Understanding Route Awareness in Flutter: Leveraging the RouteAware Class
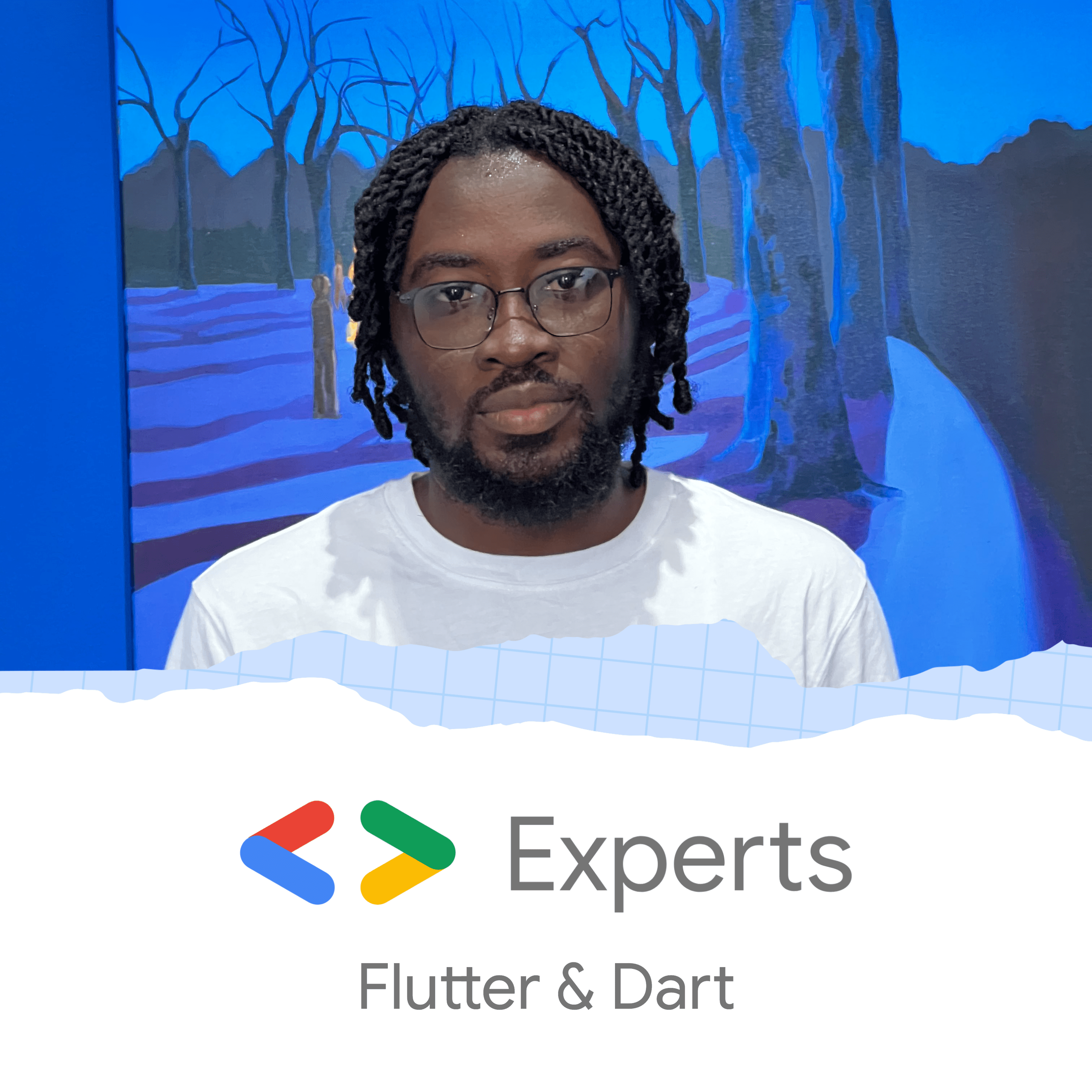

In Flutter development, managing navigation and understanding a widget’s visibility within the navigation stack is crucial for building dynamic and responsive user interfaces. The RouteAware
class in the Flutter widgets library provides a mechanism for objects to be notified of changes to the Navigator's route history. This article delves into the purpose and functionality of the RouteAware
class, drawing directly from the official Dart API documentation.
