Unit – II Modules and Package
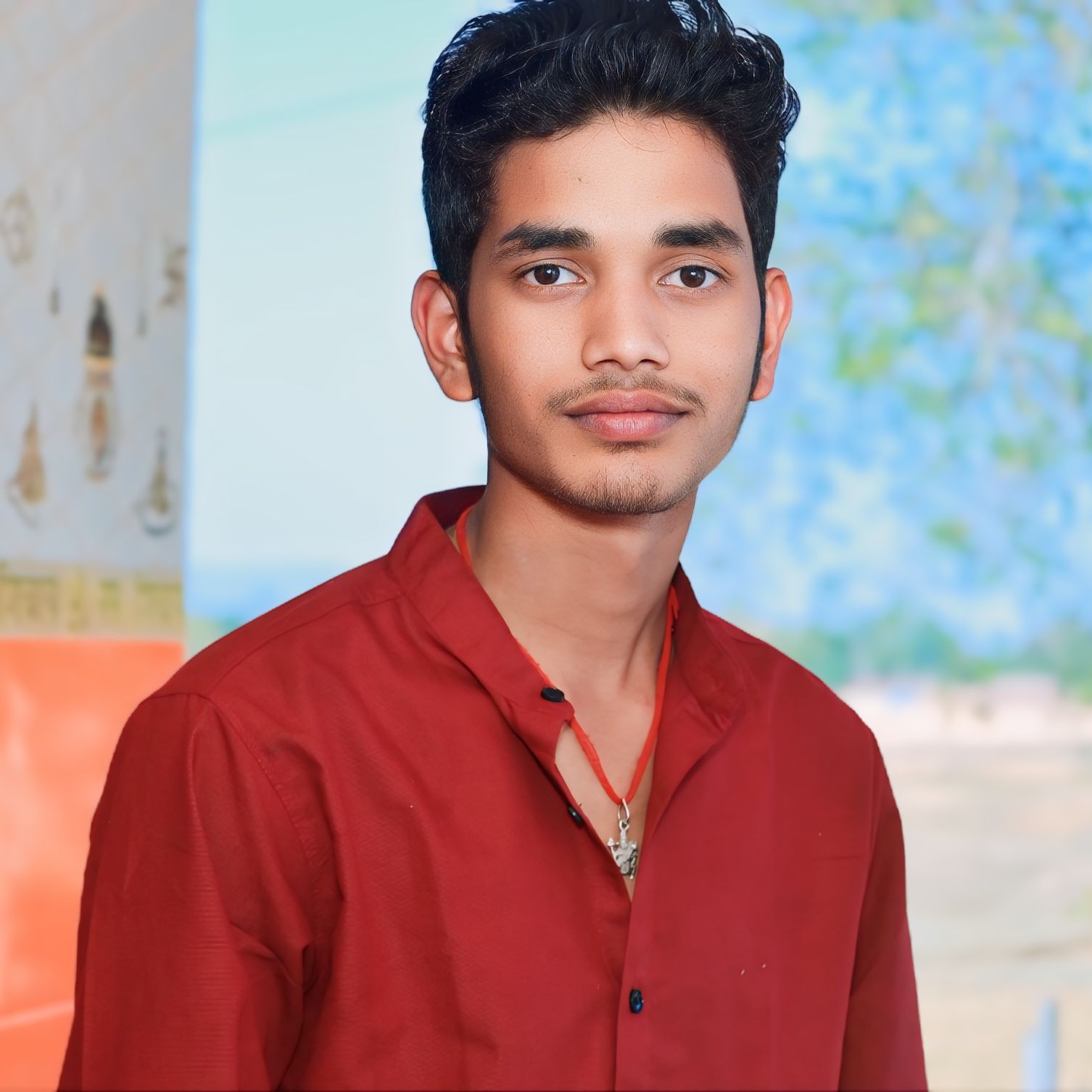
Table of contents
- Modules:
- Types of Modules in Python
- Variables in Module
- Create a Python Module
- Variables in Module
- Create a Python Module
- Variables in Module
- Create a Python Module
- Import module in Python
- Python Import From Module
- Import all Names
- Locating Python Modules
- Directories List for Modules
- Output:
- [‘/home/nikhil/Desktop/gfg’, ‘/usr/lib/python38.zip’, ‘/usr/lib/python3.8’, ‘/usr/lib/python3.8/lib-dynload’, ”, ‘/home/nikhil/.local/lib/python3.8/site-packages’, ‘/usr/local/lib/python3.8/dist-packages’, ‘/usr/lib/python3/dist-packages’, ‘/usr/local/lib/python3.8/dist-packages/IPython/extensions’, ‘/home/nikhil/.ipython’]
- Renaming the Python Module
- Python Built-in modules
- Packages
Modules:
A Python module contains Python code (functions, classes, and variables) that can be reused in different programs.
