Mastering JavaScript Functions with AI (The Heart of Reusable Code)

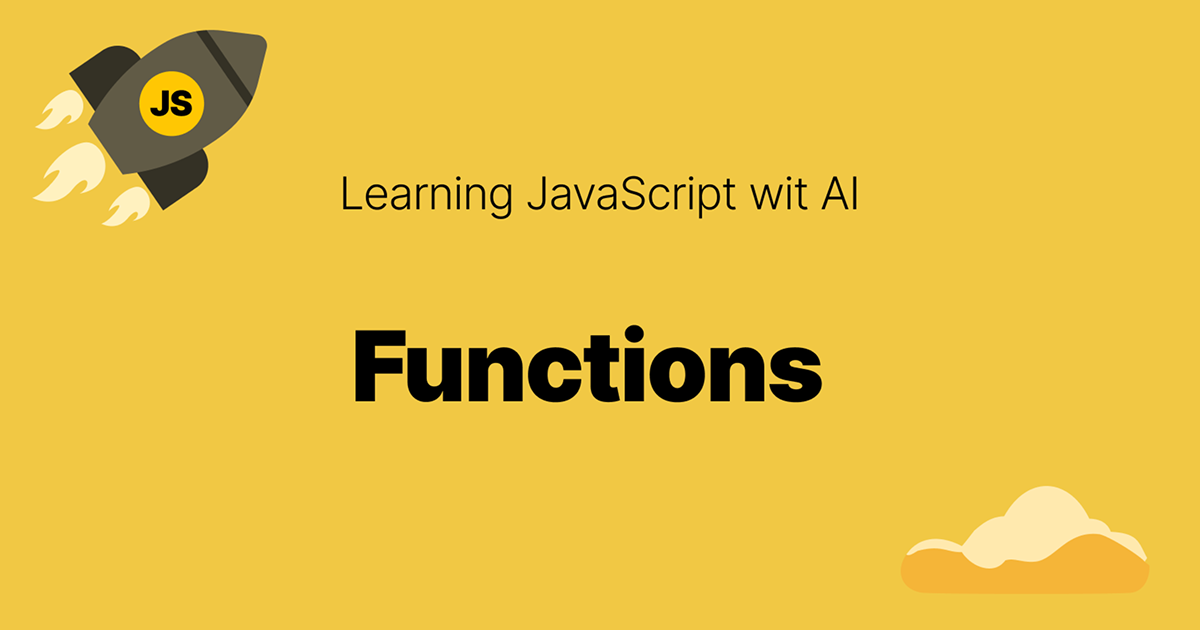
There’s a point in your JavaScript learning journey where everything starts to feel a bit more manageable — and more exciting. That’s a turning point in your learning journey: it’s usually when functions begin to click.
Functions let you organize your code, cut down on repetition, and build things that actually feel like real software programs. They’re essential. And thanks to AI tools like ChatGPT, getting the hang of them is now a whole lot easier.
Instead of memorizing syntax and hoping it sticks, you can use tools like ChatGPT to ask questions, check your code, and explore how things work in real time. That means fewer roadblocks and more lightbulb moments.
Let’s walk through the core ideas, one piece at a time.
How to create a function
At its simplest, a function is just a named block of code that does something. Here’s what that looks like:
function greetUser(name) {
console.log(`Hello, ${name}!`);
}
greetUser("Sam");
You write it once, and call it whenever you need it. That’s the beauty of it — you define the logic once and reuse it as often as you want.
This small shift — packaging logic into a function — makes your code cleaner and your brain less cluttered. And when you’re unsure why a function isn’t behaving as expected, you can ask ChatGPT directly: “Why isn’t this function running?” and it’ll walk you through it.
Functions with default parameters
Sometimes, you don’t want your function to break just because someone forgot to pass an argument. That’s where default parameters come in.
function greet(name = "Guest") {
console.log(`Welcome, ${name}!`);
}
greet(); // Welcome, Guest!
greet("Nina"); // Welcome, Nina!
