Advanced docker concepts

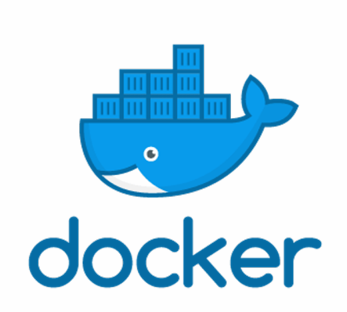
In this artictle we are going to discuss some advanced docker concepts, they are
Port Mapping
2 Multistage DockerfileDocker Networking
Docker compose
Docker volumes
1) PORT MAPPING:
Port Mapping will enable communication b/w Docker containers and outside of the world, it allows server running with containers to be accessed externally via the host’s ip address and port number combination
NOTE: To map port we need to know port number of the containers
To check the port number of a container
docker ps
CONTAINER ID IMAGE COMMAND CREATED STATUS PORTS NAMES
abc123def456 nginx:latest "/docker-entry..." 2 hours ago Up 2 hours 0.0.0.0:8080->80/tcp my_nginx
789ghi012jkl redis:latest "redis-server..." 4 hours ago Up 4 hours 0.0.0.0:6379->6379/tcp my_redis
port mapping command
docker run -p 8080:80 -d --name my_nginx nginx:latest
Here -p : is for port mapping
8080: which we can access the application
80: port number of a container.
2 ) MULTISTAGE DOCKERFILE:
A multi-stage Docker file is a powerful way to optimize your image build process by reducing the size of the final image while maintaining readability and flexibility. It allows you to use multiple FROM instructions in your Docker file to create intermediate stages, which helps keep build dependencies and unnecessary files out of the final image.
Here’s an example of how a multi-stage Docker file works:
# Stage 1: Build stage
FROM golang:1.18 AS builder
WORKDIR /app
COPY . .
RUN go build -o myapp
# Stage 2: Final image stage
FROM alpine:latest
WORKDIR /root/
COPY --from=builder /app/myapp .
ENTRYPOINT ["./myapp"]
Explanation:
Stage 1 (Builder Stage):
This stage uses the
golang:1.18
image and builds the application.It creates the binary
myapp
using thego build
command.Stage 2 (Final Stage):
This stage starts with the
alpine:latest
image, which is smaller and lighter.It copies only the necessary artifact (
myapp
) from the builder stage usingCOPY --from=builder
.The final image is lightweight and contains only the essential files.
Multi-stage builds are especially useful for applications where the build tools or dependencies are large, but the runtime requirements are minimal. It helps improve security and performance by stripping out unnecessary files from the final image.
3) DOCKER NETWORKING:
Docker networks allow containers to communicate with each other, as well as with the host machine, and even external networks. By using networks effectively, you can manage how containers connect and secure their interactions. Docker provides multiple network drivers to suit different use cases.
Types of Docker Networks:
Bridge:
Default network for containers.
Containers within the same bridge network can communicate with each other.
Useful for standalone containers.
Host:
Uses the host machine's network stack.
No isolation; the container shares the host's IP and port space.
Best for performance-sensitive applications.
Overlay:
Enables communication between containers on different hosts in a Docker Swarm.
Great for scaling distributed applications.
None:
Completely disables networking for the container.
Suitable for containers that do not require network access.
Custom Networks:
Created by the user for more granular control.
Allows defining specific subnets, gateways, and more.
Common Docker network Commands:
1) List Docker Networks:
docker network ls
NETWORK ID NAME DRIVER SCOPE
d4e5f6g7h8i9 bridge bridge local
a1b2c3d4e5f6 host host local
a1b2c3d4e5f6 none null local
2) create docker network
docker network create my_network
3) inspect a network
docker network inspect my_network
4) remove or delete docker network
docker network rm my_network
5) remove all unused docker network
docker network prune
6) To connect a running docker container to an existing network
docker network connect my_network my_nginx
7) To remove container from the network
docker network disconnect my_network my_nginx
8) Create a custom network
docker network create --driver bridge my_custom_network
4) Docker Compose:
Docker Compose is a tool that simplifies the management of multi-container Docker Application, instead of manually running multiple docker run commands, we can use Docker Compose to define and our application contains in a single YAML file called a docker-compose.yml
The docker-compose.ym
l file specifies all the services (containers, network and volumes of our application needs)
Key Features:
Multi-Container Setup: Helps you run multiple containers together as a single application.
Configuration Simplification: All configuration is stored in a YAML file.
Service Management: Easily start, stop, or restart services.
Networking: Automatically handles networking between containers.
docker-compose.yml
A docker-compose.yml
file is the configuration file used by Docker Compose to define and manage multi-container applications.
The docker-compose.yml
file contains 4 sections
Version: it represents compose yml version.
Services: it represents containers information(image-name, port mapping etc…).
Network: it represents docker network to run our application.
Volumes: it represents containers storage location.
