๐ Python List Cheat Sheet
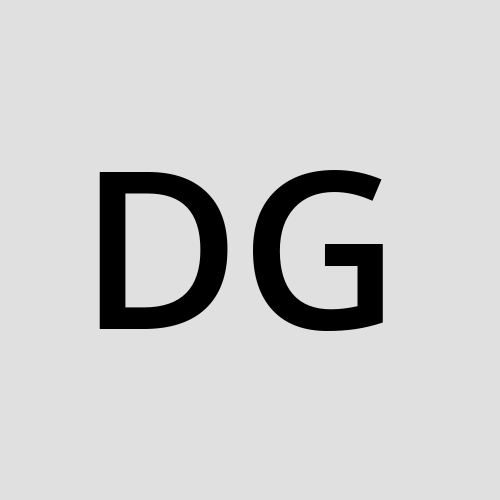

โ Creating Lists
my_list = [1, 2, 3, 4]
empty_list = []
mixed_list = [1, "hello", 3.14, True]
โ Adding Elements
my_list.append(5) # Add to end
my_list.insert(2, 99) # Insert at index 2
my_list.extend([6, 7]) # Add multiple elements
โ Removing Elements
my_list.remove(3) # Remove first occurrence of value
my_list.pop() # Remove last item
my_list.pop(1) # Remove item at index 1
del my_list[0] # Delete item at index 0
my_list.clear() # Remove all items
๐ Accessing Items
my_list[0] # First item
my_list[-1] # Last item
my_list[1:3] # Slice (index 1 to 2)
๐ Looping Through Lists
for item in my_list:
print(item)
for index, item in enumerate(my_list):
print(index, item)
๐ Searching / Checking
3 in my_list # Check existence
my_list.index(3) # Index of value
my_list.count(3) # Count occurrences
๐งน Sorting and Reversing
my_list.sort() # Sort in place
my_list.sort(reverse=True) # Descending order
sorted_list = sorted(my_list) # Return a sorted copy
my_list.reverse() # Reverse list
๐ Useful Functions
len(my_list) # Length
min(my_list) # Minimum value
max(my_list) # Maximum value
sum(my_list) # Sum of elements
๐งฑ List Comprehension
squares = [x**2 for x in range(5)] # [0, 1, 4, 9, 16]
evens = [x for x in my_list if x % 2 == 0]
๐ Joining & Splitting
joined = " ".join(["Hello", "World"]) # "Hello World"
split_list = "a,b,c".split(",") # ['a', 'b', 'c']
๐งช Copying Lists
new_list = my_list.copy()
new_list = my_list[:] # Another way to copy
Subscribe to my newsletter
Read articles from David Gostin directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
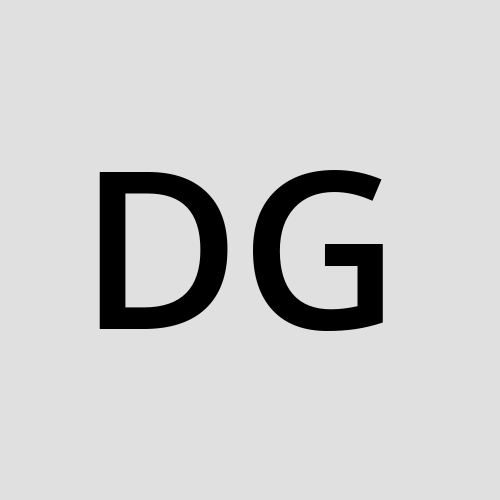
David Gostin
David Gostin
Full-Stack Web Developer with over 25 years of professional experience. I have experience in database development using Oracle, MySQL, and PostgreSQL. I have extensive experience with API and SQL development using PHP and associated frameworks. I am skilled with git/github and CI/CD. I have a good understanding of performance optimization from the server and OS level up to the application and database level. I am skilled with Linux setup, configuration, networking and command line scripting. My frontend experience includes: HTML, CSS, Sass, JavaScript, jQuery, React, Bootstrap and Tailwind CSS. I also have experience with Amazon EC2, RDS and S3.