Solving the Pain of JWT Validation: A Developer's Journey to Secure API Authentication
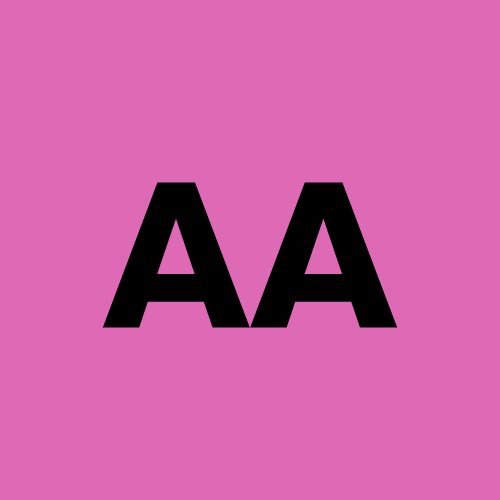
In today’s fast-paced digital world, developers are constantly striving to secure APIs and manage user authentication effectively. One technology that has become a cornerstone in this domain is JSON Web Tokens (JWT). However, anyone who has implemented JWTs knows that while they are powerful, validating and debugging these tokens can be a real headache.
In this blog post, we’ll dive deep into the common challenges developers face with JWT validation, explore best practices for a robust authentication system, and introduce a practical solution—a free JWT Validator tool—that can save you time and effort.
The Pain Points of JWT Validation
Complexity and Misconfigurations
JWTs are designed to be compact, URL-safe tokens that assert claims between two parties. They consist of three parts: a header, a payload, and a signature. While this structure offers flexibility, it also opens the door to misconfigurations and security vulnerabilities. Here are some common challenges:
Secret Management: Developers often struggle with managing secret keys. Using an incorrect or outdated key can lead to token verification failures.
Token Expiry: Handling token expiration is critical. If not configured properly, expired tokens can either grant unintended access or block legitimate users.
Algorithm Mismatch: JWTs support multiple signature algorithms. A mismatch between the algorithm used to sign the token and the one expected during validation can lead to errors.
Invalid Claims: Incorrect or missing claims in the payload can create confusion during the validation process, causing security loopholes.
Debugging Challenges
Debugging JWTs is far from straightforward. When a token fails validation, it’s often unclear whether the issue lies in the token's structure, the signature, or the claim values. Common debugging challenges include:
Lack of Clear Error Messages: Many JWT libraries do not provide descriptive error messages, making it difficult to pinpoint the problem.
Complex Token Structure: Understanding the encoded parts of the token requires manual decoding, which is not only time-consuming but also error-prone.
Interoperability Issues: When tokens are issued and consumed by different systems, inconsistencies in JWT implementation can cause unexpected issues.
Security Concerns
From a security perspective, mishandling JWT validation can be catastrophic. A minor oversight might leave your API vulnerable to token forgery, replay attacks, or other forms of exploitation. Developers need to ensure that:
Tokens are properly signed and validated: This ensures that they haven’t been tampered with.
Claims are verified correctly: For example, ensuring the correct issuer (
iss
), audience (aud
), and expiration (exp
) claims are present.No sensitive data is leaked: Even though JWTs are encoded, they are not encrypted by default.
Best Practices for JWT Validation
Before we explore our solution, let’s review some best practices that every developer should keep in mind when working with JWTs.
1. Use Robust Libraries
Leverage well-maintained libraries that support JWT operations. Popular libraries such as jsonwebtoken for Node.js or pyjwt for Python are a good starting point. These libraries not only simplify token creation and verification but also incorporate essential security measures.
2. Always Validate Token Structure
Ensure that the JWT is well-formed by validating its structure (header, payload, and signature). Use base64 decoding to inspect each part of the token and verify that they meet the expected format.
3. Validate Signature and Claims
Signature: The core of JWT validation is verifying the signature. Ensure you use the correct secret key or public key (if using asymmetric encryption) to confirm the token’s integrity.
Claims: Check that all necessary claims are present and valid. This includes the issuer, audience, and expiration time. For example, if a token is expired, it should be rejected immediately.
4. Handle Token Expiry Gracefully
Implement a strategy to refresh tokens securely. When a token is close to expiring, consider using a refresh token mechanism to issue a new JWT without forcing the user to re-authenticate.
5. Secure Token Storage
Never store sensitive JWTs in insecure places. On the client side, avoid storing tokens in local storage where they might be vulnerable to XSS attacks. Instead, use secure HTTP-only cookies to store JWTs.
Introducing a Practical Solution: The Free JWT Validator Tool
After facing numerous issues with JWT validation in real-world projects, I decided to build a tool that addresses these pain points directly. This Free JWT Validator Tool is designed with developers in mind, offering a streamlined process to validate and debug JWTs effortlessly.
Key Features of the JWT Validator Tool
Validate with Secret or JWKS URL: Whether you’re using a shared secret key or a JWKS (JSON Web Key Set) endpoint, our tool allows you to validate JWTs quickly and accurately.
