Imagine This: You’re Scrolling Through Instagram...
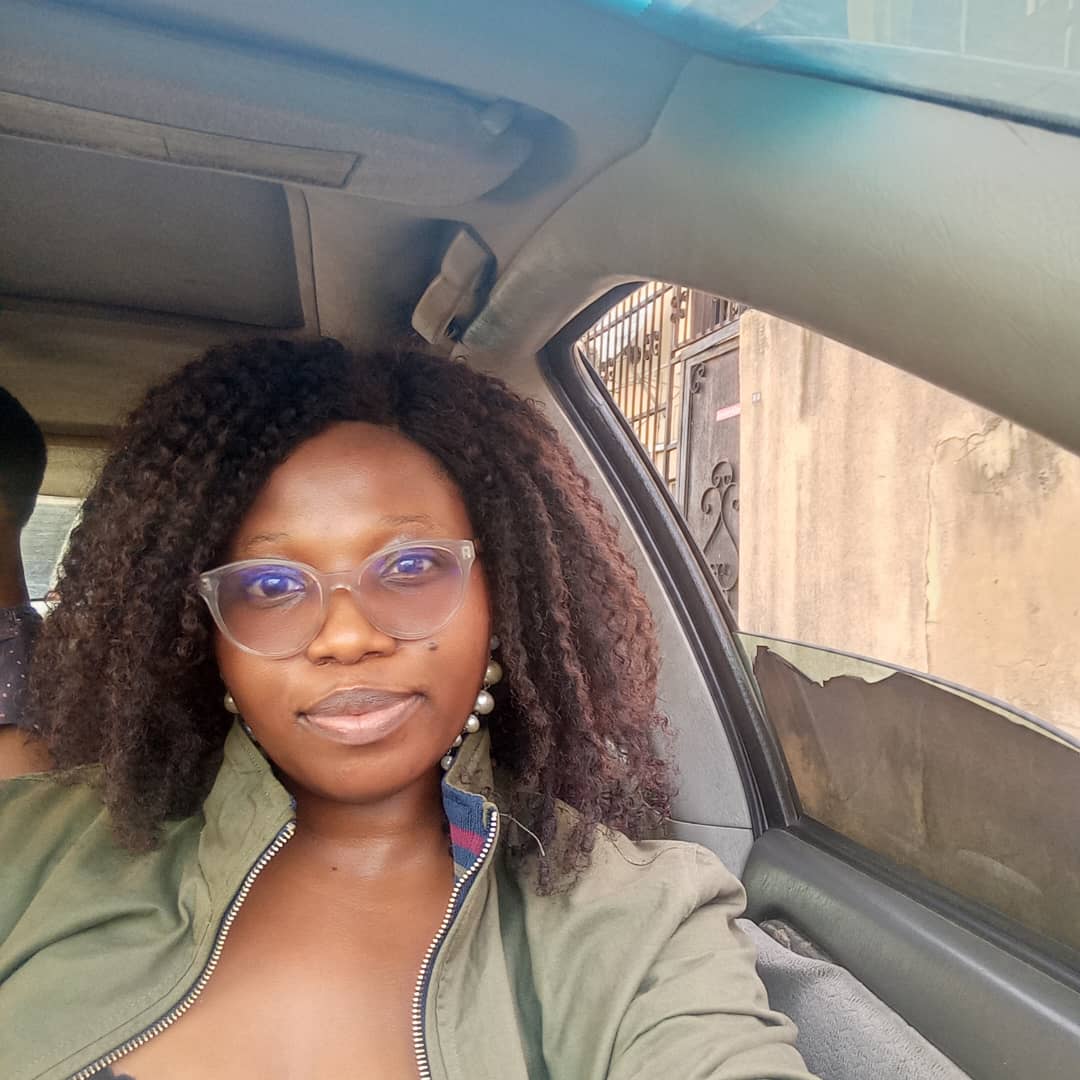

You stumble upon a captivating video that pulls you in. As you scroll back up, you notice a “SEEN” notification.
That feeling of connection? Now, imagine replicating that excitement in your coding journey! Today, I’m inviting you to build a simple visitor counter using Node.js and Redis—let's dive into a fun and interactive project!
Why Node.js is a Game-Changer
Node.js is like unlocking a new level in your coding adventure. It empowers you to use JavaScript on the server side, which means you can create fast, scalable applications without switching between languages. If you’re familiar with JavaScript from front-end development, Node.js is a seamless transition that expands your capabilities.
Meet Redis: Your Speedy Data Companion
Redis is an in-memory data structure store that’s known for its lightning-fast performance. Picture it as a super-efficient filing cabinet that helps you manage and retrieve information quickly. In this project, we’ll use Redis to track how many times our page has been visited, adding an interactive layer to our application.
Project Overview: Let’s Build Together!
In this hands-on tutorial, we’ll set up a Node.js server that connects to a Redis database. Each time someone visits our page, we’ll increment the visit count. This project is not only straightforward but also a fantastic way to solidify your understanding of backend development.
Setting Up Your Environment
Step 1: Install Redis
To kick things off, we’ll use Docker to set up Redis. If you don’t have Docker installed yet, head over to [Docker's official site] and download the version suitable for your system.
