Android Roadmap for 2025
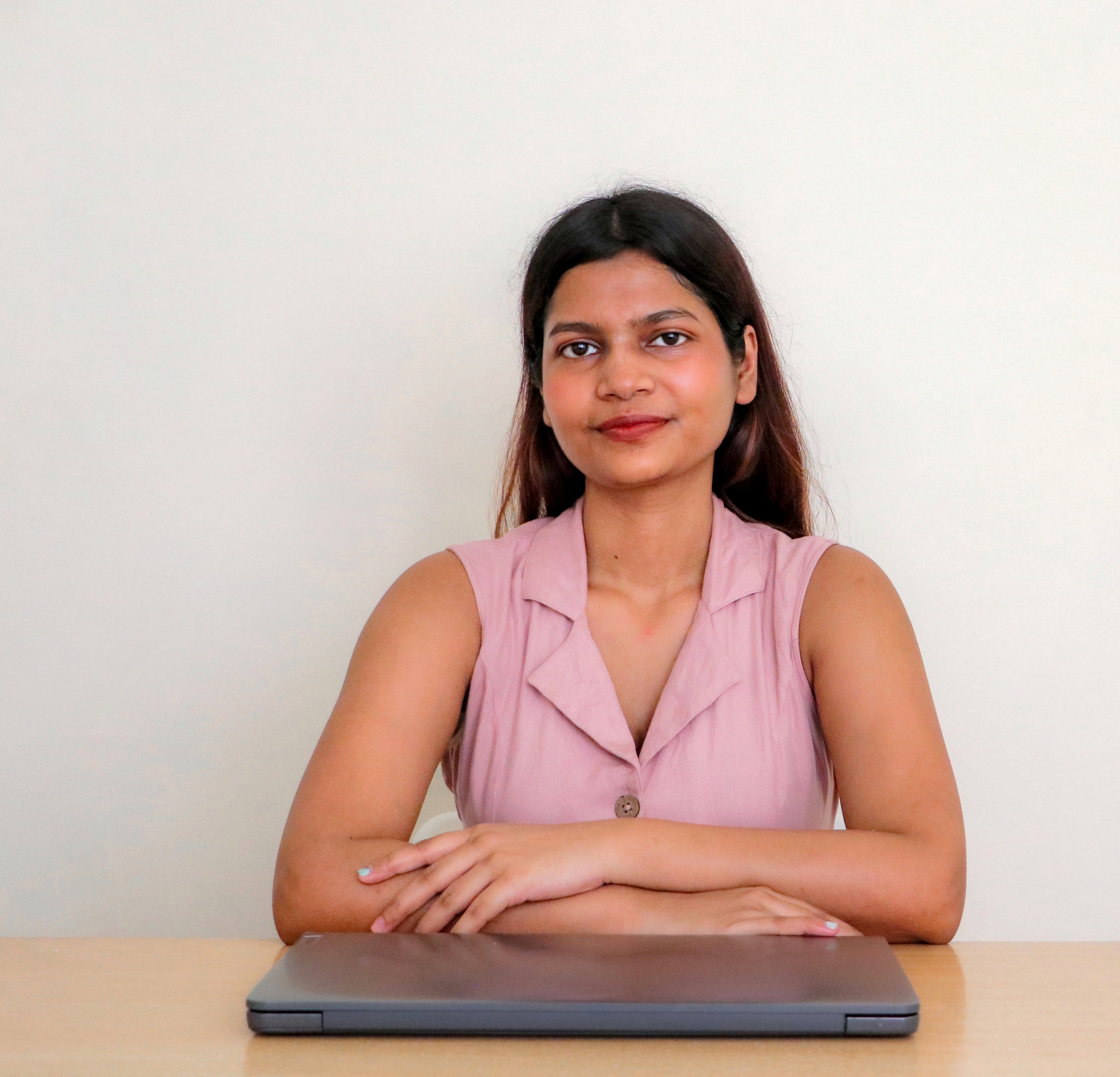
Table of contents

The world of Android development is constantly evolving, and staying industry-ready requires mastering modern tools, frameworks, and best practices. Whether you're a beginner or an experienced developer looking to upgrade your skills, this roadmap will guide you through everything you need to become a proficient Android developer in 2025.
Step 1: Programming Language - Kotlin
Kotlin is a statically typed programming language designed for conciseness and safety, making it a popular choice for Android development. There are many topics one must learn to be good at this language.
