Docker fundamentals š³

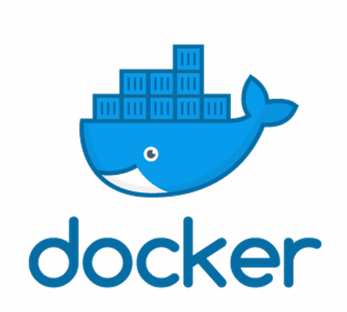
Introduction
What is Docker?
Docker is a platform that allows you to build, ship, and run applications in containers. A container is a standardized unit of software that packages up code and its dependencies so the application runs quickly and reliably across different computing environments.
Why Use Docker?
Portability: Docker containers can run on any system that supports Docker, making your applications highly portable.
Isolation: Each container is isolated from other containers and the host system, ensuring that your applications are not affected by other processes.
Efficiency: Docker containers are lightweight and start up quickly, making them ideal for microservices architectures.
Scalability: Docker makes it easy to scale your applications horizontally by adding more containers.
Prerequisites
Before you begin, make sure you have the following:
A basic understanding of Linux and the command-line interface.
A Docker account (optional, but recommended for accessing Docker Hub).What is docker ?
ā Docker is a free and open-source software used for containerization.
ā Docker is a tool to create, run and deploy application using containers
ā containers = (Application code + Application dependencies (libraries, framework runtime etc)) PACKAGE
Docker Architecture:
Docker's architecture is designed to enable the efficient building, shipping, and running of containerized applications. Below is an overview of its components and how they interact.**
Docker Client
Docker-Daemon(dockered)
Docked Images
Docker Containers
Docker Network
Docker Registry
Docker Engine:**
The Docker Engine is the heart of the Docker platform. It comprises two main components:
A) Docker Daemon (dockerd): The Docker daemon runs on the host machine and is responsible for managing Docker objects, such as images, containers, networks, and volumes.
B) Docker Client: The Docker client is a command-line interface (CLI) tool that allows users to interact with the Docker daemon through commands. Users can build, run, stop, and manage Docker containers using the Docker CLI.
Docker Images:
Docker images are the building blocks of containers. They are read-only templates that contain the application code, runtime, system tools, libraries, and other dependencies. Docker images are created from Dockerfiles, which are text files containing instructions for building the image layer by layer.
Docker Containers:
Docker containers are runnable instances of Docker images. They encapsulate the application and its dependencies, providing an isolated environment for execution. Containers can be created, started, stopped, moved, and deleted using Docker commands
Docker Registry:
Docker Registry is a centralized repository for storing and sharing Docker images. The default public registry is Docker Hub, where users can find a vast collection of images. Organizations can also set up private registries to store proprietary images securely.
Docker Networking:
Docker provides networking capabilities for containers to communicate with each other and with external networks. It uses software-defined networks (SDN) to create virtual networks, enabling connectivity and isolation. Users can create custom networks, connect containers to networks, and define network policies using Docker commands or Docker Compose.
Docker workflow
The Docker workflow is a series of steps that developers and DevOps teams follow to build, ship, and run applications using Docker. It ensures consistency, efficiency, and portability across different environments. Hereās a breakdown of the typical Docker workflow:
STEP 1: - Create a Dockerfile
Docker file is a text file containing instructions to build the docker image, to write the docker file we need to use the below keywords, they are
a) FROM: used to specify base image for our application
example: FROM ubuntu: latest ``FROM node:18-alpine
b) RUN: used to specify the instructions to execute at the time of docker image creation
NOTE : we can write multiple run instructions in a single Dockerfile and all those instructions will be processed in the order
example: RUN apt-get update && apt-get install -y nginx ``RUN yum update -y
RUN yarn install āproduction
c) MAINTAINER: used to specify the author of the Docker file
example: MAINTAINER SAIPRASAD
Sai Prasad Shetty
d) CMD : used to specify instructions to execute at the time of docker container creation.
example: CMD [ānodeā, āsrc/index.jsā]
CMD ['java -jar app.jar'] ``CMD [app.py]
Note: We can write multiple CMD instructions in single docker file but docker will process only last CMD instruction.
e) ENTRYPOINT : Entrypoint is used to execute instructions when docker container is creating.
Note: CMD instruction we can override using command line arguments where ENTRYPOINT instruction we can't override.
