How to Solve Python Deployment Issues: A Guide to Packaging & Production

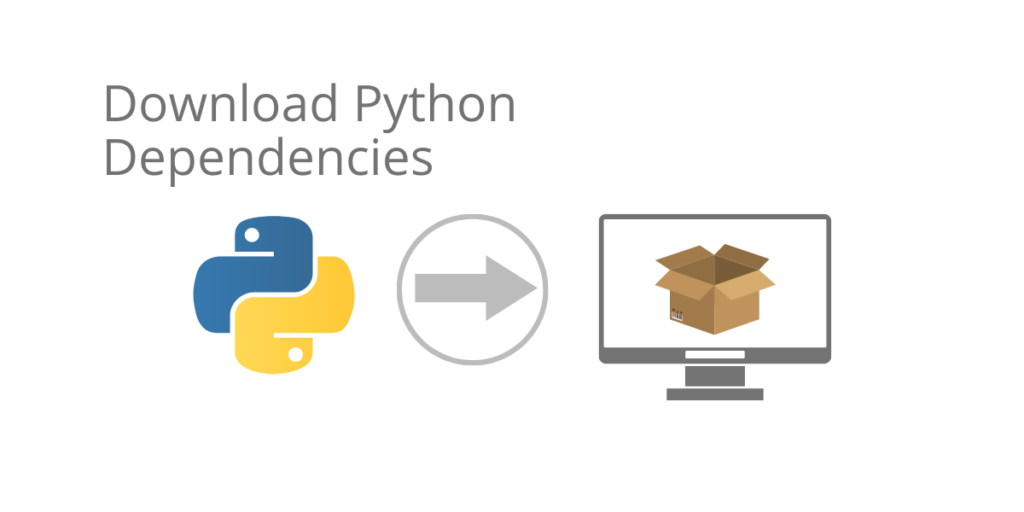
Introduction
When it comes to deploying Python applications, developers often face various challenges. Whether you're working in a local development environment, staging, or production, deploying your Python application to different environments can be tricky. In addition, integrating your application with external systems or services adds more complexity to the process.
In this article, we'll cover the common deployment challenges in Python, explore how to overcome them and discuss best practices for ensuring a smooth deployment process. By the end of this guide, you'll be equipped with the knowledge to confidently deploy your Python applications to production.
Common Challenges in Python Deployment
Environment Discrepancies: Different environments (local, staging, production) often have varying configurations and dependencies. A project that works perfectly in your local environment might not run as expected when deployed to staging or production.
Dependency Management: Python's package management system, though effective, can sometimes lead to conflicts between different versions of libraries. Dependency mismatches can break your application or lead to runtime errors.
Configuration Management: Managing environment-specific configurations, such as database URLs, API keys, and file paths, can be cumbersome. Hardcoding configurations in your application can result in security risks and maintenance headaches.
Scalability and Load Balancing: Deploying applications that handle high traffic and scaling them efficiently can be a challenge. Additionally, handling asynchronous tasks (e.g., background jobs) requires careful consideration.
Solutions to Overcome Deployment Challenges in Python
1. Use Virtual Environments
One of the most common issues developers face when deploying Python applications is ensuring that all dependencies are correctly installed and isolated from the system Python. This can be solved by using virtual environments.
A virtual environment allows you to create an isolated space for your project with its own dependencies, independent of other Python projects on the system.
To create and activate a virtual environment:
# Install virtualenv if you haven't already
pip install virtualenv
# Create a virtual environment
virtualenv venv
# Activate the virtual environment
source venv/bin/activate # On Windows, use `venv\Scripts\activate`
# Install your dependencies
pip install -r requirements.txt
This ensures that your application’s dependencies are isolated and consistent across all environments.
2. Use Docker for Consistency Across Environments
Another excellent approach to handling discrepancies between environments is containerization. Docker allows you to package your application, along with all its dependencies and environment configurations, into a container.
