How to build a GenAI RAG app from scratch
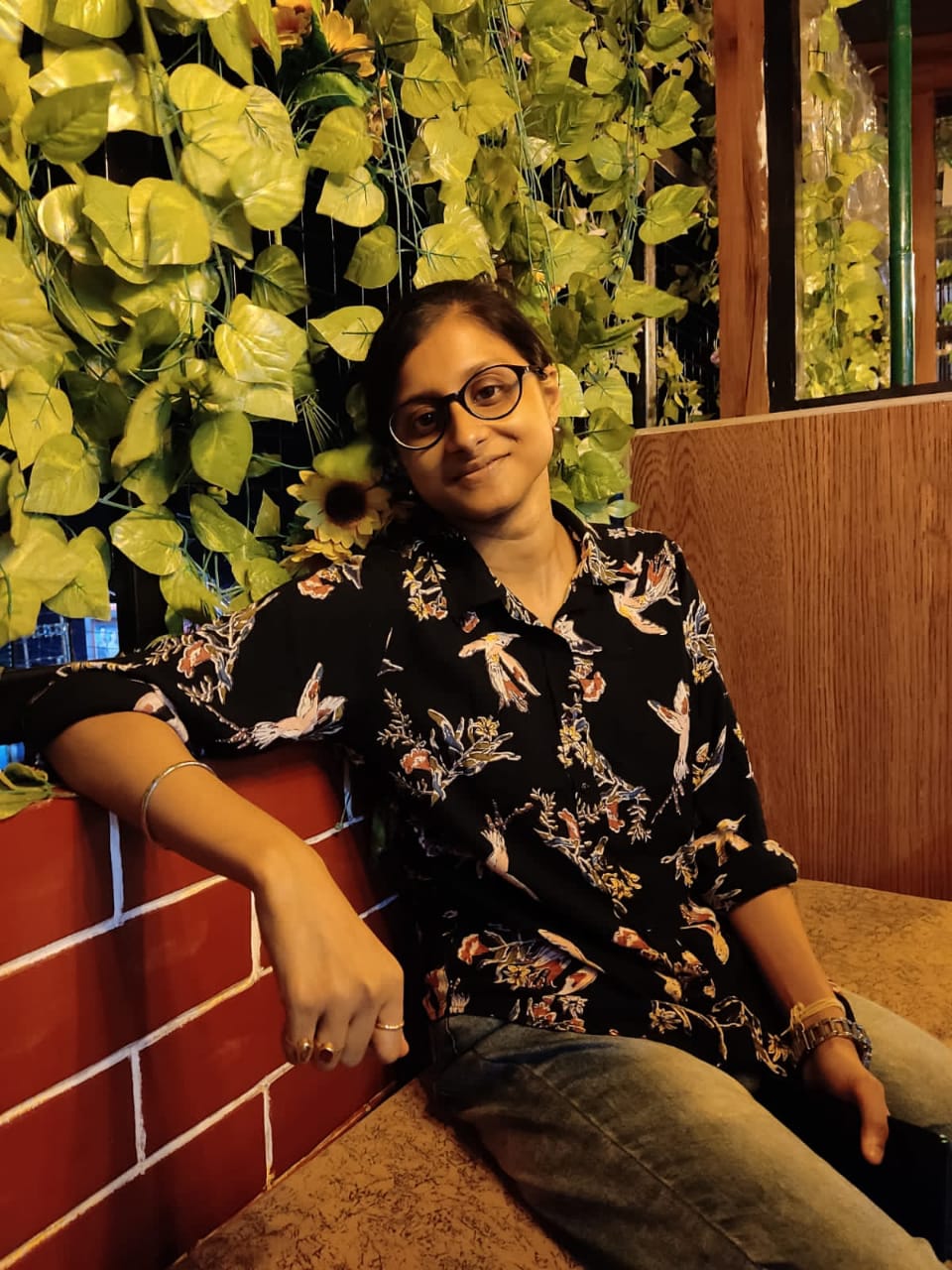

Have you ever had to pause a board game to search online for the rules of "Free Parking" in Monopoly, or debated with friends about skipping turns in UNO?
I certainly have, which led me to create BoardBuddy, a GenAI-powered assistant designed to comprehend board game rulebooks and provide answers as a human co-player would.
