CI/CD Pipeline for a Node.js App: Automated Testing and Deployment to Render
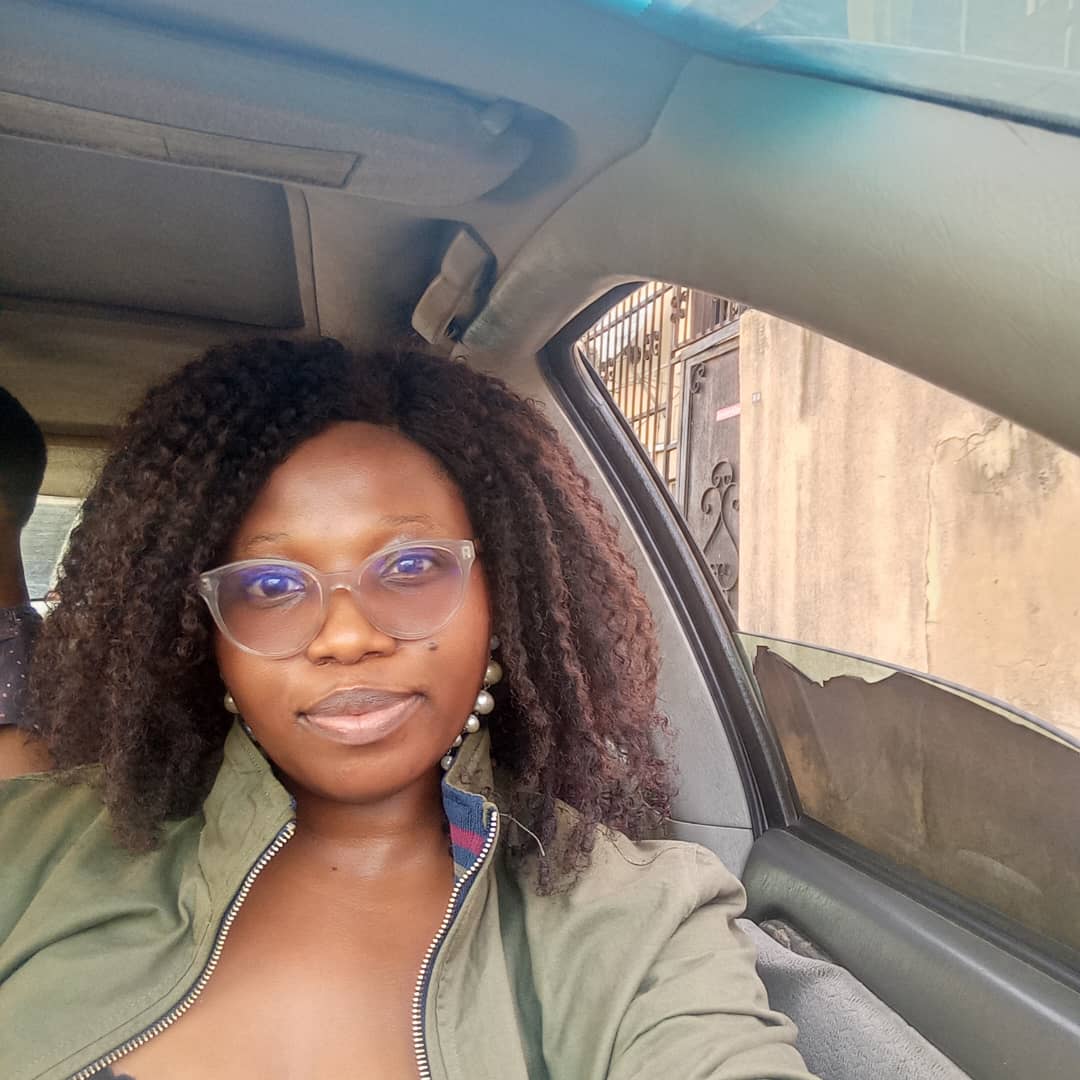

Introduction
Are you looking to streamline your development process? Setting up a CI/CD pipeline can be a game-changer, especially for Node.js applications. This guide will walk you through creating a beginner-friendly CI/CD pipeline using GitLab to automate testing and deployment for a simple Node.js app. By the end, youβll have a robust DevOps workflow that enables you to ship code faster and safer.
