Understanding Variable Declaration in JavaScript: var, let, and const
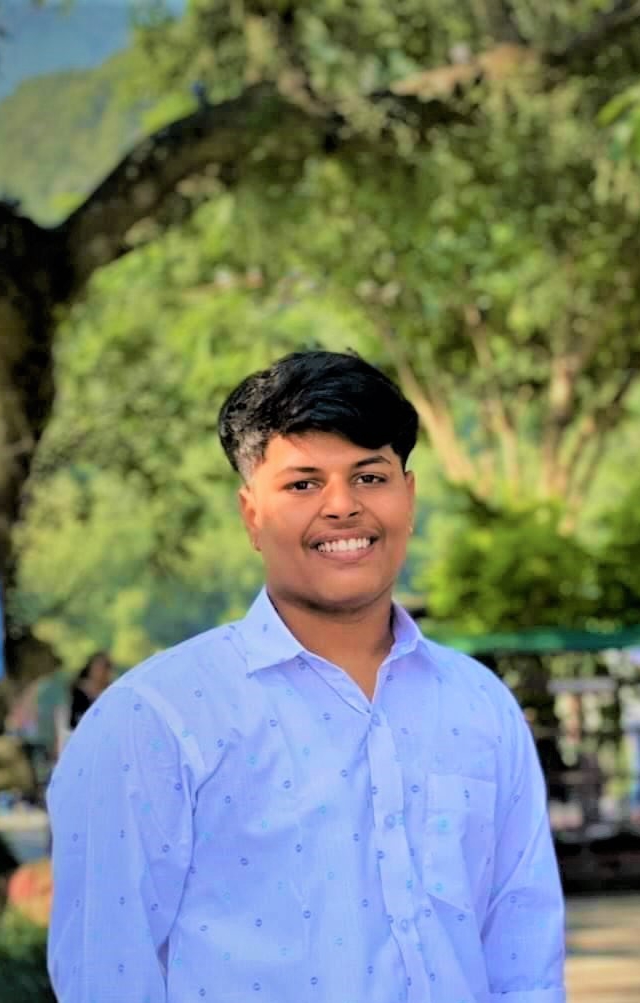
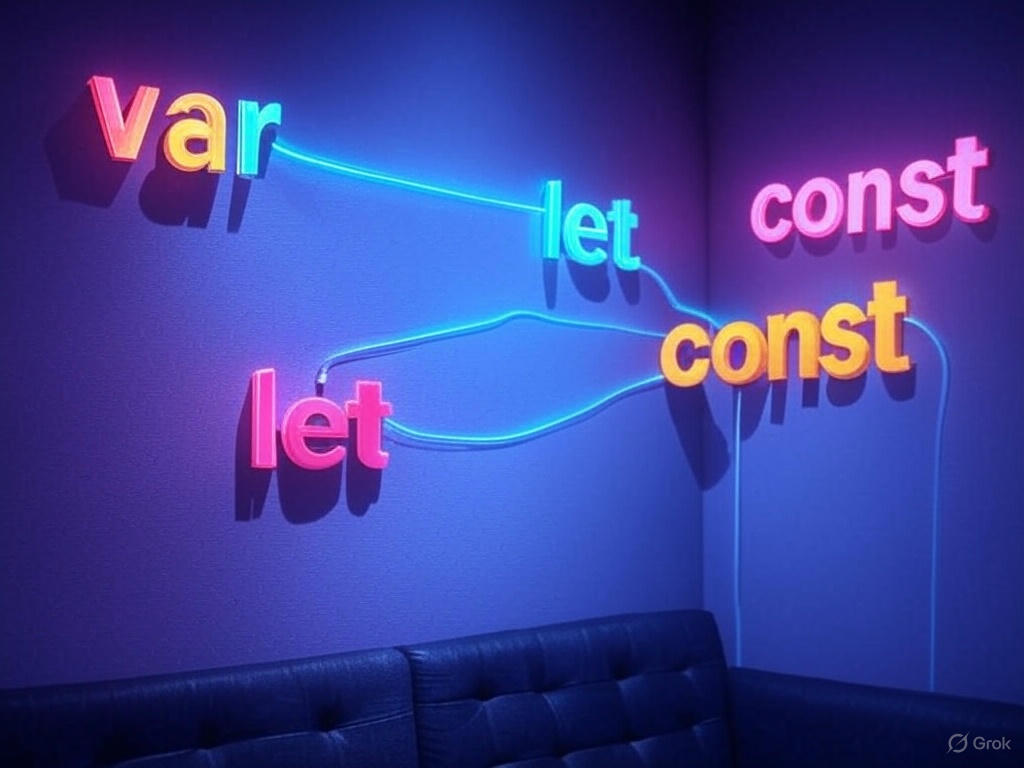
When working with JavaScript, one of the first things you encounter is variable declaration. JavaScript offers three ways to declare variables: var
, let
, and const
. Each has its own use cases, scoping rules, and behaviors. In this blog, we’ll explore their differences, best practices, and when to use each.
1. The Old Way: var
(ES5 and Earlier)
Introduced in the early days of JavaScript, var
was the primary way to declare variables until ES6 (2015).
