Dynamically Loading Redux Reducers at Runtime in a React Project

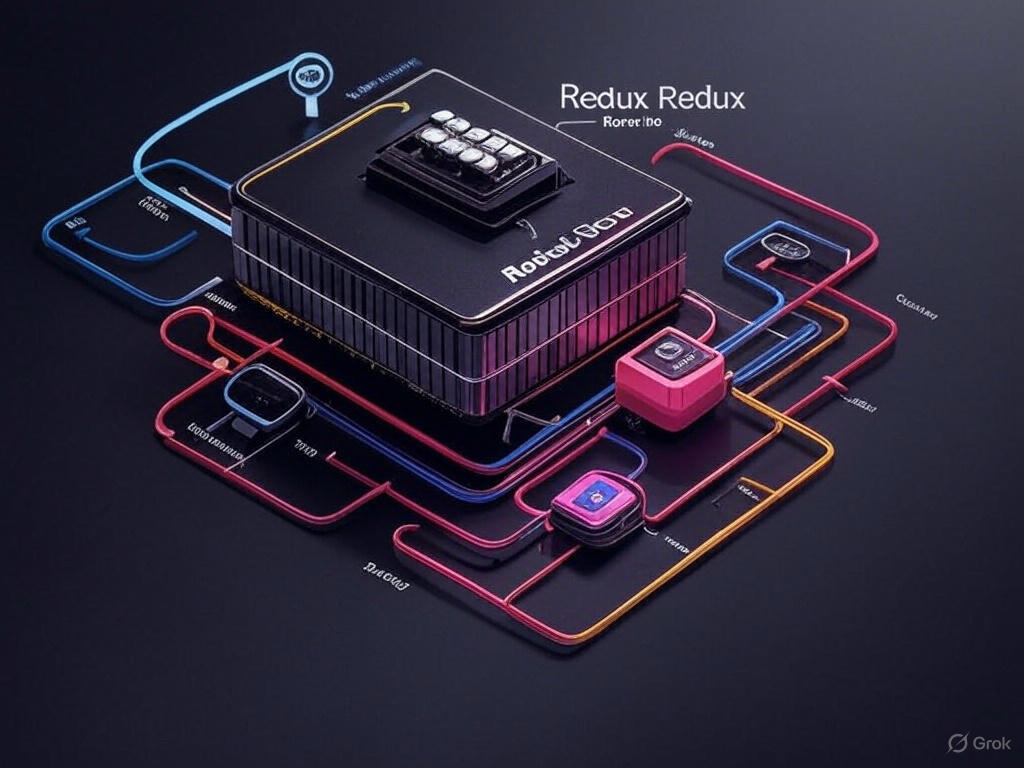
When working with Redux in a React project, it is sometimes necessary to load reducers dynamically at runtime. This can be useful in scenarios like code splitting, lazy loading, or changing the reducer logic dynamically. In this blog, we will set up a React-Redux project and dynamically replace a reducer at runtime.
1. Create a React Project
First, create a React project using Vite or Create React App:
npx create-react-app redux-dynamic-reducer
cd redux-dynamic-reducer
npm install
Alternatively, using Vite:
npm create vite@latest redux-dynamic-reducer --template react
cd redux-dynamic-reducer
npm install
2. Set Up React-Redux in the Project
Install the necessary dependencies:
npm install redux react-redux
Now, set up Redux in the project.
Define the Default (Old) Reducer
Create a file reducers.js
:
const oldReducer = (state = { content: 'This is read from oldReducer' }, action) => {
switch (action.type) {
default:
return state;
}
};
export default oldReducer;
Configure the Store
Create a store.js
file:
import { createStore } from 'redux';
import oldReducer from './reducers';
const store = createStore(oldReducer);
export default store;
3. Create a Component to Display Data
Create a component DynamicComponent.js
that reads data from the Redux store:
import React, { Component } from 'react';
import { connect } from 'react-redux';
import store from '../store';
class DynamicComponent extends Component {
constructor(props) {
super(props);
this.state = { useNewReducer: false };
}
componentDidUpdate(prevProps, prevState) {
if (this.state.useNewReducer !== prevState.useNewReducer) {
import('../newReducer').then(({ default: newReducer }) => {
store.replaceReducer(newReducer);
this.forceUpdate();
});
}
}
handleReplaceReducer = () => {
this.setState({ useNewReducer: true });
};
render() {
return (
<div>
<h1>{this.props.content}</h1>
<button onClick={this.handleReplaceReducer}>Replace Reducer</button>
</div>
);
}
}
const mapStateToProps = (state) => ({
content: state.content,
});
export default connect(mapStateToProps)(DynamicComponent);
4. Define the New Reducer
Create a new file newReducer.js
:
const newReducer = (state = { content: 'This is read from newReducer' }, action) => {
switch (action.type) {
default:
return state;
}
};
export default newReducer;
5. Use the Component in the App
Modify App.js
to include the DynamicComponent
:
import React from 'react';
import { Provider } from 'react-redux';
import store from './store';
import DynamicComponent from './components/DynamicComponent';
const App = () => (
<Provider store={store}>
<DynamicComponent />
</Provider>
);
export default App;
Conclusion
By dynamically replacing reducers at runtime using store.replaceReducer(newReducer)
, we can change Redux state logic on the fly. This technique is beneficial for optimizing performance through lazy loading and managing feature flags effectively in large-scale applications.
Subscribe to my newsletter
Read articles from Chiranjhivi Ghimire directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
