Understanding RESTful APIs: A Comprehensive Guide
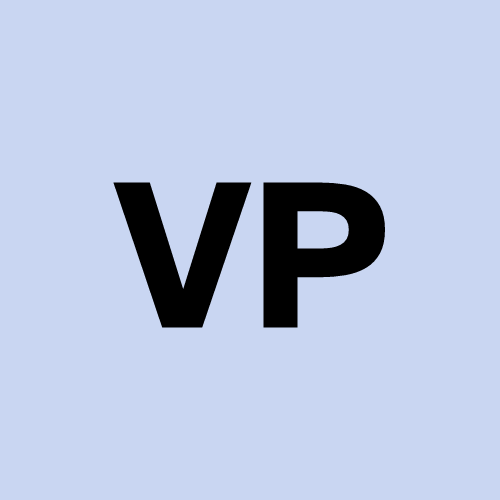
REST (Representational State Transfer) has become the backbone of modern web services, enabling seamless communication between clients and servers. Whether you're a developer or a tech enthusiast, understanding REST is crucial. Let’s break down its core principles, concepts, and how it powers the web.
What is REST?
REST stands for Representational State Transfer, a software architecture style for building scalable web services. Let’s decode the term:
State (Data): The information you want to transfer (e.g., user profiles, product details).
Representation: The format of the data, such as JSON, XML, HTML, or plain text.
Transfer: Moving data between client and server over HTTP.
REST is not a protocol but a set of guidelines for designing APIs that are efficient, stateless, and easy to scale.
RESTful API Principles
For an API to be truly RESTful, it must follow these principles:
1. Client-Server Architecture
Separation of Concerns: The client (frontend) and server (backend) operate independently.
Example: A mobile app (client) interacts with a cloud-based server without needing to know its internal workings.
2. Statelessness
No Server-Side Sessions: Each client request must contain all necessary information. The server doesn’t store user state between requests.
Example: Authentication tokens are sent with every request instead of relying on server-stored sessions.
3. Cacheable Responses
Improved Performance: Clients can cache responses (e.g., store frequently accessed data locally).
Example: Browser caching product listings to reduce server load.
4. Uniform Interface
Consistency: A standardized way to interact with resources using URIs, HTTP methods, and status codes.
Example: Using
GET /users
to retrieve all users andGET /users/1
for a specific user.
5. Layered System
Scalability: Servers can have multiple layers (e.g., security, load balancing) without the client knowing.
Example: A request might pass through a firewall, authentication layer, and database server.
Key REST Concepts
1. URI (Uniform Resource Identifier)
URIs uniquely identify resources. Follow a logical structure:
Copy
http://api.example.com/customers/1/orders/23
Resources: Entities like
customers
,orders
.Hierarchy: Use sub-resources for relationships (e.g.,
/customers/1/orders
).
2. HTTP Methods
GET: Retrieve data (e.g.,
GET /products
).POST: Create a resource (e.g.,
POST /users
with a JSON body).PUT: Update an entire resource (e.g.,
PUT /users/1
).DELETE: Remove a resource (e.g.,
DELETE /posts/5
).PATCH: Partially update a resource (e.g., update a user’s email only).
3. HTTP Status Codes
200 OK: Successful request (e.g., data returned).
201 Created: Resource created (e.g., after a
POST
).400 Bad Request: Invalid input (e.g., missing fields).
401 Unauthorized: Authentication failed.
404 Not Found: Resource doesn’t exist.
500 Internal Server Error: Generic server error.
Why REST Matters
Flexibility: Supports multiple data formats (JSON, XML).
Scalability: Statelessness and caching reduce server load.
Interoperability: Works across platforms (web, mobile, IoT).
Best Practices for RESTful APIs
Use nouns in URIs (e.g.,
/users
, not/getUsers
).Version your API (e.g.,
/v1/products
).Document endpoints thoroughly (tools like Swagger/OpenAPI help).
Secure with HTTPS and OAuth2.
Example Workflow: Order Management
Create an Order:
http
Copy
POST /orders Body: { "product": "Laptop", "quantity": 1 } Response: 201 Created
Retrieve the Order:
http
Copy
GET /orders/123 Response: 200 OK with order details
Delete the Order:
http
Copy
DELETE /orders/123 Response: 200 OK
Final Thoughts
RESTful APIs are the glue connecting modern applications. By adhering to REST principles—statelessness, cacheability, and a uniform interface—you can build robust, scalable systems. Whether you’re fetching data with GET
or securing your API with HTTPS, understanding these fundamentals ensures you’re on the right track.
Subscribe to my newsletter
Read articles from Vikash Pandey directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
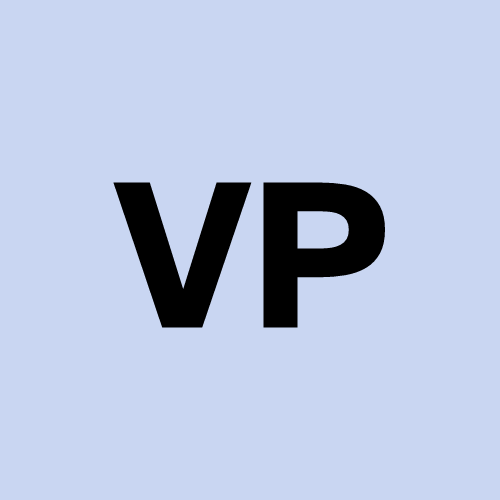