Once Docker is ready, run this command in your terminal to create a Redis container:
docker run --name redis -p 6379:6379 -d redis
Step 2: Set Up Your Node.js Project
Create a project directory:
mkdir redis-visitor-counter cd redis-visitor-counter
Initialize a new npm project:
npm init -y
Install necessary packages:
npm install express redis
Step 3: Build the Server
Create a file named app.js
and add the following code:
const express = require('express');
const redis = require('redis');
const app = express();
const client = redis.createClient();
client.connect()
.then(() => console.log("Connected to Redis"))
.catch(err => console.error("Error connecting to Redis:", err));
// Initialize visit count
client.set('visits', 0);
// Define the route
app.get('/', async (req, res) => {
try {
const visits = await client.get('visits');
const updatedVisits = parseInt(visits) + 1;
await client.set('visits', updatedVisits);
res.send(`This page has been visited ${updatedVisits} times.`);
} catch (err) {
console.error("Error interacting with Redis:", err);
res.status(500).send("Internal Server Error");
}
});
const PORT = 3000;
app.listen(PORT, () => {
console.log(`Server is running on http://localhost:${PORT}`);
});
Step 4: Run Your Application
To run your application, use the following command in your terminal:
node app.js
You should see confirmation that the server is running and connected to Redis:
Connected to Redis
Server is running on http://localhost:3000
Conclusion: Celebrate Your Achievement!
And there you have it! 🎉 You’ve just built a visitor counter using Node.js and Redis. This project is not only a great way to reinforce your backend development skills but also creates something tangible and interactive.
As you continue your coding journey, remember that hands-on projects like this can be both fun and educational. If you found this article helpful, consider following me for more beginner-friendly projects and insights into the tech world. Let’s grow together!
🔗 Check out my GitHub for more projects: [Arbythecoder's GitHub]
Subscribe to my newsletter
Read articles from Abigeal Afolabi directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
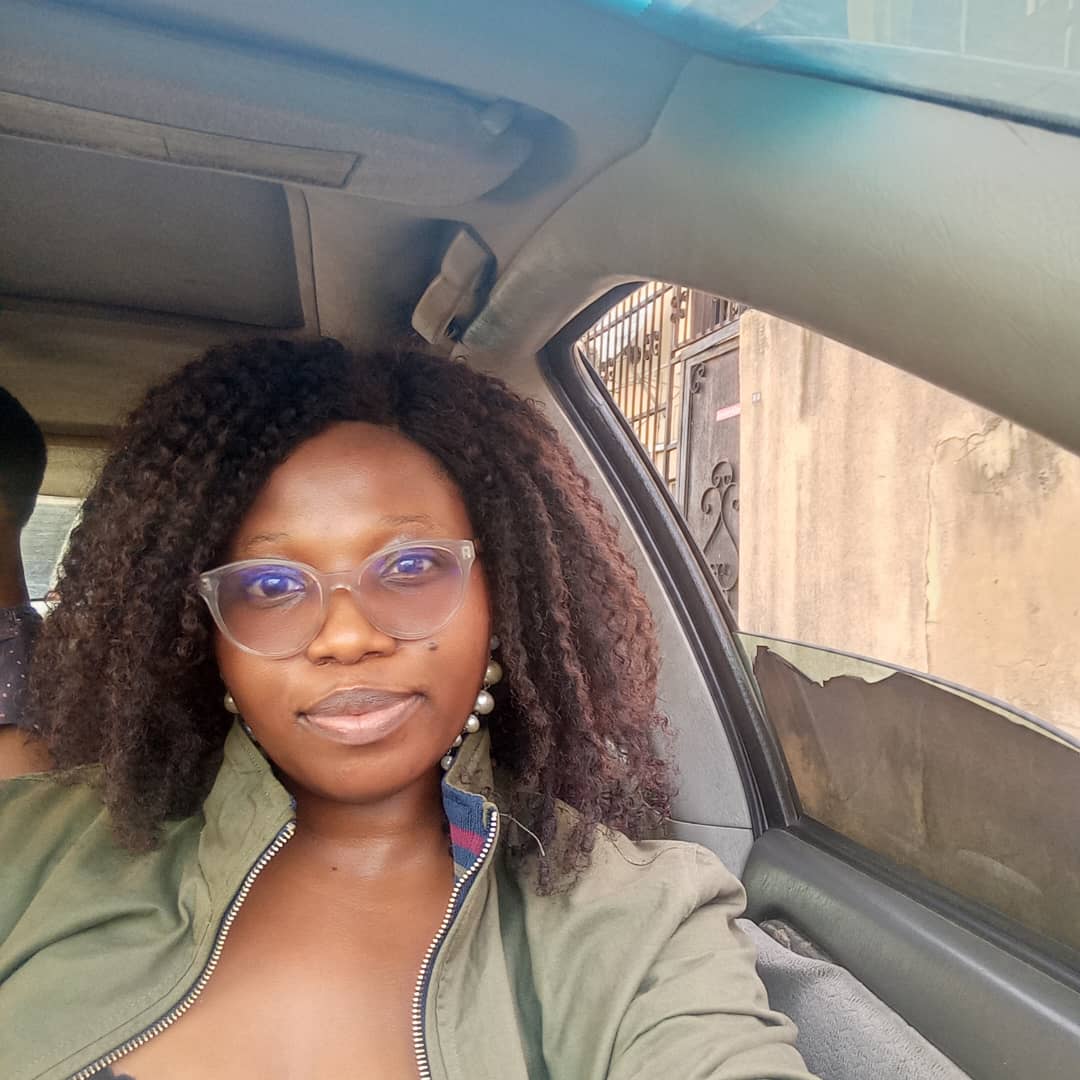
Abigeal Afolabi
Abigeal Afolabi
🚀 Software Engineer by day, SRE magician by night! ✨ Tech enthusiast with an insatiable curiosity for data. 📝 Harvard CS50 Undergrad igniting my passion for code. Currently delving into the MERN stack – because who doesn't love crafting seamless experiences from front to back? Join me on this exhilarating journey of embracing technology, penning insightful tech chronicles, and unraveling the mysteries of data! 🔍🔧 Let's build, let's write, let's explore – all aboard the tech express! 🚂🌟 #CodeAndCuriosity