Default values let your code be flexible and forgiving. It’s a small detail, but one that makes a big difference when building user-friendly programs.
Return values
Functions aren’t just about printing messages. They can also send data back.
function add(a, b) {
return a + b;
}
const result = add(3, 4); // 7
Once you start working with return values, your functions stop being just actions — and start becoming tools. You can plug them into other parts of your code, store their output in variables, and chain logic together more cleanly.
Variable scope
Scope is one of those things you might not think about until something breaks. In short, scope determines where your variables live and who can see them.
Here’s a quick look:
function sayHi() {
let name = "Jules";
console.log(name);
}
sayHi();
console.log(name); // Error - name isn't available out here
Variables in JavaScript don’t all behave the same way — they live in different “scopes,” and understanding those boundaries is key to writing predictable code.
Global scope means the variable is available everywhere in your code. If you declare something outside of any function or block, it’s globally scoped.
Local scope applies to variables declared inside a function. These variables can only be accessed within that function.
Block scope, introduced with let and const, is even more specific. A variable declared inside a block (like an if statement or a for loop) only exists within those curly braces.
Function hoisting
One weird-but-useful quirk of JavaScript is that you can sometimes use functions before you’ve even written them.
sayHello(); // Works fine
function sayHello() {
console.log("Hi!");
}
That works because JavaScript hoists function declarations to the top of their scope. But function expressions don’t get the same treatment:
ayHi(); // Error
const sayHi = function () {
console.log("Hey!");
};
Knowing what gets hoisted — and what doesn’t — can save you a lot of head-scratching.
The role of ‘this’
this is one of those keywords that feels mysterious at first. But once you understand it, it opens the door to some really powerful programming patterns.
The short version? this refers to the object that’s calling the function.
const user = {
name: "Ava",
greet() {
console.log(`Hi, I'm ${this.name}`);
}
};
user.greet(); // Hi, I'm Ava
But this can change depending on how a function is called. That’s where things get tricky. Thankfully, modern JavaScript introduced arrow functions, which help by locking in the surrounding value of this. More on that below.
Anonymous functions
Sometimes you just need a quick function on the fly — and don’t care about naming it.
etTimeout(function () {
console.log("This runs after a delay.");
}, 1000);
These unnamed functions are super useful for short tasks like timers, event handlers, or callbacks. But if your function is doing more than a couple things, giving it a name can make your code easier to debug.
Arrow functions
Arrow functions are a newer, shorter way to write functions.
const multiply = (a, b) => a * b;
They’re great for small tasks — especially when working with arrays. They also behave differently when it comes to ‘this‘, which makes them perfect in many callback situations.
Just keep in mind: shorter doesn’t always mean better. Use them when they help, not just because they look cool.
Higher-order functions
Here’s where JavaScript starts to feel really powerful.
A higher-order function is one that either takes another function as an input or returns a new one. That might sound abstract, but you’re probably already using them:
const numbers = [1, 2, 3];
const doubled = numbers.map(n => n * 2);
The .map() method is a higher-order function — it takes a callback and applies it to every item in the array.
You can also build your own function:
function runTwice(callback) {
callback();
callback();
}
runTwice(() => console.log("Hi again"));
Once you get the hang of these, you’ll start seeing opportunities to reuse logic in creative ways — and that’s when your JavaScript really starts to shine.
Final thoughts
Functions are the foundation of real-world JavaScript. Once you understand how to use them well, everything else — objects, arrays, DOM manipulation — starts to make more sense.
What makes learning functions so much better today is the ability to test ideas quickly. You can describe what you’re trying to build, paste in your code, and ask AI to explain what’s going wrong or how to improve it.
It’s like having a personal tutor that never gets tired of your questions.
This article is a summary of “Learn JavaScript with AI — A Smarter Way to Code” by D-Libro.
Subscribe to my newsletter
Read articles from David Ocean directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
