Part 7: Secure Secrets on EKS with AWS Secrets Manager, IRSA & CSI Driver (A to Z)

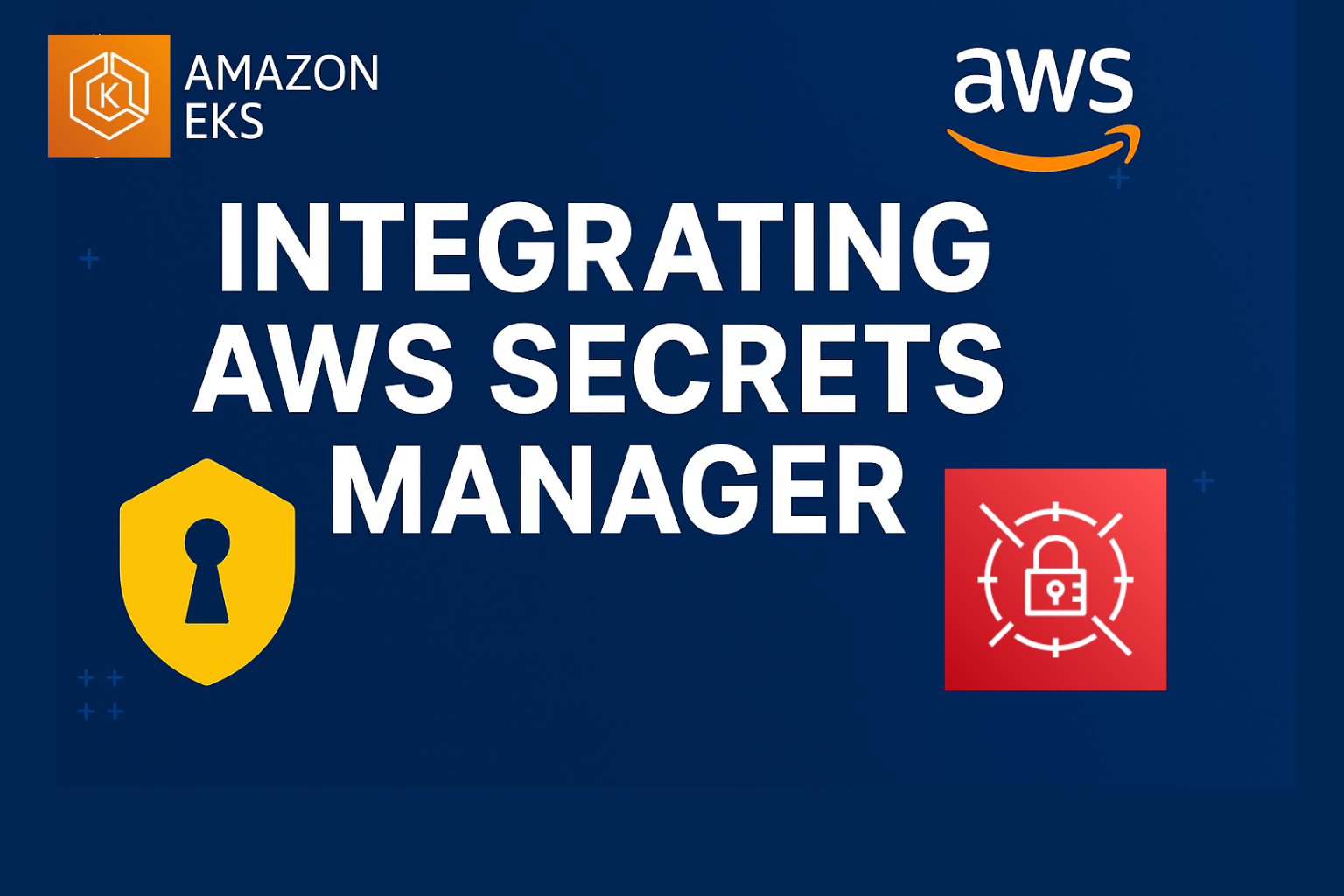
In the final part of my Amazon EKS Production Series, we’re diving into one of the most overlooked yet critical areas of Kubernetes in production: Secret Management. When I first started deploying workloads to EKS, I made the common mistake of storing secrets in ConfigMaps
or hardcoding them as environment variables in YAML manifests. Not only was this insecure, but it made rotating credentials nearly impossible and exposed secrets to version control systems like Git.
I knew I needed something better.
