Object-Oriented Programming (OOP) with Java β A Beginner's Dive from Procedural to OOP
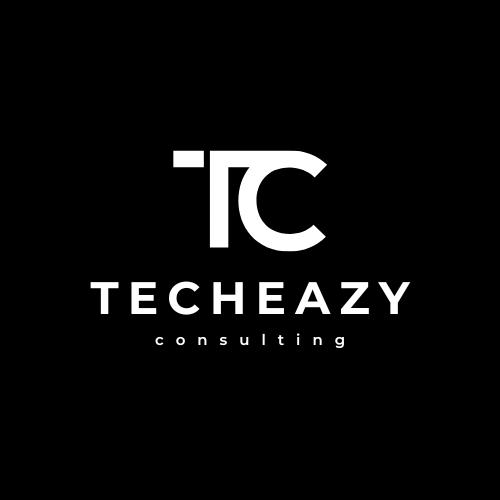
This session was part of our hands-on training program: OOPs with Java β by Techeazy Consulting, designed to help freshers bridge the gap between academic learning and real-world software development.
In this session, we transitioned from procedural thinking to an object-oriented mindset, using Java as our guide. Through real-world analogies, interactive discussions, and code examples, students learned how to model real-world problems using OOP principles.
π Procedural vs Object-Oriented β A Real-Life Analogy
Scenario: Starting an Engine
π§ Procedural Approach:
void turnOnEngine(Engine engine) {
if (engine.type.equals("Diesel")) {
// Start diesel engine
} else if (engine.type.equals("Electric")) {
// Start electric engine
}
}
Here, every time you add a new engine type, you modify the same methodβa clear violation of the Open-Closed Principle.
β OOP Approach:
abstract class Engine {
abstract void turnOn();
}
class DieselEngine extends Engine {
void turnOn() {
// Specific code to turn on Diesel Engine
}
}
class ElectricEngine extends Engine {
void turnOn() {
// Specific code to turn on Electric Engine
}
}
Now, the behavior is encapsulated inside classes, and new engines can be added without touching existing logic.
π Key OOP Concepts We Explored
Concept | What It Means | Real-Life Example |
Abstraction | Hiding details to show only essentials | A car's "Drive" button, not inner wiring |
Encapsulation | Binding data and behavior inside one unit | A washing machineβs control panel |
Inheritance | Acquiring properties from a parent class | A Dog class inheriting from Animal |
Polymorphism | One interface, many implementations | A shape.draw() for circle, square, etc. |
π Real-World Case Study: College ERP System
To make it relatable, we walked through a College ERP System.
Problem Statement:
"A college wants to store and manage records of students, teachers, and non-teaching staff. It must support adding, viewing, and calculating salaries."
OOP Modeling:
class Person {
String name;
String email;
void showDetails() { ... }
}
class Student extends Person {
String rollNumber;
void showStudentDetails() { ... }
}
class Teacher extends Person {
double salary;
void calculateSalary() { ... }
}
class NonTeachingStaff extends Person {
double wage;
void calculateSalary() { ... }
}
By using inheritance, we reduced duplication. With polymorphism, we created unified handling:
List<Person> people = List.of(new Student(), new Teacher());
for (Person p : people) {
p.showDetails(); // Calls appropriate version
}
π‘ This case study really resonated with students. Want to join similar real-world breakdowns? Check out our full OOP with Java course.
π Interview-Ready Insights
We wrapped up the session with practical, interview-focused Q&A:
Q: Difference between Abstraction and Encapsulation?
Q: Why Java doesn't support multiple inheritance with classes?
Q: What is method overloading vs overriding?
Q: Why use interfaces when we have abstract classes?
We also touched on access modifiers, constructor chaining, and real-world class design tips.
π From OOP to Enterprise Java
This foundation sets the stage for our upcoming sessions on:
β Spring Framework & Spring Boot
β REST APIs using Java
β Spring Data JPA & Hibernate
β Integration with AWS and microservices
Subscribe to my newsletter
Read articles from TechEazy Consulting directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
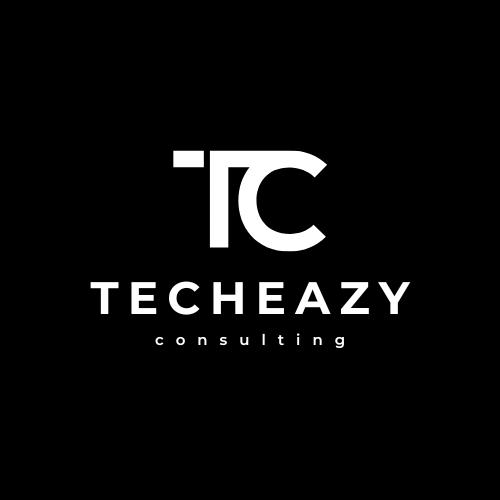