/* DISABLES CODE */: When Clang warns about unreachable code

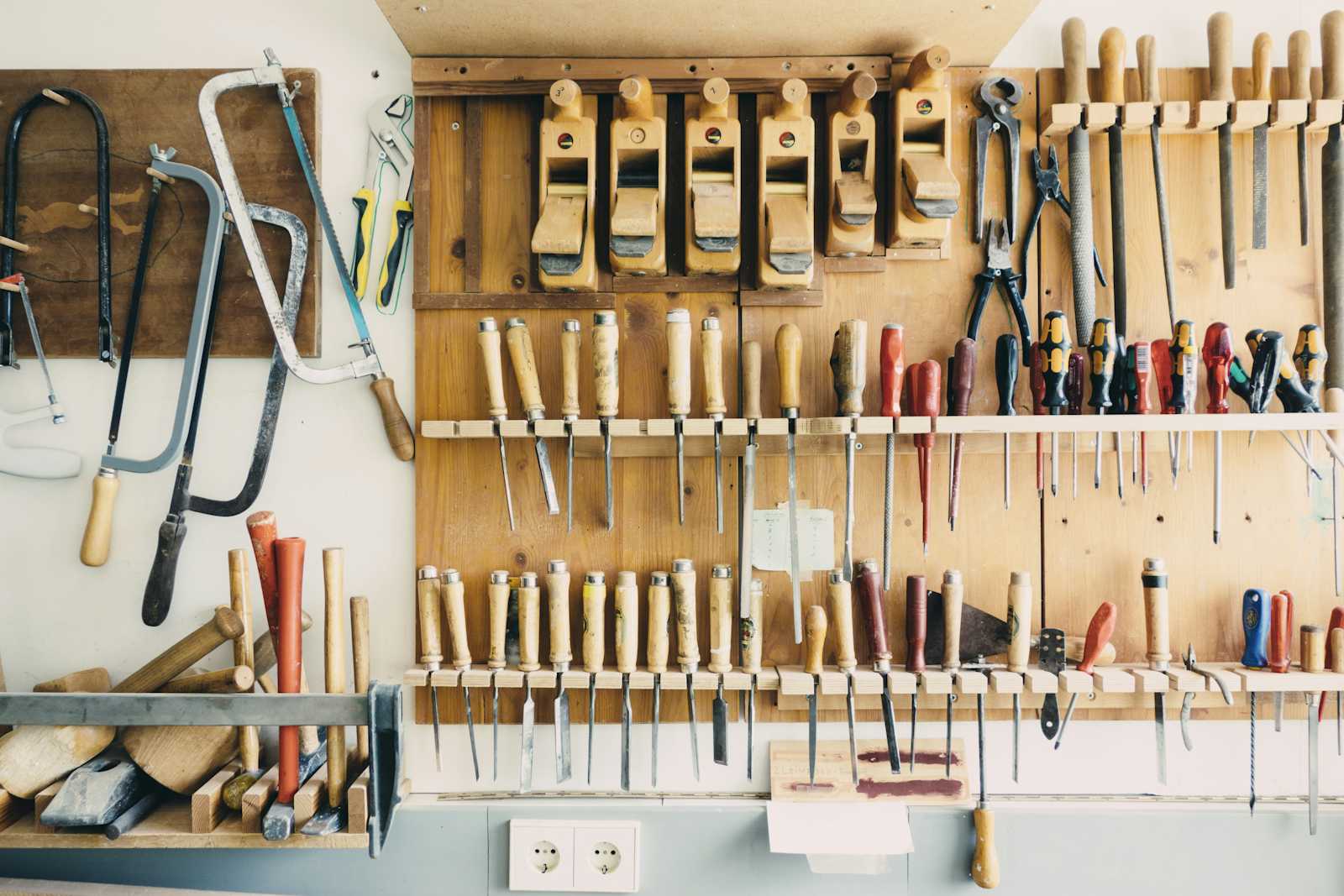
I would like to write about unreachable code in C and C++ and about the pertinent compiler warnings in this context.
Sometimes, entire blocks of our C or C++ source codes are unintentionally cut off from the control flow. Consider the following demonstrative (albeit somewhat contrived) example written in modern C.
void report_condition()
{
bool b = check_complicated_condition();
if( true ) { // author forgot to put b here.
puts("Condition holds!\n");
} else {
puts("Condition fails!\n"); // Never executed.
}
}
Such a function may be written for debugging: at each call, it reports whether the condition of interest is satisfied. However, it very much seems that the author forgot to check the actual variable b, perhaps because the function check_complicated_condition
was not available during earlier stages of coding. Therefore, the control flow will never enter the else-branch, which we can safely assume to be a mistake.
Compiler warnings
As diligent C and C++ programmers, we naturally switch on as many compiler warnings as possible. The Clang compiler offers us the -Wunreachable-code
warning flag for situations like the above. The compiler will warn us:
warning: code will never be executed [-Wunreachable-code]
This compiler flag is available to us with Clang. The GCC also had this feature for a long time, but the flag was removed because the necessary code analysis was too complex. In particular, the control flow also depends on the optimization level, complicating the matter further.
Nota bene, the compiler flag -Wunreachable-code
is not yet included in -Wall
and -Wextra
but is easily missed: we must explicitly enable it as an option.
Application in debugging
Even if we want to have as many compiler flags switched on as possible, this can be troublesome during debugging. For example, the following debugging statements can accumulate during a longer code fix session:
if(false)
{
// Code block used occasionally during debugging,
// but intentionally inactive most of the time.
}
Clang will warn us that the code block will never be executed. However, the above example is so common in practice that it warrants an explicit opt-out. One notices that the specific warning emitted by Clang is something like this:
note: silence by adding parentheses to mark code as explicitly dead
| if(false)
| ^
| /* DISABLES CODE */ ( )
It took me a while to understand how this is supposed to work, which prompted me to write this blog entry. It is enough to set brackets to mark the code as explicitly dead:
if((false))
{
// ...
}
The bracketed comment, on the other hand, is purely decorative. It is probably good practice to mark dead code accordingly, as in,
if( /* DISABLES CODE */ (false))
{
// ...
}
or with any other macro. This allows us to find explicitly inaccessible code more quickly in the code base, e.g., when grepping this specific comment string within the code base.
This being one of the more arcane features of your C and C++ compiler, I hope you found this article helpful for your future efforts in writing clean code and easing your debugging.
Subscribe to my newsletter
Read articles from Martin Licht directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
