Bypassing Telegram API Restrictions with Cloudflare Workers

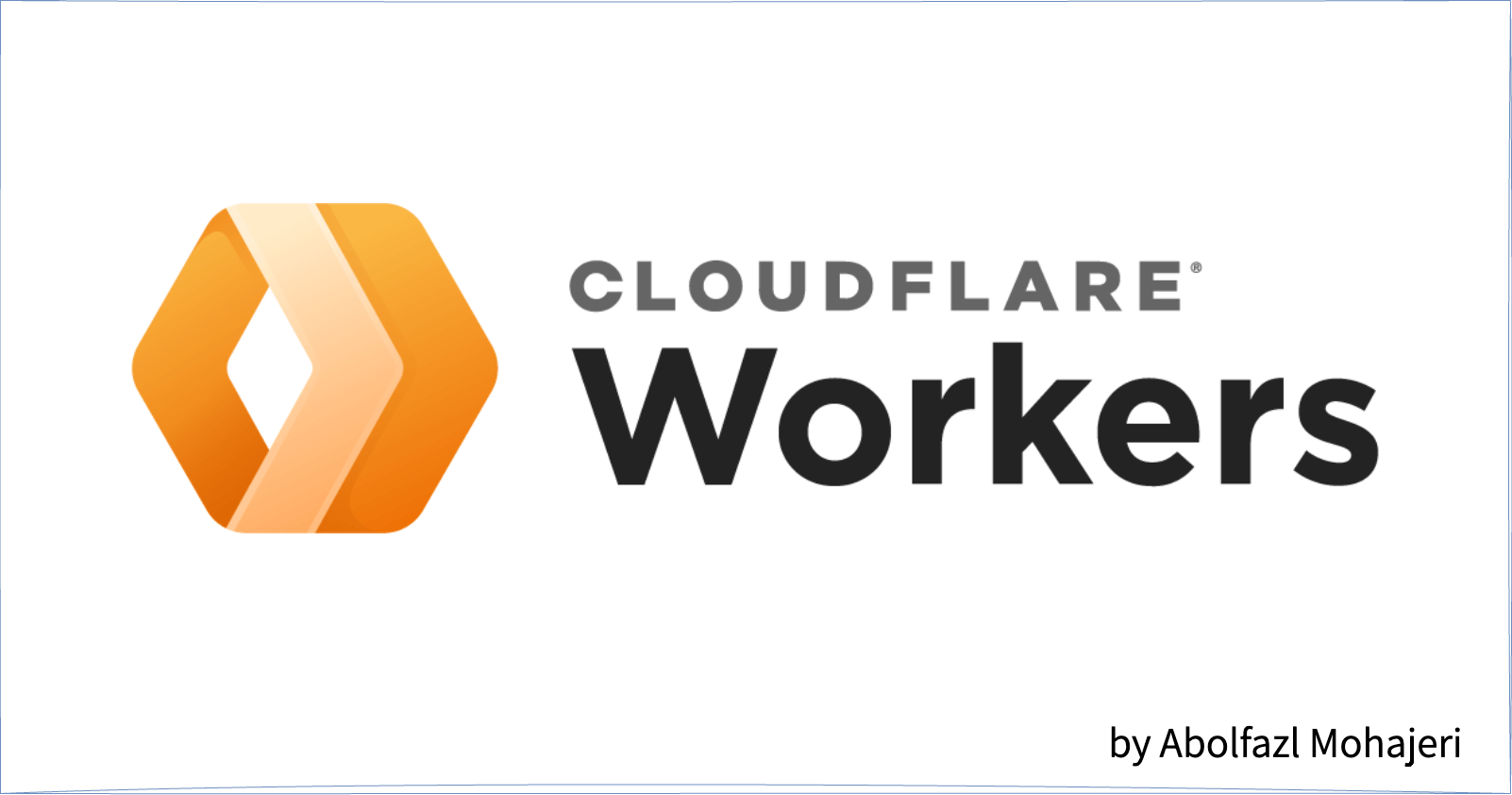
The Problem: Telegram API is Blocked in Iran
Telegram’s API is blocked in Iran, making it inaccessible on all local hosting providers. This means that if your bot is hosted on an Iranian server, it cannot directly communicate with api.telegram.org
.
I recently faced this issue while developing a Go-based Telegram bot. At first, in a simple solution, I tried using proxies inside my bot’s HTTP client, but I faced many issues and decided to use a better approach.
The Solution: Cloudflare Workers
Cloudflare Workers is a serverless platform that allows developers to run lightweight functions at the network edge. I decided to set up a Cloudflare Worker to act as a reverse proxy.
What is a Reverse Proxy?
A reverse proxy is a server that forwards requests on behalf of another server. In this case, instead of your bot directly calling:
https://api.telegram.org/bot<TOKEN>/getMe
It calls:
https://YOUR_WORKER_ADDRESS/bot<TOKEN>/getMe
The Cloudflare Worker then forwards the request to Telegram’s API and returns the response back to your bot. This completely bypasses any regional restrictions.
How to Set Up Cloudflare Workers for Telegram API
Step 1: Create a Cloudflare Worker
Go to Cloudflare Workers and create a new Worker.
Replace the default hello world script with the following code:
export default {
async fetch(request) {
const url = new URL(request.url);
url.hostname = "api.telegram.org";
const modifiedRequest = new Request(url, {
method: request.method,
headers: request.headers,
body: request.method === "GET" ? null : request.body
});
return fetch(modifiedRequest);
}
};
- Save and deploy the Worker.
Step 2: Use the Created Worker
You just need to replace https://api.telegram.org
with https://YOUR_WORKER_ADDRESS
in any framework or language. For example, in Go:
var (
Bot *tgbotapi.BotAPI
TelegramBotToken string
)
func InitTelegram() {
TelegramBotToken = os.Getenv("TELEGRAM_BOT_TOKEN")
proxyURL := os.Getenv("TELEGRAM_PROXY_URL") // https://YOUR_WORKER_ADDRESS
var err error
Bot, err = tgbotapi.NewBotAPIWithAPIEndpoint(TelegramBotToken, proxyURL+"/bot%s/%s")
if err != nil {
log.Fatal(err)
return
}
log.Printf("Authorized on account %s", Bot.Self.UserName)
}
Why Use Cloudflare Workers?
No need for a VPS
Fast and free (With some limitation)
Easy to deploy and maintain
Bypasses regional restrictions effortlessly
Conclusion
If you are facing Telegram API restrictions in Iran or any other country, Cloudflare Workers is a powerful and free solution to keep your bot running smoothly. By simply rerouting requests through a Worker, you can avoid direct blocks and enjoy uninterrupted access to Telegram’s API.
Have you tried this solution? Let me know your experience in the comments!
Subscribe to my newsletter
Read articles from Abolfazl Mohajeri directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
