#NoStupidQuestion: How to find Jira custom_field IDs using curl?
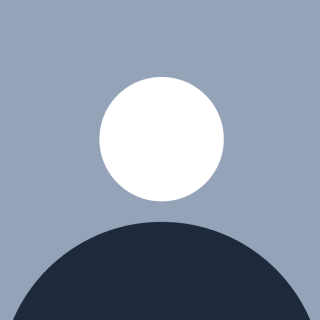

Use Case:
When we’re building forms that connect to a Jira project or board, we’ll often need to send data to Jira through its API. But Jira uses internal field IDs (like customfield_10057) — not the nice field names we see in the UI.
So before we can push our form data to Jira, we need to know:
- For example: “What’s the custom field ID for ‘Summary’, ‘Reporter’, or ‘Description’?”
Because once we know the custom field IDs, we can create a form that automatically pushes the data to Jira via the API.
Here’s how to find them the easy way!
Get a Jira API Token
Before we can talk to Jira using code or the command line, we need to authenticate.
Jira Cloud uses an API token instead of a password.
we can generate one like this:
Go to:https://id.atlassian.com/manage-profile/security/api-tokens
1. Click “Create API token”
2. Give it a name (e.g. "automation-form")
3. Click Create, and copy the token (we won’t see it again!)
Test Our Credentials
Let’s test that our email + API token works by asking Jira who we are:
curl -u your_email@example.com:your_api_token \
-X GET "https://yourcompany.atlassian.net/rest/api/3/myself"
If it works, we’ll get a JSON response showing our Jira account.
If not, double-check:
• Your email is the one tied to your Atlassian account
• Your API token is correct
• There are no spaces in the token or wrong quote marks
Fetch Custom Field IDs for a Jira Issue Type
Now the fun part — let’s ask Jira:
“What are all the fields (and their IDs) for this specific issue type in this specific project?”
Use this curl command below (replace with your email/token):
curl -s -u your_email@example.com:your_api_token \
-X GET "https://yourcompany.atlassian.net/rest/api/3/issue/createmeta?projectKeys=MYPROJECT&issuetypeIds=10001&expand=projects.issuetypes.fields" \
| jq .
What’s Happening Here?
Let’s break it down:
Part | What It Does |
-s | Silences progress output (makes it cleaner) |
-u | Your login info: email and API token |
-X GET | We’re doing a GET request (we’re asking Jira for info) |
URL | We’re asking Jira for the field metadata for the MYPROJECT project and the issue type with ID 10001 |
expand=... | Tells Jira to also include detailed field data |
jq . | Pretty-prints the JSON using jq (a JSON viewer tool) |
We’ll get something like this:
{
"customfield_10057": {
"name": "Summary",
"required": true,
"schema": {
"type": "string"
}
},
"customfield_10058": {
"name": "Description",
"required": false,
"schema": {
"type": "option"
}
}
}
What We’ll See:
• customfield_10057 and customfield_10058 are the custom field IDs.
• name tells us the human-readable name of the field (e.g., "Idea Summary").
• required tells us whether this field is required when submitting a Jira issue.
• schema gives us the field’s type (e.g., string, option).
📝 Example Field Mapping:
• customfield_10057 → "Summary" (a required text field).
• customfield_10058 → "Description" (an optional dropdown field).
These are the IDs we’ll use to submit data to Jira programmatically.
🛟 Extra Tips
• If we don’t know our issue type ID (10001), we can use this to list them:
curl -s -u your_email@example.com:your_api_token \
-X GET "https://yourcompany.atlassian.net/rest/api/3/issue/createmeta?projectKeys=MYPROJECT" \
| jq '.projects[0].issuetypes | map({id, name})'
• Don’t have jq installed? Install it with:
brew install jq # macOS
sudo apt install jq # Ubuntu
choco install jq # Windows (Chocolatey)
Happy coding!
Subscribe to my newsletter
Read articles from anj directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by