Getting Started with Jetpack Compose: My First Steps in Modern Android UI

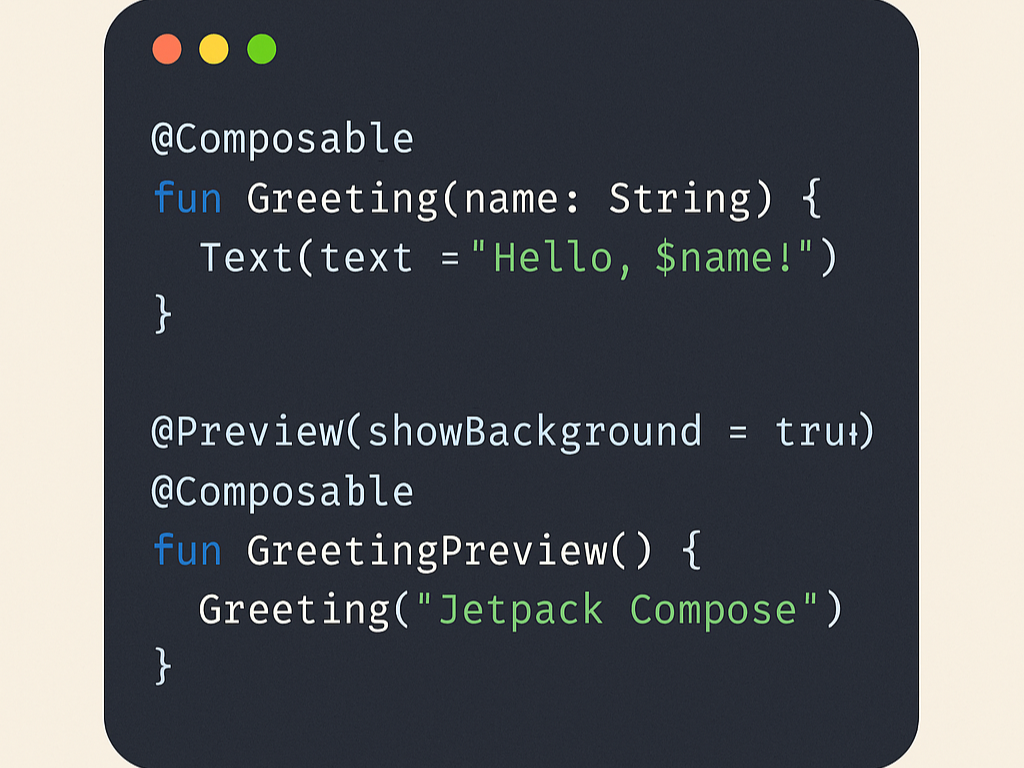
👋 Introduction
As an Android developer currently diving deep into Jetpack Compose, I’ve decided to document my learning journey - not just to help myself revise, but also to help others who are starting out. This is the first post in a series where I’ll share what I learn while exploring Jetpack Compose, the modern toolkit for building native UIs in Android.
🧠 What is Jetpack Compose?
Jetpack Compose is Android’s recommended way to build UI. Unlike the traditional XML approach, Compose lets you build UI using Kotlin code directly. It’s declarative, reactive, and very developer-friendly.
With Compose, we no longer need to define UI layouts separately in XML files. Instead, we describe the UI in composable functions - the UI updates automatically when the state changes.
🛠️ Setting Up Jetpack Compose
To start working with Jetpack Compose, I created a new project in Android Studio Giraffe or above, and selected the “Empty Compose Activity” template.
Here are the dependencies added by default:
dependencies {
implementation "androidx.activity:activity-compose:1.8.0"
implementation "androidx.compose.ui:ui:1.5.0"
implementation "androidx.compose.material3:material3:1.2.0"
implementation "androidx.lifecycle:lifecycle-runtime-ktx:2.6.1"
}
⚠️ Make sure you’re using at least Kotlin 1.9.0+ and the latest stable version of Compose.
💡 My First Composable Function
The first function I created was a basic Greeting
message:
@Composable
fun Greeting(name: String) {
Text(text = "Hello, $name!")
}
Then, I used it inside the MainActivity.kt
like this:
setContent {
MaterialTheme {
Greeting("Tejas")
}
}
This was a game-changer moment for me — writing UI directly in Kotlin is way smoother and more fun than XML!
🔍 Previewing the UI
Jetpack Compose supports live previews in Android Studio. Just add a @Preview
annotation to your composable like this:
@Preview(showBackground = true)
@Composable
fun GreetingPreview() {
Greeting("Jetpack Compose")
}
This feature helped me see UI changes without running the app on an emulator or physical device. It’s a huge time saver during development.
📝 What I Learned
Composables are the building blocks of Jetpack Compose.
You can easily preview UI using
@Preview
.The Compose setup is beginner-friendly, especially with Android Studio templates.
Learning through official Android training was very helpful.
📚 What's Next?
In my next post, I’ll dive into:
State and recomposition
Layouts like
Column
,Row
,Box
Material Design 3 with Compose
If you’re also learning Compose, feel free to follow along or share your experiences!
🙌 Thanks for Reading!
If you found this helpful, stay tuned for more posts from my Compose learning journey. You can connect with me on GitHub or drop a comment below.
Subscribe to my newsletter
Read articles from Tejas Kanazriya directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
