Chapter 1: My Journey in Web Development - HTML Level 1 (Part A)
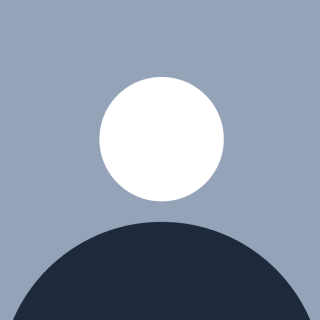
Table of contents
- Introduction to HTML
- What is HTML?
- History of HTML: From HTML 1.0 to HTML5
- The Purpose and Importance of HTML in Web Development
- Differences Between HTML, CSS, and JavaScript
- Why Learn HTML?
- Basic HTML Syntax Overview
- Conclusion
- 2. HTML Elements and Tags – A Comprehensive Guide
- What Are HTML Elements?
- Understanding Tags
- How Do We Display Information in HTML?
- HTML Tags: Building Blocks of Web Structure
- Conclusion
- 3. Hello World! (Running HTML Locally on Your System)
- The First HTML File
- Explanation of Each Line of Code
- Displaying 'Hello World' in the Browser
- Real-time Demonstration Using VS Code
- 1. Introduction to HTML Headings
- 2. Default Size of HTML Headings
- 3. Mixing Headings with Paragraphs (<p>)
- 4. Importance of Headings for Structure and SEO
- 5. Hierarchy and Accessibility
- 6. Examples and Practices
- Conclusion
- Section: 6. Practice Questions
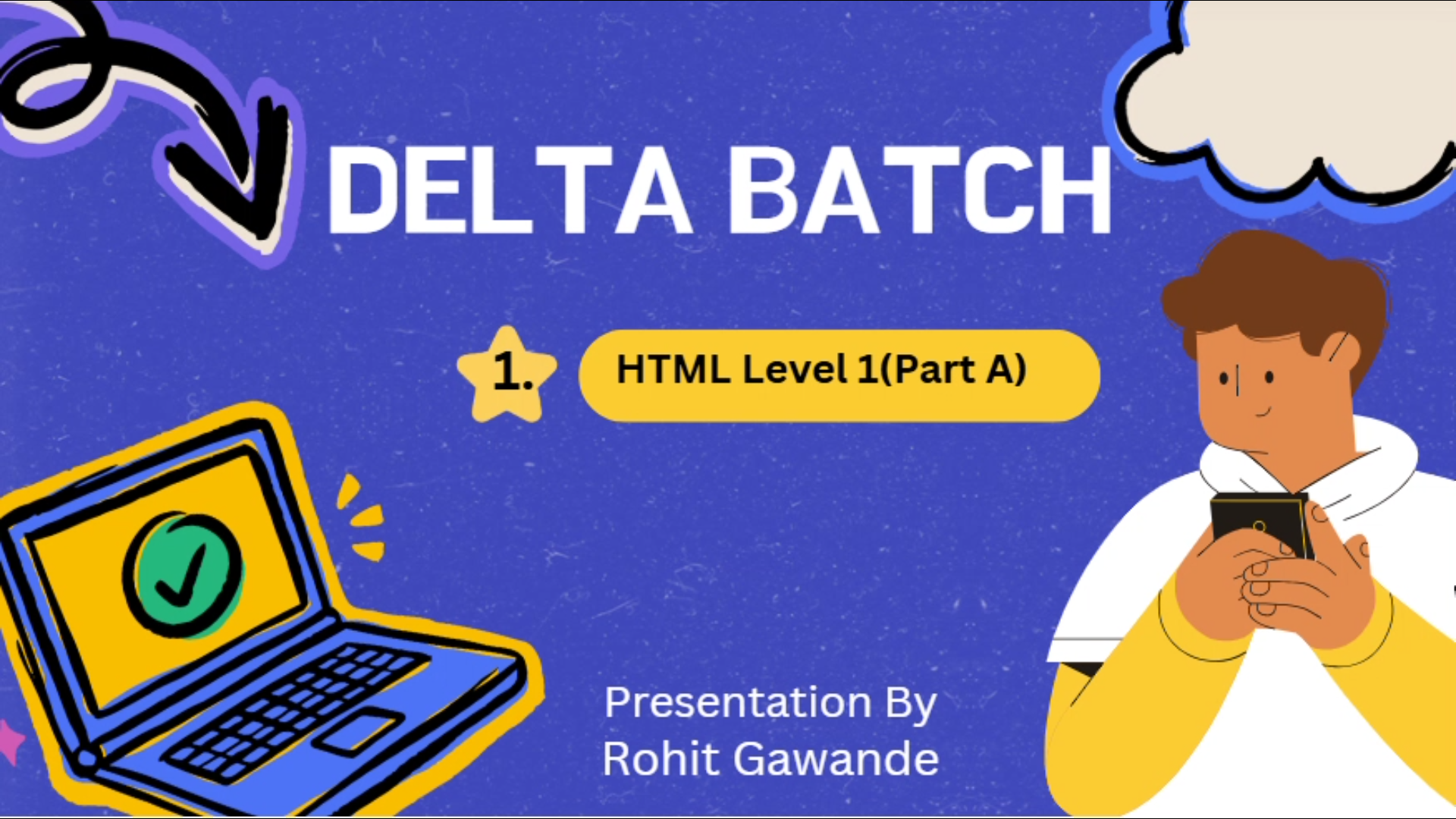
Welcome to the first chapter of my web development journey in the Delta Batch under the guidance of Shradha Khapra (Didi) from Apna College! This chapter marks the beginning of my exploration into the foundational technology of web development — HTML (HyperText Markup Language).
In this chapter, we'll dive into HTML Level 1, a part of the curriculum that introduces the building blocks of creating webpages. We'll understand the role of HTML in structuring the content of a website and learn how to use its basic elements and tags to create meaningful web pages.
Introduction to HTML
Welcome to the world of HTML (HyperText Markup Language)—the backbone of every webpage you see on the internet! 🌍✨
Before we dive into code, let’s start by understanding what HTML is, why it’s important, and how it forms the foundation of web development.
What is HTML?