BASIC DOCKER-COMPOSE.YML file
version: '3.8'
services:
web: # web or application can be mentioned
image: nginx:latest # image that pull from docker hub
ports:
- "8080:80" # port mapping which we can access
volumes:
- ./web:/usr/share/nginx/html # storage location inside the docker container
networks:
- app-network # docker network (it will create while executing file)
database:
image: mysql:latest # image from docker hub
environment:
MYSQL_ROOT_PASSWORD: examplepassword
MYSQL_DATABASE: exampledb
networks:
- app-network # docker network
volumes:
- /data/mysql # storage location inside the docker container
networks:
app-network:
Installing and executing the docker compose:
install docker compose and check the version:
sudo curl -L "https://github.com/docker/compose/releases/latest/download/docker-compose-$(uname -s)-$(uname -m)" -o /usr/local/bin/docker-compose
sudo chmod +x /usr/local/bin/docker-compos
docker-compose --version
Docker Compose version v2.18.1
Executing the docker-compose:
Note: make sure that download docker compose, docker-compose.yml file should be needed, docker images need to be present.
docker-compose up -d
docker-compose ps
Name Command State Ports
---------------------------------------------------------------------------------
projectname_web_1 "nginx -g 'daemon …" Up 0.0.0.0:8080->80/tcp
projectname_database_1 "docker-entrypoint…" Up 3306/tcp
Common Docker-compose commands:
Start Services
docker-compose up #Starts all services defined in the docker-compose.yml file
Run in Detached Mode (Background):
docker-compose up -d
to stop all the containers
docker-compose stop
Stop Services:
docker-compose down #Stops and removes containers, networks, and volumes
Stop the mentioned services
docker-compose stop <services>
Restart Services:
docker-compose restart
View Logs:
docker-compose logs
To list the running conatiners
docker-compose ps
docker-compose top <service> # shows the process of single conainer/service
Remove a Single Service:
docker-compose rm <service_name>
Docker Compose simplifies container orchestration for development and testing environments.
5) Docker volumes:
Volumes are persistent data stores for containers, created and managed by Docker. volumes are used to avoid data loss, using volumes we can make container state full.
stateless container: Data will be deleted after container is deleted
state full container: Data will be available permanently.
NOTE: To maintain data permanently, we need to make docker container as state full
To make docker container as statefull. docker volume comes into place.
you can create a volume explicitly using the docker volume create
command, or Docker can create a volume during container or service creation.
Advantages of Volumes
Persistence: Data stored in a volume is not deleted when the container stops or is removed.
Sharing: Volumes allow multiple containers to share data.
Isolation: Volumes are managed by Docker and are isolated from the host filesystem, improving security.
Flexibility: Can work with different storage drivers and cloud storage backends.
Types of Docker Volumes
1) Anonymous Volumes:
Automatically created when a container runs and mounts a volume. Anonymous volumes are given a random name that's guaranteed to be unique within a given Docker host, anonymous volumes persist even if you remove the container that uses them, except if you use the --rm
flag when creating the container.
Example:
docker run -v /container/path nginx
2) Named Volumes:
Named volumes are created and managed with a user-defined name and specific source, allowing containers to independently share data across. Named volumes are generally recommended for the production environment.
Example:
docker volume create my-volume
docker run -v my-volume:/container/path nginx
3)Host Volumes:
Maps a directory from the host system to the container.
Example:
docker run -v /host/path:/container/path nginx
Syntax
To mount a volume with the docker run
command, you can use either the --mount
or --volume
flag.
docker run --mount type=volume,src=<volume-name>,dst=<mount-path>
docker run --volume <volume-name>:<mount-path>
Options for --mount
The --mount
flag consists of multiple key-value pairs, separated by commas and each consisting of a <key>=<value>
tuple. The order of the keys isn't significant.
docker run --mount type=volume[,src=<volume-name>],dst=<mount-path>[,<key>=<value>...]
Valid options for --mount type=volume
include:
Option | Description |
source , src | The source of the mount. For named volumes, this is the name of the volume. For anonymous volumes, this field is omitted. |
destination , dst , target | The path where the file or directory is mounted in the container. |
volume-subpath | A path to a subdirectory within the volume to mount into the container. The subdirectory must exist in the volume before the volume is mounted to a container. See Mount a volume subdirectory. |
readonly , ro | If present, causes the volume to be mounted into the container as read-only. |
volume-nocopy | If present, data at the destination isn't copied into the volume if the volume is empty. By default, content at the target destination gets copied into a mounted volume if empty. |
volume-opt | Can be specified more than once, takes a key-value pair consisting of the option name and its value. |
Example
docker run --mount type=volume,src=myvolume,dst=/data,ro,volume-subpath=/foo
Create and manage volumes
Creating a volume with name my-vol:
docker volume create my-vol
List volumes:
docker volume ls
local my-vol
Inspect a volume:
docker volume inspect my-vol
[
{
"Driver": "local",
"Labels": {},
"Mountpoint": "/var/lib/docker/volumes/my-vol/_data",
"Name": "my-vol",
"Options": {},
"Scope": "local"
}
]
Remove a volume:
docker volume rm my-vol
Docker volume Commands:
docker volume create my-volume # to create a named volume
docker volume ls # to display all volumes
docker volume inspect my-volume # to inspect a specific(my-volume) volume
docker volume rm <vol-name> # to remove a specific(my-volume) volume
docker volume prune --volumes # to remove unused volumes
Subscribe to my newsletter
Read articles from SAI PRASAD ANNAM directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

SAI PRASAD ANNAM
SAI PRASAD ANNAM
Hi there! I'm Sai Prasad Annam, an enthusiastic and aspiring DevOps engineer and Cloud engineer with a passion for integrating development and operations to create seamless, efficient, and automated workflows. I'm driven by the challenges of modern software development and am dedicated to continuous learning and improvement in the DevOps field.