✅ The Goal
We’re going to securely manage and inject secrets into our pods using AWS Secrets Manager, with zero hardcoding and fine-grained IAM control via IRSA (IAM Roles for Service Accounts).
All managed using Terraform + Helm for automation.
⚠️ The Real-World Problems I Faced
Secrets were hardcoded in manifests
No rotation, no audit logging
Git accidentally exposed secrets
Managing secrets manually across environments was painful
IRSA wasn’t set up, so roles couldn’t be assigned to pods
✅ The Secure, Scalable Solution
🔐 Store secrets in AWS Secrets Manager
🔁 Enable OIDC on your EKS cluster (required for IRSA)
🔓 Assign IAM roles to service accounts using IRSA
📦 Use the Secrets Store CSI Driver + AWS Provider
⚙️ Automate everything with Terraform + Helm
📁 Mount secrets as files or inject as environment variables
📌 If You Haven’t Followed Earlier Parts…
Start with foundational components to build your EKS cluster right:
🔗 Part 2: Install Metrics Server for HPA
🔗 Part 5: NGINX Ingress Controller with Cert Manager & HTTPS
📁 GitHub Repository: terraform-eks-production-cluster
Clone this repository and start with the project.
Step-by-Step Setup
Step 1️⃣ – Enable OIDC (Only Once)
If you followed Part 6, your EKS cluster already has OIDC enabled.
Otherwise, enable it using Terraform:
data "tls_certificate" "eks" {
url = aws_eks_cluster.eks.identity[0].oidc[0].issuer
}
resource "aws_iam_openid_connect_provider" "eks" {
client_id_list = ["sts.amazonaws.com"]
thumbprint_list = [data.tls_certificate.eks.certificates[0].sha1_fingerprint]
url = aws_eks_cluster.eks.identity[0].oidc[0].issuer
}
Step 2️⃣ – Create a Secret in AWS Secrets Manager
Use the AWS CLI to create your secret:
aws secretsmanager create-secret \ ─╯
--name staging/myapp-secret-v2 \
--secret-string '{"username":"admin","password":"S3curePassw0rd"}'
Step 3️⃣ – Install Secrets Store CSI Driver + AWS Provider + IRSA Role for Secret Access
21-secrets-store-csi-driver.tf
resource "helm_release" "secrets_csi_driver" {
name = "secrets-store-csi-driver"
repository = "https://kubernetes-sigs.github.io/secrets-store-csi-driver/charts"
chart = "secrets-store-csi-driver"
namespace = "kube-system"
version = "1.4.3"
# MUST be set if you use ENV variables
set {
name = "syncSecret.enabled"
value = true
}
depends_on = [helm_release.efs_csi_driver]
}
resource "helm_release" "secrets_csi_driver_aws_provider" {
name = "secrets-store-csi-driver-provider-aws"
repository = "https://aws.github.io/secrets-store-csi-driver-provider-aws"
chart = "secrets-store-csi-driver-provider-aws"
namespace = "kube-system"
version = "0.3.8"
depends_on = [helm_release.secrets_csi_driver]
}
data "aws_iam_policy_document" "myapp_secrets" {
statement {
actions = ["sts:AssumeRoleWithWebIdentity"]
effect = "Allow"
condition {
test = "StringEquals"
variable = "${replace(aws_iam_openid_connect_provider.eks.url, "https://", "")}:sub"
values = ["system:serviceaccount:12-example:myapp"]
}
principals {
identifiers = [aws_iam_openid_connect_provider.eks.arn]
type = "Federated"
}
}
}
resource "aws_iam_role" "myapp_secrets" {
name = "${aws_eks_cluster.eks.name}-myapp-secrets"
assume_role_policy = data.aws_iam_policy_document.myapp_secrets.json
}
resource "aws_iam_policy" "myapp_secrets" {
name = "${aws_eks_cluster.eks.name}-myapp-secrets"
policy = jsonencode({
Version = "2012-10-17"
Statement = [
{
Effect = "Allow"
Action = [
"secretsmanager:GetSecretValue",
"secretsmanager:DescribeSecret"
]
Resource = "*" # "arn:*:secretsmanager:*:*:secret:my-secret-kkargS"
}
]
})
}
resource "aws_iam_role_policy_attachment" "myapp_secrets" {
policy_arn = aws_iam_policy.myapp_secrets.arn
role = aws_iam_role.myapp_secrets.name
}
output "myapp_secrets_role_arn" {
value = aws_iam_role.myapp_secrets.arn
}
Now apply the config
terraform apply -auto-approve
Copy the output role ARN—you’ll use it in your service account.