HTML stands for HyperText Markup Language and is used to create the structure and content of web pages. Unlike programming languages like Java or Python, HTML is not a hardcore programming language—it is a markup language designed to format and organize content in a way that browsers can understand.
💡 Think of HTML as the skeleton of a webpage—it defines the structure, but it doesn’t add styling (CSS) or interactivity (JavaScript).
Key Characteristics of HTML:
✔️ It uses tags and elements to define content.
✔️ It is interpreted by web browsers (like Chrome, Firefox, Edge).
✔️ It works alongside CSS (for styling) and JavaScript (for functionality).
✔️ It follows a hierarchical structure, meaning elements are nested inside one another.
History of HTML: From HTML 1.0 to HTML5
HTML has evolved significantly since its first version in 1991. Let’s take a quick look at its journey:
📌 HTML 1.0 (1991) – The first version, very basic and limited.
📌 HTML 2.0 (1995) – Introduced forms and tables.
📌 HTML 3.2 (1997) – Brought more design elements like font styling.
📌 HTML 4.01 (1999) – Added better structure and scripting support.
📌 XHTML (2000s) – A stricter, XML-based version of HTML.
📌 HTML5 (2014 - Present) – The latest version, introducing semantic elements, multimedia support, and better accessibility.
🚀 HTML5 is what we’ll be focusing on in this series!
The Purpose and Importance of HTML in Web Development
HTML is the foundation of the web—without it, websites wouldn’t exist! Every webpage you visit is built using HTML in some form.
📌 Why is HTML important?
✅ It structures web pages for browsers.
✅ It works with CSS & JavaScript to create interactive websites.
✅ It ensures accessibility for all users, including screen readers.
✅ It allows search engines to understand webpage content (SEO).
Imagine a webpage without HTML—it would just be plain text with no structure!
For example, look at this text representation:
Before HTML:
The 3 primary states of matter are -Solid, Liquid, and Gas. Solid - these include materials like Iron (Fe). Liquid - these include materials like Water (H₂O). Gas - these include materials like Oxygen (O₂).
