What is Difference Between Local Storage and Session Storage in JavaScript?
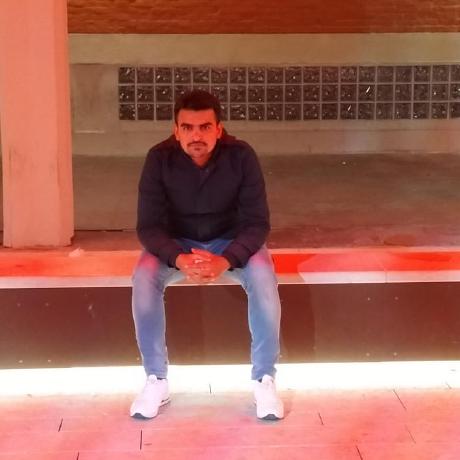

When developing modern web applications, efficient data management on the client-side plays a critical role in providing a seamless user experience. Two widely-used browser storage mechanisms in JavaScript—Local Storage and Session Storage—offer developers a way to store and retrieve key-value data. While they share similarities, they also have distinct differences that make them suitable for specific use cases.
Let’s delve into the differences between Local Storage and Session Storage, understand their APIs, and explore examples and practical use cases.
What is Local Storage?
Local Storage is a persistent storage mechanism that allows web applications to store data with no expiration time. This means that data saved in Local Storage remains available even after the browser is closed and reopened.
Key Characteristics:
Persistence: Data does not expire unless explicitly removed.
Scope: Data is shared across all tabs and windows of the same browser for a specific origin.
Capacity: Can store up to 5MB (varies slightly between browsers).
Example of Local Storage:
Here’s how you can use Local Storage to save and retrieve data:
// Save data to Local Storage
localStorage.setItem('username', 'JohnDoe');
// Retrieve data from Local Storage
const username = localStorage.getItem('username');
console.log(username); // Outputs: JohnDoe
// Remove data from Local Storage
localStorage.removeItem('username');
// Clear all data from Local Storage
localStorage.clear();
Use Case for Local Storage:
Local Storage is ideal for storing non-sensitive data that needs to persist across browser sessions. For example:
User preferences (e.g., theme or language settings)
Persistent form data for drafts
Caching non-sensitive data for offline availability
What is Session Storage?
Session Storage is a temporary storage mechanism that keeps data for the duration of the page session. Once the browser tab or window is closed, the data is automatically cleared.
Key Characteristics:
Persistence: Data is only available for the current session.
Scope: Data is isolated to the specific tab or window it was created in.
Capacity: Similar to Local Storage, typically 5MB.
Example of Session Storage:
Here’s how you can use Session Storage to save and retrieve data:
// Save data to Session Storage
sessionStorage.setItem('sessionId', 'abc123');
// Retrieve data from Session Storage
const sessionId = sessionStorage.getItem('sessionId');
console.log(sessionId); // Outputs: abc123
// Remove data from Session Storage
sessionStorage.removeItem('sessionId');
// Clear all data from Session Storage
sessionStorage.clear();
Use Case for Session Storage:
Session Storage is suitable for storing data that is only needed for the duration of the user’s session. For example:
Temporary state management for a single page application (SPA)
Data for a multi-step form that resets when the tab is closed
Session-specific tokens or flags
Key Differences Between Local Storage and Session Storage
Feature | Local Storage | Session Storage |
Persistence | Data persists until explicitly removed | Data is cleared when the tab is closed |
Scope | Shared across all tabs and windows | Specific to the current tab or window |
Capacity | Approximately 5MB | Approximately 5MB |
Use Case | Long-term storage for persistent data | Short-term storage for session data |
Considerations When Using Local Storage and Session Storage
Security: Neither Local Storage nor Session Storage is designed to store sensitive information like passwords or session tokens, as they can be accessed via JavaScript. For sensitive data, use HTTP-only cookies with secure flags.
Size Limitations: Both have limited storage capacity. For larger datasets, consider using IndexedDB or Web SQL.
Data Sharing: Local Storage data is shared across tabs, which may lead to unintended consequences in multi-tab applications.
Conclusion
Local Storage and Session Storage are essential tools in a web developer’s arsenal. By understanding their differences and use cases, you can make informed decisions to enhance the functionality and performance of your applications.
Use Local Storage when data needs to persist across sessions, such as user preferences or non-sensitive cache.
Opt for Session Storage when dealing with temporary data required only for the duration of a session.
Both storage mechanisms are easy to use and can significantly improve user experience when implemented correctly. Always remember to consider security implications and choose the right storage option based on the nature of your data.
Happy coding!
Subscribe to my newsletter
Read articles from Ahmed Raza directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
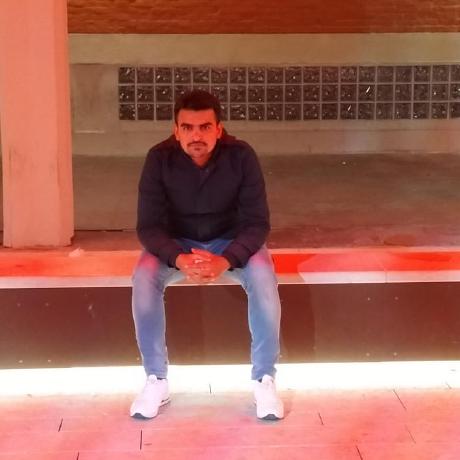
Ahmed Raza
Ahmed Raza
Ahmed Raza is a versatile full-stack developer with extensive experience in building APIs through both REST and GraphQL. Skilled in Golang, he uses gqlgen to create optimized GraphQL APIs, alongside Redis for effective caching and data management. Ahmed is proficient in a wide range of technologies, including YAML, SQL, and MongoDB for data handling, as well as JavaScript, HTML, and CSS for front-end development. His technical toolkit also includes Node.js, React, Java, C, and C++, enabling him to develop comprehensive, scalable applications. Ahmed's well-rounded expertise allows him to craft high-performance solutions that address diverse and complex application needs.