Modules in Python are just Python files with a .py extension. The name of the module is the same as the file name.
A Python module can have a set of functions, classes, or variables defined and implemented.
Types of Modules in Python
There are three ways to define a module in Python:
Written in Python
A module is simply a .py file containing Python code.
Example: mymodule.py containing functions and variables.
Written in C (Dynamically Loaded Modules)
Some modules are written in C for performance reasons and loaded dynamically at runtime.
Example: re module (for regular expressions).
Built-in Modules
These modules are part of the Python interpreter and do not require separate installation.
Example: itertools, sys, math, etc.
Variables in Module
The module can contain functions, as already described, but also variables of all types (arrays, dictionaries, objects etc):
Create a Python Module
To create a Python module, write the desired code and save that in a file with .py extension.
Some modules are written in C for performance reasons and loaded
dynamically at runtime.Example: re module (for regular expressions).
Built-in Modules
These modules are part of the Python interpreter and do not require
separate installation.Example: itertools, sys, math, etc.
Variables in Module
The module can contain functions, as already described, but also variables of all
types (arrays, dictionaries, objects etc):
Create a Python Module
To create a Python module, write the desired code and save that in a file with .py extension.
Write Python code in a .py file.
Save the file with a .py extension.
Import the module in another script using import module_name.
Code:
These modules are part of the Python interpreter and do not require
separate installation.Example: itertools, sys, math, etc.
Variables in Module
The module can contain functions, as already described, but also variables of all
types (arrays, dictionaries, objects etc):
Create a Python Module
To create a Python module, write the desired code and save that in a file with .py extension.
Write Python code in a .py file.
Save the file with a .py extension.
Import the module in another script using import module_name.
Code:
Syntax:
Def function_name():
Return “ ”
Example: def college_info():
return "Welcome to Government Polytechnic Adityapur!"
def course_details():
return ["Mechanical Engineering", "Civil Engineering", "Electrical Engineering", "Computer Science"]
Import module in Python
We can import the functions, and classes defined in a module to another module using the import statement in some other Python source file.
When the interpreter encounters an import statement, it imports the module if the module is present in the search path.
Note: A search path is a list of directories that the interpreter searches for importing a module.
For example, to import the module calc.py, we need to put the following command at the top of the script.
Syntax to Import-Module in Python
import module_name
Note: This does not import the functions or classes directly, instead imports the module only. To access the functions inside the module the dot(.) operator is used.
Importing modules in Python Example
Now, we are importing the calc that we created earlier to perform add operation.
# importing module calc.py
import calc
print(calc.add(10, 2))
Output:
12
Python Import From Module
Python’s ‘from’ statement lets you import specific attributes from a module without importing the module as a whole.
Import Specific Attributes from a Python module
Here, we are importing specific sqrt and factorial attributes from the math module.
# importing sqrt() and factorial from the
# module math
from math import sqrt, factorial
# if we simply do "import math", then
# math.sqrt(16) and math.factorial()
# are required.
print(sqrt(16))
print(factorial(6))
Output
4.0
720
Import all Names
The ‘*’ symbol used with the import statement is used to import all the names from a module to a current namespace.
Syntax:
from module_name import *
What does import * do in Python?
The use of has its advantages and disadvantages. If you know exactly what you will be needing from the module, it is not recommended to use , else do so.
# importing sqrt() and factorial from the
# module math
from math import *
if we simply do "import math", then
# math.sqrt(16) and math.factorial()
# are required.
print(sqrt(16))
print(factorial(6))
Output:
4.0
720
Locating Python Modules
Whenever a module is imported in Python the interpreter looks for several locations. First, it will check for the built-in module, if not found then it looks for a list of directories defined in the sys. path. Python interpreter searches for the module in the following manner –
First, it searches for the module in the current directory.
If the module isn’t found in the current directory, Python then searches each directory in the shell variable PYTHONPATH. PYTHON PATH is an environment variable, consisting of a list of directories.
If that also fails python checks the installation-dependent list of directories configured at the time Python is installed.