Example: ENTRYPOINT ["java", "-jar", "app.jar"]
ENTRYPOINT ["python", "
app.py
"]
f) COPY: used to copy files from host machine to container machine
example: COPY target/app.jar /usr/app/app.jar ``COPY target/app.war /usr/app/tomcat/webapps/app.war ``COPY
app.py
/usr/app/
app.py
g) ADD: It is also used to copy files from source to destination.
example: ADD
https://example.com/file.tar.gz
/app
NOTE: COPY is straight forward for copying a local files, while ADD adds extra features like extracting archives and downloading from URLās.
h) WORKDIR: It is used to set working directory inside the image, to set the location to execute further commands to change the directory.
example: WORKDIR /app
COPY target/app.jar /usr/app/app.jar
WORKDIR /usr/app
CMD 'java -jar app.jar
i) USER: It is used to set USER to run commands
example: USER nonroot
j) EXPOSE: Documents which ports the container listens on (used for networking purposes).
example: EXPOSE 8080
and many more keywords can be used to write the docker file like env, volume, label etcā¦.
Creating a basic docker file for todo-app and deployed as conatainer in an virtual machine
git clone https://github.com/docker/getting-started-app.git
vi Dockerfile (creating a dockerfile)
FROM node:18-alpine
WORKDIR /app
COPY . .
RUN npm install
RUN npm run build
RUN yarn install --production
EXPOSE 3000
CMD ["node", "src/index.js"]
:wq(save and exit the text file)
STEP 2: Build the Docker Image
Use the docker build command to create a Docker image from the Dockerfile. The image is a snapshot of the application and its environment.
docker build -t my-node-app:1.0 .
# -t my-node-app:1.0 : Tags the image with a name (my-node-app ) and version (1.0 )
# . : Specifies the build context (current directory where the Docker file is located)
STEP 3: Test the image locally
Run the Docker image as a container on your local machine to test the application
docker run -d -p 3000:3000 my-node-app
-d: Runs the container in the background
-p: Maps a container port to a port on the host machine
STEP 4: Push the Image to a Registry
Once the image is tested and ready, push it to a Docker registry (e.g., Docker Hub, AWS ECR, or a private registry) for storage and sharing
# Log in to Docker Hub (or another registry)
docker login
# Tag the image for the registry
docker tag my-node-app username/my-app:1.0
# Push the image to the registry
docker push username/my-app:1.0
Outcome: The image is uploaded and available in the registry.
STEP 5: Deploy the Image
On another machine (e.g., a production server), pull the image from the registry and run it as a container.
# Pull the image from the registry
docker pull username/my-app:1.0
# Run the image from the registry
docker run -p 3000:3000 username/my-app:1.0
# To run the container in detached mode (in the background), use the -d flag:
docker run -d -p 3000:3000 username/my-app:1.0
basic todo app after deployment
STEP 6: Monitor & Maintain
Use Docker commands to monitor running containers:
bash docker ps
* Troubleshoot containers using logs:
bash docker logs <container-id>
Basic Docker commands
1. Docker Version and Info
Check Docker Version:
docker version
View System-Wide Docker Information:
docker info
2. Images
List Docker Images:
docker images
Pull an Image from a Registry:
docker pull <image-name>:<tag>
Example:
docker pull nginx:latest
Remove an Image:
docker rmi <image-name>:<tag>
3. Containers
Run a New Container:
docker run <options> <image-name>
Example:
docker run -d -p 8080:80 nginx
List Running Containers:
docker ps
List All Containers (Including Stopped):
docker ps -a
Stop a Running Container:
docker stop <container-id>
Start a Stopped Container:
docker start <container-id>
Remove a Container:
docker rm <container-id>
View Logs of a Container:
docker logs <container-id>
4. Docker file
Build an Image from a Docker file:
docker build -t <image-name>:<tag> <path-to-dockerfile>
Example:
docker build -t my-app:1.0 .
5. System Cleanup
Remove Unused Images, Containers, Networks, and Volumes:
bash
docker system prune -a
Subscribe to my newsletter
Read articles from SAI PRASAD ANNAM directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

SAI PRASAD ANNAM
SAI PRASAD ANNAM
Hi there! I'm Sai Prasad Annam, an enthusiastic and aspiring DevOps engineer and Cloud engineer with a passion for integrating development and operations to create seamless, efficient, and automated workflows. I'm driven by the challenges of modern software development and am dedicated to continuous learning and improvement in the DevOps field.