Java & Java 8 Interview Question & Answers

Table of contents
- Introduction:
- Core Java Interview Questions and Answers:
- Q1. What are the data types in Java? Java has two types of data types:
- Q2. What are wrapper classes?
- Q3. Are there dynamic arrays in Java?
- Q4. What is JVM?
- Q5. Why is Java platform-independent?
- Q6. What are local and global variables?
- Q7. What is data encapsulation?
- Q8. What is Method overloading?
- Q9. What is Method overriding?
- Q10. Why is the main method static in Java?
- Q11. What is the difference between the throw and throws keywords in Java?
- Q12. What do you mean by singleton class?
- Q13. Does every try block need a catch block?
- Q14. What is the usage of the super keyword in Java?
- Q15. What do you mean by the final keyword?
- Q16. How is an exception handled in Java?
- Q17. How can objects in a Java class be prevented from serialization?
- Q18. What is the difference between a constructor and a method in Java?
- Q19. Why is reflection used in Java?
- Q20. What are the different types of ClassLoaders in Java?
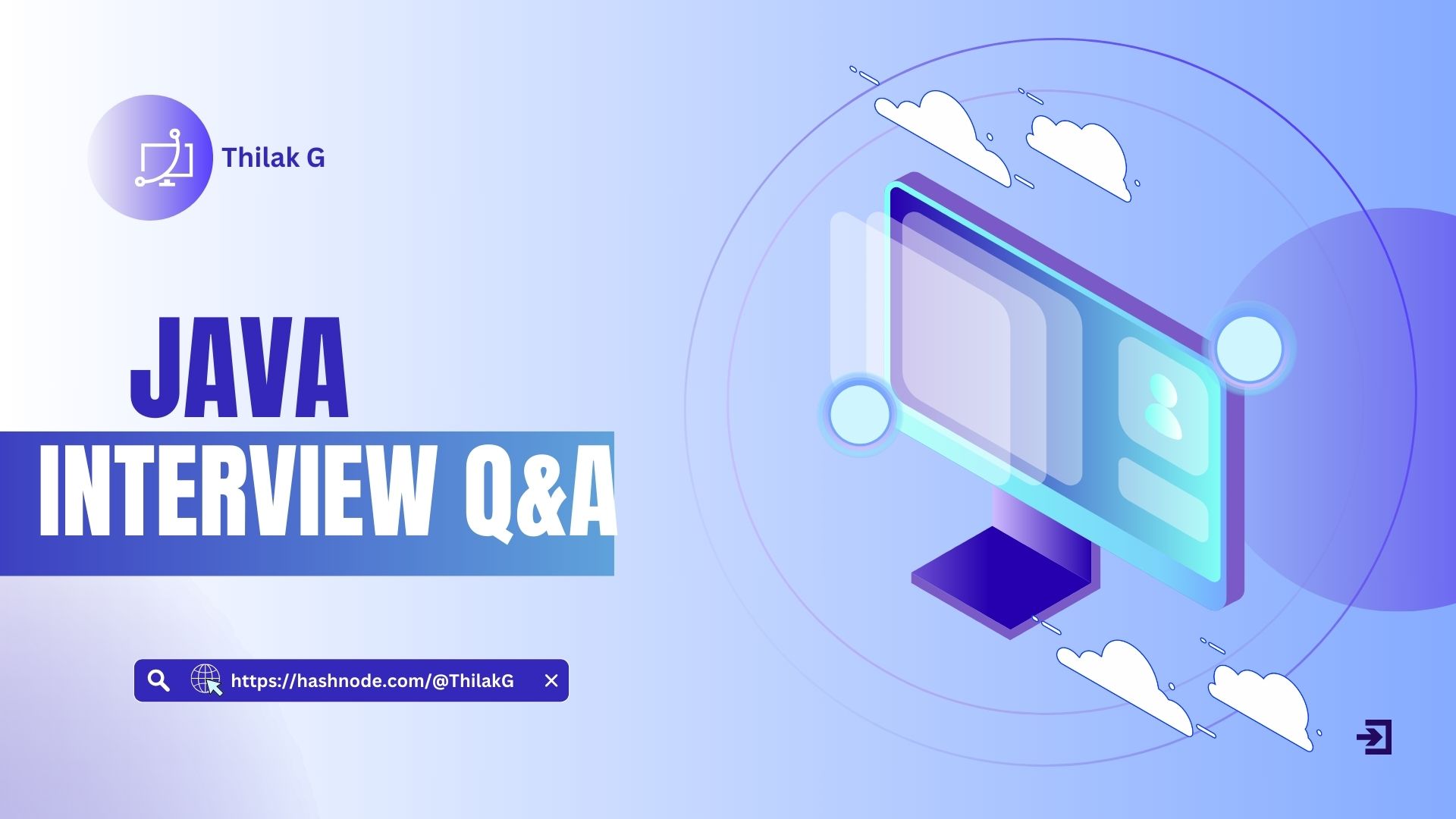
Introduction:
Hello Hi, Good day!. Very happy to see you in this blog. In this blog I will upload daily Q&A of Java for the interview preparation, which helped me ace the job interview. I will also upload some more questions on Java 8 features.