After HTML (Structured Content):
<h2>The 3 Primary States of Matter</h2>
<ul>
<li><strong>Solid</strong> – Includes materials like Iron (Fe).</li>
<li><strong>Liquid</strong> – Includes materials like Water (H₂O).</li>
<li><strong>Gas</strong> – Includes materials like Oxygen (O₂).</li>
</ul>
🎯 The difference? Better readability, structure, and clarity!
Differences Between HTML, CSS, and JavaScript
Many beginners confuse HTML, CSS, and JavaScript because they work together. Here’s a simple breakdown:
Technology | Purpose | Example |
HTML | Defines structure and content | <h1>Hello World!</h1> |
CSS | Adds styling and design | color: red; font-size: 20px; |
JavaScript | Adds interactivity and functionality | alert("Hello!"); |
💡 Think of a website like a car:
🔹 HTML = The frame & body (structure).
🔹 CSS = The paint & design (styling).
🔹 JavaScript = The engine & controls (functionality).
🚀 In this series, we’ll first master HTML before diving into CSS and JavaScript!
Why Learn HTML?
If you want to build websites, learning HTML is the first step! Even if you’re not a developer, basic HTML knowledge can help in many fields like blogging, digital marketing, and email design.
HTML is Useful For:
✅ Web Development – Every webpage needs HTML!
✅ Blogs & Content Writing – Formatting articles properly.
✅ Email Templates – Designing professional emails.
✅ SEO Optimization – Structuring content for search engines.
Fun fact: Even major websites like Facebook, Google, and YouTube are built on HTML, CSS, and JavaScript!
Basic HTML Syntax Overview
Before we start coding, let’s understand the basic syntax of HTML:
1️⃣ HTML is made up of elements that have opening and closing tags:
<p>This is a paragraph.</p>
2️⃣ Some elements don’t need closing tags (self-closing elements):
<br> <!-- Creates a line break -->
3️⃣ Elements can have attributes that provide extra information:
<a href="https://example.com">Click here</a>
🔹 The href
attribute defines the link’s destination.
Case Sensitivity & Coding Standards
✅ HTML is not case-sensitive, but best practice is to write in lowercase:
<title>Correct</title>
<TITLE>Incorrect</TITLE>
✅ Use proper indentation for readability.
✅ Always close tags unless they are self-closing.
Conclusion
✅ What HTML is and why it’s important.
✅ The history of HTML and why HTML5 is the latest standard.
✅ The role of HTML in web development.
✅ How HTML differs from CSS and JavaScript.
✅ Why learning HTML is a crucial skill.
✅ The basics of HTML syntax and structure.
2. HTML Elements and Tags – A Comprehensive Guide
In HTML, every piece of content displayed on a webpage is enclosed within elements and tags. These are fundamental building blocks that define the structure and presentation of web pages. Understanding how elements and tags work is crucial for developing well-structured, accessible, and visually appealing web pages.
What Are HTML Elements?
An HTML element consists of an opening tag, content, and a closing tag (except for self-closing elements). It represents a piece of information or a component on a webpage.
Definition of HTML Elements
An HTML element is a complete unit of code that defines the structure and semantics of a webpage. Elements can contain:
Text content – like paragraphs and headings.
Other elements – known as nested elements.
Attributes – additional information about an element.
Example of an HTML Element
<p>This is a paragraph.</p>
<p>
– Opening tagThis is a paragraph.
– Content</p>
– Closing tagComplete unit (
<p>Content</p>
) = an HTML element
Nested HTML Elements
An HTML element can contain other elements within it. This is called nesting.
Example of Nested Elements
<div>
<h1>Welcome to My Website</h1>
<p>This is a simple paragraph inside a div.</p>
</div>
<div>
is a container element.<h1>
and<p>
are nested inside<div>
.
This nesting structure helps in organizing content systematically on a webpage.
Understanding Tags
What Are HTML Tags?
HTML tags are keywords enclosed in angle brackets (<>
). They define the start and end of an element. Tags tell the browser how to render the content.
Types of HTML Tags
Opening Tags
Opening tags start an element.
Example:
<p>
opens a paragraph element.
Closing Tags
Closing tags end an element.
Example:
</p>
closes the paragraph element.
Self-Closing Tags (Void Elements)
Some elements don’t need a closing tag because they don’t contain any content.
