AOT (Ahead-of-Time) Compilation in Spring 6
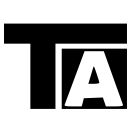
4 min read
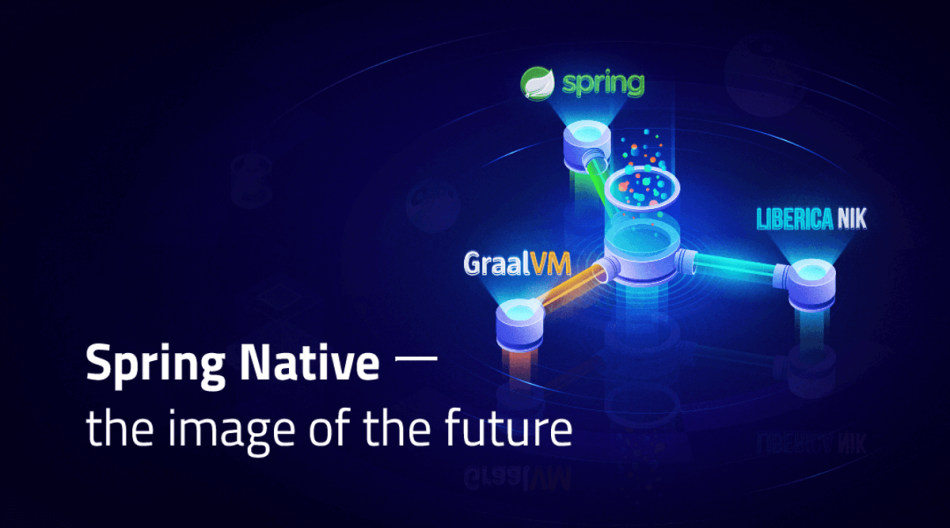
1. What is AOT Compilation?
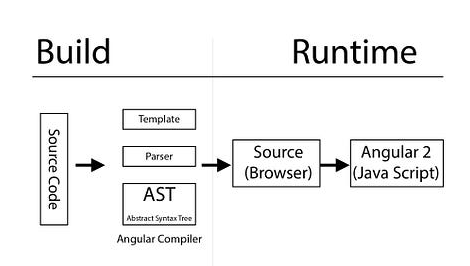
AOT compilation refers to the process of transforming high-level programming code into machine code before the program runs. In traditional Java applications, the Just-in-Time (JIT) compiler compiles code during runtime, which can introduce some delays. With AOT, Spring 6 aims to overcome these delays by performing the compilation ahead of time, which improves startup performance and resource usage.
The AOT feature integrates tightly with GraalVM to build native executables for Spring Boot applications. This also reduces the memory footprint, making it suitable for environments with limited resources.
2. Benefits of AOT in Spring 6
- Faster Startup Time: AOT-compiled Spring Boot applications start much faster than those running on the JVM.
- Reduced Memory Usage: The native image generated by AOT has a significantly smaller memory footprint, beneficial for cloud and containerized environments.
- Performance: Native applications often perform better, particularly for lightweight workloads or microservices architectures.
3. How AOT Works in Spring 6
Spring's AOT compilation works by transforming the application's bytecode into a native executable via GraalVM's Native Image tool. This involves:
- Compiling Java classes into machine code.
- Performing static analysis to remove unnecessary code.
- Using reflection-free mechanisms to replace typical Spring Framework's reflection-based techniques.
Spring AOT produces a native image that includes all the required dependencies and Spring beans, ready to be executed directly by the machine without the need for a JVM.
3.1 Key Components of AOT Compilation
- GraalVM Native Image: The GraalVM toolchain is essential for creating native images from Spring applications. It provides a high-performance runtime with ahead-of-time compilation.
- Reflection-Free: Traditional Spring relies heavily on reflection, which is slow and resource-intensive. The AOT engine replaces reflection-based mechanisms with compile-time analysis.
- Optimized Proxies: Proxies in Spring are typically created at runtime. AOT replaces these with optimized compile-time proxies, leading to faster execution.
4. Setting Up AOT Compilation
Spring Boot provides native support for AOT compilation through the spring-native project, which is now integrated into Spring 6. Here's how you can get started:
4.1 Dependencies
To enable AOT in your Spring 6 project, you'll need to add the following dependencies to your pom.xml (if using Maven):
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-aot</artifactId>
</dependency>
<dependency>
<groupId>org.graalvm.nativeimage</groupId>
<artifactId>svm</artifactId>
<version>${graalvm.version}</version>
<scope>provided</scope>
</dependency>
For Gradle, it would look like this:
implementation 'org.springframework.boot:spring-boot-starter-aot'
implementation 'org.graalvm.nativeimage:svm'
4.2 Building the Native Image
Once your dependencies are set up, you can build a native executable using GraalVM’s native-image command. Run the following Maven or Gradle command:
Maven:
mvn spring-boot:build-image -Pnative
Gradle:
./gradlew bootBuildImage --imageName=<image-name> -Pnative
This will generate a native image that can be executed directly.
5. Example of AOT Compilation in Spring 6
Consider a simple Spring Boot application that runs a REST service. We will demonstrate how to build and run a native image for this application.
5.1 Sample Code
package com.example.aot;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RestController;
@SpringBootApplication
public class AotApplication {
public static void main(String[] args) {
SpringApplication.run(AotApplication.class, args);
}
@RestController
static class AotController {
@GetMapping("/hello")
public String hello() {
return "Hello from AOT compiled Spring!";
}
}
}
5.2 Compiling to Native Image
Add the necessary GraalVM and Spring AOT dependencies.
Run the native-image command to build the executable:
mvn spring-boot:build-image -Pnative
Once the native image is built, you can run it with the following command:
./aot-application
The application will start much faster than it would on the JVM, and you can access the REST endpoint at http://localhost:8080/hello.
6. Challenges and Considerations
- Compatibility: Not all third-party libraries are compatible with AOT compilation. Some reflection-heavy libraries may require additional configuration or GraalVM hints to work correctly.
- Build Time: AOT compilation can significantly increase build times due to the static analysis performed by GraalVM.
- Debugging: Native images are more challenging to debug compared to traditional JVM-based applications due to the lack of certain runtime features.
7. Conclusion
AOT compilation in Spring 6, powered by GraalVM, is a powerful optimization technique that brings improved performance, faster startup times, and reduced memory usage. While it introduces some complexity, particularly around compatibility and build times, the benefits it offers for cloud-native and microservice applications are substantial.
This feature makes Spring 6 a compelling choice for modern Java applications where performance and resource efficiency are critical.
Read more at : AOT (Ahead-of-Time) Compilation in Spring 6
0
Subscribe to my newsletter
Read articles from Tuanhdotnet directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
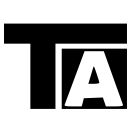
Tuanhdotnet
Tuanhdotnet
I am Tuanh.net. As of 2024, I have accumulated 8 years of experience in backend programming. I am delighted to connect and share my knowledge with everyone.