Debouncing vs Throttling: Handling Expensive Function Calls in JavaScript
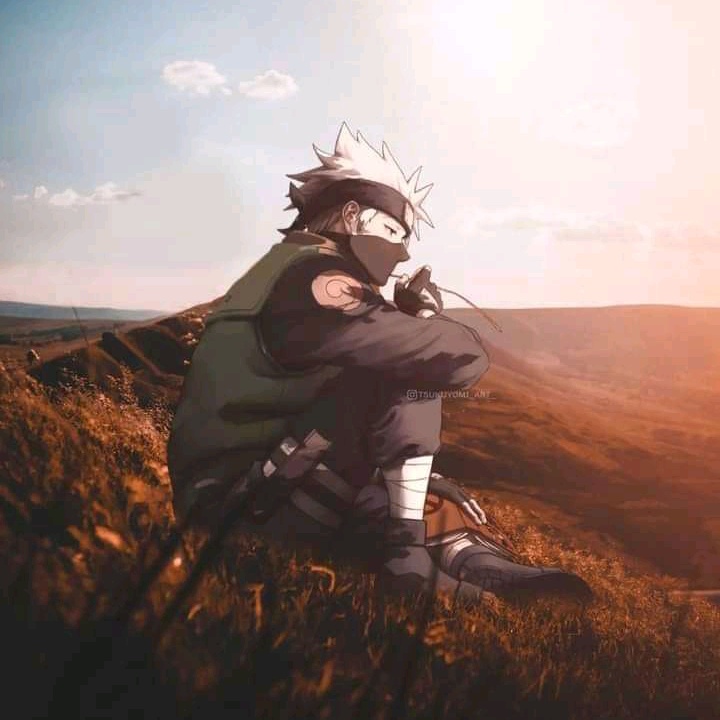
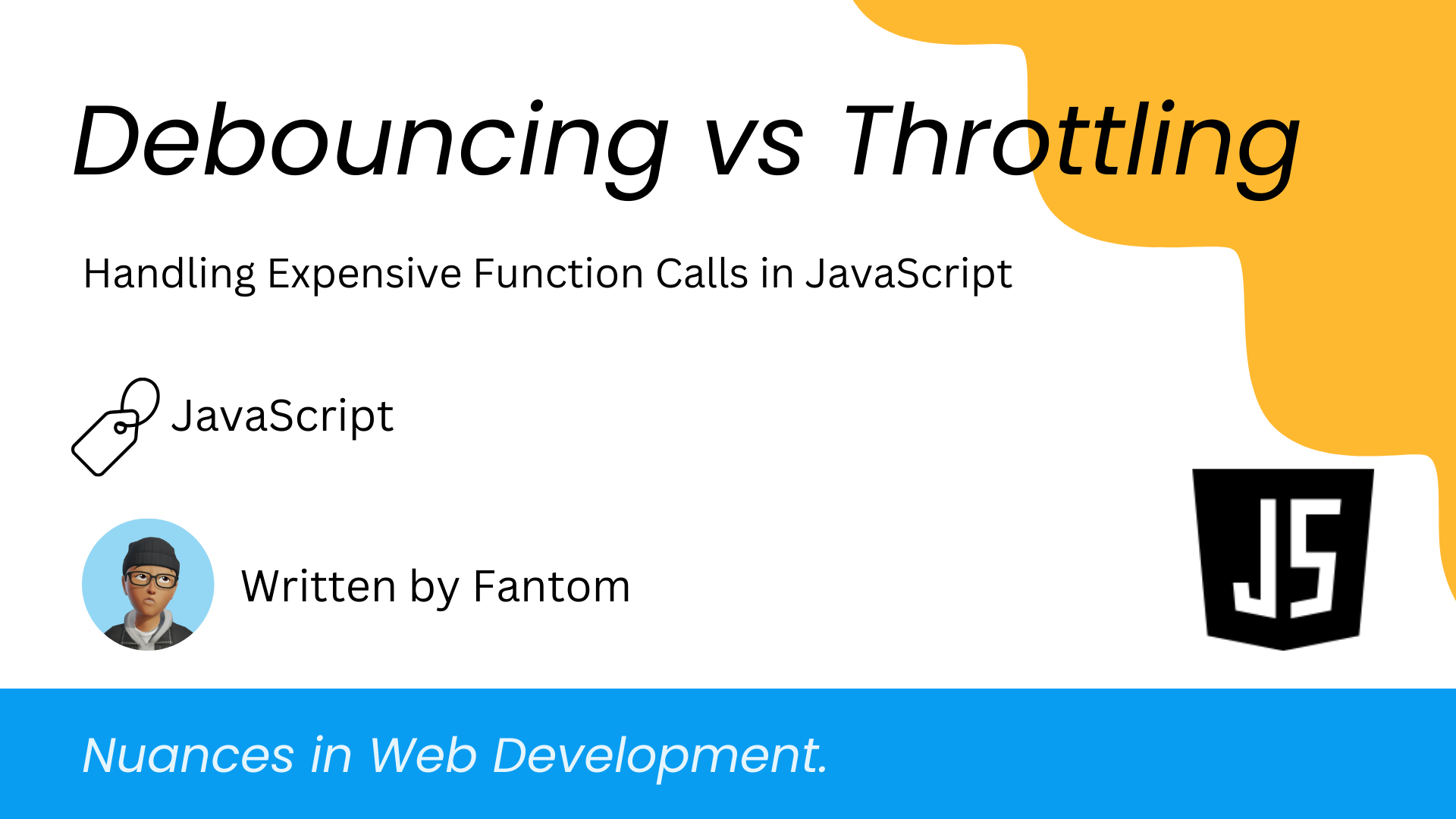
When building modern web applications, performance matters. Whether you're listening for scroll events, resizing windows, or handling real-time input, you’ll eventually run into a problem: your function is firing too often.
That’s where two powerful techniques come in: debouncing and throttling. They’re both designed to control how frequently a function is executed, but they behave very differently.
In this article, we’ll explore the nuanced differences between these two patterns and help you decide which one to use—and when.
1. The Problem: Frequent Function Execution
Imagine you attach a listener to a scroll
or keyup
event:
window.addEventListener("scroll", () => {
// Do something heavy
console.log("Scrolling...");
});
These events can fire dozens or even hundreds of times per second. Left unchecked, this can lead to:
Janky UI behavior
Laggy animations
Excessive API calls
Battery drain on mobile devices
This is where debounce and throttle come in to save the day.
2. Debouncing: Wait Until the User is Done
Debounce delays execution until a pause in activity.
With debouncing, the function will only run after the event stops firing for a certain period of time.
Real-life analogy:
Think of typing in a search bar. You don’t want to send a request on every keystroke—you want to wait until the user pauses.
Example Code:
function debounce(fn, delay) {
let timer; // Holds the current timeout ID
return function (...args) {
clearTimeout(timer); // Clear any previous timeout
timer = setTimeout(() => {
fn.apply(this, args); // Call the original function after the delay
}, delay);
};
}
// Usage
window.addEventListener(
"resize",
debounce(() => {
console.log("Window resized!");
}, 500)
);
Explanation:
debounce(fn, delay)
takes two arguments:fn
: the function you want to delaydelay
: how long to wait after the last call
let timer
stores the timeout ID, so we can cancel previous ones.The returned function does two things:
It cancels any previous timer with
clearTimeout(timer)
— this is the magic that resets the clock every time the event fires.It sets a new timer using
setTimeout
, sofn
will only run after the delay passes with no further calls.
In the example, when the user resizes the window, the function will only run once—500ms after the last resize event.
3. Throttling: Limit the Rate of Execution
Throttle ensures a function runs at most once every X milliseconds.
Rather than waiting for the event to stop, throttling allows execution at regular intervals, no matter how many times the event fires.
Real-life analogy:
Imagine a security guard letting only one person in every 2 seconds, even if there’s a crowd.