These are as follows:
Basics of Kotlin
Syntax and Fundamentals
Control Flow
Functions
Object Oriented Programming in Kotlin
Collections and Data structure
Null Safety
Exception Handling
Functional Programming
File Handling and I/O
Basic of Kotlin includes introduction to Kotlin, setting up the development environment (Android Studio), writing the first Kotlin program (Hello World Program) and Kotlin REPL (Read-Eval-Print Loop)
Understanding Syntax and Fundamentals is essential, covering variables (val
vs var
), data types, type inference, comments, operators (arithmetic, logical, comparison, and bitwise), and string manipulation.
Mastering Control Flow helps in building logic using conditional statements (if
, when
), loops (for
, while
, do-while
), ranges (..
, until
, step
, downTo
), and controlling execution with break and continue statements.
Functions are at the core of Kotlin programming, including function declaration and calling, default and named arguments, single-expression functions, higher-order functions, lambda expressions, inline functions, and tail recursion for optimized recursion.
Kotlin fully supports Object-Oriented Programming (OOP), allowing developers to define classes and objects, use constructors (primary and secondary), manage properties and fields (getters and setters), and control access using visibility modifiers (private, protected, public, internal). It also offers powerful features like inheritance and polymorphism, abstract classes and interfaces, data classes, the object keyword for singletons, companion objects, extension functions, and sealed classes for restricted class hierarchies.
Working with Collections and Data Structures is simplified with support for arrays, lists (mutable and immutable), sets, and maps, along with robust collection operations such as iterating, filtering, sorting, mapping, and reducing.
Kotlin’s Null Safety features, including nullable and non-nullable types, safe calls (?.
), the Elvis operator (?:
), non-null assertions (!!
), and late initialization (lateinit
, lazy
), help prevent NullPointerException
errors.
Handling errors effectively is possible with Exception Handling using try, catch, finally blocks, custom exceptions, and understanding the difference between checked and unchecked exceptions.
Kotlin also embraces Functional Programming, with features like lambda expressions, higher-order functions, inline functions, scope functions (let
, run
, apply
, also
, with
), and recursion.
Lastly, efficient File Handling and I/O is possible in Kotlin, enabling developers to work with reading and writing files, streams, and serialization (JSON, XML) for data processing. These features make Kotlin a powerful, expressive, and safe language for various applications.
Step 2: UI Library - Jetpack Compose
Jetpack Compose is the modern UI toolkit for Android development, enabling declarative and reactive UI building.
To get started, focus on learning:
Setup and Basics of Jetpack Compose
Basics of Compose UI
Managing State in Jetpack Compose
Composable Functions and Reusability
Layouts in Jetpack Compose
Styling and Theming
Handling User Input
Animations in Jetpack Compose
Navigation in Jetpack Compose
Working with Lists and Grids
Dialogs, Snackbars, and Toasts
Handling Side Effects
Working with ViewModel and LiveData
Performance Optimization
Testing in Jetpack Compose
Working with Platform APIs
Advanced Topics in Jetpack Compose
Jetpack Compose for Multiplatform (KMP)
Jetpack Compose is a modern UI toolkit for Android that simplifies UI development with a declarative approach. To begin, it is essential to understand the Basics of Jetpack Compose, including its introduction, setting up the development environment, and learning how to write a simple Composable function (@Composable
) while previewing it in Android Studio.
The Compose UI structure relies on components like Modifier
, layouts (Column
, Row
, Box
), alignment options, and core UI elements like Surface
and Scaffold
. Managing state in Compose is crucial, using tools like remember
, mutableStateOf
, rememberSaveable
, and state hoisting for better composability. Jetpack Compose also emphasizes Composable Functions and Reusability, allowing the creation of custom UI components, optimized rendering with key
, and best practices for designing reusable elements.
For UI layout, Compose offers LazyColumn
and LazyRow
as RecyclerView alternatives and LazyGrid
for dynamic grid-based layouts. Styling and theming in Compose are powered by Material 3, which enables dynamic theming, light/dark mode, and custom typography and colors. Handling user input involves interactive components like buttons, text fields, checkboxes, sliders, and gesture detection for seamless interaction.
Jetpack Compose makes animations intuitive using animate*
APIs, AnimatedVisibility
, Crossfade
, and MotionLayout for complex transitions. Navigation is streamlined with NavHost
, NavController
, and argument passing, supporting deep links and nested navigation. Compose also provides dialogs (AlertDialog
), bottom sheets, snackbars, and toasts for user interactions.
Handling side effects is crucial, using LaunchedEffect
, SideEffect
, DisposableEffect
, and integrating with ViewModel
and LiveData
for efficient data flow. Optimizing performance is key, avoiding unnecessary recompositions with remember
and derivedStateOf
, and improving list rendering in LazyColumn
.
