Write Code That Speaks for Itself:
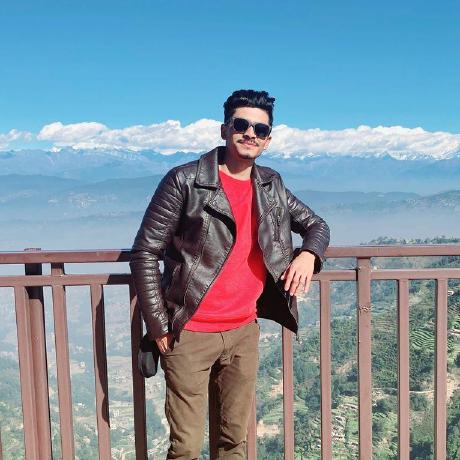
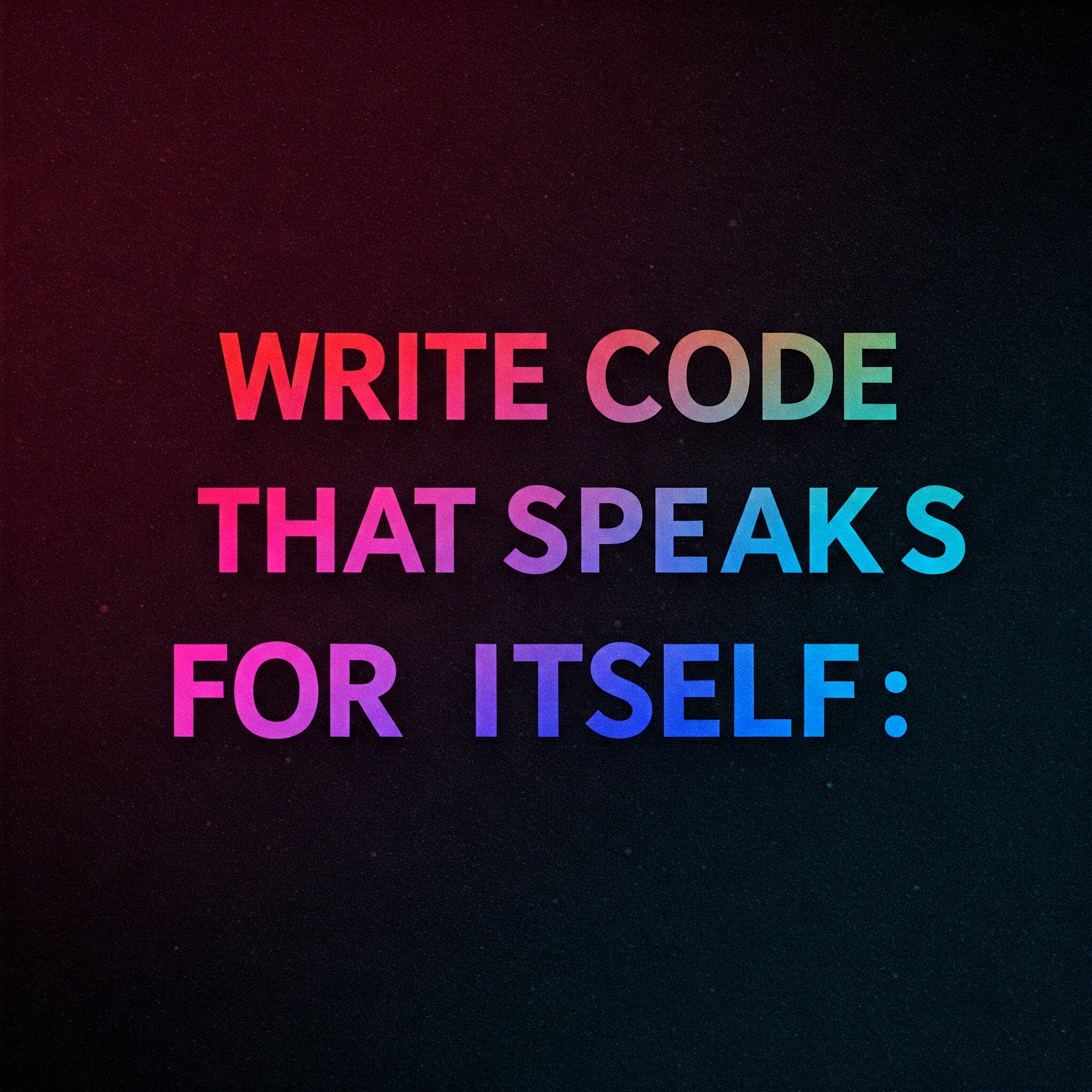
Avoid using poor naming conventions—this simple habit can significantly improve your coding experience. Unclear or inconsistent names make it much harder to understand a codebase, especially when joining a new team or revisiting a project after a long break. Using clear, descriptive names for variables, functions, and classes is essential for writing code that is both readable and maintainable.
