what is DOM ?

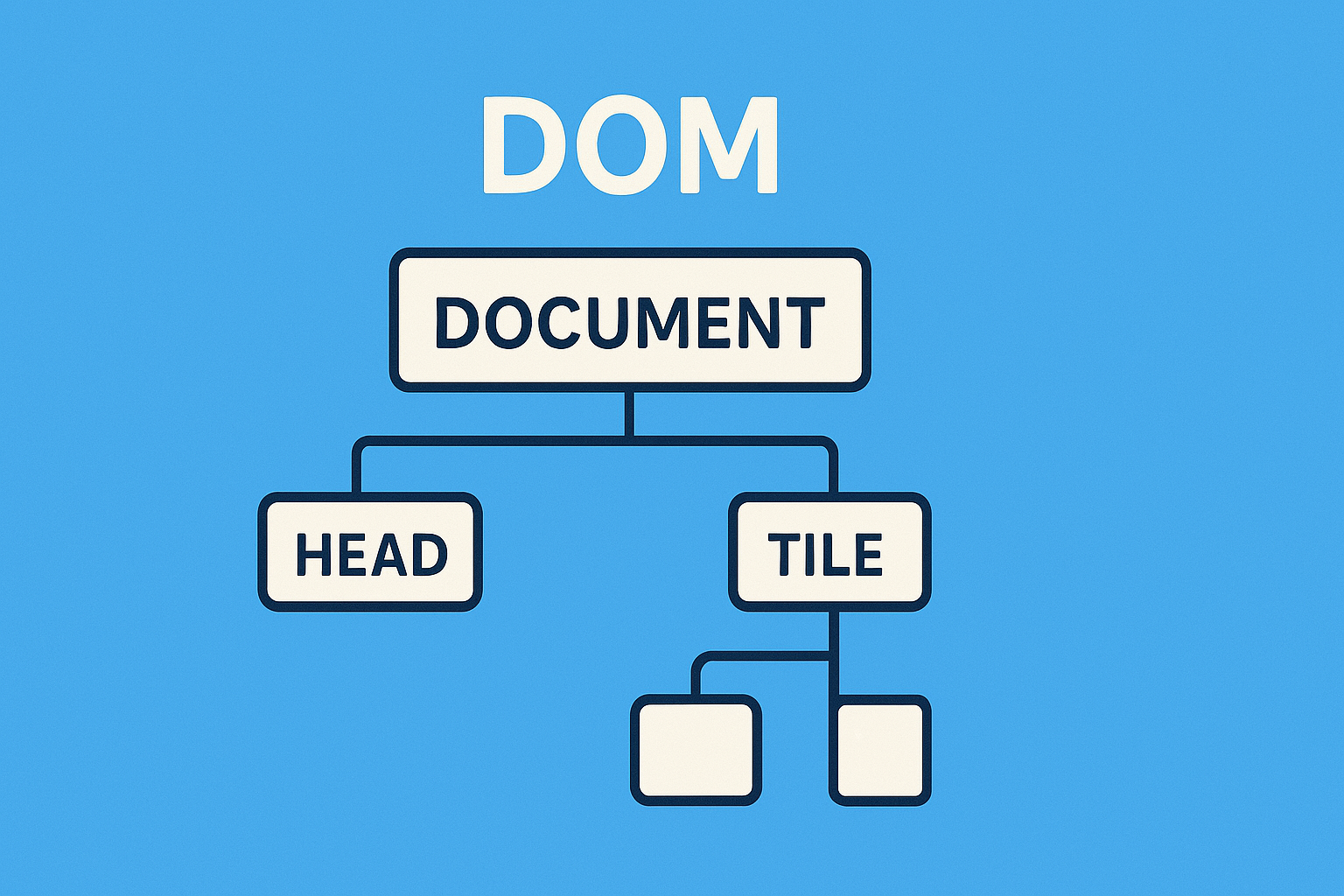
DOM stands for Document Object Model.
DOM is basically a programming interface for web documents. It represents various elements of a web document as a node/object in the form of a hierarchical tree like structure and gives users a functionality of editing the structure by adding or deleting nodes and various other functionalities through programming language.
DOM was introduced in 90’s as a standard way of manipulating web documents, becoming a core part of DHTML (Dynamic HTML). Earlier to DOM the developers faced challenges in targeting and accessing the elements due to the presence of browser-specific implementations.
DOM structure.
Now we know that DOM organizes everything in the form of hierarchical tree, so lets see how it works behind the scenes.
Node representation
Every element in our HTML document including tags, attributes are represented as a node in the DOM.
Root Element
Every document has a root or starting element and generally its the document element it’s the entry point for DOM .
Parent Child relationship
The elements in the html document are represented in a parent child relationship. Every child has a parent, parent can be a child to its own parent.