π Folder Structure
Hereβs how your project folder should look:
node-ci-cd-app/
βββ .gitlab-ci.yml # GitLab pipeline config
βββ package.json
βββ .gitignore
βββ app.js # Main server file
βββ routes/
β βββ ping.js # Example route
βββ tests/
β βββ ping.test.js # Test for the ping route
βββ README.md
β Step 1: Create a Simple Node.js App
1. Initialize Your Project
Start by creating your Node.js application:
mkdir node-ci-cd-app && cd node-ci-cd-app
npm init -y
2. Install Necessary Dependencies
Use the following commands to install Express and testing libraries:
npm install express
npm install --save-dev jest supertest
3. Create Essential Files
app.js
This is your main server file:
const express = require('express');
const pingRoute = require('./routes/ping');
const app = express();
app.use('/ping', pingRoute);
const PORT = process.env.PORT || 3000;
if (require.main === module) {
app.listen(PORT, () => console.log(`Server running on port ${PORT}`));
}
module.exports = app;
routes/ping.js
Set up a basic route:
const express = require('express');
const router = express.Router();
router.get('/', (req, res) => {
res.send('pong');
});
module.exports = router;
tests/ping.test.js
Write a test for your route:
const request = require('supertest');
const app = require('../app');
describe('GET /ping', () => {
it('should return pong', async () => {
const res = await request(app).get('/ping');
expect(res.statusCode).toBe(200);
expect(res.text).toBe('pong');
});
});
.gitignore
Create a .gitignore
file to exclude unnecessary files:
node_modules
.env
Update package.json
Modify the scripts section in your package.json
:
"scripts": {
"start": "node app.js",
"test": "jest"
}
You can now run your application:
node app.js # Start the server
npm test # Run tests
π Step 2: Push to GitLab & Set Up CI/CD Pipeline
1. Create a New GitLab Repository
Go to GitLab
Click "New Project" > "Create blank project"
Name your project
node-ci-cd-app
Click "Create Project"
2. Connect Your Local Repository
Run these commands in your terminal:
git init
git remote add origin https://gitlab.com/your-username/node-ci-cd-app.git
git config --global user.name "Your Name"
git config --global user.email "you@example.com"
git add .
git commit -m "Initial commit"
git branch -M main
git push -u origin main
β οΈ If you see the error:
error: src refspec main does not match any
, run:
git branch -M main
Then push again:
git push -u origin main
3. Create .gitlab-ci.yml
Add a CI configuration file in your root directory:
stages:
- test
run_tests:
stage: test
image: node:18
script:
- npm install
- npm test
4. Push to Trigger the Pipeline
Add the CI/CD configuration file:
git add .gitlab-ci.yml
git commit -m "Add CI pipeline for testing"
git push
5. Monitor Your Pipeline on GitLab
Navigate to CI/CD > Pipelines in your GitLab project.
Check the status and logs to ensure everything is functioning properly.
π Step 3: Next Steps β Deploy to Render
In the next part, we will:
Deploy your Node.js app to Render using Deploy Hooks.
Secure secrets with GitLab CI/CD Variables.
Add a
deploy
stage to your pipeline.
Stay tuned for the next installment!
This guide provides a comprehensive approach to setting up a CI/CD pipeline for your Node.js application. If you have questions or need further assistance, feel free to reach out in the comments!