Here are some common issues with naming conventions and tips to avoid them:
Unclear or Vague Names:
Ensure names are descriptive and convey the purpose of the variable, function, or class.
Example:
❌ x = 25 // What does 'x' represent? ❌ data = 25 // What does data refer? ✅ def maxLoginAttempts = 5 // Clearly indicates purpose
❌ def calc(x, y): // What is calc? return x * y // What are x and y ? ✅ def calculate_total_price(price, quantity): // Purpose is clear return price * quantity
Descriptive names help readers understand what’s happening without diving into external documentation or tracing logic line by line.
Misleading Names:
Avoid names that suggest a different functionality than what is actually performed.
Example:
❌ def download_pdf(): print("Uploading pdf") upload()
Wait… this function uploads pdf, not download!
✅ def upload_pdf(): print("Uploading pdf") upload()
Overuse of Generic Names:
Avoid overuse of generic names.
Example:
❌ def process(data): // What kind of data ? for item in data: generate(item) // What does generate(item) do? ✅ def processImage(images): for image in images: generatePdf(image)
Abbreviations That Obscure Meaning:
Avoid using abbreviations that are not universally understood.
Example:
❌ def chk_cfg(cfg): // Hard to understand if cfg["v"]: return True ❌ class Std {} // Hard to understand
Even if you wrote this, you might forget what "chk", "cfg", and "v" mean after a month 😀
✅ def is_config_valid(config): // Clearly conveys intent if config["version"]: return True ✅ class Student {} // Clearly conveys intent
Redundancy:
Avoid redundant words in names that do not add value or clarity.
Example:
❌ class Student: def __init__(self, student_name: str, student_address: str): // Unnecessary words. student.student.name is redundant self.student_name = student_name self.student_address = student_address ✅ class Student: def __init__(self, name: str, address: str): // Avoids unnecessary words. student.name is more clear self.name = name self.address = address
Subscribe to my newsletter
Read articles from Bikash Mainali directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
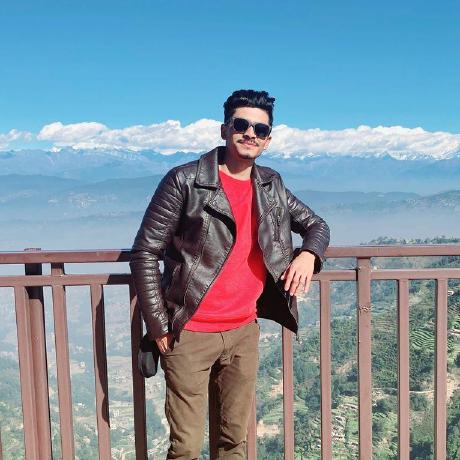
Bikash Mainali
Bikash Mainali
Experienced Java/JavaScript full-stack developer over 6 years of extensive expertise serving key role on elite technical teams developing enterprise software for healthcare, apple ad-platform, banking, and e-commerce. Adaptable problem-solver with high levels of skill in Groovy, Java, Spring, Spring Boot, Hibernate, JavaScript, TypeScript, Angular, Node, Express, React, MongoDB, IBM DB2, Oracle, PL/SQL, Docker, Kubernetes, CI/CD pipelines, AWS, Micro-service and Agile/Scrum. Strong technical skills paired with business-savvy UI design expertise. Personable team player with experience collaborating with diverse cross-functional teams.