Example Code:
function throttle(fn, limit) {
let inThrottle = false; // Tracks whether the function is "in cooldown"
return function (...args) {
if (!inThrottle) {
fn.apply(this, args); // Run the function immediately
inThrottle = true; // Block further calls
setTimeout(() => {
inThrottle = false; // Allow function to run again after limit
}, limit);
}
};
}
// Usage
document.addEventListener(
"scroll",
throttle(() => {
console.log("Scroll event (throttled)");
}, 1000)
);
Explanation:
throttle(fn, limit)
also takes two arguments:fn
: the function to limitlimit
: the minimum time between each call (in ms)
let inThrottle
is a flag to determine if we're currently blocking calls.The returned function:
Checks if we’re not in the middle of a throttle (
!inThrottle
).If so, it executes
fn
immediately and setsinThrottle
totrue
.Then it starts a
setTimeout
forlimit
ms. When that finishes, it resets the flag so the function can run again.
In the example, no matter how many scroll events happen, the function only runs once per second.
4. Key Differences: Debounce vs Throttle
Feature | Debounce | Throttle |
When is it called? | After activity has stopped | At regular intervals |
Use case | Search inputs, auto-save | Scrolling, resizing, continuous events |
Delays the call? | Yes | No (just limits frequency) |
Rate of calls | One final call after the delay | Multiple calls, spaced out |
5. Choosing the Right One
Use Debounce when:
You want to wait for a pause in activity (e.g., typing, form input).
You want to avoid unnecessary calls (e.g., search, filtering, validations).
Use Throttle when:
You want regular updates, but not too often (e.g., scroll position, resize, mouse movement).
You need guaranteed updates at consistent intervals.
6. Combining Them
Sometimes, you might want both behaviors—like updating progress regularly but also ensuring one final update after a pause. In those cases, you can combine throttling and debouncing strategies or use advanced libraries like Lodash which provide prebuilt utilities:
import { debounce, throttle } from "lodash";
Conclusion
Debouncing and throttling are subtle tools with big performance impact. While they might seem interchangeable, their timing behavior makes them suitable for very different scenarios.
Understanding the nuance between them helps prevent bugs, improves UX, and optimizes performance—especially in JavaScript-heavy applications.
This is another installment in the Nuances in Web Development series—where we explore the fine differences that separate average code from great code. Subscribe to our newsletter and stay tuned for more deep dives!
Subscribe to my newsletter
Read articles from Peter Bamigboye directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
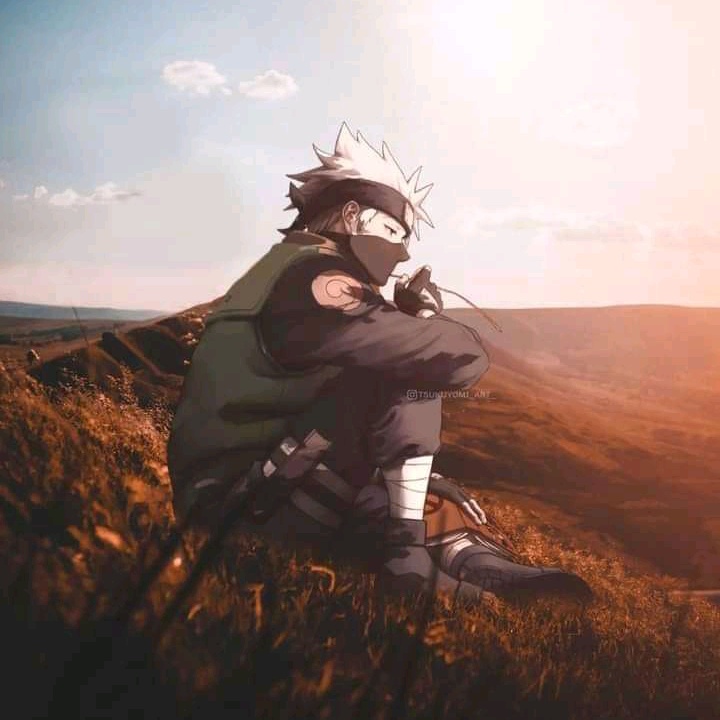
Peter Bamigboye
Peter Bamigboye
I am Peter, a front-end web developer who writes on current technologies that I'm learning or technologies that I feel the need to simplify.