Speedup Your App by adopting WebP Images

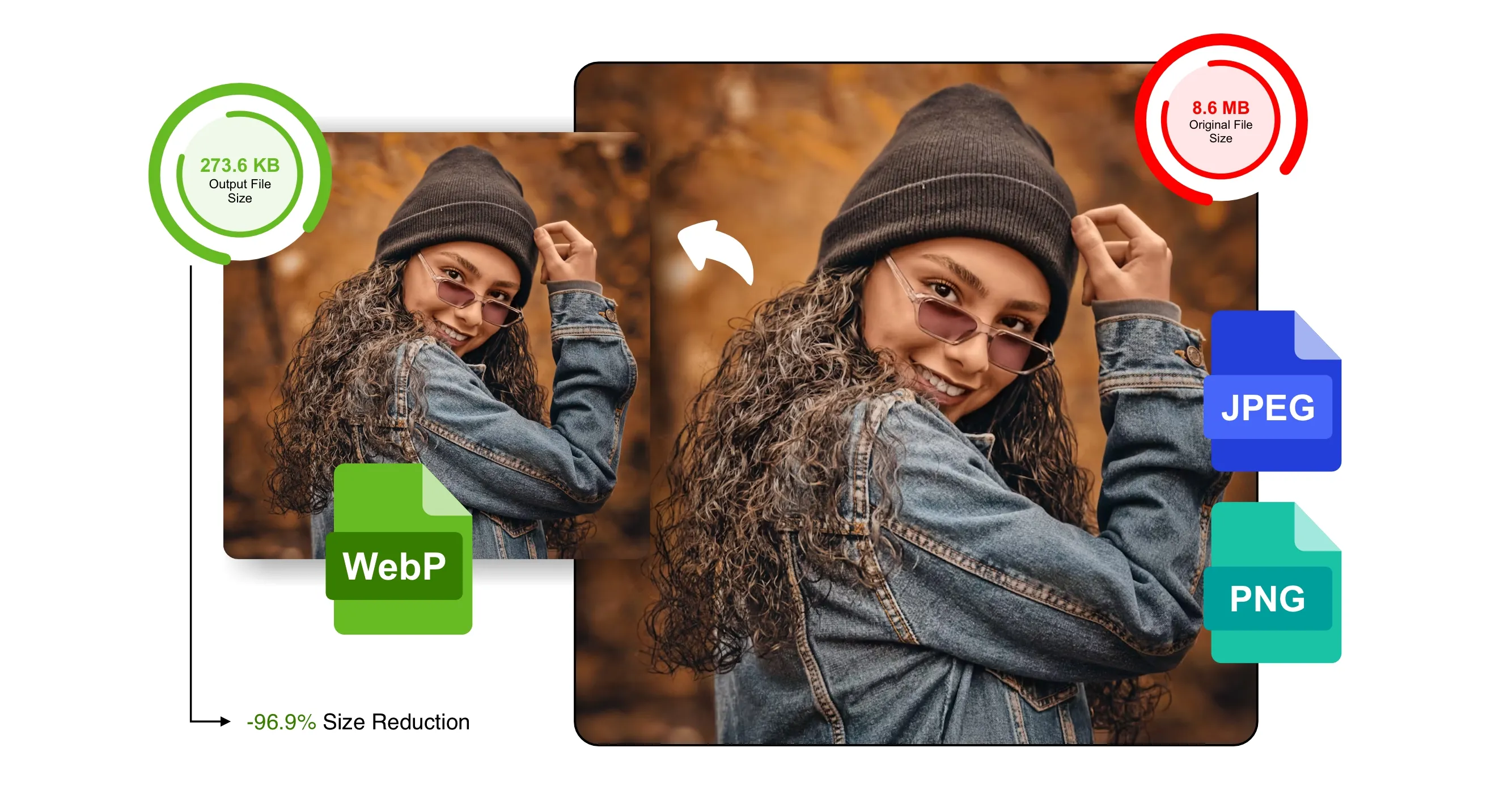
Introduction
Many apps face significant challenges with performance and optimization due to asset loading, with images often being a major factor in slowing things down. In numerous web and mobile apps, the primary delay in loading screens is caused by large image sizes. Apps must strike a balance between maintaining high-quality images and keeping file sizes small. That's where WebP becomes invaluable!
What is WebP?
Google first announced WebP on September 30, 2010, as a new open format for lossy compressed true-color graphics on the web. Compared to other image formats like PNG, JPEG, GIF, and HEIC, WebP is a modern image format. It offers better lossless and lossy compression for images. By using the WebP format, you can create smaller image sizes with higher quality, making your application faster.
Major Benefits of WebP
Compression methods: WebP supports both lossy (like JPEG) and lossless (like PNG) compression, allowing you to balance file size and image quality.
Lossy WebP compression uses predictive coding to encode an image, the same method used by the VP8 video codec to compress keyframes in videos.