Core Java Interview Questions and Answers:
Q1. What are the data types in Java? Java has two types of data types:
1. Primitive Data Types:
These include byte, short, int, long, float, double, char, and Boolean. They store simple values and are not objects.
2. Non-Primitive Data Types:
These include String, Array, Class, and Interface. They are derived from primitive data types and provide more functionalities.
Q2. What are wrapper classes?
Wrapper classes provide an object representation of primitive data types, such as Integers, Doubles, and Booleans. These classes allow primitives to be used in collections and provide useful utility methods.
Q3. Are there dynamic arrays in Java?
Java arrays are fixed in dynamically. Q4 size. However, Array List (from the Java.util package) provides a dynamic array implementation where elements can be added or removed dynamically
Q4. What is JVM?
The Java Virtual Machine (JVM) is a part of the Java Runtime Environment (JRE). It is responsible for executing Java bytecode by converting it into machine code specific to the operating system.
Q5. Why is Java platform-independent?
Java achieves platform independence through bytecode. The Java compiler converts code into bytecode, which the JVM interprets for the underlying OS, making Java write-once, run-anywhere.
Q6. What are local and global variables?
● Local variables are declared inside methods or blocks and are accessible only within their scope.
● Global variables (also called instance variables) are declared within a class but outside any method and have a wider scope.
Q7. What is data encapsulation?
Encapsulation is an OOP principle where data (variables) and code (methods) are bundled into a single unit (class). It restricts direct access to data using access modifiers (private, protected).
Q8. What is Method overloading?
Method overloading allows multiple methods to have the same name but different parameter lists. The compiler differentiates them based on the number or type of parameters.
Same Class Name
Same Method Name
Different Parameter(Different by count or different data types)
Q9. What is Method overriding?
Overriding allows a subclass to provide a specific implementation of a method defined in its superclass. It enables dynamic method dispatch (runtime polymorphism).
Different Class Name
Same Method Name
Same parameter
Q10. Why is the main method static in Java?
The main method is static so that it can be called without creating an instance of the class, allowing the program to start execution without object instantiation.
Q11. What is the difference between the throw and throws keywords in Java?
Feature | throw | Throws |
Purpose | Used to explicitly | |
throw an exception | Declares that a | |
method may throw an | ||
exception | ||
Usage | throw new | |
Exception("Error") | public void | |
myMethod() throws | ||
IOException | ||
Number of Exception | Can throw one | |
exception at a time | Can declare | |
multiple exceptions | ||
using commas |
Q12. What do you mean by singleton class?
A singleton class ensures that only one instance of the class exists throughout the application's lifecycle. It is implemented using a private constructor, a static instance variable, and a public static method that returns the single instance. The most common way to create a singleton class is using the lazy initialization or eager initialization approach.
Q13. Does every try block need a catch block?
No, a try block does not necessarily need a catch block. It can be followed by either a catch block, a final block, or both. A catch block handles exceptions that may arise in the try block, while a final block ensures that certain code (such as resource clean-up) is executed regardless of whether an exception occurs.
Q14. What is the usage of the super keyword in Java?
The super keyword in Java is used to refer to the parent class. It can be used to:
Call the constructor of the parent class.
Access the parent class’s methods and variables when they are overridden in a subclass.
Differentiate between methods and attributes of the parent and child class when they have the same name.
Q15. What do you mean by the final keyword?
The final keyword is used to restrict modifications in Java. It can be applied in three contexts:
Final variable: Its value cannot be changed once assigned.
Final method: Prevents method overriding in subclasses.
Final class: Prevents inheritance by other classes.
Q16. How is an exception handled in Java?
Java handles exceptions using the try-catch-finally mechanism:
Try block: Contains the code that might generate an exception.
Catch block: Handles the exception and defines what should be done when an error occurs.
Finally block: Executes regardless of whether an exception occurs or not, often used for resource clean-up (e.g., closing files or database connections).
Q17. How can objects in a Java class be prevented from serialization?
Serialization converts an object into a byte stream for storage or transmission. To prevent serialization:
Declare fields as transient to exclude them from serialization.
Implement writeObject() and readObject() methods to control serialization.
Extend NotSerializableException to explicitly prevent serialization.
Q18. What is the difference between a constructor and a method in Java?
Constructor | Method |
It has no return type. | It always has a return type. |
It has a return type void when not returning anything. | |
It always has the same name as the class name. | It can have any name of its choice. |
Q19. Why is reflection used in Java?
Reflection in Java allows a running program to inspect and manipulate its methods, fields, and constructors at runtime. It is commonly used in frameworks, debugging tools, and JavaBeans to dynamically access class properties.
Q20. What are the different types of ClassLoaders in Java?
Java provides three main types of ClassLoaders:
Bootstrap ClassLoader: Loads core Java classes from rt.jar and other essential libraries. It is implemented in native code and does not have a Java class representation.
Extension ClassLoader: Loads classes from the JRE/lib/ext directory or any other specified extension directories. It is implemented as sun.misc.Launcher$ExtClassLoader.
System (Application) ClassLoader: Loads application classes from the classpath (defined by CLASSPATH, -cp, or -classpath options). It is a child of the Extension ClassLoader.
:) Thanks for your Time, Visit the blog daily to get more Interview Questions and Answers.
→ If there is any queries comment down for clarification. Thank you
Subscribe to my newsletter
Read articles from Thilak G directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