This project was developed as part of the Google Cloud GenAI Capstone on Kaggle, where the objective was to utilize at least three GenAI capabilities to create something practical, engaging, and beneficial. I ultimately incorporated six capabilities.
💡 The Idea
BoardBuddy allows you to type in any natural language question, such as:
🗨️ "How do I get out of jail in Monopoly?"
🗨️ "What’s the objective in Chess?"
🗨️ "Can I stack Draw 2 cards in UNO?"
And receive a direct answer based on the rulebook. No more flipping through pages of PDFs while your game night excitement dwindles..
🔧 Tools & Tech Stack
I intentionally kept the tech lightweight so it could run on a Kaggle Notebook without needing GPUs or cloud APIs:
Function | Tool/Library |
Rulebook Parsing | Plain Text Files (.txt ) |
Text Embedding | sentence-transformers |
Vector Search | sklearn.NearestNeighbors |
Language Model for QA | GPT-2 via HuggingFace Transformers |
Interface (Optional) | Gradio |
Platform | Kaggle |
No cloud compute. No databases. Just local models and smart logic.
🧠 GenAI Capabilities Used
These are the GenAI capabilities I applied:
✅ Document Understanding — Parsing large rulebooks
✅ Embeddings — Converting text into vector representations
✅ Vector Search — Finding the most relevant rule chunks
✅ RAG (Retrieval-Augmented Generation) — Feeding context to LLM
✅ Agents — Multi-step reasoning (e.g. game-specific workflows)
✅ Structured Output — Returning JSON for developer-friendly outputs
🧱 How It Works
The app has 3 core steps:
1. Load the Rulebook
Each rulebook (Monopoly, Chess, UNO) is stored as a .txt
file and loaded dynamically based on user choice.
def load_rulebook(game="monopoly"):
with open(f"/kaggle/input/{game}-rules.txt", "r") as f:
return f.read()
2. Convert to Vectors
The rulebook is split into chunks (by paragraphs), and each chunk is converted into a vector using the all-MiniLM-L6-v2
model.
from sentence_transformers import SentenceTransformer
model = SentenceTransformer('all-MiniLM-L6-v2')
chunks = rules_text.split("\n\n")
embeddings = model.encode(chunks)
3. Search + Generate Answer
When a user asks a question, we:
Embed the query
Find the top 3 closest chunks
Feed them into GPT-2 with a prompt like:
Context:
[3 relevant rule chunks]
Question: How do I win in Monopoly?
Answer:
The language model then fills in the answer.
🔬 Sample Output
Query: "How do I get out of jail in Monopoly?"
{
"question": "How do I get out of jail in Monopoly?",
"answer": "You can get out of jail by rolling doubles, using a 'Get Out of Jail Free' card, or paying a $50 fine."
}
🎮 Supported Games (So Far)
✅ Monopoly
✅ Chess
✅ UNO
Adding more is as easy as dropping a .txt
file in the input folder.
🧪 Optional: Add a GUI
Using Gradio, you can spin up a simple interactive UI inside the notebook.
import gradio as gr
def gradio_rag(query, game):
rules_text = load_rulebook(game)
chunks = rules_text.split("\n\n")
embeddings = model.encode(chunks)
index.fit(embeddings)
return json.loads(answer_query(query))["answer"]
gr.Interface(
fn=gradio_rag,
inputs=["text", gr.Dropdown(["monopoly", "chess", "uno"])],
outputs="text"
).launch()
🔮 What’s Next?
🧠 Switch to powerful LLMs like Claude or Gemini for better answers
🧾 Add PDF and image parsing to read scanned rulebooks
🧰 Use FAISS or Weaviate for scalable vector search
💬 Support multilingual queries
✍️ What I Learned
You don’t need a GPU to build GenAI tools — local models + smart logic go a long way
RAG is super powerful for niche knowledge bases (like rulebooks)
Thinking like a user (in this case, a casual gamer) really helps shape better prompts and UX
🚀 Try It Out!
👉 [Kaggle Notebook (Kaggle)]
📁 Input: monopoly-rules.txt
, chess-rules.txt
, uno-rules.txt
🧪 Output: JSON-formatted answers you can plug into any app
🧠 Final Thought
If GenAI is the future of interaction, niche assistants like BoardBuddy are the future of contextual intelligence. Instead of building one massive brain, we can build a thousand tiny experts.