Lossless WebP compression uses previously seen image fragments to accurately reconstruct new pixels. It can also use a local palette if no suitable match is found.
Smaller File Sizes: WebP images can achieve smaller file sizes compared to JPEG and PNG while maintaining high quality, leading to faster loading times.
Transparency Support: It supports alpha transparency, similar to PNG, allowing for images with transparent backgrounds.
Open Source: WebP is an open-source format, it’s the same technology that Next.js employs for its image optimization.
Animation Support: Similar to Gifs, WebP also supports animation.
Widely Supported: WebP is compatible with major web browsers, including Chrome, Firefox, Safari, Edge, and Opera, as well as on Android and iOS.
Implementing a simple WebP converter using Sharp
Sharp is a fast Node-API module primarily used to convert large images into smaller, web-friendly formats like JPEG, PNG, WebP, GIF, and AVIF. It uses an upstream dependency called libvips which is a demand-driven, horizontally threaded image processing library. It runs quickly and uses little memory. It also supports rotation, extraction, compositing, and gamma correction, and most modern systems don't need additional dependencies. Next.js uses Sharp internally for its image optimization.
Let’s implement a simple convertToWebP
function:
interface ConversionOptions {
quality: number;
width?: number;
height?: number;
}
export async function convertToWebP(
inputPath: string,
outputPath?: string,
options: ConversionOptions = { quality: 100 }
): Promise<{ outputPath: string; size: number }> {
try {
if (!(await fs.pathExists(inputPath))) {
throw new Error(`Input file not found: ${inputPath}`);
}
if (!outputPath) {
const parsedPath = path.parse(inputPath);
outputPath = path.join(parsedPath.dir, `${parsedPath.name}.webp`);
}
const buffer = await fs.readFile(inputPath);
// Initialize Sharp instance
const sharpInstance = sharp(buffer);
// Apply resizing if width or height is provided
if (options.width || options.height) {
sharpInstance.resize(options.width, options.height, {
fit: 'inside',
withoutEnlargement: true,
});
}
// Convert to WebP
const webpBuffer = await sharpInstance
.webp({ quality: options.quality })
.toBuffer();
await fs.writeFile(outputPath, webpBuffer);
const stats = await fs.stat(outputPath);
return {
outputPath,
size: stats.size,
};
} catch (error) {
console.error('Error converting image:', error);
throw error;
}
}
You can find the complete code for this CLI in this repository. Here's the npm package as well.
Using npx (no installation required)
You can easily use the underlying technology, libvips. Alternatively, I have created a Node.js CLI that extends the Sharp package. You can run the CLI directly without installing it by using npx:
$ npx webp-image-cli convert <options>
# Basic usage - convert a single file
$ npx webp-image-cli convert input.jpg
# Convert all images in a directory
$ npx webp-image-cli convert images/
# Convert all images in a directory and its subdirectories
$ npx webp-image-cli convert images/ -r
# Convert with custom quality
$ npx webp-image-cli convert input.jpg -q 90
# Resize image
$ npx webp-image-cli convert input.jpg -w 800 -h 600
# Force overwriting existing files
$ npx webp-image-cli convert input.jpg --overwrite
Result from converting all images in a directory:
If you want to permanently install a package, you would use npm install -g webp-image-cli
instead.
Using a simple Nextjs app
A simple full-stack Next.js app was also created for a visual demonstration. You can find the codebase here.
As you can see from the image, we achieved an 82.4%
reduction in size without losing any quality. By converting the same image at 80% quality, we achieved a 96.9%
reduction in size with no noticeable loss in quality.
I usually use the CLI to convert simple image assets downloaded from Figma or other sources. You can take additional steps based on your specific needs and use case. For example, if your application relies heavily on managing a large number of image assets, you might consider a more robust solution. One effective approach is to create a Lambda function that handles all image manipulations and conversions dynamically as they are requested. This setup allows the Lambda function to process images on the fly, reducing the processing load on your main application. By offloading these tasks to a serverless function, you can improve the overall performance and responsiveness of your application, ensuring it can efficiently handle high volumes of image processing without compromising speed or user experience.
Conclusion
Adopting the WebP image format can significantly enhance your app's performance by reducing image file sizes without compromising quality. This leads to faster loading times, reduced bandwidth usage, and an overall improved user experience. As the industry continues to embrace WebP, its benefits become increasingly evident, making it a valuable tool for developers aiming to optimize their applications. By implementing WebP, you can stay ahead in the ever-evolving landscape of image optimization and ensure your app delivers a seamless and efficient experience to users.
Subscribe to my newsletter
Read articles from Taiwo Ogunola directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Taiwo Ogunola
Taiwo Ogunola
With over 5 years of experience in delivering technical solutions, I specialize in web page performance, scalability, and developer experience. My passion lies in crafting elegant solutions to complex problems, leveraging a diverse expertise in web technologies.