Mastering Python’s Exception Handling: Overcoming the Challenges with Practical Experience

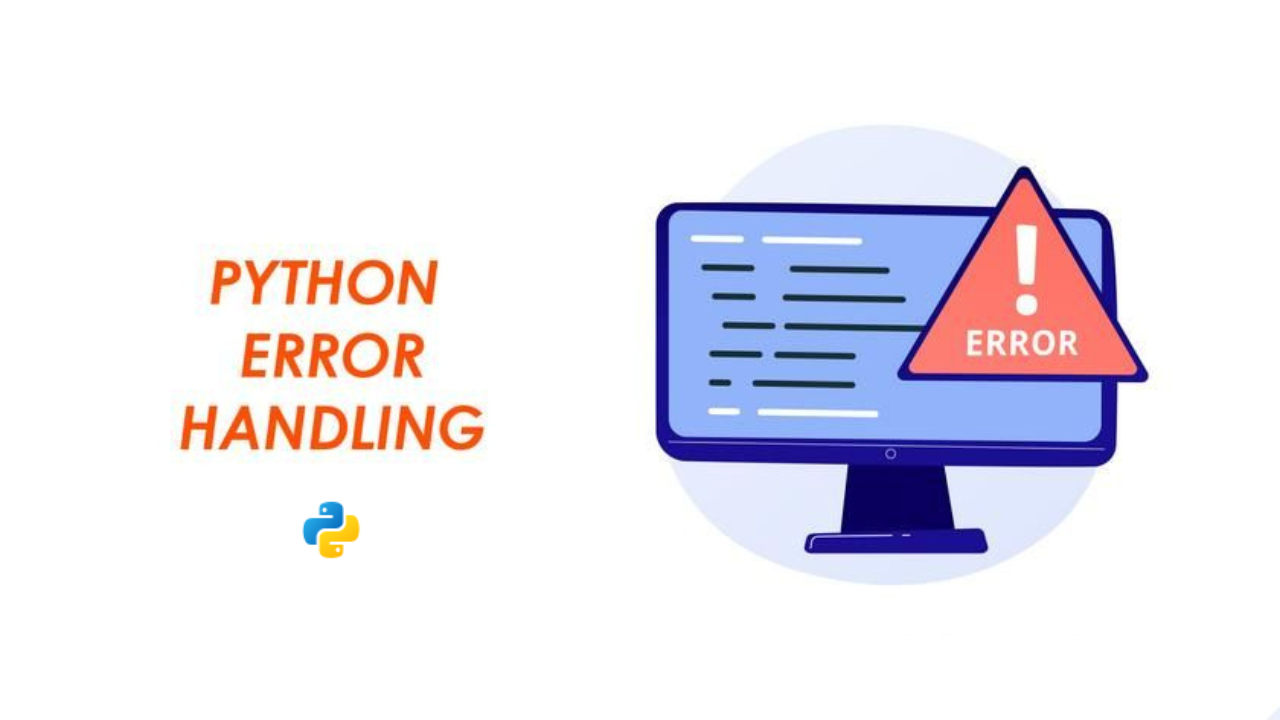
Introduction
Python is widely loved for its simplicity and readability, but one feature often leaves developers scratching their heads—exception-based error handling. While exceptions provide a structured way to manage errors, many developers find them tricky to grasp, especially in larger applications.
Effective error handling is essential for building resilient Python applications. In this article, I’ll break down the core concepts of Python’s exception handling and common challenges developers face, and share how I personally overcame these hurdles to improve my own workflow.
What is Exception Handling in Python?
In Python, exceptions are events that can modify the flow of control during the execution of a program. An exception is typically an error that occurs at runtime and can be caught and handled using try
, except
, else
, and finally
blocks.
Example Code:
try:
result = 10 / 0
except ZeroDivisionError as e:
print(f"Error: {e}")
Common Challenges with Python’s Exception Handling
1. Overuse of Try-Except Blocks
Problem: It’s tempting to wrap every line of code in a try-except block, leading to overly defensive coding and difficult-to-maintain code.
Personal Experience: Early in my Python journey, I would wrap entire functions in try-except, thinking it would protect me from all errors. However, this approach led to poor debugging experiences and made the code harder to read.
Solution: I learned to use exceptions selectively and only where they make sense—like when interacting with external systems, handling user input, or performing file I/O operations.
2. Not Handling Specific Exceptions
Problem: Catching all exceptions with a generic
except Exception:
is tempting, but it can hide the actual problem, making it difficult to trace errors.Personal Experience: When I first worked with APIs, I would catch all exceptions to prevent crashes, but this made it nearly impossible to debug issues related to bad responses.
Solution: I now make it a point to handle specific exceptions based on the task at hand. For example:
try:
response = requests.get('https://api.example.com')
response.raise_for_status()
except requests.exceptions.RequestException as e:
print(f"API Request Error: {e}")
3. Ignoring the Use of the finally
Block
Problem: A
finally
block is meant to execute code after atry
block, no matter what, whether an exception was raised or not. Many developers overlook it, which can lead to important cleanup tasks being skipped.Personal Experience: Early on, I didn’t fully appreciate the
finally
block, which led to unclosed files and unreleased resources in my applications.Solution: After learning about the significance of cleanup, I started using it
finally
to ensure that resources were always released.
try:
file = open('data.txt', 'r')
data = file.read()
except IOError as e:
print(f"File error: {e}")
finally:
file.close() # Ensure that the file is always closed
Best Practices for Effective Exception Handling
1. Be Specific with Exceptions
- Always catch the specific exception you expect. This makes the code easier to debug and more predictable.
2. Don’t Overuse Try-Except
- Use try-except blocks for expected, recoverable errors, not for controlling regular program flow.
3. Log Exceptions
- Use logging to capture details about the error, such as the type of exception and the stack trace, for easier debugging.
4. Use Custom Exceptions
- For complex applications, define custom exceptions to handle application-specific errors.
class CustomError(Exception):
pass
5. Clean up Resources with finally
- Always ensure that resources like files, network connections, and database connections are properly closed or released.
Personal Experience: How I Overcame These Challenges
When I first started working on larger Python projects, handling exceptions became a huge pain point. I often felt overwhelmed by the complexity and error-prone nature of managing many possible exceptions. However, as I gained more experience and worked on real-world applications, I realised that a methodical approach to exceptions was key.
Key lessons I learnt:
Start with the basics: Focus on understanding simple try-except blocks before moving on to more complex error-handling strategies.
Testing is vital. Running unit tests with edge cases helped me better understand which exceptions needed handling and how they interacted with the rest of the code.
Stay organised: Keeping track of which exceptions I was handling and reviewing logs regularly helped me spot patterns and reduce errors in the long run.
Conclusion: Mastering Python Exception Handling
Python’s exception-based error handling can be a powerful tool when used effectively. While it may feel daunting at first, with practice and the right strategies, you can make your Python applications more reliable and easier to maintain. Focus on specific, actionable error handling, don’t overuse try-except blocks, and always clean up your resources with finally
. Through trial and error, and with time, you'll master exception handling and feel more confident writing robust Python code.
Subscribe to my newsletter
Read articles from Binshad directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Binshad
Binshad
💻 Exploring the intersection of technology and finance. 📈 Sharing insights on tech dev, Ai,market trends, and innovation. 💡 Simplifying the complex world of investing