What’s New in C# 14? Key Features and Updates You Need to Know

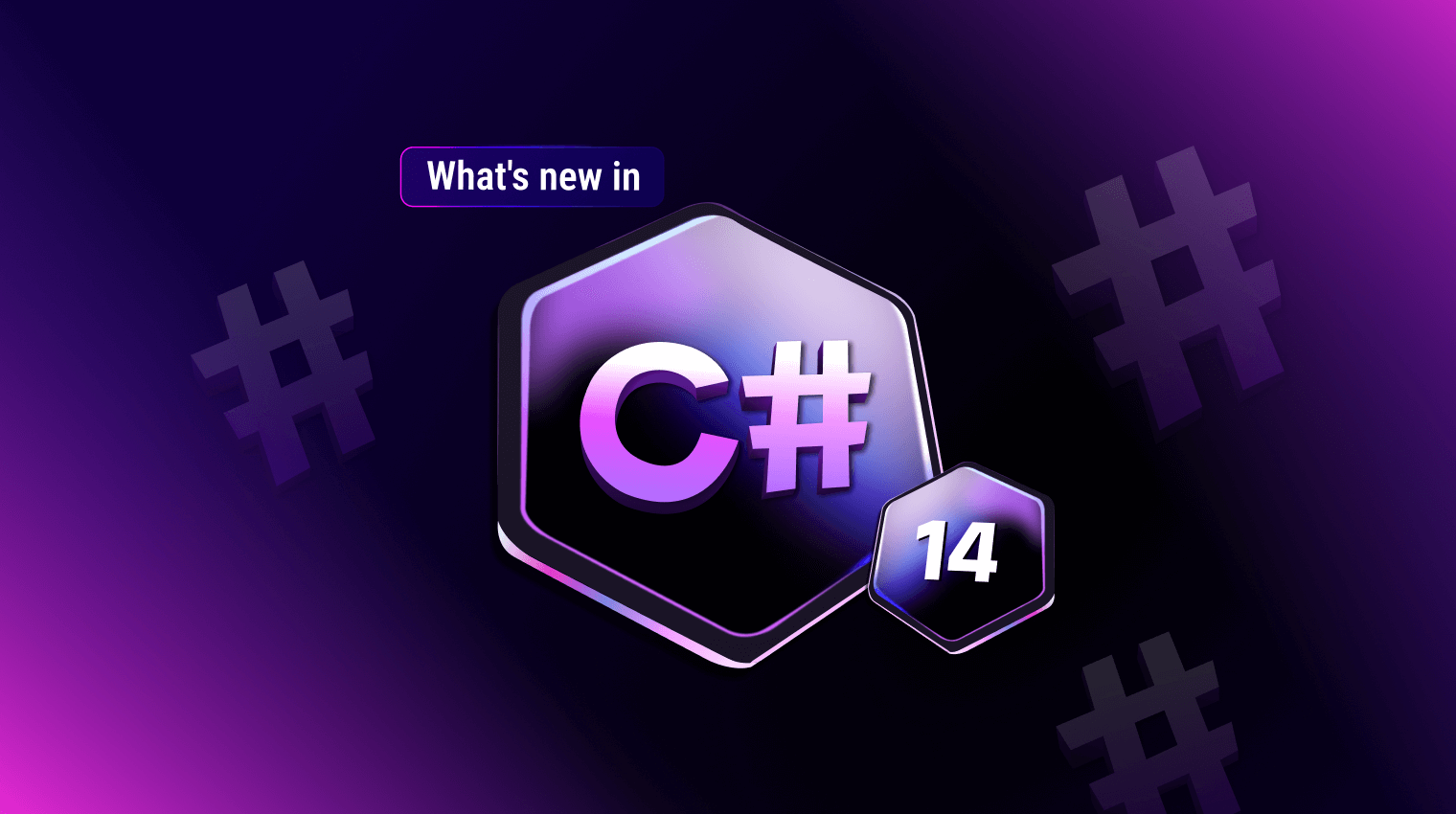
TL;DR: C# 14 introduces features like implicit span conversions, nameof unbound generics, lambda parameter modifiers, the field keyword, and partial events/constructors for improved development.