Directories List for Modules
Here, sys.path is a built-in variable within the sys module. It contains a list of directories that the interpreter will search for the required module.
# importing sys module
import sys
# importing sys.path
print(sys.path)
Output:
[‘/home/nikhil/Desktop/gfg’, ‘/usr/lib/python38.zip’, ‘/usr/lib/python3.8’, ‘/usr/lib/python3.8/lib-dynload’, ”, ‘/home/nikhil/.local/lib/python3.8/site-packages’, ‘/usr/local/lib/python3.8/dist-packages’, ‘/usr/lib/python3/dist-packages’, ‘/usr/local/lib/python3.8/dist-packages/IPython/extensions’, ‘/home/nikhil/.ipython’]
Renaming the Python Module
We can rename the module while importing it using the keyword
Syntax: Import Module_name as Alias_name
# importing sqrt() and factorial from the
# module math
import math as mt
# if we simply do "import math", then
# math.sqrt(16) and math.factorial()
# are required.
print(mt.sqrt(16))
print(mt.factorial(6))
Output:
4.0
720
Python Built-in modules
There are several built-in modules in Python, which you can import whenever you like.
# importing built-in module math
import math
# using square root(sqrt) function contained
# in math module
print(math.sqrt(25))
# using pi function contained in math module
print(math.pi)
# 2 radians = 114.59 degrees
print(math.degrees(2))
# 60 degrees = 1.04 radians
print(math.radians(60))
# Sine of 2 radians
print(math.sin(2))
# Cosine of 0.5 radians
print(math.cos(0.5))
# Tangent of 0.23 radians
print(math.tan(0.23))
# 1 2 3 * 4 = 24
print(math.factorial(4))
# importing built in module random
import random
# printing random integer between 0 and 5
print(random.randint(0, 5))
# print random floating point number between 0 and 1
print(random.random())
# random number between 0 and 100
print(random.random() * 100)
List = [1, 4, True, 800, "python", 27, "hello"]
# using choice function in random module for choosing
# a random element from a set such as a list
print(random.choice(List))
# importing built in module datetime
import datetime
from datetime import date
import time
# Returns the number of seconds since the
# Unix Epoch, January 1st 1970
print(time.time())
# Converts a number of seconds to a date object
print(date.fromtimestamp(454554))
Output:
5.0
3.14159265359
114.591559026
1.0471975512
0.909297426826
0.87758256189
0.234143362351
24
3
0.401533172951
88.4917616788
True
1461425771.87
Packages
Python packages are a way to organize and structure code by grouping related modules into directories. A package is essentially a folder that contains an init.py file and one or more Python files (modules). This organization helps manage and reuse code effectively, especially in larger projects. It also allows functionality to be easily shared and distributed across different applications. Packages act like toolboxes, storing and organizing tools (functions and classes) for efficient access and reuse.
Key Components of a Python Package
Module: A single Python file containing reusable code (e.g., math.py).
Package: A directory containing modules and a special init.py file.
Sub-Packages: Packages nested within other packages for deeper organization.
How to create and access packages in python
Create a Directory: Make a directory for your package. This will serve as the root folder.
Add Modules: Add Python files (modules) to the directory, each representing specific functionality.
Include init.py: Add an init.py file (can be empty) to the directory to mark it as a package.
Add Sub packages (Optional): Create subdirectories with their own init.py files for sub packages.
Import Modules: Use dot notation to import, e.g., from mypackage.module1 import greet.
Example :
In this example, we are creating a Math Operation Package to organize Python code into a structured package with two sub-packages: basic (for addition and subtraction) and advanced (for multiplication and division). Each operation is implemented in separate modules, allowing for modular, reusable and maintainable code.
math_operations/__init__.py:
This init.py file initializes the main package by importing and exposing the calculate function and operations (add, subtract, multiply, divide) from the respective sub-packages for easier access.
# Initialize the main package
from .calculate import calculate
from .basic import add, subtract
from .advanced import multiply, divide
math_operations/calculator.py:
This calculate file is a simple placeholder that prints “Performing calculation…”, serving as a basic demonstration or utility within the package.
def calculate():
print("Performing calculation...")
math_operations/basic/__init__.py:
This init.py file initializes the basic sub-package by importing and exposing the add and subtract functions from their respective modules (add.py and sub.py). This makes these functions accessible when the basic sub-package is imported.
