🚀 Building a Flutter App for Smartwatches: A Quick Start Guide
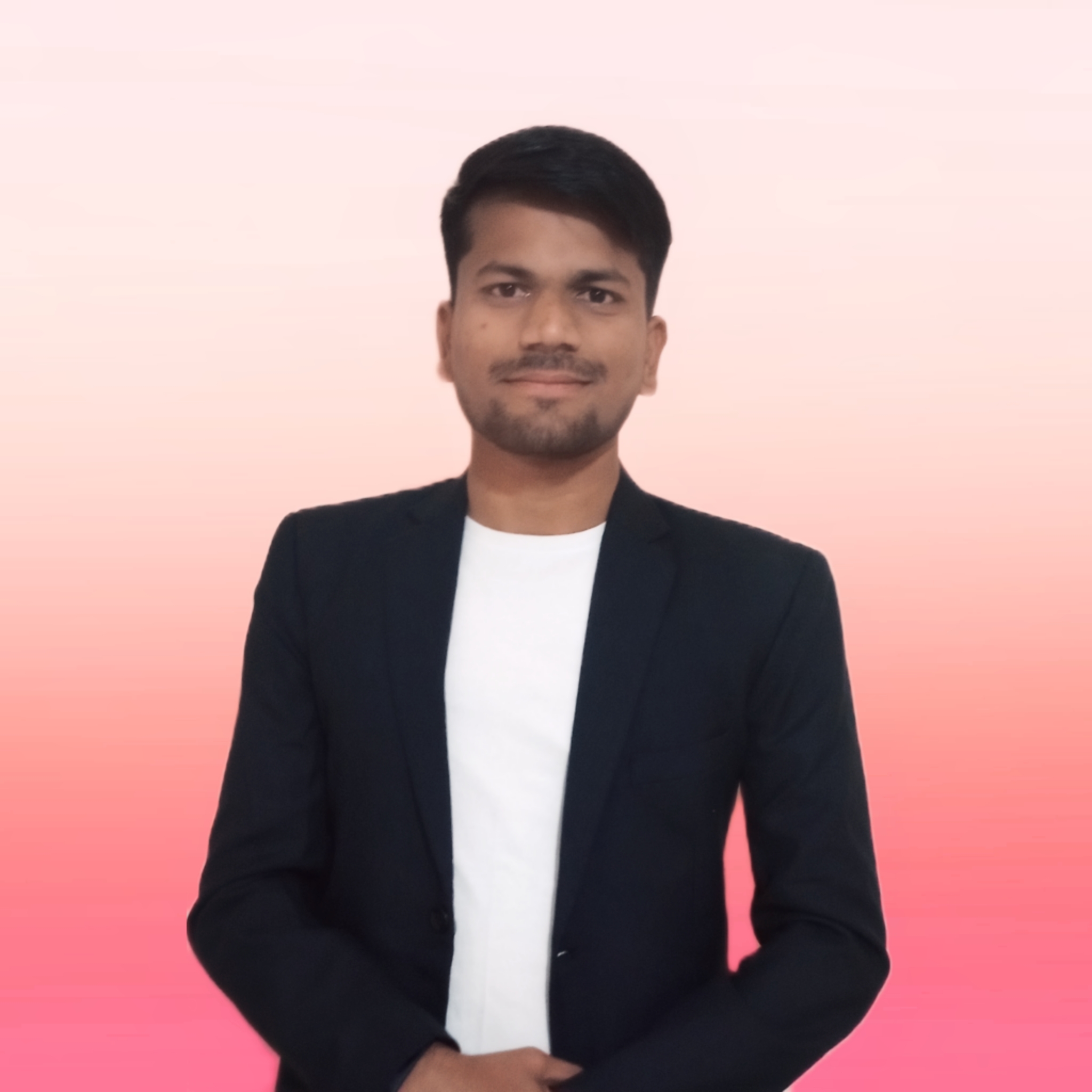

Flutter has made app development fast, beautiful, and cross-platform. While it's commonly used for mobile, web, and desktop, developers are now exploring wearables like smartwatches. In this blog, we’ll dive into how to build a Flutter app for smartwatches and what you need to keep in mind while designing for the small screen.
🕒 Why Flutter for Smartwatches?
Flutter is primarily optimized for mobile and desktop, but with a little creativity and some third-party support, you can make it work on smartwatches too.
Here’s why Flutter works:
Single Codebase for Android phones and Wear OS watches.
Custom UI: Flutter’s widget system allows you to build highly customized and animated UIs that can fit a tiny screen.
Growing Ecosystem: Packages and community support are expanding into wearables.
🛠Requirements
To build a Flutter smartwatch app, you’ll need:
Flutter SDK (latest version)
Android Studio or VS Code
A Wear OS emulator or real Wear OS device
Basic knowledge of Flutter widgets and layouts
Note: Flutter does not natively support Apple Watch (watchOS) at this time.
📱 Designing for a Watch Screen
Smartwatches have a much smaller display, usually round or square. You must keep the UI simple and minimal.
Key Tips:
Use large buttons for easy tapping.
Prioritize essential information—no clutter.
Use dark themes to save battery and reduce glare.
Round UI? Use
ClipOval
or custom painters to simulate circular designs.
