JavaBeans – Wait, What Are These Beans Doing in Java? ☕
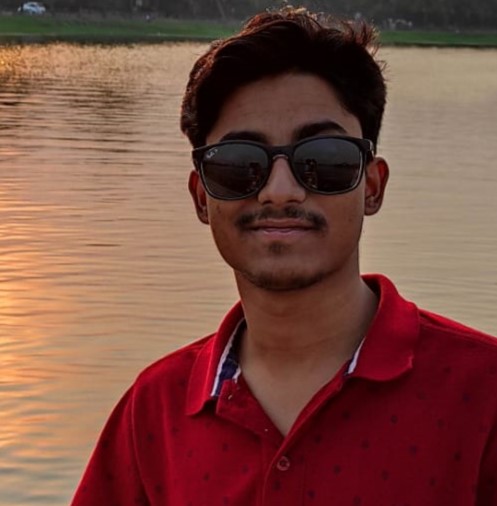

So, I recently started learning Spring Framework, and right at the beginning—boom 💥—I ran into this term: JavaBean.
At first, I was like, “Is this another one of those unnecessarily fancy Java names?” But then I Googled, watched a few YouTube videos, and yeah... it actually made sense.
Let’s break it down—student to student.
So, What the Heck Is a JavaBean? 🫘
A JavaBean is basically just a simple Java class that follows a few rules. That’s it. Nothing crazy.
Here’s the recipe (yes, like cooking):
It should have a no-arg constructor – Like, default constructor with zero parameters.
All properties should be private – So no one can mess with your variables directly.
Use getters and setters – To access and modify those private variables.
Should be serializable (sometimes) – Not mandatory always, but good to have if you’re planning to save the bean's state.
Example time:
public class Student {
private String name;
private int age;
public Student() {
// No-arg constructor
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
}
Yup. That’s literally a JavaBean. Just a clean, modular way to manage data.
Why JavaBeans Though? 🤷♂️
This was my next question. Like, “Why can’t we just use regular classes?”
Turns out, JavaBeans are super useful, especially when you're working with frameworks like Spring, JSP, or any tool that needs to plug into your code and magically manage things behind the scenes.
Here’s what JavaBeans bring to the table:
✨ Encapsulation: Keeps your code clean and maintainable.
🔗 Framework Friendly: Spring uses JavaBeans for Dependency Injection (you’ll see it a lot!).
💾 Easily Serializable: Great when you need to transfer data or store object state.
Think of a JavaBean like a polite guest. It doesn’t mess around, follows the rules, and works well with others.
Okay, But Where Do We Use This?
I ran into JavaBeans while learning Spring, and here’s how it clicks:
In Spring, we often create classes like User
, Product
, Employee
, etc., and use them as beans to hold and pass around data.
Also, frameworks can automatically detect these beans and inject them wherever needed—no manual effort. That’s the whole “Inversion of Control” and “Dependency Injection” magic you’ll eventually learn (or maybe already have 😅).
In a Nutshell (TLDR) 🥜
A JavaBean is just a normal Java class that follows some structure: private fields, public getters/setters, and a no-arg constructor.
Used heavily in frameworks like Spring to represent data and help with dependency injection.
Keeps your code modular, clean, and framework-compatible.
You’ll be writing a lot of JavaBeans while working with Spring, so better get comfy with it early on.
Let me know if this helped or if you still find “JavaBean” a weird name 😂 (I still kinda do). Catch you in the next post!
Subscribe to my newsletter
Read articles from Arkadipta Kundu directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
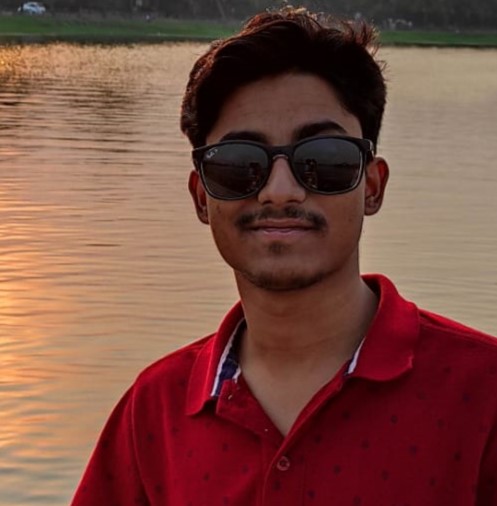
Arkadipta Kundu
Arkadipta Kundu
I’m a Computer Science undergrad from India with a passion for coding and building things that make an impact. Skilled in Java, Data Structures and Algorithms (DSA), and web development, I love diving into problem-solving challenges and constantly learning. Right now, I’m focused on sharpening my DSA skills and expanding my expertise in Java development.