Step 5️⃣ – Create the Kubernetes Resources
📁 Folder: 12-aws-secretsmanager-irsa-csi
0-namespace.yaml
---
apiVersion: v1
kind: Namespace
metadata:
name: 12-example
1-secret-provider-class.yaml
---
apiVersion: secrets-store.csi.x-k8s.io/v1
kind: SecretProviderClass
metadata:
name: myapp-aws-secrets
namespace: 12-example
spec:
provider: aws
parameters:
region: us-east-1
objects: |
- objectName: staging/myapp-secret-v2
objectType: secretsmanager
jmesPath:
- path: username
objectAlias: myusername
- path: password
objectAlias: mypassword
secretObjects:
- secretName: myapp-k8s-secret
type: Opaque
data:
- objectName: myusername
key: k8s-myusername
- objectName: mypassword
key: k8s-mypassword
2-service-account.yaml
---
apiVersion: v1
kind: ServiceAccount
metadata:
name: myapp
namespace: 12-example
annotations:
eks.amazonaws.com/role-arn: arn:aws:iam::377027906194:role/production-demo-myapp-secrets
Replace the eks.amazonaws.com/role-arn: value with your actual output role ARN that you copied earlier.
3-deployment.yaml
---
apiVersion: apps/v1
kind: Deployment
metadata:
name: myapp
namespace: 12-example
spec:
replicas: 1
selector:
matchLabels:
app: myapp
template:
metadata:
labels:
app: myapp
spec:
serviceAccountName: myapp
containers:
- name: myapp
image: nginx:1.14.2
ports:
- containerPort: 80
volumeMounts:
- name: secrets
mountPath: /mnt/secrets
readOnly: true
env:
- name: MY_USERNAME
valueFrom:
secretKeyRef:
name: myapp-k8s-secret
key: k8s-myusername
- name: MY_PASSWORD
valueFrom:
secretKeyRef:
name: myapp-k8s-secret
key: k8s-mypassword
volumes:
- name: secrets
csi:
driver: secrets-store.csi.k8s.io
readOnly: true
volumeAttributes:
secretProviderClass: myapp-aws-secrets
🚀 Deploy & Verify
kubectl apply -f 12-aws-secretsmanager-irsa-csi/
kubectl get pods -n 12-example
kubectl exec -it -n 12-example <pod-name> -- bash
# Check secrets as file
cat /mnt/secrets/myusername
cat /mnt/secrets/mypassword
# Or check as environment variable
printenv MY_USERNAME MY_PASSWORD
✅ Congratulations! You've now successfully integrated AWS Secrets Manager with your Amazon EKS cluster, enabling your applications to securely access secrets at runtime—without hardcoding any sensitive values.
Your workloads are now powered by an enterprise-grade secret management system that scales, rotates, and audits—all without touching the AWS Console.
🔍 What You’ve Achieved
✅ Injected secrets into pods as mounted files and environment variables
✅ Eliminated insecure practices like hardcoding or using ConfigMaps
✅ Implemented least-privilege IAM access via IRSA and OIDC
✅ Achieved full auditability and traceability through AWS CloudTrail
✅ Laid the foundation for automatic secret rotation
✅ Automated the entire process using Terraform + Helm for repeatability and DevOps best practices
📢 What’s Coming Next (Bonus Topics?)
The journey doesn't end here. If you'd like to level up your EKS security and automation even further, here’s what we may explore in bonus episodes:
🔄 Automated Secret Rotation & Sync
Configure seamless syncing between AWS Secrets Manager and Kubernetes Secrets with restart triggers for zero-downtime rotation.
🔑 HashiCorp Vault Integration
Integrate HashiCorp Vault as a secure backend for dynamic secret generation, revocation, and fine-tuned RBAC.
🔐 GitOps Secrets with ArgoCD
Learn how to manage secrets in GitOps workflows using sealed secrets or external secret operators with ArgoCD.
Subscribe to my newsletter
Read articles from Neamul Kabir Emon directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Neamul Kabir Emon
Neamul Kabir Emon
Hi! I'm a highly motivated Security and DevOps professional with 7+ years of combined experience. My expertise bridges penetration testing and DevOps engineering, allowing me to deliver a comprehensive security approach.