Using @Formula in Hibernate: A Comprehensive Guide with Best Practices, Code Examples, and Results
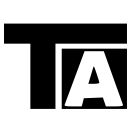
5 min read
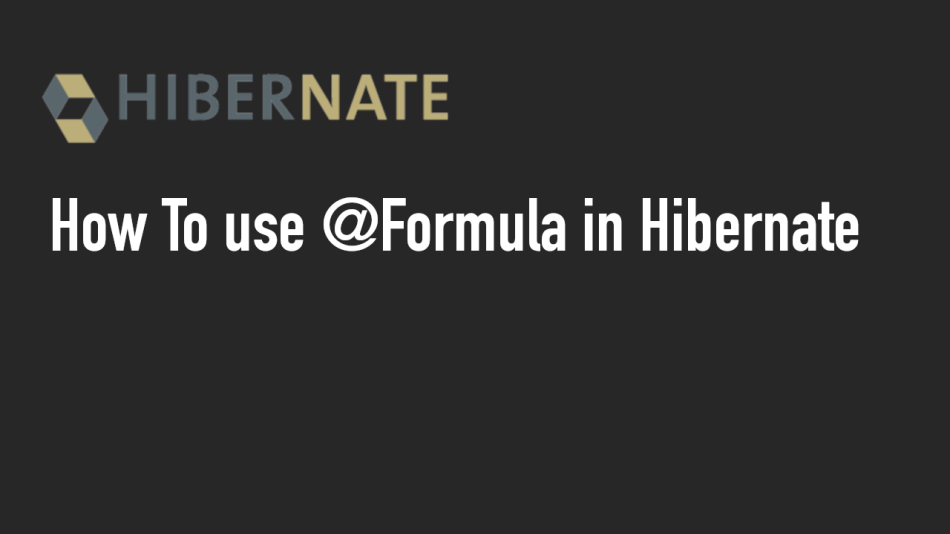
Source: Using @Formula in Hibernate: A Comprehensive Guide with Best Practices, Code Examples, and Results
1. What is @Formula in Hibernate?
1.1 Calculating Values on the Fly
The @Formula annotation in Hibernate allows you to map a calculated field in an entity. Instead of directly mapping a database column to a Java field, @Formula lets you define a SQL expression that Hibernate will use to fetch the field’s value. This is particularly useful for read-only fields where the value depends on other columns or tables.
For instance, if you have an Employee entity and you want to calculate the employee’s full name from the first_name and last_name columns, you can use @Formula to do this without storing the full name in the database.
@Entity
public class Employee {
@Id
private Long id;
private String firstName;
private String lastName;
@Formula("concat(first_name, ' ', last_name)")
private String fullName;
// getters and setters
}
1.2 How It Works
Hibernate injects the SQL defined in the @Formula directly into the SELECT statement when querying the entity. The expression in the @Formula is evaluated each time the entity is fetched. This makes it perfect for fields that are calculated based on other columns or even data from related tables.
In the example above, whenever an Employee is loaded, Hibernate executes the SQL SELECT concat(first_name, ' ', last_name) AS full_name FROM employee to calculate the fullName field.
1.3 Read-Only Nature
A key aspect to remember about @Formula is that it’s a read-only feature. You can calculate a value and have it mapped to your entity, but you cannot modify this field and expect Hibernate to save the changes back to the database. This is important for performance optimization since the field is recalculated each time it's queried.
2. Practical Use Cases for @Formula
2.1 Calculating Aggregated Values
Let’s consider a more complex example where you need to calculate the total price of an order from multiple order lines. Using @Formula, you can create a calculated field that sums up the prices of all the order lines.
@Entity
public class Order {
@Id
private Long id;
private String customerName;
@OneToMany(mappedBy = "order")
private List<OrderLine> orderLines;
@Formula("(select sum(ol.price * ol.quantity) from order_line ol where ol.order_id = id)")
private Double totalPrice;
// getters and setters
}
In this case, @Formula runs a subquery to calculate the total price from the OrderLine entity related to the Order entity. This way, you avoid storing a redundant total price in the database, and it gets recalculated every time the order is fetched.
2.2 Combining Data from Multiple Tables
You can use @Formula to fetch and combine data from multiple related tables. Suppose you want to calculate a score for an employee based on data in several other related entities, like PerformanceReview and Training. With @Formula, you can define a complex SQL expression that combines this data into a single field.
@Entity
public class Employee {
@Id
private Long id;
private String firstName;
@Formula("(select avg(pr.score) from performance_review pr where pr.employee_id = id)")
private Double averageReviewScore;
@Formula("(select count(t.id) from training t where t.employee_id = id)")
private Integer totalTrainingsCompleted;
// getters and setters
}
2.3 Best Practices for Using @Formula
Optimize Your SQL Expression: Since @Formula gets executed every time the entity is loaded, it’s important to ensure that the SQL expression is optimized. Complex subqueries can have performance impacts, so you should use them cautiously.
Use in Read-Only Scenarios: Since the field annotated with @Formula is read-only, use it for derived fields that do not need to be updated directly. For write scenarios, consider mapping directly to a column or use @Transient if you want to manage calculations entirely in Java.
Combine with Lazy Loading: If your formula depends on complex queries or relationships, consider using lazy loading strategies to avoid unnecessary performance hits when you don’t need the calculated field.
Limitations with Sorting: Be careful when using @Formula in queries where sorting is involved. If you try to sort by a field annotated with @Formula, it can have a negative impact on performance because the sorting will happen at the database level based on the SQL expression.
2.4 Demo Results
Let’s see the results of applying @Formula in a real-world example. Assume we have an Order entity with several OrderLine entries. Here’s how the SQL query will look when we fetch an order along with its total price.
SELECT o.id, o.customer_name,
(SELECT SUM(ol.price * ol.quantity) FROM order_line ol WHERE ol.order_id = o.id) AS total_price
FROM orders o
WHERE o.id = 1;
The resulting output would be something like this:
{
"id": 1,
"customerName": "John Doe",
"totalPrice": 150.75
}
As you can see, the totalPrice is calculated dynamically based on the current state of the OrderLine table.
3. Conclusion
In this article, we’ve explored the @Formula annotation in Hibernate, looking at how it can be used to calculate values dynamically in your entities. From simple concatenations to complex SQL expressions, @Formula is a powerful tool that can enhance the flexibility of your Hibernate mappings. However, like any powerful tool, it should be used carefully to avoid performance issues. By following the best practices outlined here, you can use @Formula to simplify your entity mappings and keep your database schema clean.
If you have any questions about @Formula or Hibernate, feel free to drop a comment below!
Read more at : Using @Formula in Hibernate: A Comprehensive Guide with Best Practices, Code Examples, and Results
0
Subscribe to my newsletter
Read articles from Tuanhdotnet directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
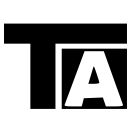
Tuanhdotnet
Tuanhdotnet
I am Tuanh.net. As of 2024, I have accumulated 8 years of experience in backend programming. I am delighted to connect and share my knowledge with everyone.