How to Use React for Beautiful Email Templates with Resend

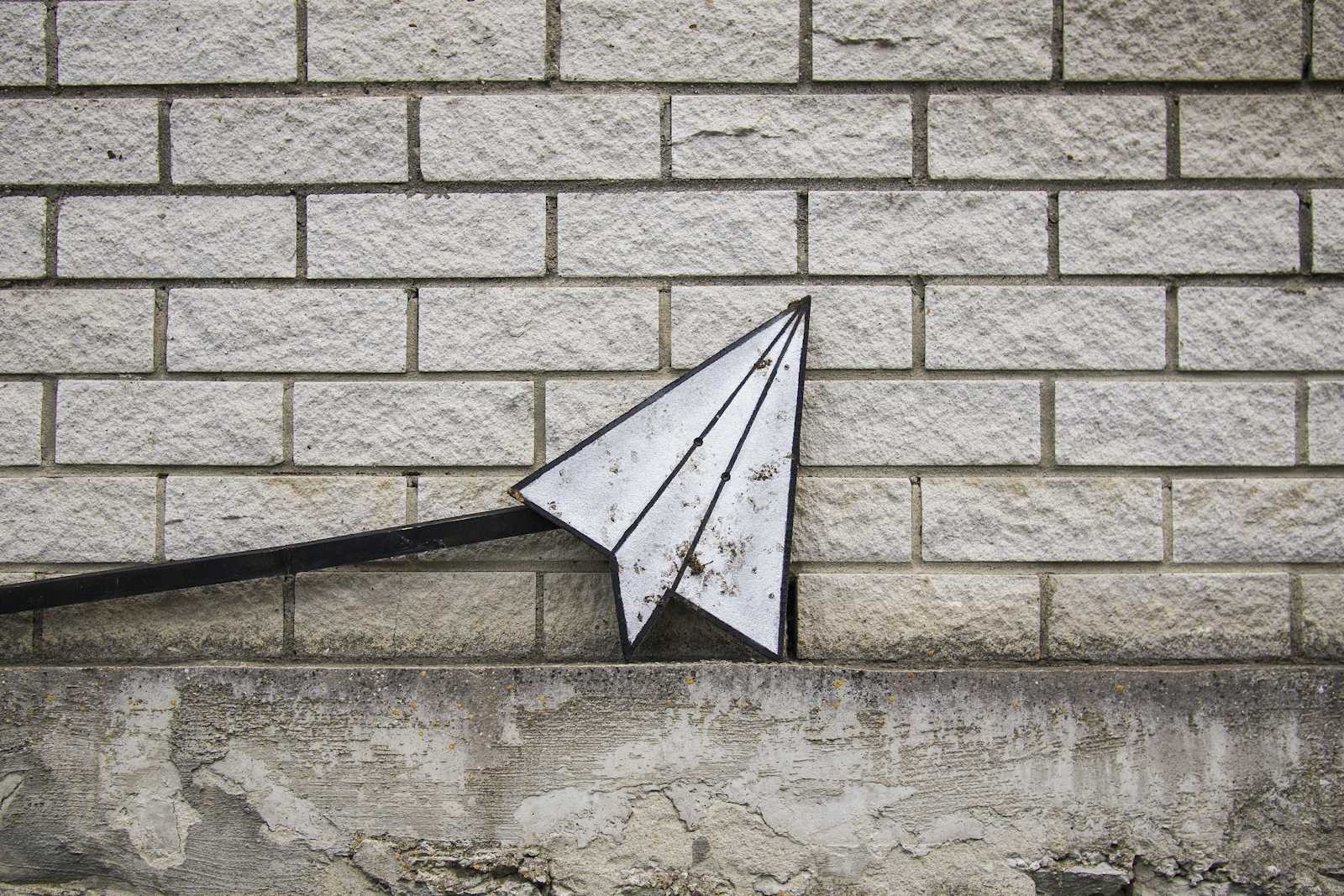
Introduction
Emails are a crucial part of any web application, whether it's for user onboarding, notifications, or marketing. But plain text emails can be dull and lack branding. Wouldn't it be great if you could design emails the same way you build web components? With Resend and React, you can!
In this blog, I'll show you how to use React components to create modular, reusable, and visually appealing email templates.
Setting Up Resend
Resend is an email service that makes it easy to send emails with React. First, head over to Resend and create an account. Then, add the domain of your app and verify it such that you can be able to send emails with something like noreply@yourdomain.com. After that, create an API Key that will be used to authenticate you when sending emails. Now you are good to go!
First, install the Resend package:
npm install resend
Then, initialize Resend in your project:
import { Resend } from 'resend';
const resend = new Resend('your-resend-api-key');
Now, let's build a React email template!
Creating a React Email Template
Resend supports JSX-based email components, making it simple to structure your emails like a regular React component. Here's an example of a sign-up verification email:
import * as React from 'react';
export const SignupEmail = ({ firstName, verificationCode }) => {
return (
<div style={{ fontFamily: 'Arial, sans-serif', padding: '20px' }}>
<h2>Welcome, {firstName}!</h2>
<p>Thank you for signing up. Your verification code is:</p>
<h3 style={{ background: '#f4f4f4', padding: '10px', display: 'inline-block' }}>{verificationCode}</h3>
<p>Enter this code in the app to verify your email.</p>
</div>
);
};
This component takes in a firstName
and a verificationCode
as props to personalize the email for each user.
Sending an Email with React
Once the template is ready, use it in your email-sending function like this:
const sendVerificationEmail = async (receiverEmail, firstName, verificationCode) => {
try {
const response = await resend.emails.send({
from: 'YourApp <no-reply@yourdomain.com>',
to: receiverEmail,
subject: 'Verify Your Email',
react: <SignupEmail firstName={firstName} verificationCode={verificationCode} />,
});
console.log('Email sent successfully:', response);
} catch (error) {
console.error('Error sending email:', error);
}
};
Now, whenever a new user signs up, you can call sendVerificationEmail
with their email, name, and a generated code.
Conclusion
Using React for email templates makes your emails dynamic, modular, and easier to manage. Instead of writing static HTML emails, you can leverage JSX to create beautiful, reusable templates. With Resend, sending these emails is seamless and straightforward.
Give it a try in your next project, and say goodbye to boring emails! ๐
Subscribe to my newsletter
Read articles from Mayimuna Kizza Lugonvu directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
