In-depth Guide to Manipulating Embedded Images in Excel with Golang
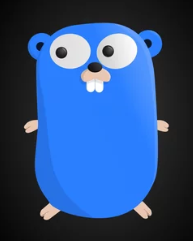

Excel is an incredibly powerful tool for managing and presenting data, and the ability to manipulate embedded images within Excel files can be a game-changer for automating workflows, generating reports, and adding branding elements like logos. However, working with images in Excel programmatically can be tricky—unless you’re using the right tools.
In this guide, we’ll explore how to manipulate embedded images in Excel files using Golang. With the help of the powerful Go package excelize
, we’ll walk through how to add, modify, and automate the embedding of images into Excel workbooks. This can significantly enhance your workflow, whether you're creating dynamic reports or handling large-scale document generation.
For more resources, tips, and tools, be sure to visit UniDoc.
What You'll Learn:
The basics of working with Excel files in Golang
How to add and manipulate embedded images in Excel
Step-by-step implementation with the Go
excelize
libraryTips for automating image management in Excel files
1. Setting Up Your Golang Environment
Before we dive into manipulating images within Excel files, let’s set up the necessary environment.
First, you’ll need to install Golang if it’s not already on your machine. Visit the official Golang website (https://golang.org/dl/) and follow the installation instructions for your operating system.
Next, we need to install the excelize package. Excelize is a popular Go library that allows you to read, write, and manipulate Excel files (XLSX) without relying on Excel itself.
Run the following command in your terminal to install the package:
bashCopygo get github.com/xuri/excelize/v2
With the Golang environment and excelize library set up, you’re ready to start working with Excel files.
2. Understanding Excel Image Embedding
In Excel, images can be embedded in two ways:
Inline images: These are embedded within the cell but don’t affect the cell’s data.
Floating images: These images are placed above the grid and are independent of the cells.
Both types of images can be manipulated, but for this guide, we will focus on embedding and manipulating floating images using the excelize
library.
3. Working with Excelize
a. Creating a New Excel File
Let’s start by creating a simple Excel file and embedding an image into it.
Here’s how to create an Excel file using excelize
and add a floating image:
goCopypackage main
import (
"github.com/xuri/excelize/v2"
"log"
)
func main() {
// Create a new Excel file
file := excelize.NewFile()
// Add an image to the Excel file
err := file.AddPicture("Sheet1", "A1", "path/to/image.png", `{"x_scale": 0.5, "y_scale": 0.5}`)
if err != nil {
log.Fatal(err)
}
// Save the file
err = file.SaveAs("example.xlsx")
if err != nil {
log.Fatal(err)
}
}
In this code:
We create a new Excel file using
excelize.NewFile()
.The
AddPicture
function adds an image to the sheet. The image is placed at the top-left corner of cellA1
in Sheet1.The
x_scale
andy_scale
options are used to resize the image.Finally, the
SaveAs
function saves the Excel file with the embedded image.
b. Modifying an Existing Excel File with Images
You can also manipulate an existing Excel file and add or modify embedded images. Let’s open an existing file and replace an existing image.
goCopypackage main
import (
"github.com/xuri/excelize/v2"
"log"
)
func main() {
// Open an existing Excel file
file, err := excelize.OpenFile("existing_file.xlsx")
if err != nil {
log.Fatal(err)
}
// Replace an existing image with a new one
err = file.AddPicture("Sheet1", "B2", "path/to/new-image.png", `{"x_scale": 0.7, "y_scale": 0.7}`)
if err != nil {
log.Fatal(err)
}
// Save the modified file
err = file.Save()
if err != nil {
log.Fatal(err)
}
}
Here, the code opens an existing Excel file and adds a new image at the specified location (B2
cell) with resizing options. After modification, the file is saved with the new image embedded.
4. Manipulating Image Properties
One of the key features when manipulating images in Excel is being able to adjust properties such as position, size, and scale. The AddPicture
function in excelize
allows you to define these properties in the form of a JSON string.
Positioning: You can control the image’s position using the
"x_offset"
and"y_offset"
parameters to adjust its placement relative to the cell.Scaling: The
"x_scale"
and"y_scale"
parameters adjust the image’s width and height as a percentage of the original size.Rotation: If you want to rotate the image, you can use the
"rotation"
parameter.
Here’s an example of applying these properties:
goCopyerr := file.AddPicture("Sheet1", "A1", "path/to/image.png", `{
"x_offset": 10,
"y_offset": 20,
"x_scale": 0.5,
"y_scale": 0.5,
"rotation": 45
}`)
This will embed the image at cell A1
with a 10-pixel horizontal offset, a 20-pixel vertical offset, and rotate it by 45 degrees.
5. Automating Image Handling in Excel Reports
Now that you know how to manipulate images in Excel using Go, let’s take it a step further and automate the image handling process. This can be useful for scenarios like generating monthly reports with dynamically embedded company logos or charts.
For example, you can use Go to automate the embedding of logos based on data conditions. If you're generating a report for a specific company, you can load the company’s logo from the disk and place it at the top of the sheet.
goCopyfunc addCompanyLogo(file *excelize.File, companyName string) error {
logoPath := "logos/" + companyName + ".png"
if err := file.AddPicture("Sheet1", "A1", logoPath, `{"x_scale": 0.4, "y_scale": 0.4}`); err != nil {
return err
}
return nil
}
In this example, depending on the company name, the program dynamically selects the correct logo and embeds it into the Excel report.
6. Tips for Efficient Excel Image Manipulation
Image Optimization: Large images can slow down Excel file processing. Consider optimizing images before embedding them to reduce file size.
Batch Processing: If you need to add multiple images to an Excel file, consider batching the image insertion to reduce processing time.
Error Handling: Implement error handling for situations where an image might not exist or the file path is incorrect.
Conclusion
Manipulating embedded images in Excel using Golang offers significant benefits for automating report generation, dynamic branding, and improving productivity. By using the excelize
package, you can easily embed and modify images in Excel files, whether you are creating new documents or updating existing ones. The flexibility to adjust image properties such as position, scaling, and rotation makes Golang an excellent choice for automating Excel workflows.
By leveraging Golang’s speed and the excelize
library, you can integrate advanced Excel manipulation into your automation workflows with ease.
Subscribe to my newsletter
Read articles from unidoclib directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
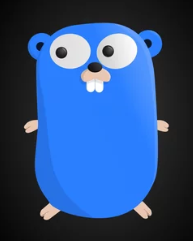
unidoclib
unidoclib
Welcome to our Hashnode profile, where we share our expertise in PDF and office document generation and manipulation in Golang. As a company dedicated to creating and publishing content in this domain, we are passionate about utilizing the power of Golang to streamline document handling and enhance user experiences. Our blogs and articles aim to simplify the complexities of PDF and office document manipulation, making them accessible and beneficial for our audience. Whether you're a developer seeking to integrate powerful document generation capabilities into your projects or an enthusiast interested in learning more about Golang's potential, we've got you covered. Join us on this exciting journey as we explore the advanced features of the Golang PDF library, uncovering its hidden gems to create stunning and functional documents effortlessly. From rendering text and images to seamless page manipulation and implementing advanced features like watermarking and digital signatures, we cover various topics to cater to multiple interests. As an organization that values collaboration and growth, we encourage you to connect with us and share your thoughts, ideas, and questions. Let's foster a vibrant community where we can learn from one another and push the boundaries of document handling in Golang. Stay tuned for regular updates as we continue to provide valuable insights, tips, and practical examples, empowering you to harness the full potential of Golang for efficient document generation and manipulation. Thank you for being a part of our journey, and we look forward to embarking on this exciting adventure together!