Example
<html lang="en">
<head>
<title>Document</title>
</head>
<body>
<div>
<h1>HELLO</h1>
</div>
</body>
The DOM for above HTML document would look like:
we can see that the elements are hierarchically arranged in a tree like structure and we can also see the parent-child relationship between the elements.
We can individually target these elements and manipulate the DOM tree as per our need, we have various inbuilt functions for the same in JS.
Selecting and modifying HTML elements in DOM.
For selecting and modifying the elements using html, we have various kind of selectors in JS that can be used for selecting the HTML elements. Each selector has its own purpose associated to it and we can modify various properties . Some of the most common DOM selectors in JS are:
getElementById
Selects an element by its unique id assigned to it.
const element = document.getElementById('myId');
//HTML element having id attribute as "myId" would be selected.
getElementsByClassName
Selects a group of elements associated to a particular class in HTML. Returns an HTML collection.
const elements = document.getElementsByClassName('myClass');
//All the HTML elements having class attribute as "myClass" would be selected.
getElementsByTagName
Selects a group of elements based on a particular tag specified. Returns an HTML collection.
const elements = document.getElementsByTagName('div');
//All the HTML elements with Tag div would be selected
querySelector
Selects the first element that matches a specified CSS selector. Returns a single element or
null
.We can use CSS selectors like “.class_Name” , “#ID_Name”, ”Tag_name”.
const element = document.querySelector('.myClass');
// Selects the first element with class "myClass"
const elementById = document.querySelector('#myUniqueId');
// Selects the first element with Id"myUniqueId"
const firstParagraph = document.querySelector('p');
// Select the first <p> element
querySelectorAll
Selects all elements that match a specified CSS selector. Returns a static NodeList. Selectors are same as for querySelector.
const elements = document.querySelectorAll('div.myClass');
// Selects all <div> elements with class "myClass"
when a group of elements are to be selected, be it using querySelectorAll
or GetElementsByClassName
, generally two types of collection are returned:
NodeList: A NodeList is a collection of nodes that can include elements, text nodes and other types of nodes.
HTMLCollection: An HTMLCollection is a specific type of collection that only contains HTML elements (i.e., it does not include text nodes or comments).
These are some of the most common selectors in JS for targeting the HTML elements and further modifying them. There are also various other Selectors like childNodes
, firstChild
and lastChild
, children
, but those are used less often.
we have used a word quiet often while using the selectors and that’s document. Document is basically the entry point for DOM. It represents the entire HTML document that’s loaded in the browser and provides the ways to manipulate it.
Modifying the DOM
we have various kinds of modification available for the DOM elements, once they are selected. Some of the most common modifications that can be made to DOM elements are:
const element = document.getElementById('myId');
//HTML element having id attribute as "myId" would be selected.
element.textContent = 'New Text Content';
// Changes the text inside the element
element.innerHTML = '<strong>New HTML Content</strong>';
// Changes the inner HTML
element.setAttribute('class', 'newClass');
// Changes the class attribute
element.className = 'newClass';
// Another way to change the class
element.style.color = 'red';
// Changes the text color to red
element.style.backgroundColor = 'yellow';
// Changes the background color to yellow
element.classList.add('newClass');
// Adds a new class
element.classList.remove('oldClass');
// Removes an existing class
element.classList.toggle('toggleClass');
// Toggles a class on or off
These are some of the most common modifications that can be made to a selected element in DOM.
Creating and removing elements using DOM
We can create and HTML element and append it to HTML document using DOM:
const newElement = document.createElement('div');
// Create a new <div> element
newElement.textContent = 'I am a new element!';
document.body.appendChild(newElement);
// Append the new element to the body
Output:
CSS is used for setting height, width, background-color of the div.
we can also remove elements from DOM tree using the DOM like:
const element = document.querySelector('#myElement');
element.remove();
// Removes the element from the DOM
// Removing the child element of a parent element
const parent = element.parentNode;
parent.removeChild(element);
// Removes the element using its parent.
My Challenges
While learning DOM I personally faced various challenges, some of them are
Selector usage: while using selectors like
querySelector
andquerySelectorAll
we have to use (.) and (#) for selecting elements with class name or ID. I made this mistake quiet a few times and used to wonder why I wasn’t getting the desired result.Appending the element: when we are creating the elements using DOM and modifying their properties they might not be visible on the webpage as we need to append them to HTML body, by using document.body.appendChild() then only the element will be visible on webpage.
These were some of the mistakes I made while learning DOM. I have mentioned them for a reference if someone encounters the similar situation while learning DOM.
Conclusion
In conclusion we can say that DOM acts as a bridge between HTML document and the programming languages. It allows developers to create dynamic behaviors on the webpages. DOM also allows manipulation of various HTML elements through inbuilt selectors and modifiers. I have also mentioned various challenges that I faced while learning DOM.
If this article adds even a bit to your knowledge, please leave a like to show your appreciation. ☺️🚀
Thanks for watching.☺️
Subscribe to my newsletter
Read articles from Sumrit Gaba directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