Instant Debugging: The tool provides clear error messages and feedback on token issues, making it easier to pinpoint and resolve problems.
No Data Storage: Your security is paramount. The tool does not store any token data, ensuring that your sensitive information remains confidential.
User-Friendly Interface: With a clean, developer-centric interface, you can easily paste your token and view detailed validation results in real time.
How the Tool Can Simplify Your Workflow
Imagine you’re in the middle of a critical API development project, and suddenly, JWT validation errors start to block your progress. Instead of sifting through logs or debugging manually, you can simply paste the token into our tool, and within seconds, you’ll know exactly what’s wrong—whether it’s an expired token, a signature mismatch, or a missing claim.
This not only saves you valuable time but also improves your overall development efficiency. Instead of guessing and testing multiple scenarios, you get immediate, actionable feedback.
Why a Developer-Centric Approach Matters
As developers, we appreciate tools that cater to our specific needs without unnecessary fluff. The JWT Validator tool is built with a deep understanding of the common hurdles we face daily:
Time Efficiency: Developers often work under tight deadlines. Having a tool that delivers quick results can be a game-changer.
Clear Insights: With clear error messages and debugging information, you don’t have to second-guess what’s going wrong with your token.
Enhanced Security: By providing robust validation mechanisms, the tool helps ensure that your implementation is secure from common vulnerabilities.
Implementing JWT Validation in Your Project
While the JWT Validator tool is an excellent resource for debugging, integrating JWT validation into your project requires a systematic approach. Here’s a brief overview of how you can incorporate JWT validation into your API authentication process:
Step 1: Choose the Right Library
Depending on your programming language, choose a library that supports JWT operations. For example:
Node.js: Use jsonwebtoken
Python: Use pyjwt
Java: Use java-jwt
Step 2: Configure Your Token Generation
Ensure that when you generate a JWT, you include all necessary claims and use a secure algorithm (e.g., HS256 for symmetric keys or RS256 for asymmetric keys). Here’s a sample snippet in Node.js:
javascriptCopyEditconst jwt = require('jsonwebtoken');
const payload = {
sub: '1234567890',
name: 'John Doe',
admin: true,
iss: 'your-issuer',
aud: 'your-audience'
};
const secret = 'your-secret-key';
const token = jwt.sign(payload, secret, { expiresIn: '1h' });
console.log(token);
Step 3: Validate the Token on Incoming Requests
When your API receives a request, extract the JWT from the request headers and validate it. Here’s how you can do it:
javascriptCopyEditconst express = require('express');
const jwt = require('jsonwebtoken');
const app = express();
app.use((req, res, next) => {
const token = req.headers['authorization']?.split(' ')[1];
if (!token) return res.status(401).send('Token missing');
jwt.verify(token, 'your-secret-key', (err, decoded) => {
if (err) return res.status(403).send('Token invalid');
req.user = decoded;
next();
});
});
app.get('/secure-data', (req, res) => {
res.send('This is secured data');
});
app.listen(3000, () => console.log('Server running on port 3000'));
Step 4: Debugging Token Issues
Whenever you encounter a token validation error, instead of spending hours manually decoding the token, use our free JWT Validator tool. Simply paste the token into the tool, and you’ll receive detailed insights into what might be going wrong.
Conclusion
JWTs have revolutionized API authentication by offering a compact and efficient method of transmitting user claims securely. However, as we’ve discussed, validating and debugging JWTs can present significant challenges, from misconfigurations to unclear error messages. The journey to secure and reliable API authentication demands that developers pay meticulous attention to detail.
To address these challenges, I built a free JWT Validator tool designed specifically for developers. Whether you’re struggling with secret management, debugging token issues, or simply want to ensure that your implementation is secure, this tool is here to streamline your workflow and reduce the friction associated with JWT validation.
Give it a try at https://jwt.compile7.org/ and see how it can help you debug and secure your tokens more efficiently. And as always, I welcome your feedback and suggestions—let’s continue to improve our tools and processes together.
Happy coding, and here’s to building more secure and robust APIs!
Subscribe to my newsletter
Read articles from Andy Agarwal directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
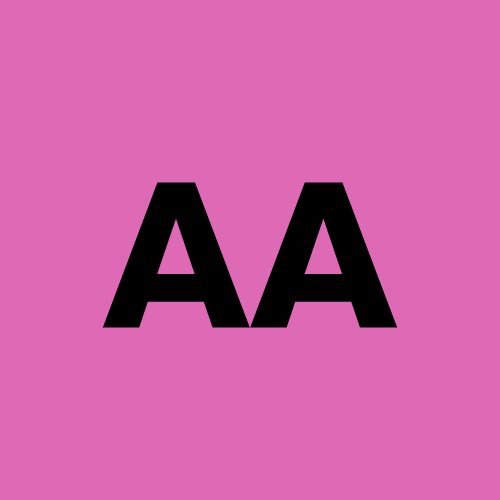