Day 6: React Learning Journey: Mastering Mapping in React
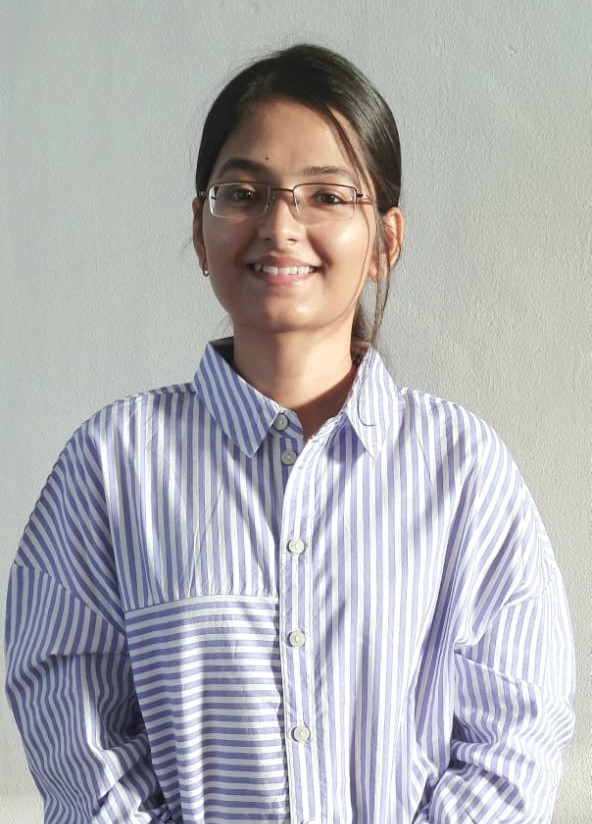
Introduction
Welcome to Day 6 of your React Learning Journey! So far, you’ve explored components, props, and states, which are essential building blocks for any React application. Today, we’ll be focusing on another core concept in React—Mapping. Specifically, we’ll be looking at how to map over arrays and render dynamic content using the map()
method in React.
Let’s dive in and explore how mapping helps you create lists, tables, and other dynamic elements in your React apps.
What is mapping in React?
In React, mapping refers to the process of taking an array of data and transforming it into a list of React elements. This is done using the JavaScriptmap()
function, which creates a new array by calling a function on every element of the original array.
When you have a list of items—whether it's data fetched from an API or static information—you'll often want to render each item as a JSX element. React’smap()
function is perfect for this.
Why Do We Use map()
in React?
In JavaScript, themap()
function is a core method of functional programming. It allows you to transform an array by applying a function to each element and returning a new array with the transformed elements. React leverages this to dynamically render content.
For example, if you have an array of items, you can use Reactmap()
to create a React component for each item and then render it within a parent component. This keeps your code clean and reusable while avoiding hard-coding each element individually.
How Mapping Works in React
At its core, mapping in React is just using JavaScript’s map()
method inside a React component to return JSX elements. Let’s break it down:
Array of data: You need an array, which could come from props, states, or external data (like an API).
Mapping through the array: Use the
map()
method to iterate over the array.Returning JSX: Inside the
map()
function, return JSX elements, which React will render to the DOM.
Mapping Arrays to JSX Elements
Here’s a basic example of mapping an array in React:
const fruits = ['Apple', 'Banana', 'Orange'];
function FruitList() {
return (
<ul>
{fruits.map((fruit, index) => (
<li key={index}>{fruit}</li>
))}
</ul>
);
}
In this example, we take the fruits
array and map over each fruit, returning an <li>
element for each one. The result is an ordered list rendered in the DOM.
Keys in React: Why They Matter in Mapping
Whenever you map over an array in React, you need to provide a special prop called key
. Keys help React identify which items have changed, added, or removed. This is critical for efficient rendering because React uses the key to optimize updates.
{fruits.map((fruit, index) => (
<li key={fruit}>{fruit}</li>
))}
In this case, the fruit
itself serves as the key. React will use these keys to ensure that each list item is tracked correctly.
Best Practices for Using Keys in React Mapping
Avoid using array indexes as keys unless the list is static and will not change. Using the index can lead to performance issues and unexpected UI behavior when the list changes dynamically.
Use unique identifiers for keys whenever possible, such as IDs from an API or database.
Mapping with Dynamic Data
A real-world scenario often involves fetching data from an API and then rendering it in your React component. Here’s an example of mapping over data fetched from an API:
import { useState, useEffect } from 'react';
function UserList() {
const [users, setUsers] = useState([]);
useEffect(() => {
fetch('https://jsonplaceholder.typicode.com/users')
.then(response => response.json())
.then(data => setUsers(data));
}, []);
return (
<ul>
{users.map(user => (
<li key={user.id}>{user.name}</li>
))}
</ul>
);
}
In this example, we fetch a list of users and then map over the users
array to render each user’s name as an <li>
element.
Mapping and Conditional Rendering
Often, you’ll want to combine mapping with conditional rendering. For example, if you want to render a message when the array is empty or only show certain items based on a condition:
const fruits = ['Apple', 'Banana', 'Orange'];
function FruitList() {
return (
<ul>
{fruits.length > 0 ? (
fruits.map((fruit, index) => <li key={fruit}>{fruit}</li>)
) : (
<p>No fruits available.</p>
)}
</ul>
);
}
In this case, we first check if the fruits
array has any items before rendering the list. If it’s empty, we display a fallback message.