Example:
<br>
(line break),<img>
(image),<hr>
(horizontal rule).
Example of Different Tags
<!DOCTYPE html>
<html>
<head>
<title>My First HTML Page</title>
</head>
<body>
<h1>This is a Heading</h1>
<p>This is a paragraph.</p>
<img src="image.jpg" alt="A sample image">
<br>
</body>
</html>
Key Differences Between Tags and Elements
Feature | HTML Tag | HTML Element |
Definition | A tag defines an element's beginning and end. | An element includes the tag and content. |
Example | <h1> (opening tag), </h1> (closing tag) | <h1>Hello World</h1> |
Self-Closing? | Some tags are self-closing (like <br> , <img> ). | Every element follows a structure (opening tag, content, closing tag). |
How Do We Display Information in HTML?
To display content in HTML, we use standard elements that the browser recognizes. The most commonly used elements include:
1. Paragraphs (<p>
)
The <p>
tag is used to define paragraphs in a webpage.
Example:
<p>This is a paragraph of text.</p>
2. Headings (<h1>
to <h6>
)
Headings define the importance of text, from <h1>
(largest) to <h6>
(smallest).
Example:
<h1>Main Title</h1>
<h2>Subheading</h2>
<h3>Another Subheading</h3>
HTML Tags: Building Blocks of Web Structure
HTML tags serve as containers for content or other elements, defining the webpage’s layout and design.
Structure of an HTML Element
<tagname>Content</tagname>
Opening tag –
<p>
Content –
"This is a paragraph."
Closing tag –
</p>
Example: A Complete HTML Structure
<!DOCTYPE html>
<html>
<head>
<title>Example Page</title>
</head>
<body>
<h1>Welcome to HTML</h1>
<p>This is a paragraph explaining HTML basics.</p>
</body>
</html>
<html>
– Root element.<head>
– Contains metadata.<title>
– Sets the page title.<body>
– Contains visible content.
Conclusion
HTML Elements consist of opening tags, content, and closing tags.
Tags define elements and tell the browser how to display content.
Common HTML Elements like
<p>
,<h1>
, and<img>
are used to create web pages.Nesting Elements helps structure the webpage effectively.
3. Hello World! (Running HTML Locally on Your System)
When you write and save an HTML file on your local system, it can be opened and displayed in a web browser. However, since it is stored locally, it does not require an internet connection or a web server. This section will guide you through creating your first HTML file, explaining the code, and understanding how browsers render HTML content.
The First HTML File
The first step in web development is to create a simple HTML file and display content in a browser. We’ll start with a basic "Hello World" example.
Creating Your First HTML File
Follow these steps to create your first HTML file:
Open a text editor (e.g., Notepad, VS Code, Sublime Text).
Write the HTML code (explained below).
Save the file with a
.html
extension (e.g.,hello.html
).Open it in a browser (Chrome, Firefox, Edge, etc.).
Writing the Code:
Create a new file in your text editor and add the following code:
<!DOCTYPE html>
<html>
<head>
<title>My First HTML Page</title>
</head>
<body>
<p>Hello World</p>
<p>Rohit Gawande</p>
</body>
</html>
Explanation of Each Line of Code
Code | Explanation |
<!DOCTYPE html> | Declares the document as an HTML5 file. |
<html> | The root element that wraps all content on the page. |
<head> | Contains metadata, title, and external links. |
<title>My First HTML Page</title> | Sets the title of the webpage (visible in the browser tab). |
<body> | Contains all visible content displayed in the browser. |
<p>Hello World</p> | Displays "Hello World" as a paragraph. |
<p>Rohit Gawande</p> | Displays "Rohit Gawande" as another paragraph. |
Displaying 'Hello World' in the Browser
Once you’ve saved your hello.html
file, follow these steps to open it in your browser:
Steps to Open an HTML File in a Browser
Locate the file: Go to the folder where you saved
hello.html
.Right-click on the file: Select "Open with" → Choose a browser (Chrome, Firefox, Edge).
View the output: The browser will render the HTML content.
Expected Output in the Browser
After opening the file, you should see the following displayed in your browser:
The text will be left-aligned and displayed in separate lines, as each <p>
tag creates a new paragraph.