What is RouteAware?
The RouteAware
class is defined as an abstract mixin that serves as an interface for objects that need to be aware of their current Route
. It is specifically designed to be used in conjunction with RouteObserver
, a class that listens to route changes in a Navigator
. By mixing in RouteAware
into a widget and registering it with a RouteObserver
, that widget can receive callbacks whenever its associated route's visibility changes.
Key Features and Functionality:
The RouteAware
class provides several methods that are called at different points in a route's lifecycle:
didPop()
: This method is invoked when the current route has been popped off the navigation stack. This signifies that the route is no longer visible, and the user has navigated back to the previous screen.didPopNext()
: This method is called when the route above the current route has been popped off, and consequently, the current route becomes visible again. This is useful for scenarios where a widget needs to react when it reappears after another route is dismissed.didPush()
: This method is triggered when the current route has been pushed onto the navigation stack. This indicates that the route has become the top-most route and is now visible to the user.didPushNext()
: This method is invoked when a new route has been pushed on top of the current route, meaning the current route is no longer visible. Widgets using this callback can perform actions when they are obscured by a new route.
Other Members:
Besides these lifecycle methods, the RouteAware
class also includes the following inherited members:
hashCode
: This property provides the hash code for theRouteAware
object.runtimeType
: This property gives a representation of the runtime type of the object.noSuchMethod(Invocation invocation)
: This inherited method is invoked when a nonexistent method or property is accessed.toString()
: This inherited method returns a string representation of theRouteAware
object.operator ==(Object other)
: This inherited operator defines how equality is checked forRouteAware
objects.
Example:
import 'package:flutter/material.dart';
// Step 1: Create your route observer
final RouteObserver<PageRoute> routeObserver = RouteObserver<PageRoute>();
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
// Step 2: Register the route observer in MaterialApp
navigatorObservers: [routeObserver],
home: HomePage(),
routes: {
'/details': (context) => DetailsPage(),
},
);
}
}
class HomePage extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(title: Text('Home Page')),
body: Center(
child: ElevatedButton(
child: Text('Go to Details'),
onPressed: () {
Navigator.of(context).pushNamed('/details');
},
),
),
);
}
}
// Step 3: Create a state class that implements RouteAware
class DetailsPage extends StatefulWidget {
@override
_DetailsPageState createState() => _DetailsPageState();
}
class _DetailsPageState extends State<DetailsPage> with RouteAware {
@override
void didChangeDependencies() {
super.didChangeDependencies();
// Step 4: Subscribe to the route observer
routeObserver.subscribe(this, ModalRoute.of(context)!);
}
@override
void dispose() {
// Step 5: Unsubscribe from the route observer
routeObserver.unsubscribe(this);
super.dispose();
}
// Step 6: Implement RouteAware callbacks
@override
void didPush() {
// Called when this route is pushed onto navigator and page appears
print('Details page didPush: page is now visible');
// You could start animations, fetch data, etc.
}
@override
void didPopNext() {
// Called when the route on top of this one is popped
print('Details page didPopNext: returning to this page');
// You could refresh data, restart animations, etc.
}
@override
void didPushNext() {
// Called when a new route is pushed on top of this one
print('Details page didPushNext: another page is now visible');
// You could pause animations, save state, etc.
}
@override
void didPop() {
// Called when this route is popped off the navigator
print('Details page didPop: this page is being removed');
// You could save user data, stop services, etc.
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(title: Text('Details Page')),
body: Center(
child: Text('This is a RouteAware page'),
),
);
}
}
In Summary:
The RouteAware
class is a powerful tool in Flutter for creating widgets that are sensitive to navigation events. By implementing the RouteAware
interface and working with a RouteObserver
, developers can build more sophisticated UIs that react intelligently to route transitions, such as pausing animations, releasing resources, or updating their state based on their visibility within the application's navigation flow. The methods provided by RouteAware
offer precise callbacks for various stages of a route's lifecycle, enabling fine-grained control over a widget's behavior in response to navigation changes.
Originally posted on medium.com
And that’s all for now. If you have any specific topic you would like me to write on, leave your suggestions in the comment section, and also if you need any clarifications on this topic, do well to reach out to me on X(Twitter) @_iamEtornam.
