Asynchronous JavaScript Explained

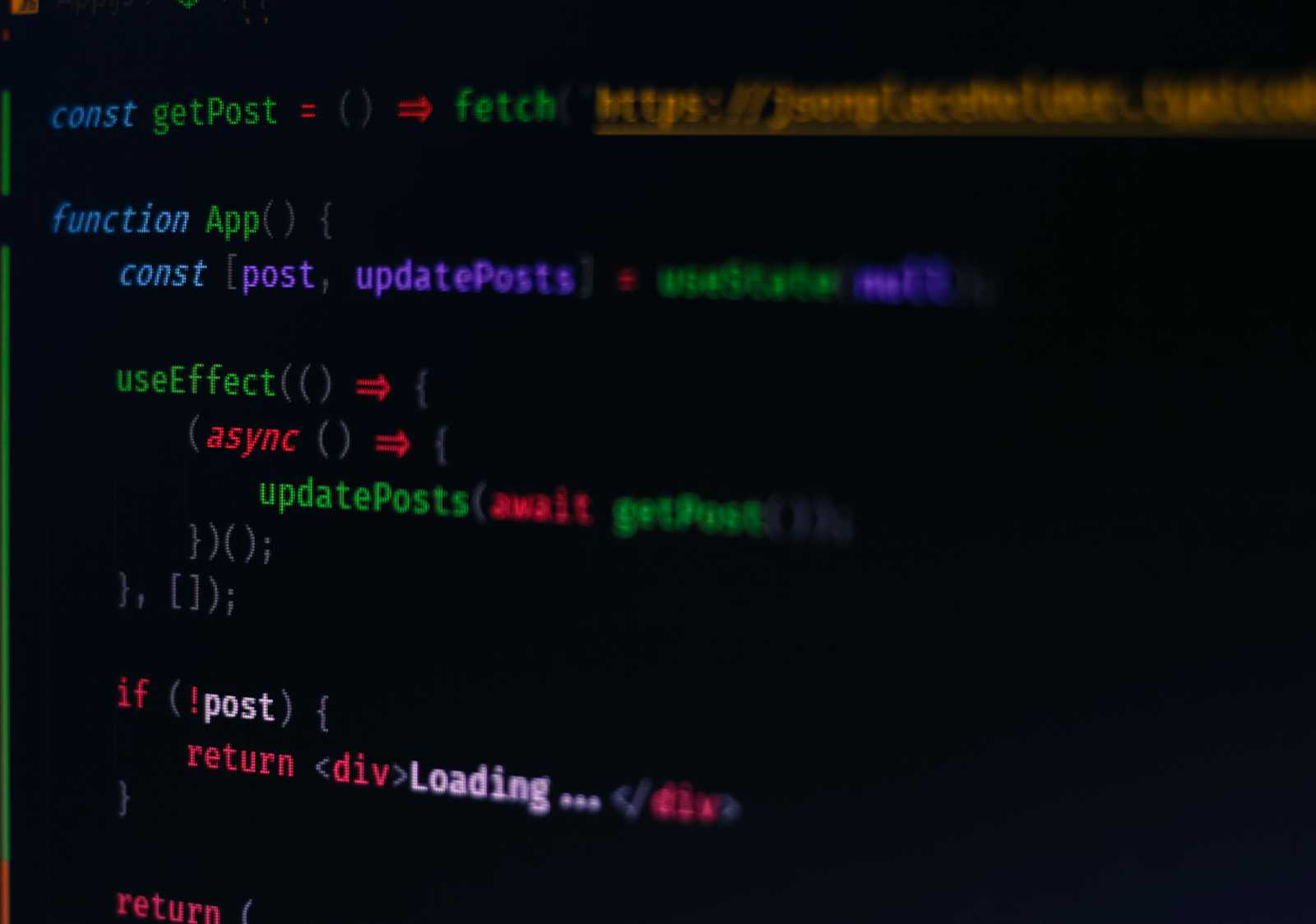
1. What is Asynconous Programming?
Asynchronous programming is a way to handle operations that might take some time to complete (like fetching data from a server, reading files, or processing large amounts of data) without blocking the rest of your code from executing.
In JavaScript, code normally runs synchronously - meaning one line executes after another in sequence. However, this can be problematic for time-consuming operations.
2. Key concepts
Callbacks
The original way to handle async operations in JavaScript was through callbacks:
function fetchData(callback) {
setTimeout(() => {
const data = "Hello World";
callback(data);
}, 2000);
}
fetchData((result) => {
console.log(result); // Prints after 2 seconds
});
console.log("This prints first!");
However, callbacks can lead to callback hell when multiple async operations need to be chained:
fetchData1((result1) => {
fetchData2(result1, (result2) => {
fetchData3(result2, (result3) => {
console.log(result3);
});
});
});
Promises
Promises provide a more elegant way to handle async operations. A Promise is an object representing the eventual completion (or failure) of an async operation.
const myPromise = new Promise((resolve, reject) => {
setTimeout(() => {
const success = true;
if (success) {
resolve("Operation succeeded!");
} else {
reject("Operation failed!");
}
}, 2000);
});
myPromise
.then(result => console.log(result))
.catch(error => console.error(error));
Promises have three states:
Pending: Initial state
Fulfilled: Operation completed successfully
Rejected: Operation failed
Async/Await
Async/await is built on top of Promises and provides an even cleaner syntax for handling async operations
async function fetchUserData() {
try {
const response = await fetch('<https://api.example.com/user>');
const userData = await response.json();
return userData;
} catch (error) {
console.error('Error:', error);
}
}
// Using the async function
async function init() {
const userData = await fetchUserData();
console.log(userData);
}
init();
3. The Event Loop
The event loop is what makes async operations possible in JavaScript. Here's how it works:
Synchronous code executes on the main thread
Async operations are delegated to the Web APIs (in browsers) or C++ APIs (in Node.js)
When async operations complete, their callbacks are placed in the callback queue
The event loop checks if the call stack is empty
If empty, it takes the first callback from the queue and pushes it to the stack for execution
4. Common Async Operations
Promise.all()
Executes multiple Promises in parallel and waits for all to complete:
const promise1 = fetch('<https://api.example.com/data1>');
const promise2 = fetch('<https://api.example.com/data2>');
Promise.all([promise1, promise2])
.then(([result1, result2]) => {
console.log(result1, result2);
})
.catch(error => console.error(error));
Promise.race()
const promise1 = new Promise(resolve => setTimeout(() => resolve('First'), 2000));
const promise2 = new Promise(resolve => setTimeout(() => resolve('Second'), 1000));
Promise.race([promise1, promise2])
.then(result => console.log(result)); // Prints 'Second'
5. Best Practices
Always handle errors in async operations using try/catch or .catch()
Avoid mixing callbacks with Promises/async-await
Use Promise.all() when multiple independent async operations need to complete
Consider using async/await for cleaner, more readable code
Remember that await can only be used inside async functions
6. Common Use Cases
API calls
File operations
Database queries
Timer operations
Event handling
Animation frames
WebSocket communications
Understanding async JavaScript is crucial for building modern web applications that need to handle multiple operations simultaneously while maintaining a responsive user interface.
Subscribe to my newsletter
Read articles from Neeraj Pallikonda directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Neeraj Pallikonda
Neeraj Pallikonda
I make the world a better place by solving one problem at a time✌