Real-time Demonstration Using VS Code
Using Visual Studio Code (VS Code) for HTML
VS Code is a popular text editor for writing HTML, CSS, and JavaScript. Here's how to use it:
Open VS Code
Create a new file: Press
Ctrl + N
.Save the file: Press
Ctrl + S
, name ithello.html
, and select "All Files" as the format.Write your HTML code (as shown above).
Run the file:
Right-click inside VS Code and select "Open with Live Server" (if you have the extension installed).
OR manually open the file in a browser by navigating to its location.
4. The Paragraph (<p>
) Element
What is the <p>
Element?
The <p>
element in HTML represents a paragraph of text. It is one of the most fundamental elements used to structure content on a webpage. The browser automatically adds space (margin) before and after a paragraph to separate it from other elements.
Key Characteristics:
Used to display blocks of text.
Automatically adds line breaks and spacing between paragraphs.
Ignores extra spaces within the text.
Can contain inline elements such as
<strong>
,<em>
,<a>
, and<br>
.
Example:
<p>This is my first paragraph.</p>
<p>This is my second paragraph.</p>
🔹 Output:
This is my first paragraph.
This is my second paragraph.
👉 Note: Browsers insert a space (margin) between paragraphs by default.
Definition and Purpose of the Paragraph Tag
The <p>
tag is used to define a block of text within an HTML document. It helps in organizing text content, making it more readable.
Common Use Cases:
Writing articles, blogs, and documentation.
Structuring content on webpages.
Creating text blocks in forms and sections.
Example - A Simple Webpage Content Layout
<h1>About HTML</h1>
<p>HTML stands for HyperText Markup Language.</p>
<p>It is the foundation of web development and is used to structure webpages.</p>
🔹 Output:
About HTML
HTML stands for HyperText Markup Language.
It is the foundation of web development and is used to structure webpages.
Adding Text Content Within <p>
The <p>
element wraps text to make it a paragraph. However, you can also include inline elements like links, bold text, and italic text inside it.
Example - Adding Inline Elements
<p>this is a website</p>
<p><strong>Note:</strong> HTML is not a programming language.</p>
<p><em>Italic text</em> can be used for emphasis.</p>
🔹 Output:
this is a website
Note: HTML is not a programming language.
Italic text can be used for emphasis.
Nesting: Using Nested Tags Inside <p>
A nested tag is an HTML tag inside another tag. Some elements can be nested inside <p>
, while others cannot.
✅ Allowed Inside <p>
:
<strong>
(Bold text)<em>
(Italic text)<a>
(Links)<span>
(Inline styling)
❌ Not Allowed Inside <p>
(Block Elements):
<div>
<h1>
,<h2>
, etc.<section>
Example - Proper Nested Tags
<p>This is <strong>bold text</strong> inside a paragraph.</p>
<p>This is an <em>italic</em> word.</p>
Incorrect Nesting (Not Allowed):
<p>This is a paragraph.</p>
<div>This is a div inside a paragraph.</div> <!-- Incorrect -->
🔴 Error: The <div>
element cannot be directly inside a <p>
.
Line Breaks and Spacing
By default, HTML ignores multiple spaces and new lines. If you need to force a new line, use the <br>
tag.
Using <br>
for Line Breaks
The <br>
tag is a self-closing tag (<br>
) that forces a line break without starting a new paragraph.
Example - Using <br>
Inside a Paragraph
<p>This is the first line.<br>This is the second line.</p>
🔹 Output:
This is the first line.
This is the second line.
Avoiding Multiple Spaces in HTML
HTML automatically ignores multiple spaces and extra new lines.
Example - Extra Spaces are Ignored
<p>This is a paragraph.</p>
🔹 Output:
This is a paragraph. (Extra spaces are removed)
✅ How to Preserve Spaces?
Use the
(Non-Breaking Space) character to add extra spaces.
Example - Using
for Extra Spaces
<p>This is extra spaced text.</p>
🔹 Output:
This is extra spaced text.
Summary of <p>
Element
✅ <p>
is used for paragraphs and separates text content.
✅ Browsers automatically add space before and after <p>
.
✅ The <br>
tag is used to insert line breaks inside a paragraph.