Nested Mapping in React
Sometimes, you may need to map over nested data. For example, if you have an array of objects and each object contains another array:
const users = [
{ name: 'John', hobbies: ['Reading', 'Gaming'] },
{ name: 'Jane', hobbies: ['Cooking', 'Hiking'] }
];
function UserHobbies() {
return (
<div>
{users.map((user) => (
<div key={user.name}>
<h2>{user.name}</h2>
<ul>
{user.hobbies.map(hobby => (
<li key={hobby}>{hobby}</li>
))}
</ul>
</div>
))}
</div>
);
}
Here, we first map over the users
array and then, inside the mapped user, we map again over the hobbies
array.
Common Errors When Mapping in React
Forgetting to include a key: React will throw a warning if you don’t provide a
key
prop when mapping.Using non-unique keys: Using duplicate or unstable keys (like array indexes) can lead to unexpected behaviour, especially when the list changes dynamically.
Optimizing Performance When Mapping Large Lists
For rendering large lists, performance can become an issue. One common solution is to use libraries like react-window
or react-virtualized
, which render only the visible items in a list, improving performance.
import { FixedSizeList as List } from 'react-window';
function BigList({ items }) {
return (
<List
height={
500}
itemCount={items.length}
itemSize={35}
width={300}
>
{({ index, style }) => (
<div style={style}>
{items[index]}
</div>
)}
</List>
);
}
This approach is especially useful for rendering thousands of items efficiently without affecting performance.
Handling Empty or Undefined Data in Mapping
When dealing with dynamic data, it’s important to handle scenarios where the data might be empty, null, or undefined. Always ensure you have fallback logic to render something else when data isn’t available.
function FruitList({ fruits }) {
return (
<ul>
{fruits && fruits.length > 0 ? (
fruits.map((fruit) => <li key={fruit}>{fruit}</li>)
) : (
<p>No fruits available.</p>
)}
</ul>
);
}
Mapping Complex Data Structures
In some cases, you may need to map over complex data structures, such as objects instead of arrays. Here’s how you can map over an object’s keys:
const user = {
name: 'John',
age: 25,
hobbies: ['Reading', 'Gaming']
};
function UserProfile() {
return (
<ul>
{Object.keys(user).map(key => (
<li key={key}>
{key}: {Array.isArray(user[key]) ? user[key].join(', ') : user[key]}
</li>
))}
</ul>
);
}
Here, we useObject.keys()
to map over the keys of the user
object and render each key-value pair.
Conclusion
Mapping in React is a powerful tool that allows you to render lists dynamically based on data arrays. By mastering themap()
function, you can handle dynamic content efficiently, whether it’s static arrays, API data, or complex nested data structures. Understanding how keys work and optimizing performance are crucial aspects of working with lists in React.
As you continue your React journey, practice mapping with different types of data and experiment with conditional rendering and handling edge cases like empty or undefined arrays.
FAQs
Can I map over objects in React?
Yes, you can map over objects by usingObject.keys()
,Object.values()
, orObject.entries()
to extract the keys, values, or key-value pairs.What happens if I forget to add a
key
prop when mapping?
React will log a warning in the console, and you may experience performance issues or incorrect UI updates.How can I prevent unnecessary re-renders when mapping lists?
Ensure that your keys are unique and consistent to help React efficiently manage list updates and avoid rendering items unnecessarily.What’s the difference between
map()
andforEach()
in React?
map()
returns a new array, which is necessary for JSX rendering, whereasforEach()
simply iterates over an array and does not return anything.How do I handle async data with
map()
in React?
You can handle async data by usinguseEffect
to fetch the data and then map over it once it's available in the state.
Subscribe to my newsletter
Read articles from Sonal Diwan directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
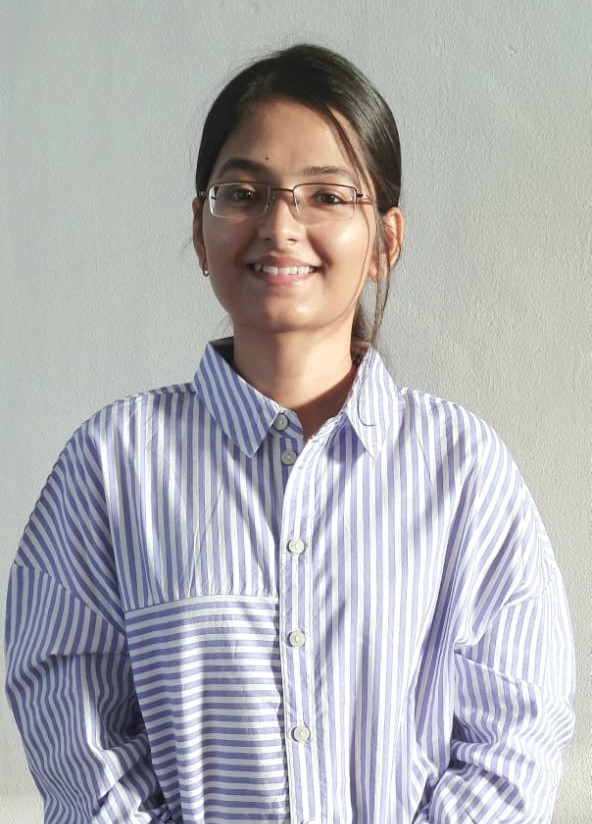
Sonal Diwan
Sonal Diwan
Data-Driven Fresher | Leveraging full stack technologies | Exploring tech-trends