This ensures that the application behaves consistently regardless of where it’s deployed. Docker can eliminate issues such as:
Missing libraries
Version conflicts
OS-specific issues
Create a simple Dockerfile
to package your application:
# Use an official Python runtime as a parent image
FROM python:3.9-slim
# Set the working directory
WORKDIR /app
# Copy the current directory contents into the container at /app
COPY . /app
# Install any needed packages specified in requirements.txt
RUN pip install --no-cache-dir -r requirements.txt
# Make port 5000 available for the app
EXPOSE 5000
# Define environment variable
ENV NAME World
# Run app.py when the container launches
CMD ["python", "app.py"]
This way, you can run the same Docker container on your local machine, staging, and production environments, ensuring consistency across all stages of development.
3. Use Environment Variables for Configuration
Hardcoding sensitive or environment-specific configurations like API keys, database URLs, or other secrets is a security risk. Instead, use environment variables to manage configurations securely.
To load environment variables in Python, you can use the python-dotenv
package:
# Install python-dotenv
pip install python-dotenv
In your Python code, load the variables from a .env
file:
from dotenv import load_dotenv
import os
load_dotenv() # Load environment variables from .env file
API_KEY = os.getenv('API_KEY') # Access the environment variable
This ensures that sensitive configurations are not exposed in your code and can easily be changed depending on the environment.
4. Use CI/CD Pipelines for Automated Deployment
Manual deployment processes can be error-prone and time-consuming. Continuous Integration (CI) and Continuous Deployment (CD) tools can automate the process, ensuring that your application is always up to date.
Popular CI/CD tools for Python deployments include GitHub Actions, GitLab CI, and CircleCI.
For example, in GitHub Actions, you can create a simple .yml
file to automate deployment:
name: Python application
on:
push:
branches:
- main
jobs:
build:
runs-on: ubuntu-latest
steps:
- name: Checkout code
uses: actions/checkout@v2
- name: Set up Python
uses: actions/setup-python@v2
with:
python-version: '3.9'
- name: Install dependencies
run: |
python -m pip install --upgrade pip
pip install -r requirements.txt
- name: Deploy to production
run: |
# Add your deployment script here
echo "Deploying application"
This setup ensures that each time you push to the main
branch, the application is automatically tested and deployed to your production environment.
5. Scaling with WSGI Servers and Load Balancers
To handle increased traffic and ensure high availability, Python applications need to be properly scaled. For web applications, use a WSGI server (Web Server Gateway Interface) such as Gunicorn or uWSGI.
For example, to run a Flask application with Gunicorn:
# Install Gunicorn
pip install gunicorn
# Run the application
gunicorn -w 4 app:app # The -w 4 flag specifies 4 worker processes
Additionally, use a load balancer (such as Nginx) to distribute traffic across multiple instances of your app.
6. Handle Asynchronous Tasks with Celery
For background tasks such as sending emails, processing images, or handling large jobs, you can use Celery. Celery is a distributed task queue that works well with Python and allows you to offload tasks outside the request-response cycle.
To set up Celery, first install it:
pip install celery
Then, configure Celery in your Python app:
from celery import Celery
app = Celery('tasks', broker='redis://localhost:6379/0')
@app.task
def add(x, y):
return x + y
With Celery, you can process long-running tasks asynchronously without blocking your main application, improving scalability and user experience.
Conclusion
Deploying Python applications, while often challenging, can be simplified with the right tools and strategies. By using virtual environments, Docker, environment variables, CI/CD pipelines, WSGI servers, and tools like Celery, you can overcome common deployment obstacles and ensure that your application is stable, secure, and scalable across different environments.
As Python continues to evolve, adopting best practices and automation will allow developers to focus on building great applications rather than worrying about deployment headaches.
Key Takeaways:
Virtual environments ensure consistent dependencies across different stages of development.
Docker eliminates environment discrepancies, allowing for consistent deployment.
Use environment variables to manage sensitive configurations securely.
CI/CD pipelines streamline deployment and reduce the risk of errors.
For high-traffic applications, consider using a WSGI server and load-balancing techniques.
Use Celery for handling asynchronous tasks effectively.
By following these best practices, you’ll be well on your way to solving deployment challenges and ensuring your Python applications run smoothly in production.
Subscribe to my newsletter
Read articles from Binshad directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Binshad
Binshad
💻 Exploring the intersection of technology and finance. 📈 Sharing insights on tech dev, Ai,market trends, and innovation. 💡 Simplifying the complex world of investing