๐ข๏ธSQLAlchemy Cheat Sheet
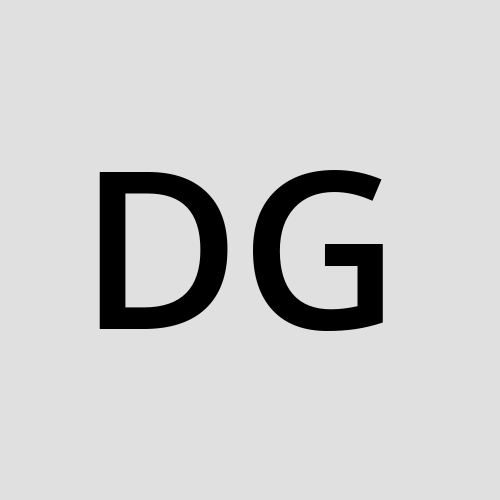

pip install sqlalchemy
Optional for SQLite (included by default) or install a specific database driver:
pip install psycopg2 # for PostgreSQL
pip install pymysql # for MySQL
๐๏ธ Define Models (ORM Style)
from sqlalchemy import Column, Integer, String
from sqlalchemy.ext.declarative import declarative_base
Base = declarative_base()
class User(Base):
__tablename__ = "users"
id = Column(Integer, primary_key=True)
name = Column(String)
email = Column(String)
๐ Connect to Database
from sqlalchemy import create_engine
engine = create_engine("sqlite:///mydb.db") # SQLite example
๐ ๏ธ Create Tables
Base.metadata.create_all(engine)
๐งต Create a Session
from sqlalchemy.orm import sessionmaker
Session = sessionmaker(bind=engine)
session = Session()
โ Add Records
new_user = User(name="Alice", email="alice@example.com")
session.add(new_user)
session.commit()
๐ Query Records
# Get all users
users = session.query(User).all()
# Get one user by filter
user = session.query(User).filter_by(name="Alice").first()
# Get by primary key
user = session.get(User, 1)
โ๏ธ Update Records
user = session.query(User).filter_by(name="Alice").first()
user.email = "newalice@example.com"
session.commit()
โ Delete Records
session.delete(user)
session.commit()
๐ Relationships
from sqlalchemy import ForeignKey
from sqlalchemy.orm import relationship
class Post(Base):
__tablename__ = "posts"
id = Column(Integer, primary_key=True)
title = Column(String)
user_id = Column(Integer, ForeignKey("users.id"))
user = relationship("User", back_populates="posts")
User.posts = relationship("Post", back_populates="user")
โ๏ธ Common Column Types
from sqlalchemy import Integer, String, Float, Boolean, Date, DateTime, Text
๐ Useful Tips
session.rollback()
โ Undo changes if something goes wrongsession.close()
โ Clean up sessionBase.metadata.drop_all(engine)
โ Drop all tables
Subscribe to my newsletter
Read articles from David Gostin directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
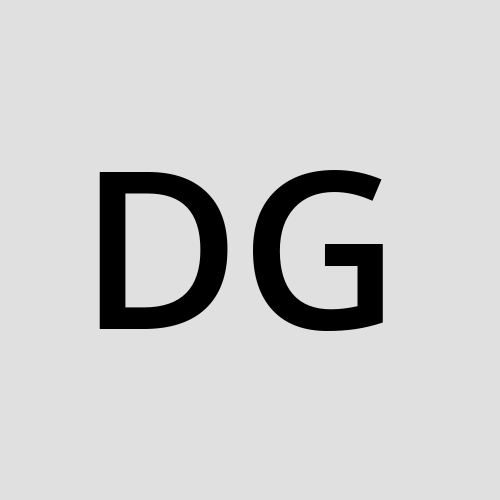
David Gostin
David Gostin
Full-Stack Web Developer with over 25 years of professional experience. I have experience in database development using Oracle, MySQL, and PostgreSQL. I have extensive experience with API and SQL development using PHP and associated frameworks. I am skilled with git/github and CI/CD. I have a good understanding of performance optimization from the server and OS level up to the application and database level. I am skilled with Linux setup, configuration, networking and command line scripting. My frontend experience includes: HTML, CSS, Sass, JavaScript, jQuery, React, Bootstrap and Tailwind CSS. I also have experience with Amazon EC2, RDS and S3.