My Hibernate Learning Journey: A Student’s Perspective
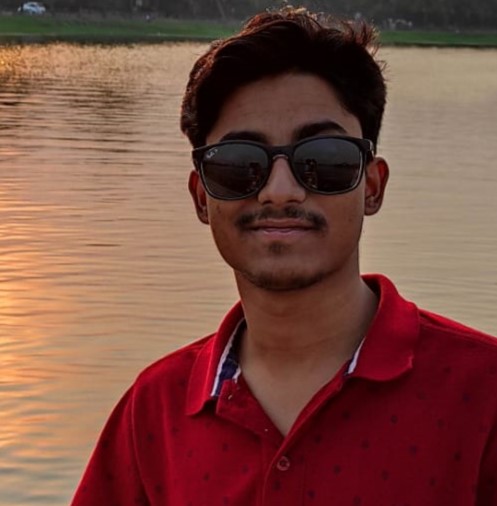

For the past two weeks, I have been exploring Hibernate, one of the most popular ORM (Object-Relational Mapping) frameworks in Java. Coming from a background in JavaScript and recently diving into Java, I was initially unsure about how Hibernate works. But after spending time watching tutorials, reading documentation, and writing code, I can finally say that I have built a solid foundation.
In this article, I'll share everything I learned about Hibernate, from basic concepts to annotations, HQL, transaction management, and more. Since I am a student still learning, this won't be a master guide, but rather a beginner-friendly explanation from my perspective.
If you're also new to Hibernate and struggling with where to start, this article might help you!
Resources I Used
Before diving into what I learned, here are the resources I used:
📺 YouTube Playlist: Hibernate Course on YouTube
🎓 Udemy Course: Navin Reddy’s Hibernate Course
📜 Official Documentation: Hibernate 6.6 Documentation
💻 My Practice Code: GitHub Repository
Now, let’s break down what I learned!
What is Hibernate?
Before learning Hibernate, I used JDBC for database operations. While JDBC works fine, handling SQL queries manually can be quite painful. Hibernate solves this issue by providing an ORM (Object-Relational Mapping) framework, which allows Java objects to be stored directly in a database without writing raw SQL.
Key Features of Hibernate:
✅ Eliminates Boilerplate SQL – No need to write long INSERT
, UPDATE
, or DELETE
queries manually.
✅ Works with Multiple Databases – Easily switch between MySQL, PostgreSQL, Oracle, etc.
✅ Uses Annotations – Reduces XML configuration and makes code cleaner.
✅ Supports HQL (Hibernate Query Language) – A more object-oriented way of querying data.
✅ Automatic Table Creation – No need to manually create tables in the database.
Setting Up Hibernate
To use Hibernate in a Java project, I followed these steps:
1️⃣ Adding Dependencies (Maven)
Since I was using Maven, I added the Hibernate dependencies in my pom.xml
file:
<dependencies>
<!-- Hibernate Core -->
<dependency>
<groupId>org.hibernate</groupId>
<artifactId>hibernate-core</artifactId>
<version>6.6.0.Final</version>
</dependency>
<!-- MySQL Driver -->
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<version>8.0.33</version>
</dependency>
<!-- Jakarta Persistence API -->
<dependency>
<groupId>jakarta.persistence</groupId>
<artifactId>jakarta.persistence-api</artifactId>
<version>3.1.0</version>
</dependency>
</dependencies>
2️⃣ Configuring hibernate.cfg.xml
Hibernate needs a configuration file (hibernate.cfg.xml
) where we define database connection details:
<hibernate-configuration>
<session-factory>
<property name="hibernate.connection.driver_class">com.mysql.cj.jdbc.Driver</property>
<property name="hibernate.connection.url">jdbc:mysql://localhost:3306/hibernatedb</property>
<property name="hibernate.connection.username">root</property>
<property name="hibernate.connection.password">password</property>
<property name="hibernate.dialect">org.hibernate.dialect.MySQLDialect</property>
<property name="hibernate.show_sql">true</property>
<property name="hibernate.hbm2ddl.auto">update</property>
</session-factory>
</hibernate-configuration>
Hibernate Annotations (No More XML!)
