Elixir with Ash. Generated Functions
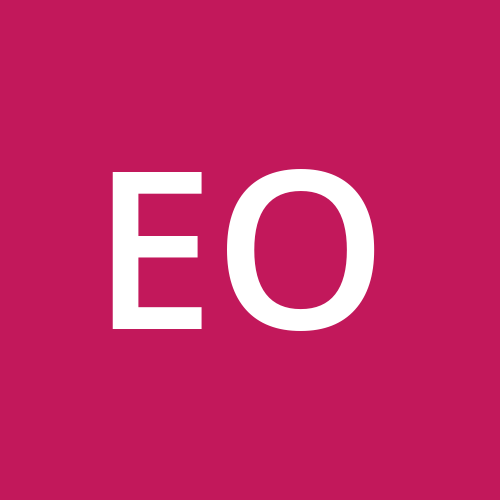
Ash is a declarative framework in Elixir that centers on the idea of a resource. In Ash, your application's entire behavior is driven by how you describe your resources. Describe your resource and derive the rest. Ash is designed for speed and simplicity.
This post assumes you have an intermediate to expert level understanding of elixir.
Generate a new Project
Here is a look at a section of my resource file :
....
actions do
defaults [:create, :read, :destroy]
default_accept [:name, :biography]
read :search do
argument :query, :ci_string do
constraints allow_empty?: true
default ""
end
argument :sort_by, :string, allow_nil?: true, default: nil
argument :direction, :string, allow_nil?: true, default: nil
filter expr(contains(name, ^arg(:query)))
pagination offset?: true, default_limit: 12
end
end
We have an action `search` that takes three arguments, which have default values: nil and an empty string for the query arguments. This ensures flexibility and ease of use!
This section of the resource file is how expose this action to the outside world using `code_interface`.
...
resources do
resource Tunez.Music.Artist do
define :search_artists, action: :search, args: [:query, :sort_by]
end
end
...
This function may look simple at first glance, but what you don't see is that Ash has generated multiple versions of it behind the scenes—each with the same name but different arities. Specifically, an options
argument is always added by default, which can accept various keyword options depending on the action.
You can refer to the documentation for more details, or run your server and use the following command (adjusting for your resource and action names) to explore what's been generated:
h Tunez.Music.search_artists
which returns
def search_artists(query, sort_by, direction, params \ nil, opts \ nil)
Calls the search action on Tunez.Music.Artist.
Options
:page - Pagination options, see Ash.read/2 for more.
• :load (t:term/0) - A load statement to add onto the query
• :max_concurrency (t:non_neg_integer/0) - The maximum number of processes allowed to be started for parallel loading of relationships and calculations. Defaults to System.schedulers_online() * 2
• :lock (t:term/0) - A lock statement to add onto the query
• :return_query? (t:boolean/0) - If true, the query that was ultimately used is returned as a third tuple element. The query goes through many potential changes during a request, potentially adding authorization filters, or replacing relationships for other data layers with their corresponding ids. This option can be used to get the true query that was sent to the data layer. The default value is false.
These are just a few of the available options—I've shortened the full list for brevity. However, here's an interesting observation you might overlook. If you remove the argument definition from the code_interface
in your resource, it now looks like this:
...
resources do
resource Tunez.Music.Artist do
define :search_artists, action: :search
end
end
...
The generated function will now accept any two arguments. So, we'll end up with something like this:
def search_artists(params \ nil, opts \ nil)
We can still pass arguments into search_artists
—the only difference now is that the arguments are passed inside a map, while options
, which defaults to nil
, is a keyword list.
Hope you found this interesting! These code snippets are from the new Ash Framework by Zach Daniel and Rebecca Le. You can check out the full code here.
Subscribe to my newsletter
Read articles from edward omondi directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
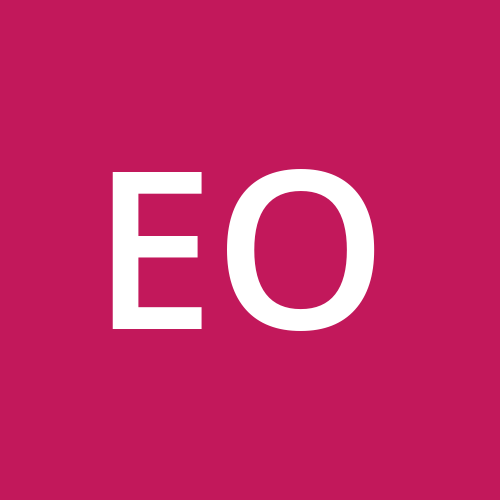