS has revolutionized front-end development by enabling developers to build dynamic and interactive web applications.
However, one fundamental question arises: How does a React JS compiler work?
This article provides an in-depth look at the React JS compiler, its working mechanism, benefits, and related technologies that help in optimizing React applications.
What is a React JS Compiler?
A React JS compiler refers to the tools and processes that transform JSX (JavaScript XML) into standard JavaScript that browsers can interpret. React does not run JSX directly; instead, it compiles JSX code into pure JavaScript before execution.
Why Does React Need a Compiler?
React uses JSX, which is an extension of JavaScript that allows writing HTML-like syntax in JavaScript files. However, browsers do not understand JSX, requiring a compiler to convert it into vanilla JavaScript. The compilation ensures:
Browser compatibility
Performance optimization
Efficient execution of React applications
How React JS Compilation Works
React uses tools like Babel and SWC (Speedy Web Compiler) to transform JSX into JavaScript. The compilation process generally follows these steps:
1. Parsing JSX Code
When a developer writes JSX, the compiler first parses the code, analyzing its structure and breaking it down into tokens.
2. Transforming JSX to JavaScript
The JSX syntax is transformed into standard JavaScript using functions like React.createElement()
.
Example:
JSX Code:
const element = <h1>Hello, World!</h1>;
Compiled JavaScript:
const element = React.createElement("h1", null, "Hello, World!");
3. Optimizing the Code
The compiler optimizes the transformed code for better performance, such as:
Minimizing bundle size
Removing unused code (tree shaking)
Inlining static content
4. Generating the Final JavaScript Output
After transformation and optimization, the final JavaScript code is generated, which is executed by the browser.
Key React JS Compilers
Several tools are available to compile JSX and optimize React applications:
1. Babel
Babel is the most commonly used compiler in React applications. It translates modern JavaScript (including JSX) into ES5-compatible code.
Features:
Converts JSX into JavaScript
Supports latest ES6+ features
Optimizes and minifies JavaScript code
2. SWC (Speedy Web Compiler)
SWC is a Rust-based JavaScript compiler that provides faster compilation compared to Babel.
Features:
20x faster than Babel
Supports JSX transformation
Optimized for large-scale applications
3. esbuild
esbuild is another high-speed JavaScript bundler and compiler used in modern React applications.
Features:
Lightning-fast compilation
Supports tree-shaking
Minifies JavaScript efficiently
Benefits of Using React JS Compilers
1. Better Performance
React compilers optimize the code to ensure faster execution and reduced load times.
2. Cross-Browser Compatibility
Older browsers may not support ES6+ syntax. The compiler converts modern JavaScript into ES5, ensuring compatibility with all browsers.
3. Improved Code Maintainability
With JSX, developers can write readable and maintainable code while letting the compiler handle the transformation.
4. Support for Modern Features
React compilers allow developers to use the latest JavaScript features without worrying about browser limitations.
How to Set Up a React Compiler
To use Babel as a React compiler, follow these steps:
1. Install Dependencies
npm install --save-dev @babel/core @babel/preset-react babel-loader
2. Create a Babel Configuration File
Add a .babelrc
file in the project root:
{
"presets": ["@babel/preset-react"]
}
3. Configure Webpack (Optional)
If using Webpack, add the following rule to webpack.config.js
:
module.exports = {
module: {
rules: [
{
test: /\.jsx?$/,
exclude: /node_modules/,
use: {
loader: "babel-loader",
},
},
],
},
};
Future of React JS Compilation
With advancements in JavaScript compilers, React compilation is becoming faster and more efficient. Some trends include:
Increased adoption of SWC and esbuild for faster compilation
Improved tree-shaking for smaller bundle sizes
Automatic code splitting for better performance
Conclusion
A React JS compiler is essential for converting JSX into browser-compatible JavaScript, optimizing performance, and enhancing code maintainability. With compilers like Babel, SWC, and esbuild, React applications can run smoothly across various environments.
Understanding how React compilers work helps developers write optimized, efficient, and scalable React applications. If you're working with React, leveraging the right compiler is key to building high-performance applications!
Subscribe to my newsletter
Read articles from React Masters directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
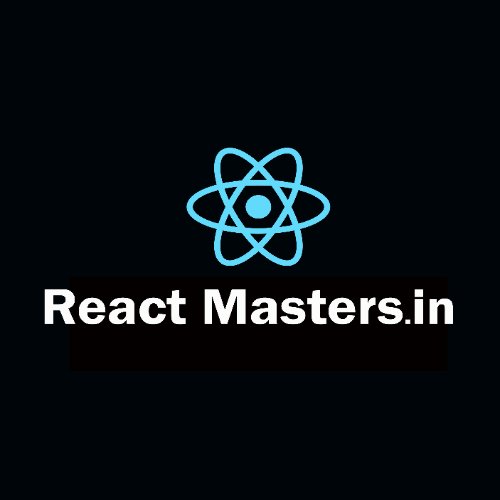
React Masters
React Masters
React Masters is one of the leading React JS training providers in Hyderabad. We strive to change the conventional training modes by bringing in a modern and forward program where our students get advanced training. We aim to empower the students with the knowledge of React JS and help them build their career in React JS development. React Masters is a professional React training institute that teaches the fundamentals of React in a way that can be applied to any web project. Industry experts with vast and varied knowledge in the industry give our React Js training. We have experienced React developers who will guide you through each aspect of React JS. You will be able to build real-time applications using React JS.