Instead of XML configurations, Hibernate allows us to use Java Annotations for mapping classes to database tables. Here’s an example:
import jakarta.persistence.*;
@Entity // Marks this class as a table
@Table(name = "students")
public class Student {
@Id // Marks this as the primary key
@GeneratedValue(strategy = GenerationType.IDENTITY)
private int id;
@Column(name = "student_name", nullable = false)
private String name;
@Column(name = "student_email", unique = true)
private String email;
// Constructors, Getters, and Setters
}
Explanation:
@Entity
→ Marks the class as a Hibernate entity.@Table(name="students")
→ Maps the class to a database table.@Id
→ Specifies the primary key field.@GeneratedValue
→ Automatically generates ID values.@Column(name="student_name")
→ Custom column name.
With just this simple class, Hibernate will automatically create the table in the database! 🚀
CRUD Operations in Hibernate
🔹 Inserting Data
Session session = sessionFactory.openSession();
Transaction tx = session.beginTransaction();
Student student = new Student();
student.setName("John Doe");
student.setEmail("john@example.com");
session.save(student);
tx.commit();
session.close();
🔹 Fetching Data
Student student = session.get(Student.class, 1); // Fetch student with ID 1
System.out.println(student.getName());
🔹 Updating Data
student.setEmail("newemail@example.com");
session.update(student);
tx.commit();
🔹 Deleting Data
session.delete(student);
tx.commit();
Hibernate Query Language (HQL)
HQL (Hibernate Query Language) is similar to SQL but works with objects instead of tables.
Query query = session.createQuery("from Student where name=:name");
query.setParameter("name", "John Doe");
List<Student> students = query.list();
Unlike SQL, we don’t need to worry about table names—just work with Java objects!
Transaction Management in Hibernate
By default, Hibernate does not auto-commit transactions. Every operation needs to be wrapped inside a transaction like this:
Transaction tx = session.beginTransaction();
session.save(student);
tx.commit(); // Changes are committed to the database
If anything fails, we can rollback:
tx.rollback(); // Undo changes
This ensures data consistency in case of errors.
Conclusion
Learning Hibernate has been an exciting journey. Initially, it felt overwhelming, but once I started writing code, things became much clearer.
📌 Key Takeaways:
Hibernate makes database interactions easier with ORM.
It eliminates the need for raw SQL queries.
Annotations reduce the need for XML configurations.
HQL is an object-oriented alternative to SQL.
Transaction management ensures data consistency.
For now, I have learned the basics, but I know there’s much more—Caching, Lazy Loading, Many-to-Many relationships, etc. I’ll continue exploring and share my experiences in future articles! 🚀
In a Nutshell
Hibernate is an ORM framework that simplifies Java database operations. It replaces raw SQL with Java objects, making development faster and cleaner. By using annotations, HQL, and transaction management, Hibernate provides a powerful way to interact with databases without writing complex queries. I am still learning, but so far, it has been an exciting journey!
👉 You can check out my practice codes here: GitHub Repository.
That’s it for today! If you're also learning Hibernate, let me know in the comments. Let’s learn together! 🚀
Subscribe to my newsletter
Read articles from Arkadipta Kundu directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
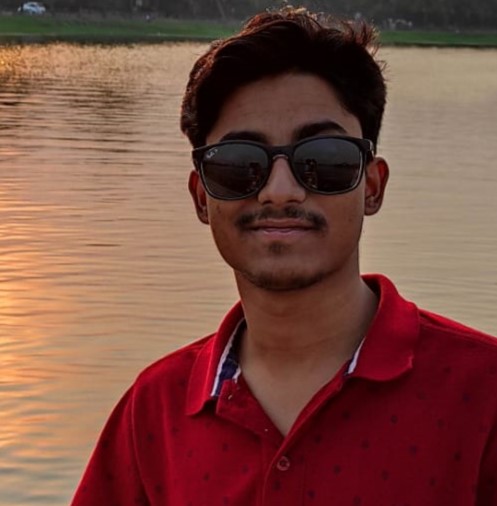
Arkadipta Kundu
Arkadipta Kundu
I’m a Computer Science undergrad from India with a passion for coding and building things that make an impact. Skilled in Java, Data Structures and Algorithms (DSA), and web development, I love diving into problem-solving challenges and constantly learning. Right now, I’m focused on sharpening my DSA skills and expanding my expertise in Java development.