Subscribe to my newsletter
Read articles from Bright Etornam Sunu directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
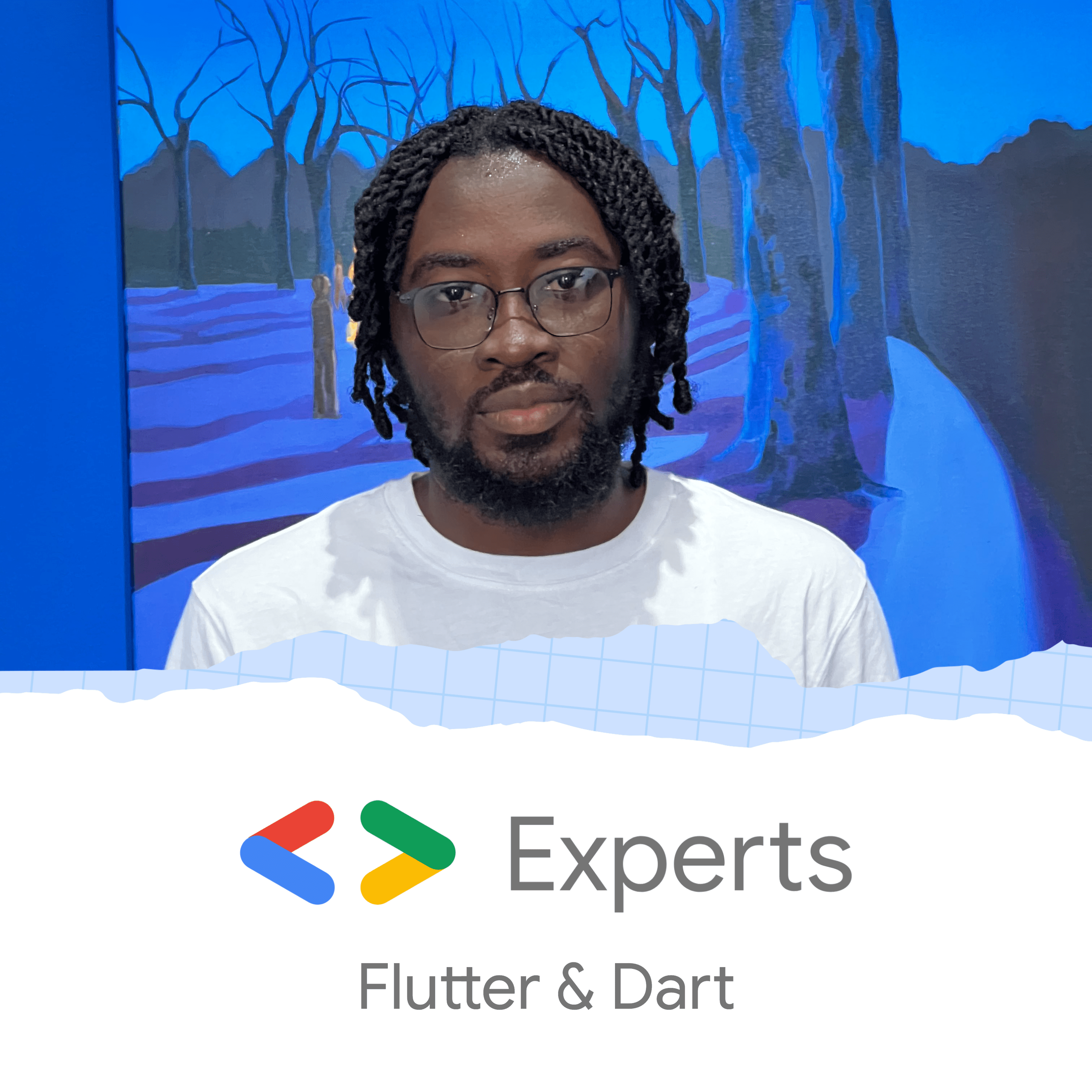
Bright Etornam Sunu
Bright Etornam Sunu
Etornam is a Software Engineer and a Google Developer Expert(GDE) who specialises in developing resilient Mobile applications for android & iOS and web services. He understands modern software engineering and is always looking for a better approach to designing software. Aside from his day-to-day activities as a software engineer, he actively contributes to open-source projects and co-organises at Flutter Africa.