Compose supports UI testing with ComposeTestRule
, Espresso, and debugging tools. Interoperability with Android Views is possible via AndroidView
and ComposeView
, enabling seamless integration with platform APIs like camera and sensors. Advanced topics include custom drawing (Canvas
, Path
), gesture handling, and building custom Composables. Jetpack Compose also extends beyond mobile with Compose for Wear OS, TV, Desktop, and Multiplatform (Kotlin Multiplatform - KMP), making it a versatile UI toolkit for modern Android development.
Step 3: Android Basics
Learn Android Basics like activities, intents, Broadcasts, Service and Resources. Also learn the Basics of SDK, android applications, and app store publishing requirements.
Step 4: Coroutines
To get started, focus on learning:
Introduction to coroutines
Suspending functions
Coroutine scopes (GlobalScope, CoroutineScope)
Coroutine builders (launch, async, withContext)
Structured concurrency
Flow API (Replacing RxJava)
Kotlin coroutines simplify concurrency in Android by providing an efficient way to perform asynchronous tasks without blocking the main thread. Suspending functions allow execution to be paused and resumed without blocking resources, making them ideal for network calls and database operations. Coroutine scopes such as GlobalScope
, CoroutineScope
, and viewModelScope
define the lifecycle of coroutines, ensuring they are properly managed and canceled when no longer needed. Coroutine builders like launch
(for fire-and-forget tasks) and async
(for parallel computations with await
) help execute tasks efficiently, while withContext
allows switching between threads for optimal performance. Structured concurrency ensures coroutines are properly managed within a hierarchy, preventing memory leaks. The Flow API replaces RxJava for reactive programming, providing a cold, asynchronous data stream that supports operators like map
, filter
, and collect
. By leveraging coroutines, developers can write clean, efficient, and responsive Android applications.
Step 5: Database - Room
SQLite is a lightweight, embedded database used in Android for local data storage. However, managing SQLite manually can be complex, which is why Room, a part of Jetpack, provides an abstraction layer over SQLite for easier database handling with compile-time verification, type safety, and minimal boilerplate code.
To get started, focus on learning:
Setting Up Room in an Android Project
Creating a Room Database
Entity
Data Access Object
Using Room in ViewModel
Performing Database Operations
Room with Coroutines and Flow
Handling Migrations
Step 6: Rest APIs - Ktor
Ktor is a modern Kotlin-based framework for making network requests in Android applications. Unlike Retrofit, Ktor is lightweight, asynchronous, and built with coroutines, making it efficient for REST API communication.
To get started, focus on learning:
Setting Up Ktor in an Android Project
Creating a Ktor HTTP Client
Making a GET/POST/PUT Request
Using Ktor in ViewModel
Handling Errors with Try-Catch
Using Ktor with Flow for Live Updates
Cancelling API Requests
Ktor Interceptors for Custom Headers
Using Dependency Injection with Ktor
Step 7: Dependency Injection - KOIN or Dagger Hilt
Koin is a lightweight Dependency Injection (DI) framework for Android and Kotlin. Unlike Dagger/Hilt, Koin is easy to set up, requires no code generation, and uses a Service Locator pattern with a simple DSL (Domain-Specific Language) to define dependencies.
To get started, focus on learning:
Setting up KOIN in Android Project
Defining Dependencies in Koin
Initializing Koin in Application Class
Injecting Dependencies into ViewModel
Injecting Dependencies in Repository & Services
Injecting Dependencies in Jetpack Compose
Koin with Coroutines & Flow
Injecting Dependencies with Parameters
Scopes in Koin (ViewModel, Activity, Fragment)
Step 8: Architecture - MVVM Or MVI
Android apps should follow a clean architecture to improve maintainability, scalability, and testability. The two most popular architectures are MVVM (Model-View-ViewModel) and MVI (Model-View-Intent). MVVM is great for traditional XML-based apps. MVI is better for Jetpack Compose and reactive applications.
Step 9: Reactive Programming - Flows
Reactive Programming allows Android apps to handle asynchronous data streams efficiently. In Kotlin, Flow (part of Kotlin Coroutines) is the recommended reactive API for handling streams of data in Android.
Flow is a cold (lazy) stream that emits multiple values sequentially over time. It replaces RxJava Observables and integrates seamlessly with Coroutines & Jetpack Compose.
To get started, focus on learning:
Creating a Simple Flow
Collecting Flow Data
Flow Operators
Using Flow with ViewModel
Flow Types
Handling Flow with Coroutines
Flow in Jetpack Compose
After Words
I hope you follow this roadmap to enhance your knowledge and become proficient in Android development. Whether you are a beginner just starting or an experienced developer looking to deepen your expertise, this guide covers everything from basics to advanced topics—including Kotlin, Jetpack Compose, MVVM/MVI Architecture, Dependency Injection, Coroutines, Flows, Room Database, REST APIs with Ktor, and more.
