The Art of Isolation in Programming
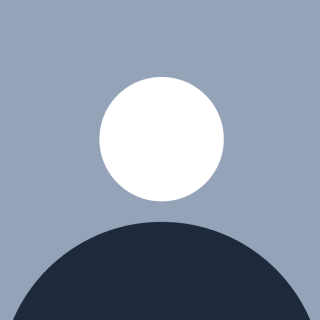
Ever felt like refactoring all of the code just because of a minor change somewhere? Yeah, it’s painful. A tiny tweak, and suddenly you’re rewriting half the application.
Isolation is tough in real life—people struggle with it all the time. But in programming? It’s an art. Breaking down tasks into micro-tasks and attacking them one by one lets you do the unimaginable.
Example: Layered Approach in TypeScript
Imagine a simple user management system. Instead of dumping everything into one giant file, we split it into layers—presentation, logic, and data.
Before (Messy, Hard to Change)
// One big chunk doing everything
function handleUserUpdate(userId: string, newData: any) {
// Validate
if (!newData.email.includes("@")) throw new Error("Invalid email");
// Fetch old data
const oldUser = db.query("SELECT * FROM users WHERE id = ?", [userId]);
// Update
db.execute("UPDATE users SET email = ? WHERE id = ?", [newData.email, userId]);
// Log
console.log(`User ${userId} updated from ${oldUser.email} to ${newData.email}`);
// Notify
sendEmail(oldUser.email, "Your email was updated!");
}
After (Isolated, Scalable)
// Data Layer - Only deals with storage
class UserRepository {
static getUser(id: string) {
return db.query("SELECT * FROM users WHERE id = ?", [id]);
}
static updateUser(id: string, newData: { email: string }) {
db.execute("UPDATE users SET email = ? WHERE id = ?", [newData.email, id]);
}
}
// Logic Layer - Handles rules
class UserService {
static validateEmail(email: string) {
if (!email.includes("@")) throw new Error("Invalid email");
}
static async updateEmail(userId: string, newEmail: string) {
this.validateEmail(newEmail);
const oldUser = await UserRepository.getUser(userId);
await UserRepository.updateUser(userId, { email: newEmail });
return oldUser;
}
}
// Presentation Layer - Handles interaction
async function handleUserUpdate(userId: string, newEmail: string) {
const oldUser = await UserService.updateEmail(userId, newEmail);
Logger.logChange(userId, oldUser.email, newEmail);
Notifier.sendEmailUpdateAlert(oldUser.email);
}
Why This Rocks
Change one layer without breaking others – Need a new email validator? Only touch
UserService
.Easier to understand – Each piece does one thing.
Scalable – Adding a new feature? Just extend, don’t rewrite.
Isolation isn’t just good practice—it’s what separates "it works" from "it works, and I didn’t lose my mind maintaining it."
Next time you code, think in layers. Your future self will thank you.
Subscribe to my newsletter
Read articles from Dichan Shrestha directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by