Why Async/Await Still Matters in Modern JavaScript

1 min read
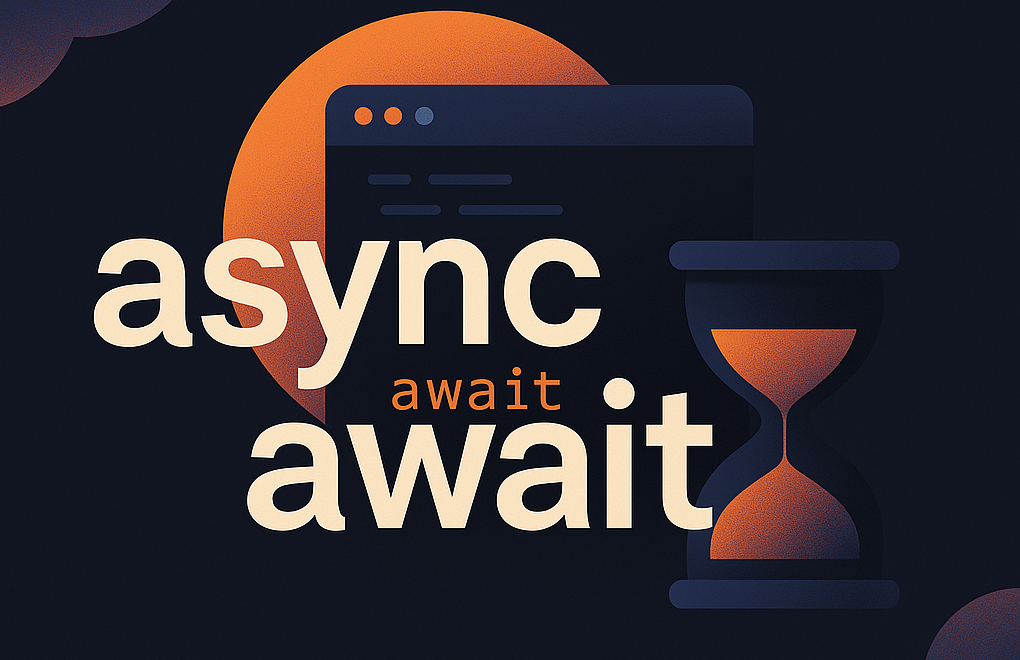
๐ This article has been moved to my official blog with improved formatting and bilingual layout.
๐ Read the updated version here:
https://site.softmaker.kz/en/js/mastering-async-await-elevate-your-javascript-skills.html
(๐ท๐บ Russian version is also available via the language switch at the top.)
0
Subscribe to my newsletter
Read articles from Viktor Sivulskiy directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Viktor Sivulskiy
Viktor Sivulskiy
I build tools, write code, and try not to touch things that already work. Mostly JavaScript, or something dangerously close to it.