By following this structured approach, you will build modern, scalable, and high-performance Android applications. Keep learning, experimenting, and applying these concepts in real-world projects to truly master Android development.
Happy Coding!
Subscribe to my newsletter
Read articles from Jyoti Maurya directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
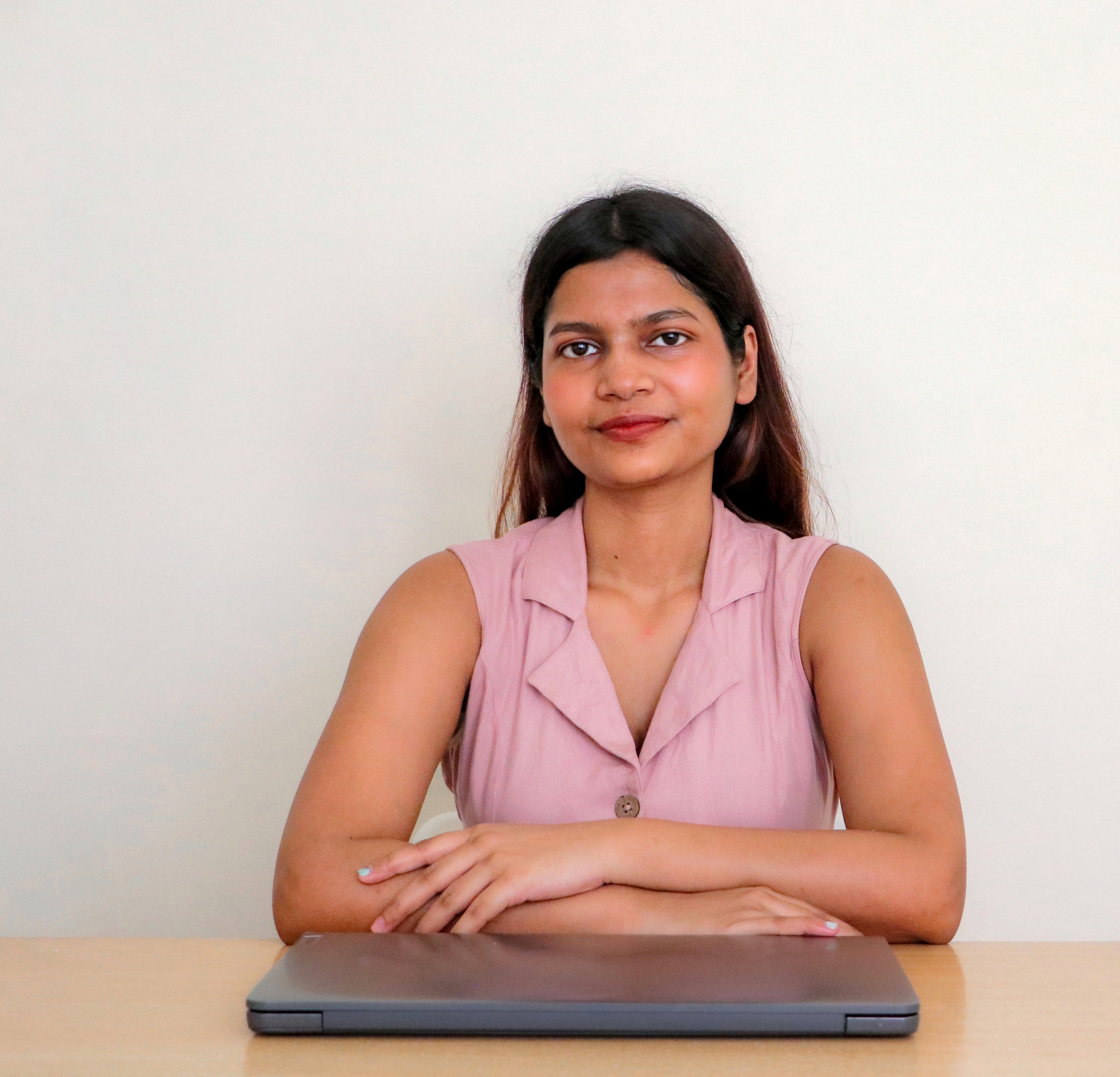
Jyoti Maurya
Jyoti Maurya
I create cross platform mobile apps with AI functionalities. Currently a PhD Scholar at Indira Gandhi Delhi Technical University for Women, Delhi. M.Tech in Artificial Intelligence (AI).