# Export functions from the basic sub-package
from .add import add
from .sub import subtract
math_operations/basic/add.py:
def add(a, b):
return a + b
math_operations/basic/sub.py:
def subtract(a, b):
return a - b
In the same way we can create the sub package advanced with multiply and divide modules. Now, let’s take an example of importing the module into a code and using the function:
from math_operations import calculate, add, subtract
# Using the placeholder calculate function
calculate()
# Perform basic operations
print("Addition:", add(5, 3))
print("Subtraction:", subtract(10, 4))
Output:
6
8
Subscribe to my newsletter
Read articles from Sagar Sangam directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
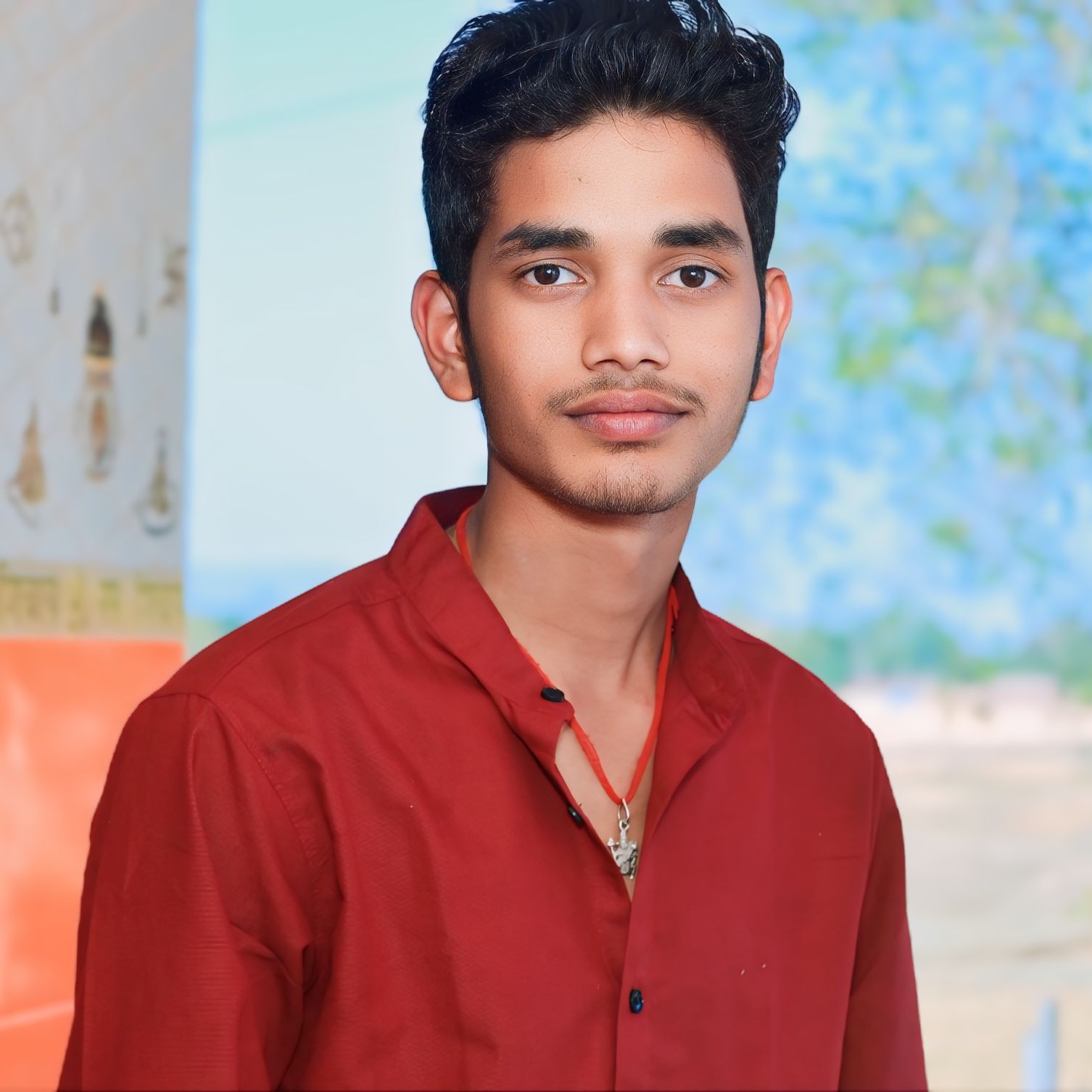