C#, a cornerstone of the Microsoft ecosystem, continues to evolve, offering developers new tools and improvements to enhance their coding experience. Version 14, expected to be released in November 2025, has a significant set of features in the works to boost productivity, improve code readability, and streamline development workflows. Let’s look at the key additions that C# 14 plans to offer, drawing from available sources.
nameof supports Unbound generic types: Improved reflection capabilities
The nameof operator in C# 14 will gain the ability to work with unbound generic types:
Getting generic type names: You can now use the nameof(List<>), which will evaluate to List.
Contrast with earlier versions: Previously, nameof could only be used with closed generic types like nameof(List) to get the type name.
More implicit conversions for Span and ReadOnlySpan: Native span support
C# 14 will add first-class language support for System.Span and System.ReadOnlySpan, which are types used for high-performance, safe memory access. These types are crucial for scenarios where you need to work with slices of arrays or other memory buffers without creating additional allocations.
Natural span programming: This support will include new implicit conversions, making it more intuitive to work with these types.
Performance and safety: Span and ReadOnlySpan improve performance without compromising safety in various C# and runtime scenarios.
Enhanced interoperability: C# 14 will recognize the relationships among ReadOnlySpan, Span, and T[], supporting certain conversions among them.
Flexibility in usage: Span types will be able to serve as extension method receivers and work seamlessly with other conversions and generic type inference.
The specifics of these implicit conversions can be found in the article on built-in types in the language reference. A more detailed explanation is available in the feature specification for First class span types.
Modifiers on simple Lambda parameters: Increased Lambda expression flexibility
C# 14 will allow the use of parameter modifiers like scoped, ref, in, out, or ref readonly on lambda expression parameters even without explicitly specifying the parameter types:
- Simplified Lambda syntax: This will remove the current requirement of having to declare parameter types when using modifiers.
Example:
delegate bool TryParse<T>(string text, out T result);
TryParse<int> parse1 = (text, out result) =>
Int32.TryParse(text, out result); // Valid in C# 14
// Currently required:
TryParse<int> parse2 = (string text, out int result) =>
Int32.TryParse(text, out result);
- Exception: The params modifier will still necessitate an explicitly typed parameter list.
Further details on these changes can be found in the article on lambda expressions in the C# language reference.
field backed properties: Streamlined property definitions
C# 14 will introduce the contextual keyword field within property accessors:
Implicit backing fields: This keyword allows you to reference the compiler-synthesized backing field of an auto-implemented property without explicitly declaring it.
Simplified null checks: This is particularly useful for implementing logic within property setters, such as null checks.
Example: Instead of:
private string _msg;
public string Message
{
get => _msg;
set => _msg = value ?? throw new ArgumentNullException(nameof(value));
}
You can now write:
public string Message
{
get;
set => field = value ?? throw new ArgumentNullException(nameof(value));
}
Accessor flexibility: You can declare a body for either or both the get and set accessors of a field-backed property.
Potential disambiguation: If a type already has a symbol named field, you can use @field or this.field to differentiate it from the keyword or consider renaming the existing symbol.
Preview in C# 13: It’s worth noting that the field keyword is available as a preview feature in C# 13.
More partial members: Expanding partial type capabilities
C# 14 will be extending the concept of partial members to include instance constructors and events:
Partial constructors and events: You will be able to define the signature of a constructor or event in one part of a partial type and provide its implementation in another.
Defining and implementing declarations: Partial constructors and events will require exactly one defining declaration and one implementing declaration.
Constructor initializers: Only the implementing declaration of a partial constructor can include a constructor initializer this() or base().
Primary constructor syntax: Only one partial type declaration can include the primary constructor syntax.
Event accessors: The implementing declaration of a partial event must include get and remove accessors, while the defining declaration declares a field-like event.
Conclusion
These new features planned for C# 14 will collectively contribute to a more expressive, efficient, and developer-friendly programming language. By understanding and utilizing these enhancements for .NET 10, developers can write cleaner, more maintainable, and better-performing code
If you have questions, contact us through our support forum, support portal, or feedback portal. We are always happy to assist you!
Related Blogs
Subscribe to my newsletter
Read articles from syncfusion directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

syncfusion
syncfusion
Syncfusion provides third-party UI components for React, Vue, Angular, JavaScript, Blazor, .NET MAUI, ASP.NET MVC, Core, WinForms, WPF, UWP and Xamarin.