Key Characteristics:
Function-scoped: Variables declared with
var
are scoped to the nearest function block (not block-scoped likeif
orfor
loops).Hoisting:
var
declarations are hoisted to the top of their scope and initialized withundefined
.Re-declaration Allowed: You can re-declare the same variable multiple times.
Example:
javascript
function example() {
if (true) {
var x = 10;
console.log(x); // 10
}
console.log(x); // 10 (accessible outside the block)
}
example();
console.log(x); // ReferenceError (x is not defined globally)
Issues with var
:
No Block Scoping: Can lead to unintended variable leakage.
Hoisting Quirks: Can cause bugs due to variables being accessible before declaration.
Re-declaration: Can lead to accidental overwrites.
When to Use var
?
Rarely needed in modern JavaScript (legacy codebases may still use it).
Prefer
let
andconst
instead.
2. The Modern Choice: let
(ES6+)
Introduced in ES6, let
provides block-scoping and fixes many issues with var
.
Key Characteristics:
Block-scoped: Only accessible within the nearest
{}
block (e.g.,if
,for
,while
).Hoisting: Still hoisted but remains in the "Temporal Dead Zone" (TDZ) until declared.
No Re-declaration: Cannot re-declare the same variable in the same scope.
Example:
javascript
function example() {
if (true) {
let y = 20;
console.log(y); // 20
}
console.log(y); // ReferenceError (y is block-scoped)
}
example();
When to Use let
?
When you need to reassign a variable (e.g., counters in loops).
When block scoping is required.
3. The Constant Way: const
(ES6+)
Also introduced in ES6, const
is used for variables that should not be reassigned.
Key Characteristics:
Block-scoped: Like
let
, but cannot be reassigned after declaration.Must be Initialized: Must assign a value when declared.
Immutable Binding: The variable reference cannot change, but the object's properties can be modified (if it's an object or array).
Example:
javascript
const PI = 3.14159;
// PI = 3.14; // TypeError (reassignment not allowed)
const person = { name: "Alice" };
person.name = "Bob"; // Allowed (object properties can change)
// person = {}; // Error (reassignment not allowed)
When to Use const
?
For constants (e.g., configuration values).
When you want to prevent accidental reassignment.
Default choice unless you need reassignment (
let
).
Comparison Table: var
vs let
vs const
Feature | var | let | const |
Scope | Function | Block | Block |
Hoisting | Yes (undefined) | Yes (TDZ) | Yes (TDZ) |
Re-declare | Yes | No | No |
Reassign | Yes | Yes | No |
Initialization | Optional | Optional | Required |
Best Practices
Prefer
const
by default – Use it unless you need reassignment.Use
let
when reassignment is needed – For loop counters or dynamic variables.Avoid
var
– Legacy, prone to bugs due to hoisting and scoping issues.Always initialize variables – Prevents
undefined
issues.
Conclusion
var
→ Legacy, function-scoped, avoid in modern code.let
→ Block-scoped, allows reassignment.const
→ Block-scoped, no reassignment (default choice).
By understanding these differences, you can write cleaner, more predictable JavaScript code. 🚀
What’s your preferred way of declaring variables? Let me know in the comments! 😊
Subscribe to my newsletter
Read articles from Bimarsh Pandey directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
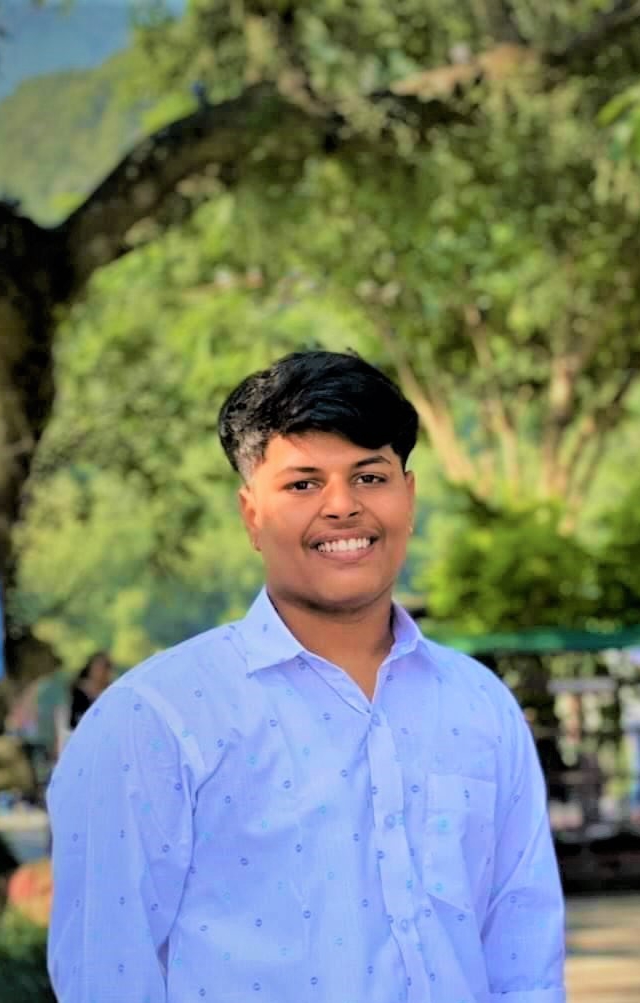