Thanks for reading! Let me know what game you want me to add next. 🎲♟️🃏
Would you like this post exported as a markdown file for Hashnode, or want a catchy banner image to go with it?
Subscribe to my newsletter
Read articles from Sulagna Dutta Roy directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
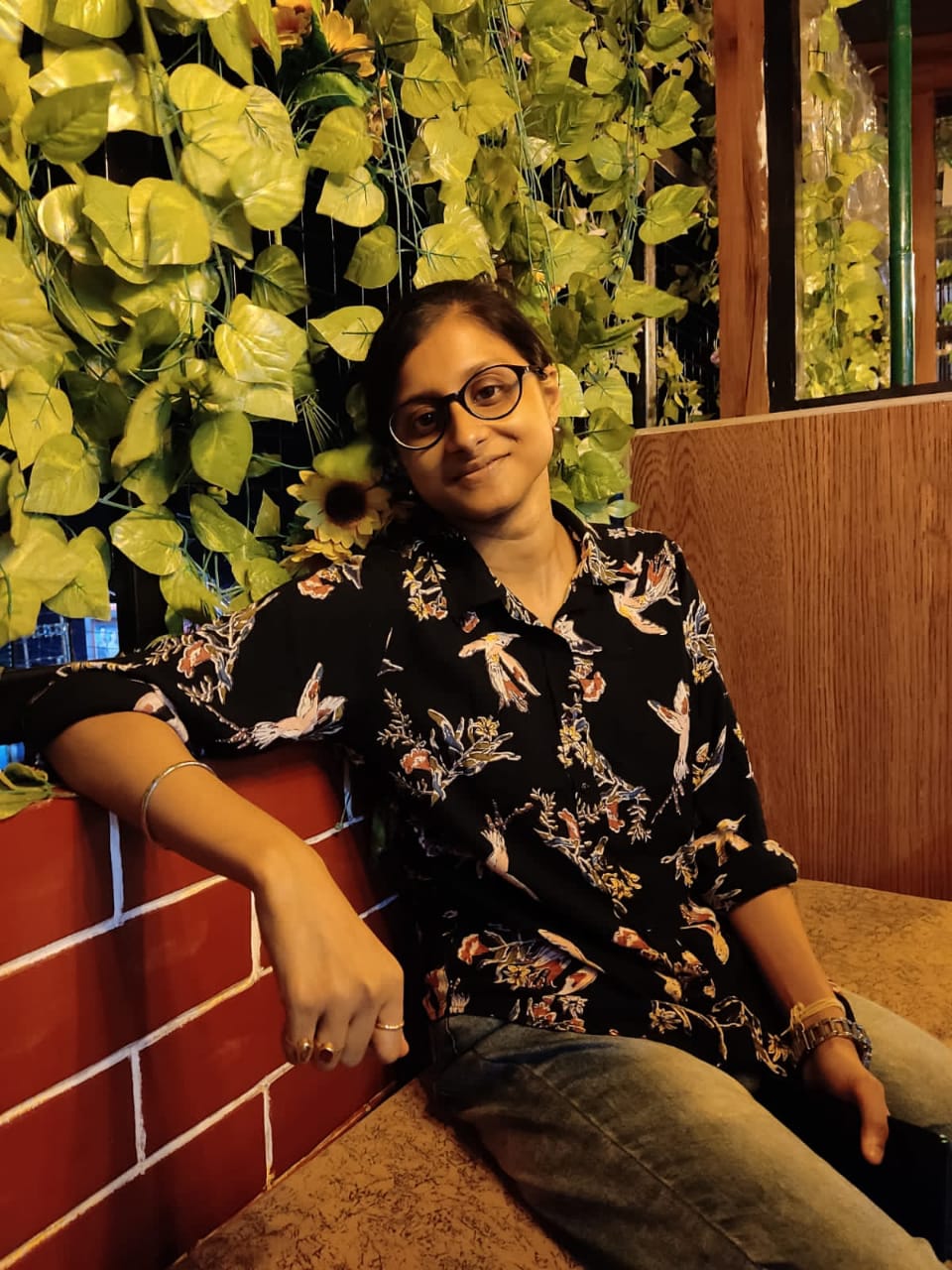
Sulagna Dutta Roy
Sulagna Dutta Roy
Your friendly software developer who loves to explore different tech stacks.