Subscribe to my newsletter
Read articles from Abigeal Afolabi directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
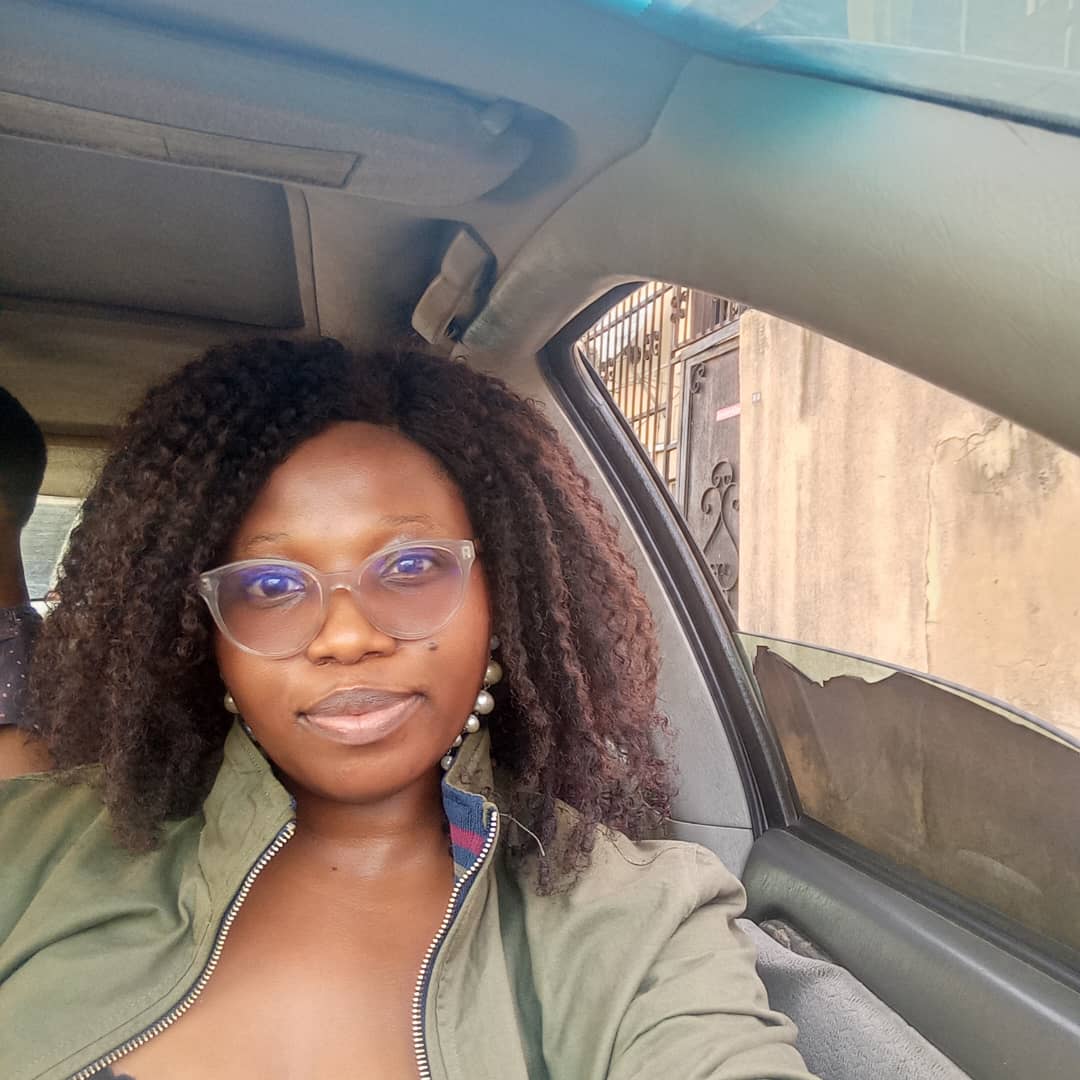
Abigeal Afolabi
Abigeal Afolabi
π Software Engineer by day, SRE magician by night! β¨ Tech enthusiast with an insatiable curiosity for data. π Harvard CS50 Undergrad igniting my passion for code. Currently delving into the MERN stack β because who doesn't love crafting seamless experiences from front to back? Join me on this exhilarating journey of embracing technology, penning insightful tech chronicles, and unraveling the mysteries of data! ππ§ Let's build, let's write, let's explore β all aboard the tech express! ππ #CodeAndCuriosity