✅ Multiple spaces are ignored unless
is used.
Understanding HTML Heading Elements (<h1>
to <h6>
)
1. Introduction to HTML Headings
In HTML, headings are used to define the structure of a webpage. They range from <h1>
(the most important) to <h6>
(the least important).
Hierarchy of Headings
<h1>
: Used for the main title or most important heading on a webpage.<h2>
: Represents major sections under<h1>
.<h3>
: Used for subsections under<h2>
.<h4>
,<h5>
,<h6>
: Represent further subdivisions within sections.
Example structure:
<h1>Website Title</h1>
<h2>About Us</h2>
<h3>Our Team</h3>
<h4>John Doe - CEO</h4>
<h5>Experience</h5>
<h6>Certifications</h6>
This hierarchy helps browsers, search engines, and screen readers understand the content’s organization.
2. Default Size of HTML Headings
Each heading has a default font size set by the browser. Below is the default styling:
Tag | Default Font Size (px) | Default Style |
<h1> | 32px | Bold |
<h2> | 24px | Bold |
<h3> | 18.72px | Bold |
<h4> | 16px | Bold |
<h5> | 13.28px | Bold |
<h6> | 10.72px | Bold |
3. Mixing Headings with Paragraphs (<p>
)
Headings are often used alongside paragraph (<p>
) elements to provide context. Below is an example of a structured content layout:
<h1>Rohit Gawande</h1>
<p>Is a BTech Final Year CSE student.</p>
<h3>Education</h3>
<p>Studying Computer Science Engineering at XYZ University.</p>
<h3>Skills</h3>
<p>Core Java, JDBC, Servlets, HTML, CSS, JavaScript.</p>
How It Helps
This structure makes the content visually appealing.
Helps in better readability and SEO.
Maintains a clear and logical flow of information.
4. Importance of Headings for Structure and SEO
Why Use Proper Headings?
Enhances Readability: Organizes content into sections for better user experience.
Improves SEO: Search engines use headings to understand page content.
Accessibility: Screen readers use headings to help visually impaired users navigate content.
For example, in SEO:
<h1>
should be unique and describe the main topic.<h2>
and<h3>
should introduce subtopics and keywords.
Bad Example (SEO-Unfriendly)
<h1>Services</h1>
<h1>Contact Us</h1> <!-- Multiple <h1> tags reduce SEO effectiveness -->
Good Example (SEO-Friendly)
<h1>Our Services</h1>
<h2>Web Development</h2>
<h2>SEO Optimization</h2>
<h2>Graphic Design</h2>
This structure makes it clear what the page is about.
5. Hierarchy and Accessibility
Using headings properly improves accessibility. Here are some best practices:
Creating a Proper Heading Structure
Use one
<h1>
per page (main topic).Follow the hierarchy: Do not skip levels (e.g.,
<h1>
→<h3>
without an<h2>
).Use headings only for structuring content, not for styling.
Example of a Well-Structured Page:
<h1>Web Development Course</h1>
<h2>Introduction to HTML</h2>
<h3>HTML Tags and Elements</h3>
<h3>Practice Exercises</h3>
<h2>Introduction to CSS</h2>
<h3>Selectors and Properties</h3>
Semantic HTML Tips
Use
<h1>
for page titles.Use
<h2>
for main sections.Use
<h3>
for subsections.Avoid skipping heading levels (e.g.,
<h1>
→<h3>
without<h2>
).Do not use headings just for bold text—use CSS instead.
6. Examples and Practices
Writing Headings for a Sample Webpage
Below is an example of a personal portfolio webpage:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Rohit Gawande - Portfolio</title>
</head>
<body>
<h1>Rohit Gawande</h1>
<p>BTech Final Year Computer Science and Engineering Student</p>
<h2>Education</h2>
<p>Priyadarshini College of Engineering , Bachelor of Technology in Computer Science and Engineering </p>
<h2>Skills</h2>
<h3>Programming Languages</h3>
<p>Java, Python, JavaScript</p>
<h3>Web Development</h3>
<p>HTML, CSS, JavaScript, React</p>
<h3>Databases</h3>
<p>MySQL, MongoDB</p>
<h2>Projects</h2>
<h3>Library Management System</h3>
<p>A Java-based system to manage book lending and records.</p>
<h3>Portfolio Website</h3>
<p>Personal website showcasing my projects and skills.</p>
</body>
</html>
Conclusion
HTML headings
<h1>
to<h6>
define content hierarchy.Proper structure improves SEO, readability, and accessibility.