🔧 Creating a Basic Watch UI in Flutter
Here’s a basic example of a circular watch UI with time display and a button:
dartCopyEditimport 'package:flutter/material.dart';
import 'dart:async';
void main() {
runApp(WatchApp());
}
class WatchApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
theme: ThemeData.dark(),
home: WatchHome(),
);
}
}
class WatchHome extends StatefulWidget {
@override
_WatchHomeState createState() => _WatchHomeState();
}
class _WatchHomeState extends State<WatchHome> {
String _time = '';
@override
void initState() {
super.initState();
_updateTime();
}
void _updateTime() {
Timer.periodic(Duration(seconds: 1), (timer) {
final now = DateTime.now();
setState(() {
_time = "${now.hour}:${now.minute}:${now.second}";
});
});
}
@override
Widget build(BuildContext context) {
return Scaffold(
body: Center(
child: ClipOval(
child: Container(
width: 250,
height: 250,
color: Colors.black,
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: [
Text(
_time,
style: TextStyle(fontSize: 24, fontWeight: FontWeight.bold),
),
SizedBox(height: 20),
ElevatedButton(
onPressed: () {
// Add action here
},
child: Text("Tap Me"),
)
],
),
),
),
),
);
}
}
📦 Useful Packages
wear
: Detects if app is running on a Wear OS device.flutter_compass
: For compass features.pedometer
: Step counter support.
🔮 Future Scope
Flutter’s support for wearables is still growing. Google’s Wear OS is the most practical platform currently. In the future, we may see better support for Apple Watch or even custom wearable hardware.
🧠Final Thoughts
Creating a smartwatch app with Flutter is totally doable, especially for Wear OS. While not officially specialized for wearables yet, Flutter’s flexibility lets you create powerful, beautiful, and responsive mini-apps for the wrist.
If you’re already building Flutter apps, stepping into wearables is just a few lines of code away!
Subscribe to my newsletter
Read articles from Manoj Jaiswal directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
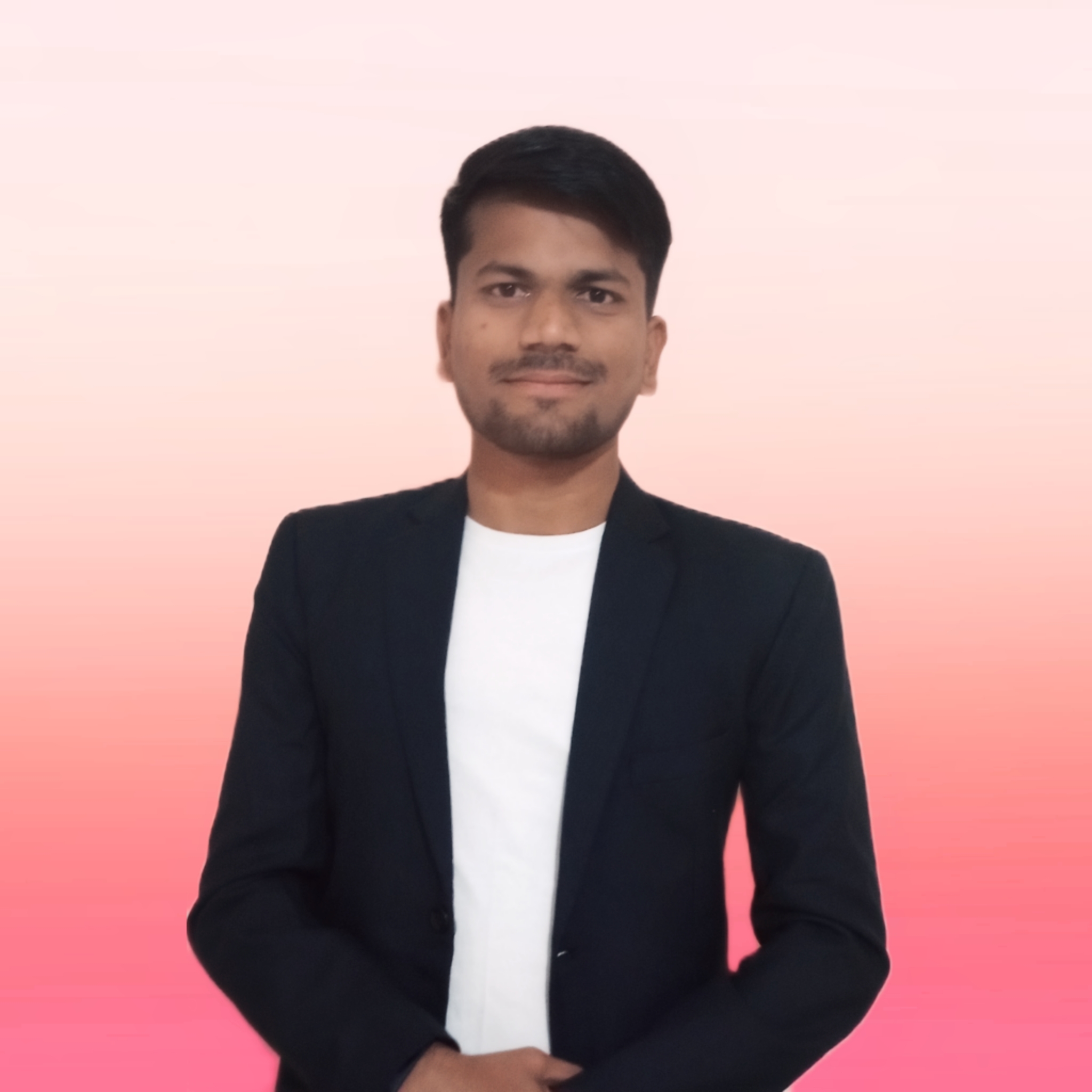
Manoj Jaiswal
Manoj Jaiswal
I am a Software Engineer with 3+ years of experience designing and developing mobile and web applications using flutter and python django. I specialization in flutter and have professional experience working with dart language. Also i have experience working with python django and node js.