Avoid using multiple
<h1>
tags for better ranking.Use headings only for structuring, not just for making text bold.
Section: 6. Practice Questions
Introduction
Practice is essential when learning HTML. This section provides exercises ranging from basic to advanced levels to reinforce your understanding of HTML structure, tags, and elements.
Practice Question 1: Avengers Introduction
Code Implementation
Below is an HTML file that introduces three Avengers—Iron Man, Captain America, and Hulk—along with their real names.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Practice Qs 1</title>
</head>
<body>
<h1>Avengers</h1>
<h2>Iron Man</h2>
<p>Also goes by the name <b>Tony Stark</b></p>
<h2>Captain America</h2>
<p>Also goes by the name <b>Steve Rogers</b></p>
<h2>Hulk</h2>
<p>Also goes by the name <b>Bruce Banner</b></p>
</body>
</html>
Explanation of the Code
Explanation of Tags Used
<h1>
(Main Heading):- Displays the title "Avengers" as the primary heading.
<h2>
(Subheadings):- Each Avenger's name is enclosed in
<h2>
, making them subheadings.
- Each Avenger's name is enclosed in
<p>
(Paragraphs):- Provides additional information about each Avenger.
<b>
(Bold Text):- Used to highlight the real names of the Avengers.
Expected Output
When the above HTML file is run in a browser, it displays:
Conclusion
This practice question provides a foundation for working with headings, paragraphs, bold text, and HTML structure.
7. Boilerplate Code
The HTML boilerplate is the standard structure of an HTML document. It includes essential tags to set up a webpage:
<!DOCTYPE html>
<html>
<head>
<title>Webpage Title</title>
</head>
<body>
<!-- Content goes here -->
</body>
</html>
<!DOCTYPE html>
: Declares the document type (HTML5 in this case).<html>
: The root element that contains all HTML content.<head>
: Contains meta-information about the document (e.g., title, character set).<title>
: Sets the title displayed in the browser tab.<body>
: Contains all visible content of the webpage.
By the end of this chapter, you will have a solid understanding of HTML basics and the confidence to create your own webpages!
Other Series:
Connect with Me
Stay updated with my latest posts and projects by following me on social media:
LinkedIn: Connect with me for professional updates and insights.
GitHub: Explore my repository and contributions to various projects.
LeetCode: Check out my coding practice and challenges.
Your feedback and engagement are invaluable. Feel free to reach out with questions, comments, or suggestions. Happy coding!
Rohit Gawande
Full Stack Java Developer | Blogger | Coding Enthusiast
Subscribe to my newsletter
Read articles from Rohit Gawande directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
Rohit Gawande
Rohit Gawande
🚀 Tech Enthusiast | Full Stack Developer | System Design Explorer 💻 Passionate About Building Scalable Solutions and Sharing Knowledge Hi, I’m Rohit Gawande! 👋I am a Full Stack Java Developer with a deep interest in System Design, Data Structures & Algorithms, and building modern web applications. My goal is to empower developers with practical knowledge, best practices, and insights from real-world experiences. What I’m Currently Doing 🔹 Writing an in-depth System Design Series to help developers master complex design concepts.🔹 Sharing insights and projects from my journey in Full Stack Java Development, DSA in Java (Alpha Plus Course), and Full Stack Web Development.🔹 Exploring advanced Java concepts and modern web technologies. What You Can Expect Here ✨ Detailed technical blogs with examples, diagrams, and real-world use cases.✨ Practical guides on Java, System Design, and Full Stack Development.✨ Community-driven discussions to learn and grow together. Let’s Connect! 🌐 GitHub – Explore my projects and contributions.💼 LinkedIn – Connect for opportunities and collaborations.🏆 LeetCode – Check out my problem-solving journey. 💡 "Learning is a journey, not a destination. Let’s grow together!" Feel free to customize or add more based on your preferences! 😊